Home » JavaScript Tutorial » JavaScript Comma Operator

JavaScript Comma Operator
Summary : in this tutorial, you’ll learn about the JavaScript comma operator and its usage.
Introduction to the JavaScript comma operator
JavaScript uses a comma ( , ) to represent the comma operator. A comma operator takes two expressions, evaluates them from left to right, and returns the value of the right expression.
Here’s the syntax of the comma operator:
For example:
In this example, the 10, 10+20 returns the value of the right expression, which is 10+20. Therefore, the result value is 30.
See the following example:
In this example, we increase the value of x by one ( x++ ), add one to x ( x+1 ) and assign x to y . Therefore, x is 11 , and y is 12 after the statement.
However, to make the code more explicit, you can use two statements rather than one statement with a comma operator like this:
This code is more explicit.
In practice, you might want to use the comma operator inside a for loop to update multiple variables each time through the loop.
The following example uses the comma operator in a for loop to display an array of nine elements as a matrix of 3 rows and three columns:
How it works.
- The code begins by defining an array called board with values from 1 to 9. This array represents a simple board or grid.
- The code then initializes an empty string s and enters a for loop.
- Inside the loop, two variables are declared: i and j . i is used to iterate over the elements of the board array, and j is a counter that increments with each iteration.
- Within the loop, each element of the board array is appended to the string s followed by a space.
- After appending an element to the string, the code checks if j (the counter) is divisible evenly by 3 using the modulus operator ( % ). This condition ( j % 3 == 0 ) checks if the current iteration is the third element.
- If the condition is true (meaning it’s the third element), the current string s is printed to the console using console.log(s) . This effectively prints a row of three elements from the board .
- The string s is then reset to an empty string for the next row.
- The loop continues until all elements of the board array have been processed.
- The final output is a series of rows, each containing three elements from the board array.
- A comma operator takes two expressions, evaluates them from left to right, and returns the value of the right expression.
- Use the comma operator ( , ) inside a for loop to update multiple variables once.
- Use two statements rather than the comma operator elsewhere to make the code more explicit and easier to understand.
- Skip to main content
- Select language
- Skip to search
- Expressions and operators
- Operator precedence
Left-hand-side expressions
« Previous Next »
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more.
A complete and detailed list of operators and expressions is also available in the reference .
JavaScript has the following types of operators. This section describes the operators and contains information about operator precedence.
- Assignment operators
- Comparison operators
- Arithmetic operators
- Bitwise operators
Logical operators
String operators, conditional (ternary) operator.
- Comma operator
Unary operators
- Relational operator
JavaScript has both binary and unary operators, and one special ternary operator, the conditional operator. A binary operator requires two operands, one before the operator and one after the operator:
For example, 3+4 or x*y .
A unary operator requires a single operand, either before or after the operator:
For example, x++ or ++x .
An assignment operator assigns a value to its left operand based on the value of its right operand. The simple assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x .
There are also compound assignment operators that are shorthand for the operations listed in the following table:
Destructuring
For more complex assignments, the destructuring assignment syntax is a JavaScript expression that makes it possible to extract data from arrays or objects using a syntax that mirrors the construction of array and object literals.
A comparison operator compares its operands and returns a logical value based on whether the comparison is true. The operands can be numerical, string, logical, or object values. Strings are compared based on standard lexicographical ordering, using Unicode values. In most cases, if the two operands are not of the same type, JavaScript attempts to convert them to an appropriate type for the comparison. This behavior generally results in comparing the operands numerically. The sole exceptions to type conversion within comparisons involve the === and !== operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality. The following table describes the comparison operators in terms of this sample code:
Note: ( => ) is not an operator, but the notation for Arrow functions .
An arithmetic operator takes numerical values (either literals or variables) as their operands and returns a single numerical value. The standard arithmetic operators are addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). These operators work as they do in most other programming languages when used with floating point numbers (in particular, note that division by zero produces Infinity ). For example:
In addition to the standard arithmetic operations (+, -, * /), JavaScript provides the arithmetic operators listed in the following table:
A bitwise operator treats their operands as a set of 32 bits (zeros and ones), rather than as decimal, hexadecimal, or octal numbers. For example, the decimal number nine has a binary representation of 1001. Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
The following table summarizes JavaScript's bitwise operators.
Bitwise logical operators
Conceptually, the bitwise logical operators work as follows:
- The operands are converted to thirty-two-bit integers and expressed by a series of bits (zeros and ones). Numbers with more than 32 bits get their most significant bits discarded. For example, the following integer with more than 32 bits will be converted to a 32 bit integer: Before: 11100110111110100000000000000110000000000001 After: 10100000000000000110000000000001
- Each bit in the first operand is paired with the corresponding bit in the second operand: first bit to first bit, second bit to second bit, and so on.
- The operator is applied to each pair of bits, and the result is constructed bitwise.
For example, the binary representation of nine is 1001, and the binary representation of fifteen is 1111. So, when the bitwise operators are applied to these values, the results are as follows:
Note that all 32 bits are inverted using the Bitwise NOT operator, and that values with the most significant (left-most) bit set to 1 represent negative numbers (two's-complement representation).
Bitwise shift operators
The bitwise shift operators take two operands: the first is a quantity to be shifted, and the second specifies the number of bit positions by which the first operand is to be shifted. The direction of the shift operation is controlled by the operator used.
Shift operators convert their operands to thirty-two-bit integers and return a result of the same type as the left operand.
The shift operators are listed in the following table.
Logical operators are typically used with Boolean (logical) values; when they are, they return a Boolean value. However, the && and || operators actually return the value of one of the specified operands, so if these operators are used with non-Boolean values, they may return a non-Boolean value. The logical operators are described in the following table.
Examples of expressions that can be converted to false are those that evaluate to null, 0, NaN, the empty string (""), or undefined.
The following code shows examples of the && (logical AND) operator.
The following code shows examples of the || (logical OR) operator.
The following code shows examples of the ! (logical NOT) operator.
Short-circuit evaluation
As logical expressions are evaluated left to right, they are tested for possible "short-circuit" evaluation using the following rules:
- false && anything is short-circuit evaluated to false.
- true || anything is short-circuit evaluated to true.
The rules of logic guarantee that these evaluations are always correct. Note that the anything part of the above expressions is not evaluated, so any side effects of doing so do not take effect.
In addition to the comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values together, returning another string that is the union of the two operand strings.
For example,
The shorthand assignment operator += can also be used to concatenate strings.
The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two values based on a condition. The syntax is:
If condition is true, the operator has the value of val1 . Otherwise it has the value of val2 . You can use the conditional operator anywhere you would use a standard operator.
This statement assigns the value "adult" to the variable status if age is eighteen or more. Otherwise, it assigns the value "minor" to status .
The comma operator ( , ) simply evaluates both of its operands and returns the value of the last operand. This operator is primarily used inside a for loop, to allow multiple variables to be updated each time through the loop.
For example, if a is a 2-dimensional array with 10 elements on a side, the following code uses the comma operator to update two variables at once. The code prints the values of the diagonal elements in the array:
A unary operation is an operation with only one operand.
The delete operator deletes an object, an object's property, or an element at a specified index in an array. The syntax is:
where objectName is the name of an object, property is an existing property, and index is an integer representing the location of an element in an array.
The fourth form is legal only within a with statement, to delete a property from an object.
You can use the delete operator to delete variables declared implicitly but not those declared with the var statement.
If the delete operator succeeds, it sets the property or element to undefined . The delete operator returns true if the operation is possible; it returns false if the operation is not possible.
Deleting array elements
When you delete an array element, the array length is not affected. For example, if you delete a[3] , a[4] is still a[4] and a[3] is undefined.
When the delete operator removes an array element, that element is no longer in the array. In the following example, trees[3] is removed with delete . However, trees[3] is still addressable and returns undefined .
If you want an array element to exist but have an undefined value, use the undefined keyword instead of the delete operator. In the following example, trees[3] is assigned the value undefined , but the array element still exists:
The typeof operator is used in either of the following ways:
The typeof operator returns a string indicating the type of the unevaluated operand. operand is the string, variable, keyword, or object for which the type is to be returned. The parentheses are optional.
Suppose you define the following variables:
The typeof operator returns the following results for these variables:
For the keywords true and null , the typeof operator returns the following results:
For a number or string, the typeof operator returns the following results:
For property values, the typeof operator returns the type of value the property contains:
For methods and functions, the typeof operator returns results as follows:
For predefined objects, the typeof operator returns results as follows:
The void operator is used in either of the following ways:
The void operator specifies an expression to be evaluated without returning a value. expression is a JavaScript expression to evaluate. The parentheses surrounding the expression are optional, but it is good style to use them.
You can use the void operator to specify an expression as a hypertext link. The expression is evaluated but is not loaded in place of the current document.
The following code creates a hypertext link that does nothing when the user clicks it. When the user clicks the link, void(0) evaluates to undefined , which has no effect in JavaScript.
The following code creates a hypertext link that submits a form when the user clicks it.
Relational operators
A relational operator compares its operands and returns a Boolean value based on whether the comparison is true.
The in operator returns true if the specified property is in the specified object. The syntax is:
where propNameOrNumber is a string or numeric expression representing a property name or array index, and objectName is the name of an object.
The following examples show some uses of the in operator.
The instanceof operator returns true if the specified object is of the specified object type. The syntax is:
where objectName is the name of the object to compare to objectType , and objectType is an object type, such as Date or Array .
Use instanceof when you need to confirm the type of an object at runtime. For example, when catching exceptions, you can branch to different exception-handling code depending on the type of exception thrown.
For example, the following code uses instanceof to determine whether theDay is a Date object. Because theDay is a Date object, the statements in the if statement execute.
The precedence of operators determines the order they are applied when evaluating an expression. You can override operator precedence by using parentheses.
The following table describes the precedence of operators, from highest to lowest.
A more detailed version of this table, complete with links to additional details about each operator, may be found in JavaScript Reference .
- Expressions
An expression is any valid unit of code that resolves to a value.
Every syntactically valid expression resolves to some value but conceptually, there are two types of expressions: with side effects (for example: those that assign value to a variable) and those that in some sense evaluates and therefore resolves to value.
The expression x = 7 is an example of the first type. This expression uses the = operator to assign the value seven to the variable x . The expression itself evaluates to seven.
The code 3 + 4 is an example of the second expression type. This expression uses the + operator to add three and four together without assigning the result, seven, to a variable. JavaScript has the following expression categories:
- Arithmetic: evaluates to a number, for example 3.14159. (Generally uses arithmetic operators .)
- String: evaluates to a character string, for example, "Fred" or "234". (Generally uses string operators .)
- Logical: evaluates to true or false. (Often involves logical operators .)
- Primary expressions: Basic keywords and general expressions in JavaScript.
- Left-hand-side expressions: Left values are the destination of an assignment.
Primary expressions
Basic keywords and general expressions in JavaScript.
Use the this keyword to refer to the current object. In general, this refers to the calling object in a method. Use this either with the dot or the bracket notation:
Suppose a function called validate validates an object's value property, given the object and the high and low values:
You could call validate in each form element's onChange event handler, using this to pass it the form element, as in the following example:
- Grouping operator
The grouping operator ( ) controls the precedence of evaluation in expressions. For example, you can override multiplication and division first, then addition and subtraction to evaluate addition first.
Comprehensions
Comprehensions are an experimental JavaScript feature, targeted to be included in a future ECMAScript version. There are two versions of comprehensions:
Comprehensions exist in many programming languages and allow you to quickly assemble a new array based on an existing one, for example.
Left values are the destination of an assignment.
You can use the new operator to create an instance of a user-defined object type or of one of the built-in object types. Use new as follows:
The super keyword is used to call functions on an object's parent. It is useful with classes to call the parent constructor, for example.
Spread operator
The spread operator allows an expression to be expanded in places where multiple arguments (for function calls) or multiple elements (for array literals) are expected.
Example: Today if you have an array and want to create a new array with the existing one being part of it, the array literal syntax is no longer sufficient and you have to fall back to imperative code, using a combination of push , splice , concat , etc. With spread syntax this becomes much more succinct:
Similarly, the spread operator works with function calls:
Document Tags and Contributors
- l10n:priority
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Legacy generator function expression
- Logical Operators
- Object initializer
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
JavaScript disabled. A lot of the features of the site won't work. Find out how to turn on JavaScript HERE .

Comma(,) special operator JS Home << JS Reference << Comma(,)
- << String
- Conditional >>
Expression operator.
Description
The Comma (,) special operator allows use of mutiple expressions where an expression is required.
Following is an examples of the Comma (,) special operator, which changes two expressions counters to produce part of the 9 times table.
Press the button below to action the above code:
Basic operators, maths
We know many operators from school. They are things like addition + , multiplication * , subtraction - , and so on.
In this chapter, we’ll start with simple operators, then concentrate on JavaScript-specific aspects, not covered by school arithmetic.
Terms: “unary”, “binary”, “operand”
Before we move on, let’s grasp some common terminology.
An operand – is what operators are applied to. For instance, in the multiplication of 5 * 2 there are two operands: the left operand is 5 and the right operand is 2 . Sometimes, people call these “arguments” instead of “operands”.
An operator is unary if it has a single operand. For example, the unary negation - reverses the sign of a number:
An operator is binary if it has two operands. The same minus exists in binary form as well:
Formally, in the examples above we have two different operators that share the same symbol: the negation operator, a unary operator that reverses the sign, and the subtraction operator, a binary operator that subtracts one number from another.
The following math operations are supported:
- Addition + ,
- Subtraction - ,
- Multiplication * ,
- Division / ,
- Remainder % ,
- Exponentiation ** .
The first four are straightforward, while % and ** need a few words about them.
Remainder %
The remainder operator % , despite its appearance, is not related to percents.
The result of a % b is the remainder of the integer division of a by b .
For instance:
Exponentiation **
The exponentiation operator a ** b raises a to the power of b .
In school maths, we write that as a b .
Just like in maths, the exponentiation operator is defined for non-integer numbers as well.
For example, a square root is an exponentiation by ½:
String concatenation with binary +
Let’s meet the features of JavaScript operators that are beyond school arithmetics.
Usually, the plus operator + sums numbers.
But, if the binary + is applied to strings, it merges (concatenates) them:
Note that if any of the operands is a string, then the other one is converted to a string too.
For example:
See, it doesn’t matter whether the first operand is a string or the second one.
Here’s a more complex example:
Here, operators work one after another. The first + sums two numbers, so it returns 4 , then the next + adds the string 1 to it, so it’s like 4 + '1' = '41' .
Here, the first operand is a string, the compiler treats the other two operands as strings too. The 2 gets concatenated to '1' , so it’s like '1' + 2 = "12" and "12" + 2 = "122" .
The binary + is the only operator that supports strings in such a way. Other arithmetic operators work only with numbers and always convert their operands to numbers.
Here’s the demo for subtraction and division:
Numeric conversion, unary +
The plus + exists in two forms: the binary form that we used above and the unary form.
The unary plus or, in other words, the plus operator + applied to a single value, doesn’t do anything to numbers. But if the operand is not a number, the unary plus converts it into a number.
It actually does the same thing as Number(...) , but is shorter.
The need to convert strings to numbers arises very often. For example, if we are getting values from HTML form fields, they are usually strings. What if we want to sum them?
The binary plus would add them as strings:
If we want to treat them as numbers, we need to convert and then sum them:
From a mathematician’s standpoint, the abundance of pluses may seem strange. But from a programmer’s standpoint, there’s nothing special: unary pluses are applied first, they convert strings to numbers, and then the binary plus sums them up.
Why are unary pluses applied to values before the binary ones? As we’re going to see, that’s because of their higher precedence .
Operator precedence
If an expression has more than one operator, the execution order is defined by their precedence , or, in other words, the default priority order of operators.
From school, we all know that the multiplication in the expression 1 + 2 * 2 should be calculated before the addition. That’s exactly the precedence thing. The multiplication is said to have a higher precedence than the addition.
Parentheses override any precedence, so if we’re not satisfied with the default order, we can use them to change it. For example, write (1 + 2) * 2 .
There are many operators in JavaScript. Every operator has a corresponding precedence number. The one with the larger number executes first. If the precedence is the same, the execution order is from left to right.
Here’s an extract from the precedence table (you don’t need to remember this, but note that unary operators are higher than corresponding binary ones):
As we can see, the “unary plus” has a priority of 14 which is higher than the 11 of “addition” (binary plus). That’s why, in the expression "+apples + +oranges" , unary pluses work before the addition.
Let’s note that an assignment = is also an operator. It is listed in the precedence table with the very low priority of 2 .
That’s why, when we assign a variable, like x = 2 * 2 + 1 , the calculations are done first and then the = is evaluated, storing the result in x .
Assignment = returns a value
The fact of = being an operator, not a “magical” language construct has an interesting implication.
All operators in JavaScript return a value. That’s obvious for + and - , but also true for = .
The call x = value writes the value into x and then returns it .
Here’s a demo that uses an assignment as part of a more complex expression:
In the example above, the result of expression (a = b + 1) is the value which was assigned to a (that is 3 ). It is then used for further evaluations.
Funny code, isn’t it? We should understand how it works, because sometimes we see it in JavaScript libraries.
Although, please don’t write the code like that. Such tricks definitely don’t make code clearer or readable.
Chaining assignments
Another interesting feature is the ability to chain assignments:
Chained assignments evaluate from right to left. First, the rightmost expression 2 + 2 is evaluated and then assigned to the variables on the left: c , b and a . At the end, all the variables share a single value.
Once again, for the purposes of readability it’s better to split such code into few lines:
That’s easier to read, especially when eye-scanning the code fast.
Modify-in-place
We often need to apply an operator to a variable and store the new result in that same variable.
This notation can be shortened using the operators += and *= :
Short “modify-and-assign” operators exist for all arithmetical and bitwise operators: /= , -= , etc.
Such operators have the same precedence as a normal assignment, so they run after most other calculations:
Increment/decrement
Increasing or decreasing a number by one is among the most common numerical operations.
So, there are special operators for it:
Increment ++ increases a variable by 1:
Decrement -- decreases a variable by 1:
Increment/decrement can only be applied to variables. Trying to use it on a value like 5++ will give an error.
The operators ++ and -- can be placed either before or after a variable.
- When the operator goes after the variable, it is in “postfix form”: counter++ .
- The “prefix form” is when the operator goes before the variable: ++counter .
Both of these statements do the same thing: increase counter by 1 .
Is there any difference? Yes, but we can only see it if we use the returned value of ++/-- .
Let’s clarify. As we know, all operators return a value. Increment/decrement is no exception. The prefix form returns the new value while the postfix form returns the old value (prior to increment/decrement).
To see the difference, here’s an example:
In the line (*) , the prefix form ++counter increments counter and returns the new value, 2 . So, the alert shows 2 .
Now, let’s use the postfix form:
In the line (*) , the postfix form counter++ also increments counter but returns the old value (prior to increment). So, the alert shows 1 .
To summarize:
If the result of increment/decrement is not used, there is no difference in which form to use:
If we’d like to increase a value and immediately use the result of the operator, we need the prefix form:
If we’d like to increment a value but use its previous value, we need the postfix form:
The operators ++/-- can be used inside expressions as well. Their precedence is higher than most other arithmetical operations.
Compare with:
Though technically okay, such notation usually makes code less readable. One line does multiple things – not good.
While reading code, a fast “vertical” eye-scan can easily miss something like counter++ and it won’t be obvious that the variable increased.
We advise a style of “one line – one action”:
Bitwise operators
Bitwise operators treat arguments as 32-bit integer numbers and work on the level of their binary representation.
These operators are not JavaScript-specific. They are supported in most programming languages.
The list of operators:
- AND ( & )
- LEFT SHIFT ( << )
- RIGHT SHIFT ( >> )
- ZERO-FILL RIGHT SHIFT ( >>> )
These operators are used very rarely, when we need to fiddle with numbers on the very lowest (bitwise) level. We won’t need these operators any time soon, as web development has little use of them, but in some special areas, such as cryptography, they are useful. You can read the Bitwise Operators chapter on MDN when a need arises.
The comma operator , is one of the rarest and most unusual operators. Sometimes, it’s used to write shorter code, so we need to know it in order to understand what’s going on.
The comma operator allows us to evaluate several expressions, dividing them with a comma , . Each of them is evaluated but only the result of the last one is returned.
Here, the first expression 1 + 2 is evaluated and its result is thrown away. Then, 3 + 4 is evaluated and returned as the result.
Please note that the comma operator has very low precedence, lower than = , so parentheses are important in the example above.
Without them: a = 1 + 2, 3 + 4 evaluates + first, summing the numbers into a = 3, 7 , then the assignment operator = assigns a = 3 , and the rest is ignored. It’s like (a = 1 + 2), 3 + 4 .
Why do we need an operator that throws away everything except the last expression?
Sometimes, people use it in more complex constructs to put several actions in one line.
Such tricks are used in many JavaScript frameworks. That’s why we’re mentioning them. But usually they don’t improve code readability so we should think well before using them.
The postfix and prefix forms
What are the final values of all variables a , b , c and d after the code below?
The answer is:
Assignment result
What are the values of a and x after the code below?
- a = 4 (multiplied by 2)
- x = 5 (calculated as 1 + 4)
Type conversions
What are results of these expressions?
Think well, write down and then compare with the answer.
- The addition with a string "" + 1 converts 1 to a string: "" + 1 = "1" , and then we have "1" + 0 , the same rule is applied.
- The subtraction - (like most math operations) only works with numbers, it converts an empty string "" to 0 .
- The addition with a string appends the number 5 to the string.
- The subtraction always converts to numbers, so it makes " -9 " a number -9 (ignoring spaces around it).
- null becomes 0 after the numeric conversion.
- undefined becomes NaN after the numeric conversion.
- Space characters are trimmed off string start and end when a string is converted to a number. Here the whole string consists of space characters, such as \t , \n and a “regular” space between them. So, similarly to an empty string, it becomes 0 .
Fix the addition
Here’s a code that asks the user for two numbers and shows their sum.
It works incorrectly. The output in the example below is 12 (for default prompt values).
Why? Fix it. The result should be 3 .
The reason is that prompt returns user input as a string.
So variables have values "1" and "2" respectively.
What we should do is to convert strings to numbers before + . For example, using Number() or prepending them with + .
For example, right before prompt :
Or in the alert :
Using both unary and binary + in the latest code. Looks funny, doesn’t it?
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
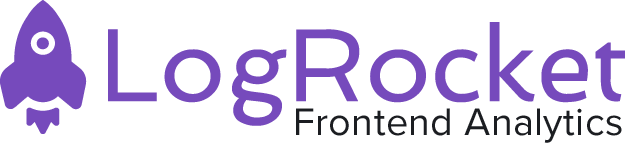
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Best practices for using trailing commas in JavaScript
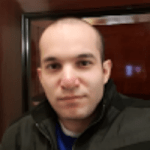
A trailing comma, also known as a dangling or terminal comma, is a comma symbol that is typed after the last item of a list of elements. Since the introduction of the JavaScript language , trailing commas have been legal in array literals. Later, object literals joined arrays. And with the introduction of ES2017, also known as ES8, trailing commas became allowed pretty much everywhere.
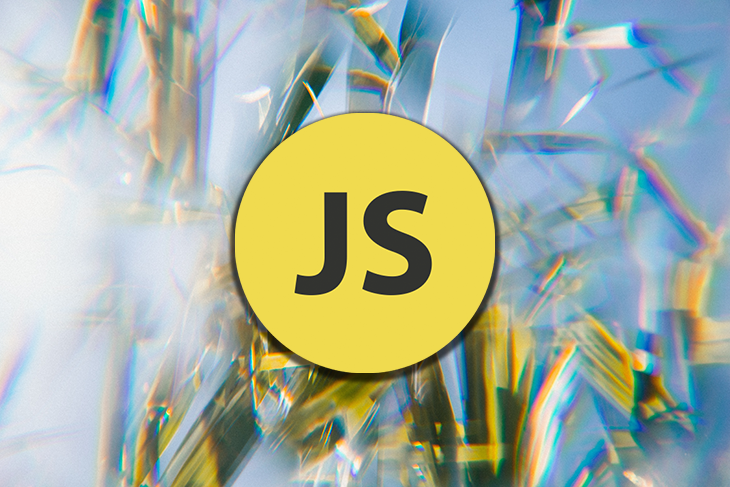
It seems like a little change, but there are some hard-to-notice consequences. And while most new language features are welcomed, this one can land you in trouble if you’re not careful.
In this guide, we’ll look at trailing commas in detail. We’ll start with the common data types, like arrays and objects, where you can safely add a trailing comma to the end of a list of items. Then we’ll move on to language constructs such as parameter lists, function calls, and destructuring assignments. At the end of the article, we’ll discuss the dos and don’ts of using trailing commas.
Using trailing commas in arrays
You can safely include a trailing comma after the last expression in an array like this:
Be careful not to add more than one comma to the end, or you’ll be creating an undefined element. For example, the following array is perfectly legal but contains four elements:
Arrays don’t necessarily have contiguous indices starting at 0 . You can create an array that contains several “gaps” — such an array is known as a sparse array . For instance, the following array contains six elements, three of which are undefined :
So, it’s important to remember that the value of the length property doesn’t always indicate the number of elements in the array. You may even have an array that has no elements and a length of 1 :
In practice, however, you rarely need to work with a sparse array. And if you do, your existing code will most likely handle it just as it would handle a normal array containing undefined elements.
Using trailing commas in objects
Similar to arrays, you can have a comma following the last property of an object:
Trailing commas in object literals have been legal since ECMAScript 5. Some JavaScript style guides, like those created by Airbnb and Google , even encourage you to make a habit of including a trailing comma all the time so that you’re less likely to encounter a syntax error when adding a new property at the end of an object at a later time.
Note that, unlike with arrays, you cannot create sparse objects, and attempting to do so results in a syntax error:
As I mentioned before, there are several other constructs in JavaScript besides arrays and objects that may have a trailing comma.

Using trailing commas in parameter lists and function calls
It’s sometimes useful to put the parameters of a function on a separate line, particularly if there’s a long list of parameters or you want to accommodate comments describing each parameter. For example:
As the function evolves, you may find yourself in a situation where you need to add more parameters to the function. But for each new parameter you add, you have to go to the previous line and add a comma:
Even experienced developers do not always remember to add a comma to the previous line, which results in an error. What’s worse is that the commit diffs will show a code change in that line simply because you later added a comma (more on this later).
Fortunately, ES2017 made it legal to add a trailing comma to function parameters, too:
This is just a change in the coding style and doesn’t add an unnamed parameter or cause any other side effect.
What’s more, the ES2017 update gave us the ability to have a trailing comma at the ends of arguments in function calls. Some programmers like to put each argument of a function call on its own line. If you’re one of them, then trailing commas, again, will save you from potential errors in the future:
This code calls the createRectangle() function with two arguments. If you later decide to add a third argument, you won’t have to edit any existing line. The same rule applies to method definitions for classes or objects since they’re also functions:
Using trailing commas in the destructuring assignment syntax
The destructuring assignment syntax allows you to quickly extract values from arrays or objects into distinct variables. When destructuring, you can add a trailing comma to the left-hand side of the assignment. For instance, the following code destructures an array:
Similarly, you can use destructuring to “unpack” the properties of an object:
But what about JSON objects, which are similar to plain JavaScript objects? Can they use trailing commas?
Using trailing commas in JSON
The JSON file format was introduced in the early 2000s. Since JSON is based on JavaScript’s object syntax, and it was invented before ECMAScript 5 was introduced in 2009, trailing commas cannot be used in JSON (remember, trailing commas in object literals became legal in ES5).
For example, the following code will throw an error:
So does this line of code:
There are a number of online tools that can help you with this problem. For example, you can take advantage of this JSON Formatter to automatically find and remove trailing commas in your JSON code.
Trailing commas in module imports and exports
In modern JavaScript, it’s a common practice to create programs that are composed of independent chunks of code called modules. Just as it’s legal to add a trailing comma to objects in classic scripts, it’s legal to have a comma following the last item of exports in modules. This comes in handy when you want to include more exports at a later time. For example:
This code uses the export keyword to make the foo , bar , and baz variables public. This means other modules in separate files can use the import statement to access these variables:
Why should you start using trailing commas?
JavaScript programmers used to avoid including a trailing comma in arrays because early versions of Internet Explorer would throw an error (even though it was legal in JavaScript from the beginning). But things have changed. Many coding styles now recommend using trailing commas all the time, and there are good reasons for that.
If you frequently add new items to the ends of arrays, objects, or argument/parameter lists, then having a trailing comma already in place means you won’t have to remember to go to the line before and add a comma if you need to make an addition later.
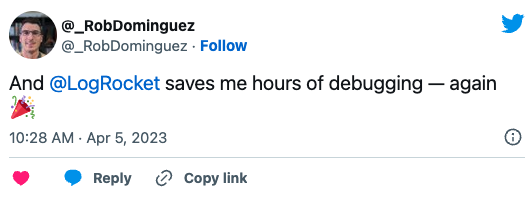
Over 200k developers use LogRocket to create better digital experiences

You may also find yourself frequently cutting and pasting properties. Again, having a trailing comma could make reordering the items less troublesome and prevent syntax errors in the future.
Moreover, because you won’t need to change the line that used to be the last item, version control systems would produce cleaner diffs. Say you have this function:
If you add a new parameter called p3 , the diff output would look something like:
Here, you have two changes in the function declaration and two changes in the function invocation. Let’s see what would happen if your function already had a trailing comma:
With a trailing comma in place, you’ll only have two changes in the diff output:
The takeaway from this section is that using trailing commas makes it easier to add new parameters to your functions or copy/paste properties in arrays and objects. It also helps with producing cleaner diff output.
But trailing commas do not work everywhere, and if you’re not careful, using them might actually backfire.
When not to use trailing commas
You might assume that you can use trailing commas with the rest parameter syntax, too, because trailing commas are allowed in various other JavaScript constructs. But that is actually not true:
Using a trailing comma after the rest parameter is illegal, even if you use it in the destructuring syntax:
So, keep in mind that although using a trailing comma in destructuring syntax is valid, you cannot use it after the rest parameter.
Besides the destructuring syntax, there’s one more place where using a trailing comma may land you in trouble: functions. Consider the following example:
The first line of this code defines a function with no parameter and a comma, and that causes a SyntaxError . You can either have no parameters with no comma, or a trailing comma after parameters. The same is true when you invoke a function: you cannot have a function call whose only argument is a comma.
The usage of the comma symbol has undergone several revisions in the JavaScript language, and with each revision , more language constructs have added support for trailing commas. In this article, we looked at how the trailing comma works in different constructs, including arrays, objects, JSON objects, parameter lists, function calls, and module imports and exports.
Then, we learned where the trailing comma is legal to use and where it’s not. In general, you should make use of trailing commas when you frequently copy/paste properties or add new items to the end of a list. You can also take advantage of them to produce cleaner diff outputs. But, remember, you should not use them with the rest parameter syntax, and you cannot have a function declaration/invocation whose only parameter is a comma.
LogRocket : Debug JavaScript errors more easily by understanding the context
Debugging code is always a tedious task. But the more you understand your errors, the easier it is to fix them.
LogRocket allows you to understand these errors in new and unique ways. Our frontend monitoring solution tracks user engagement with your JavaScript frontends to give you the ability to see exactly what the user did that led to an error.
LogRocket records console logs, page load times, stack traces, slow network requests/responses with headers + bodies, browser metadata, and custom logs. Understanding the impact of your JavaScript code will never be easier!
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #vanilla javascript
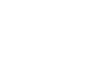
Stop guessing about your digital experience with LogRocket
Recent posts:.
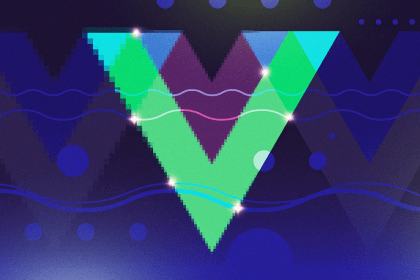
Optimizing rendering in Vue
This guide covers methods for enhancing rendering speed in Vue.js apps using functions and techniques like `v-once`, `v-for`, `v-if`, and `v-show`.
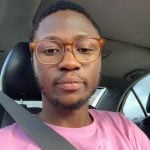
Using Google Magika to build an AI-powered file type detector
Magika offers extremely accurate file type identification, using deep learning to address the limitations of traditional methods.

Unistyles vs. Tamagui for cross-platform React Native styles
Unistyles and Tamagui both help address the challenges of creating consistent and responsive styles across different devices.
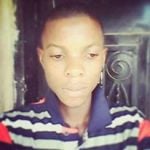
Biome adoption guide: Overview, examples, and alternatives
Biome combines linters and formatters into one tools, helping developers write better code faster and with less setup and configuration.
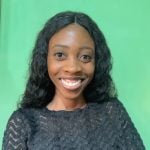
2 Replies to "Best practices for using trailing commas in JavaScript"
👍🏽 nice summary. Thanks 🙏🏽.
Very bikeshed. The only meaningful arguments I’ve ever heard in support of the practice are “it makes templating and transformation by regex easier.” The compiler certainly doesn’t care in operational cases.
“Cleaner” diffs are an opinion on the order of “clean code,” albeit with marginally superior provenance. If you’ve seen a few diffs, you know what you’re looking at from experience. If you haven’t, the reduction is fairly obvious.
There’s a stronger argument to be made here for left-sided dots and commas. All the benefits if you care, no fashion characters.
Leave a Reply Cancel reply
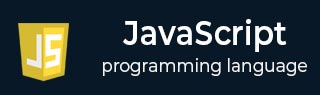
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Garbage Collection
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- ECMAScript 2023
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Generators
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Random Number
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - Export and Import
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Execution Context
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Design Patterns
- JavaScript - Reference Type
- JavaScript - LocalStorage
- JavaScript - SessionStorage
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Common Mistakes
- JavaScript - Performance
- JavaScript - Best Practices
- JavaScript - Style Guide
- JavaScript - Ninja Code
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Resources
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
JavaScript Comma Operator
The comma operator (,) in JavaScript evaluates the multiple expression from left to right. You can use the resultant value of the left expression as an input of the right expression. After evaluating all expressions, it returns the resultant value of the rightmost expression.
However, the comma operator is also used in the 'for' loop, array, object, etc. In this chapter, you will learn all the use cases of the comma operator.
You should follow the below syntax to use the comma expression to evaluate multiple expressions.
Return value
It returns the resultant value of the last expression only.
Let's understand the JavaScript comma operator in details with the help of some exmaples
Example: The Comma Operator with Strings
In the example below, we have added the 4 comma seprated strings in the braces. Here, each string works as an expression. The code will evaluate the string and return the last string. In the output, you can see that it prints the 'CSS' as it is the rightmost string.
Example: The Comma Operator with Expressions
In the example below, we have defined the variable 'a' and initialized it with 5. In the 'ans' variable, we store the resultant value the comma operator returns. The first expression updates the value of a to 8, the second expression increments the value of a by 1, and the third expression adds 2 to the updated value of the variable 'a'.
The value of the 'ans' is 11, which is returned by the rightmost expression of the comma operator.
Example: The comma operator with functions
In the example below, we have defined the first() and second() functions. Also, it prints the message and returns the value from the function according to the function name.
We use the comma operator to execute the multiple functions. In the output, you can see that it invokes both functions but prints the returned value from the second() function only.
Description
Specifications, browser compatibility.
The comma operator evaluates each of its operands (from left to right) and returns the value of the last operand.
The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/interactive-examples and send us a pull request.
You can use the comma operator when you want to include multiple expressions in a location that requires a single expression. The most common usage of this operator is to supply multiple parameters in a for loop.
The comma operator is fully different from the comma within arrays, objects, and function arguments and parameters.
If a is a 2-dimensional array with 10 elements on each side, the following code uses the comma operator to increment i and decrement j at once.
The following code prints the values of the diagonal elements in the array:
Note that the comma operators in assignments may appear not to have the normal effect of comma operators because they don't exist within an expression. In the following example, a is set to the value of b = 3 (which is 3), but the c = 4 expression still evaluates and its result returned to console (i.e., 4). This is due to operator precedence and associativity .
Processing and then returning
Another example that one could make with comma operator is processing before returning. As stated, only the last element will be returned but all others are going to be evaluated as well. So, one could do:
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.ListFormat
- Intl.Locale
- Intl.NumberFormat
- Intl.PluralRules
- Intl.RelativeTimeFormat
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comparison operators
- Conditional (ternary) operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical operators
- Object initializer
- Operator precedence
- (currently at stage 1) pipes the value of an expression into a function. This allows the creation of chained function calls in a readable manner. The result is syntactic sugar in which a function call with a single argument can be written like this:">Pipeline operator
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for await...of
- for each...in
- function declaration
- import.meta
- try...catch
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration`X' before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: 'x' is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use 'in' operator to search for 'x' in 'y'
- TypeError: cyclic object value
- TypeError: invalid 'instanceof' operand 'x'
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- X.prototype.y called on incompatible type
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Tutorial
JavaScript Basics
- Introduction to JavaScript
- JavaScript Versions
- How to Add JavaScript in HTML Document?
- JavaScript Statements
- JavaScript Syntax
- JavaScript Output
- JavaScript Comments
JS Variables & Datatypes
- Variables and Datatypes in JavaScript
- Global and Local variables in JavaScript
- JavaScript Let
- JavaScript Const
- JavaScript var
JS Operators
- JavaScript Operators
- Operator precedence in JavaScript
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
- JavaScript Bitwise Operators
- JavaScript Ternary Operator
JavaScript Comma Operator
- JavaScript Unary Operators
- JavaScript Relational operators
- JavaScript String Operators
- JavaScript Loops
- 7 Loops of JavaScript
- JavaScript For Loop
- JavaScript While Loop
- JavaScript for-in Loop
- JavaScript for...of Loop
- JavaScript do...while Loop
JS Perfomance & Debugging
- JavaScript | Performance
- Debugging in JavaScript
- JavaScript Errors Throw and Try to Catch
- Objects in Javascript
- Introduction to Object Oriented Programming in JavaScript
- JavaScript Objects
- Creating objects in JavaScript (4 Different Ways)
- JavaScript JSON Objects
- JavaScript Object Reference
JS Function
- Functions in JavaScript
- How to write a function in JavaScript ?
- JavaScript Function Call
- Different ways of writing functions in JavaScript
- Difference between Methods and Functions in JavaScript
- Explain the Different Function States in JavaScript
- JavaScript Function Complete Reference
- JavaScript Arrays
- JavaScript Array Methods
- Best-Known JavaScript Array Methods
- What are the Important Array Methods of JavaScript ?
- JavaScript Array Reference
- JavaScript Strings
- JavaScript String Methods
- JavaScript String Reference
- JavaScript Numbers
- How numbers are stored in JavaScript ?
- How to create a Number object using JavaScript ?
- JavaScript Number Reference
- JavaScript Math Object
- What is the use of Math object in JavaScript ?
- JavaScript Math Reference
- JavaScript Map
- What is JavaScript Map and how to use it ?
- JavaScript Map Reference
- Sets in JavaScript
- How are elements ordered in a Set in JavaScript ?
- How to iterate over Set elements in JavaScript ?
- How to sort a set in JavaScript ?
- JavaScript Set Reference
- JavaScript Date
- JavaScript Promise
- JavaScript BigInt
- JavaScript Boolean
- JavaScript Proxy/Handler
- JavaScript WeakMap
- JavaScript WeakSet
- JavaScript Function Generator
- JavaScript JSON
- Arrow functions in JavaScript
- JavaScript this Keyword
- Strict mode in JavaScript
- Introduction to ES6
- JavaScript Hoisting
- Async and Await in JavaScript
JavaScript Exercises
- JavaScript Exercises, Practice Questions and Solutions
JavaScript Comma Operator mainly evaluates its operands from left to right sequentially and returns the value of the rightmost operand. It is used as a separator for multiple expressions at a place that requires a single expression. When a comma operator is placed in an expression, it executes each expression and returns the rightmost expression.
In the above syntax, multiple expressions are separated using a comma operator. During execution, each expression will be executed from left to right and the rightmost expression will be returned.
Example 1: Below is an example of the Comma operator.
Example 2: The most useful application of the comma operator is in loops. In loops, it is used to update multiple variables in the same expression.
Example 3: Using the comma operator to initialize multiple variables in a single statement.
We have a complete list of JavaScript Operators, to check those please go through the Javascript Operators Complete Reference article.
Supported Browsers
- Google Chrome
- Internet Explorer
- Apple Safari
Please Login to comment...
Similar reads.
- javascript-operators
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
possible duplicate of `x = y, z` comma assignment in JavaScript - Dagg Nabbit. Mar 18, 2013 at 20:11 @DaggNabbit No, it's not the comma operator - Bergi. Feb 14, 2021 at 21:31. ... In the context of a var declaration, the comma is just a separator between the list of variable initializations. It's not the comma operator; ...
Because commas have the lowest precedence — even lower than assignment — commas can be used to join multiple assignment expressions. In the following example, a is set to the value of b = 3 (which is 3). Then, the c = 4 expression evaluates and its result becomes the return value of the entire comma expression.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
If a is a 2-dimensional array with 10 elements on each side, the following code uses the comma operator to increment two variables at once. The following code prints the values of the diagonal elements in the array: for (var i = 0, j = 9; i <= 9; i++, j--) console.log('a[' + i + '][' + j + '] = ' + a[i][j]); Note that the comma in assignments ...
A comma operator takes two expressions, evaluates them from left to right, and returns the value of the right expression. Here's the syntax of the comma operator: For example: console .log(result); Code language: JavaScript (javascript) Output: In this example, the 10, 10+20 returns the value of the right expression, which is 10+20.
The shorthand assignment operator += can also be used to concatenate strings. For example, var mystring = 'alpha'; mystring += 'bet'; // evaluates to "alphabet" and assigns this value to mystring. Conditional (ternary) operator. The conditional operator is the only JavaScript operator that takes three operands. The operator can have one of two ...
JavaScript Basics JavaScript Basics JavaScript Intermediate JavaScript Intermediate JavaScript Advanced JavaScript Advanced JavaScript Reference JavaScript Entities Globals Statements Operators Arithmetic Assignment Bitwise Comparison Logical Member String Special Operators Comma(,) Conditional delete function get in instanceof new set this ...
In this example, we will start by declaring a variable with the name " x ", and assign it the value of 10. We then use JavaScript's addition assignment operator ( +=) to increase the value of our " x " variable by 11. let x = 10; x += 11; console.log( x); Copy. Below, you can see how this operator increased the value of our " x ...
An operator is binary if it has two operands. The same minus exists in binary form as well: let x = 1, y = 3; alert( y - x ); // 2, binary minus subtracts values. Formally, in the examples above we have two different operators that share the same symbol: the negation operator, a unary operator that reverses the sign, and the subtraction ...
Using trailing commas in the destructuring assignment syntax. The destructuring assignment syntax allows you to quickly extract values from arrays or objects into distinct variables. When destructuring, you can add a trailing comma to the left-hand side of the assignment. For instance, the following code destructures an array:
The comma operator (,) in JavaScript evaluates the multiple expression from left to right. You can use the resultant value of the left expression as an input of the right expression. After evaluating all expressions, it returns the resultant value of the rightmost expression. However, the comma operator is also used in the 'for' loop, array ...
The comma operator is fully different from the comma within arrays, objects, and function arguments and parameters. Examples. If a is a 2-dimensional array with 10 elements on each side, the following code uses the comma operator to increment i and decrement j at once. The following code prints the values of the diagonal elements in the array:
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
JavaScript Comma Operator. JavaScript Comma Operator mainly evaluates its operands from left to right sequentially and returns the value of the rightmost operand. It is used as a separator for multiple expressions at a place that requires a single expression. When a comma operator is placed in an expression, it executes each expression and ...
The comma operator ', ' evaluates each of its operands ( from left to right) and returns the value of the last operand.You can have multiple expressions evaluated with the final value been the value of the rightmost expression. let x = 9; x = (x++, x*2, x*3) // output: x = 30. This use of compound expressions and assignment is helpful in ...
Basic keywords and general expressions in JavaScript. These expressions have the highest precedence (higher than operators ). The this keyword refers to a special property of an execution context. Basic null, boolean, number, and string literals. Array initializer/literal syntax. Object initializer/literal syntax.
The comma operator chains multiple expressions together, and the result of the operation is the value of the last operand. The only real use for it is when you need multiple side effects to occur, such as assignment or function calls.
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables. ... // SyntaxError: rest element may not have a trailing comma // Always consider using rest operator as the last element. Examples. Array destructuring.