
public class Deque<Item> implements Iterable<Item> { // construct an empty deque public Deque() // is the deque empty? public boolean isEmpty() // return the number of items on the deque public int size() // add the item to the front public void addFirst(Item item) // add the item to the back public void addLast(Item item) // remove and return the item from the front public Item removeFirst() // remove and return the item from the back public Item removeLast() // return an iterator over items in order from front to back public Iterator<Item> iterator() // unit testing (required) public static void main(String[] args) }
public class RandomizedQueue<Item> implements Iterable<Item> { // construct an empty randomized queue public RandomizedQueue() // is the randomized queue empty? public boolean isEmpty() // return the number of items on the randomized queue public int size() // add the item public void enqueue(Item item) // remove and return a random item public Item dequeue() // return a random item (but do not remove it) public Item sample() // return an independent iterator over items in random order public Iterator<Item> iterator() // unit testing (required) public static void main(String[] args) }
~/Desktop/queues> cat distinct.txt A B C D E F G H I ~/Desktop/queues> java Permutation 3 C G A ~/Desktop/queues> java Permutation 3 E F G ~/Desktop/queues> cat duplicates.txt AA BB BB BB BB BB CC CC ~/Desktop/queues> java Permutation 8 BB AA BB CC BB BB CC BB
public class Permutation { public static void main(String[] args) }
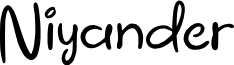

Niyander Tech
Learn with fun
- All Coursera Quiz Answers
Programming Assignment: Array and object iteration Solution
In this article i am gone to share Programming with JavaScript by meta Week 3 | Programming Assignment: Array and object iteration Solution with you..
Enroll Link: Programming with JavaScript
Visit this link: Programming Assignment: Building an object-oriented program Solution
Lab Instructions: Advanced JS Features
Tips: Before you Begin
To view your code and instructions side-by-side, select the following in your VSCode toolbar:
- View -> Editor Layout -> Two Columns
- To view this file in Preview mode, right click on this README.md file and Open Preview
- Select your code file in the code tree, which will open it up in a new VSCode tab.
- Drag your assessment code files over to the second column.
- Great work! You can now see instructions and code at the same time.
- Questions about using VSCode? Please see our support resources here: Visual Studio Code on Coursera
To run your JavaScript code
- Select your JavaScript file
- Select the “Run Code” button in the upper right hand toolbar of VSCode.
- Ex: It looks like a triangular “Play” button.
Task: Iterate Over an Array
In this exercise, you’ll use the for….of loop to iterate over an array and to iterate over an object’s own properties.
Step 1. You are given an array of dairy products:
The expected output should be:
Step 2. You are given the following starter code:
Step 3. Using the same starter code as in task 2, create a function called `animalCan` and within it, loop over all the properties in both the bird object and its prototype – the animal object – using the for…in loop.
Final Step: Let’s submit your code!
Nice work! To complete this assessment:
- Save your file through File -> Save
- Select “Submit Assignment” in your Lab toolbar.
Your code will be autograded and return feedback shortly on the “Grades” tab. You can also see your score in your Programming Assignment “My Submission” tab.
- Copy & paste this code on solution.js File..
- And save your file and then submit.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Greetings, Hey i am Niyander, and I hail from India, we strive to impart knowledge and offer assistance to those in need.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Iterate Over an Array
In this exercise, you'll use the for....of loop to iterate over an array and to iterate over an object's own properties.
Step 1. You are given an array of dairy products:
Create a function called logDairy. Within it, console log each of the items in the dairy array, using the for...of loop. The expected output should be:
Step 2. You are given the following starter code:
Create a function called birdCan , within it, loop over the bird object's properties and console log each one, using the for...of loop. Remember, you need to console log both the key and the value of each of the bird object's properties.
Step 3. Using the same starter code as in task 2, create a function called animalCan and within it, loop over all the properties in both the bird object and its prototype - the animal object - using the for...in loop.
// Task 1 function logDairy() { const logDairy = ['cheese', 'sour cream', 'milk', 'yogurt', 'ice cream', 'milkshake']; for (let i = 0; i < logDairy.length; i++) { console.log(logDairy[i]) } } logDairy(); // Task 2 function birdCan() { const animal = { canJump: true }; const bird = Object.create(animal); bird.canFly = true; bird.hasFeathers = true; for (prop of Object.keys(bird)) { console.log(prop + ":" + bird[prop]) } } birdCan(); // Task 3 function animalCan() { const animal = { canJump: true }; const bird = Object.create(animal); bird.canFly = true; bird.hasFeathers = true; for (prop in animal) { console.log(prop); } for (prop in bird) { console.log(prop); } } animalCan();
I have passed task 1 but not 2nd and 3rd
Passed: Console logged expected values for logDairy FAILED: Console logged expected values for birdCan - returned canFly:truehasFeathers:true but expected canFly: truehasFeathers: true FAILED: Console logged expected values for animalCan - returned canJumpcanFlyhasFeatherscanJump but expected canFly: truehasFeathers: truecanJump: true
tell me where did i go wrong?
- 1 Can you spot the difference between canFly:true , and canFly: true ? – C3roe Commented Nov 22, 2022 at 12:02

5 Answers 5
- As it’s currently written, your answer is unclear. Please edit to add additional details that will help others understand how this addresses the question asked. You can find more information on how to write good answers in the help center . – Community Bot Commented Nov 25, 2022 at 8:48

//Step:1 var dairy = ['cheese', 'sour cream', 'milk', 'yogurt', 'ice cream', 'milkshake'] // function to show the output function logDairy(product){ console.log(product) } // for of loop to iterate over the array for(product of dairy){ logDairy(product) } //Step:2 // in this step the condition was to loop over the object using for of loop // for of loop can only be used on arrays // so we will create an array or keys from the object using Object.key(obj) and use for of loop on the object, // we will receive a single key for each iteration, the key will be passed down to function as param // then we will use bracket notation to find the value of the key. // animal object const animal = { canJump: true }; // sending obj as param animalCan(animal) //bird object const bird = Object.create(animal); bird.canFly = true; bird.hasFeathers = true; // sending object as param animalCan(bird) // function to show key value from object function birdCan(prop){ console.log(`${prop}: ${bird[prop]}`) } // for of loop to iterate over the keys array for(const prop of Object.keys(bird)){ // sending keys to function as param birdCan(prop) } //Step:3 // using for in loop we can loop over the object sent as param and iterate over it to genrate results // animalCan function to loop over the object sent as param function animalCan(param){ for(const prop in param){ console.log(`${prop}:${param[prop]}`) } }
this should be enough to understand
// Task 1 function logDairy() { var dairy = ['cheese', 'sour cream', 'milk', 'yogurt', 'ice cream', 'milkshake'] for (word of dairy) { console.log(word) } } logDairy() console.log(); // Task 2 const animal = { canJump: true }; const bird = Object.create(animal); bird.canFly = true; bird.hasFeathers = true; function birdCan() { for (const [key, value] of Object.entries(bird)) { console.log(`${key}: ${value}`); } } birdCan(); console.log(); // Task 3 function animalCan() { for (const key in bird) { console.log(`${key}: ${bird[key]}`); } } animalCan();
- Your answer could be improved with additional supporting information. Please edit to add further details, such as citations or documentation, so that others can confirm that your answer is correct. You can find more information on how to write good answers in the help center . – Community Bot Commented Dec 30, 2022 at 19:42
Successful Passed Test
Passed: Console logged expected values for logDairy Passed: Console logged expected values for birdCan Passed: Console logged expected values for animalCan

- Expected Output follow to our code output console and relate to README.md file with matching , and loop variable declare important for pass test – Nazir Rizwan Commented Apr 24, 2023 at 22:32
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript or ask your own question .
- The Overflow Blog
- Where developers feel AI coding tools are working—and where they’re missing...
- He sold his first company for billions. Now he’s building a better developer...
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Should low-scoring meta questions no longer be hidden on the Meta.SO home...
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- An everyday expression for "to dilute something with two/three/four/etc. times its volume of water"
- If a professor wants to hire a student themselves, how can they write a letter of recommendation for other universities?
- Is the Earth still capable of massive volcanism, like the kind that caused the formation of the Siberian Traps?
- Does a ball fit in a pipe if they are exactly the same diameter?
- Should punctuation (comma, period, etc.) be placed before or after the inches symbol when listing heights?
- Do "always prepared" Spells cost spell slots?
- Print 4 billion if statements
- What exactly is a scratch file (starting with #)? Does it still work today?
- If I was ever deported, would it only be to my country of citizenship? Or can I arrange something else?
- How can moving observer explain non-simultaneity?
- I need a temporary hoist and track system to lift a dead chest freezer up through a tight stairwell
- Can a floppy disk be wiped securely using the Windows format command with the passes-parameter?
- A time-travel short story where the using a time-travel device, its inventor provided an alibi for his future murderer (his wife)
- Pulling myself up with a pulley attached to myself
- Secure flag cookies are sent on a non-secure connection
- Tic-tac-toe encode them all
- How was the year spoken in late 1800s England?
- What is the simplest formula for calculating the circumference of a circle?
- Why is China not mentioned in the Fallout TV series despite its significant role in the games' lore?
- Can ATmega328P microcontroller work at 3.3 V with 8 MHz oscillator
- How long has given package been deferred due to phasing?
- US Visa Appointment error: "Your personal details match a profile which already exists in our database"
- Are there individual protons and neutrons in a nucleus?
- I want to find a smooth section of the map from the Stiefel manifold to the Grassmanian manifold
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Latest commit
File metadata and controls, coursera-programming-assignment-array-and-object-iteration.
Coursera Programming Assignment: Array and object iteration solution! Course Name: Programming with JavaScript, Week-3, Topic: Array and object iteration

IMAGES
VIDEO
COMMENTS
In this exercise, you'll use the for....of loop to iterate over an array and to iterate over an object's own properties. Step 1. You are given an array of dairy products: Create a function called logDairy. Within it, console log each of the items in the dairy array, using the for...of loop. Step 2.
Programming Assignment: Array and object iteration Week 3Coursera Meta Frontend Development Course professional Updated source Code: https://docs.google.com...
My Coursera-Meta "Programming with Javascript" Learnings. Week 3: Array and Object Iteration - THISISNINA/week3-ArrayAndIteration. My Coursera-Meta "Programming with Javascript" Learnings. Week 3: Array and Object Iteration - THISISNINA/week3-ArrayAndIteration ... Select "Submit Assignment" in your Lab toolbar.
Array and object iteration (Coursera practice) Pen Settings. HTMLCSSJS. BehaviorEditor. HTML. HTML Preprocessor. About HTML Preprocessors. HTML preprocessors can make writing HTML more powerful or convenient. For instance, Markdown is designed to be easier to write and read for text documents and you could write a loop in Pug.
The purpose of this assignment is to give you practice writing programs with arrays. Discrete distribution. Write a program DiscreteDistribution.java that takes an integer command-line argument m, followed by a sequence of positive integer command-line arguments a1, a2, …, an a 1, a 2, …, a n. , and prints m random indices (separated by ...
Coursera Programming Assignment: Array and object iteration solution! Meta front-end Developer Professional Certificate, Course Name: Programming with JavaSc...
A double-ended queue or deque (pronounced "deck") is a generalization of a stack and a queue that supports adding and removing items from either the front or the back of the data structure. Create a generic data type Deque that implements the following API: public class Deque<Item> implements Iterable<Item> {. // construct an empty deque.
In this exercise, you'll use the for….of loop to iterate over an array and to iterate over an object's own properties. Step 1. You are given an array of dairy products:
Programming Assignment - Building an Object Oriented Program; Programming Assignment - Array and Object Iteration; Quiz - Introduction to Functional Programming ... react javascript css git bootstrap meta html5 version-control reactjs coursera coding-interviews front-end-development coursera-assignment coursera-specialization ui-ux-design meta ...
Create a function called birdCan, within it, loop over the bird object's properties and console log each one, using the for...of loop.Remember, you need to console log both the key and the value of each of the bird object's properties. Step 3. Using the same starter code as in task 2, create a function called `animalCan` and within it, loop over all the properties in both the bird object and ...
In this exercise, you'll use the for....of loop to iterate over an array and to iterate over an object's own properties. Step 1. You are given an array of dairy products: var dairy = ['cheese', 'sour cream', 'milk', 'yogurt', 'ice cream', 'milkshake'] Create a function called logDairy. Within it, console log each of the items in the dairy array ...
Coursera Programming Assignment: Array and object iteration solution! Course Name: Programming with JavaScript, Week-3, Topic: Array and object iteration Footer
#viralvideo #courseraanswers #trending #courseracourseanswers #problemsolving My Facebook profile-https://www.facebook.com/isratjahan.tisha.547Subscribe free...