

Overloading & Creating New Operators In Swift 5
We'll cover everything you need to know about operator overloading and creating custom operators with unique precedence and associativity behavior.
Operator overloading allows you to change how existing operators (e.g. + , - , * , / , etc.) interact with custom types in your codebase. Leveraging this language feature correctly can greatly improve the readability of your code.
Let’s say we had a struct to represent Money :
Now, imagine we’re building an e-commerce application. We’d likely need a convenient way of adding up the prices of all items in our shopping cart.
With operator overloading, instead of only being able to add numeric values together, we could extend the + operator to support adding Money objects together. Moreover, as part of this implementation, we could even add logic to support adding different currency types together!
In order to take advantage of this language feature, we just need to provide a custom implementation for the operator in our type's implementation:
You may have even used operator overloading without realizing it. If you've ever implemented the Equatable protocol, it requires you to provide a custom implementation for the == operator:
I hope you'll agree that, in these examples, operator overloading has increased the code's readability and expressiveness. However, we should be careful not to overdo it.
Whenever you're creating or overriding an operator, make sure its use is obvious and undisputed. Language features like this often produce diminishing returns, as the more custom behavior we introduce, the harder it is for other developers to understand our code.
Creating Custom Operators
Whenever we're discussing custom operators, overloading an existing operator is always going to be the easier option. Assuming, of course, this doesn't compromise the code's legibility.
Instead, if we want to create our own operator, we'll need to specify 3 additional pieces of information: the type of the operator, the precedence order, and the associativity behavior.
When we're simply overloading an existing operator, we inherit all of this information from the parent operator directly.
Operator Types
When we want to create our own operator, we’ll need to specify whether it's of the prefix , postfix , or infix variety.
prefix - describes an operator that comes before the value it is meant to be used with (e.x. !isEmpty )
postfix - describes an operator that comes after the value it is meant to be used with (e.x. the force-unwrapping operator - user.firstName! )
prefix and postfix are also referred to as "unary” operators as they only affect a single value.
infix - describes an operator that comes in between the value it is meant to be used with and is the most common type (e.x. + , - , / , * are all infix operators)
infix are also referred to as "binary” operators since they operate on two values.
For the statement - 2 + 5 x 5 - we know that the answer is 2 + (5 x 5) => 27 because the precedence of the operators involved tell us the order in which to evaluate the expression.
In the same way that the higher precedence of multiplication resolves the ambiguity in the order of operations here, we need to provide the compiler with similar information when we create a custom operator.
By specifying the precedence, we can control the order in which the expression is evaluated as operations belonging to a higher precedence group are always evaluated first.
We'll see how to specify the precedence of our operator shortly, but let's understand the current state of affairs in Swift first.
The following image shows a list of all precedence group types in Swift from the highest priority to the lowest priority:
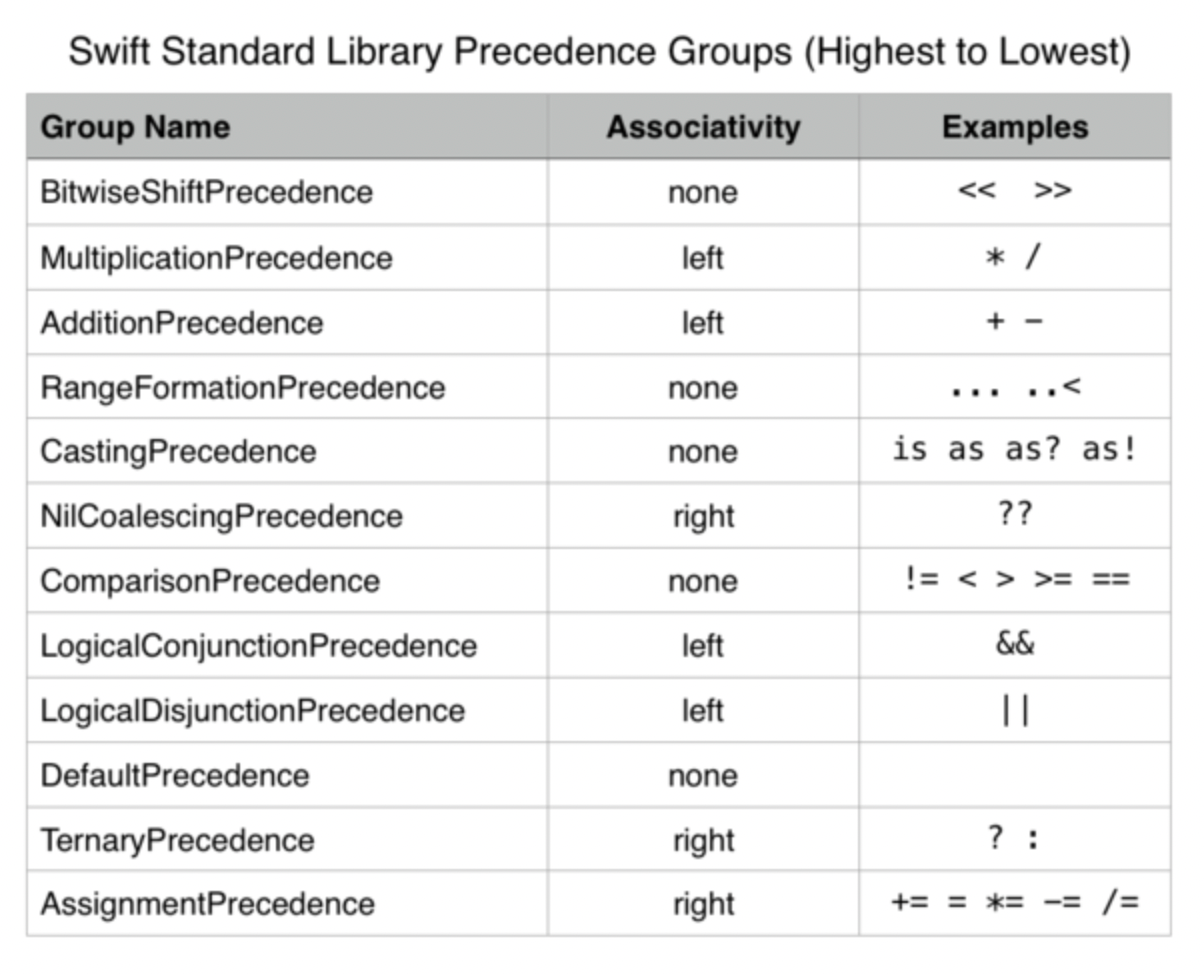
If you declare a new operator without specifying a precedence group, it is a member of the DefaultPrecedence precedence group which has no associativity.
Associativity
The associativity of an operator is simply a property that specifies how operators of the same precedence level are grouped in the absence of parentheses.
Imagine we have an expression with multiple operators all belonging to the same precedence level:
We could process this expression in 2 different ways:
(20 / 2) / 5
20 / (2 / 5)
which would give us 2 and 50 , respectively.
This ambiguity is exactly what the operator's associativity helps us resolve.
An operator can be associative (meaning the operations can be grouped arbitrarily), left-associative (meaning the operations are grouped from the left), and right-associative (meaning the operations are grouped from the right).
In the simplest terms, when we say an operator is left-associative we simply evaluate our expression from left to right. Conversely, for a right-associative operator, we evaluate our expression from right to left.
As another example, we know that * , / , and % all have the same precedence, but by changing their associativity, we can get wildly different results:
Left-Associative
(4 * 8) / 2 % 5 ==> (32 / 2) % 5 ==> 16 % 5 ==> 1
Right-Associative
4 * 8 /(2 % 5) ==> 4 * ( 8 / 2) ==> 4 * 4 ==> 16
Put differently, operator associativity allows us to specify how an expression should be evaluated when it involves multiple operators of the same precedence group.
All arithmetic operators are left-associative.
In order for expressions involving our custom operator to evaluate correctly, we'll need to be mindful of both the operator's precedence and associativity behavior.
Creating A Custom Operator
With all of the theory out of the way, let's create a custom operator that will allow us to easily perform exponentiation.
Since exponentiation has a higher precedence than multiplication and is right-associative, we'll need to create a new precedence group as this operation doesn't match any of Swift's existing precedence group options.
We'll create the precedencegroup by filling in the relevant fields from this template:
Next, we need to let the compiler know about the existence of our custom operator and specify its behavior:
And now, anywhere else in our code we're free to use our custom operator:
2 ^^ 8 => 256.0
It's important to mention here that our precedence group declaration and all operator declarations and functions must be placed at the file scope - outside of any enclosing type. Don't worry if you forget this, the compiler will dutifully remind you.
Limitations Of Operator Overloading
There are a few additional caveats to mention.
While ternary operator types (e.g. var userStatus = user.age > 18 ? .approved : .rejected ) also exist in Swift, the language does not currently allow for overloading their operation.
This same restriction applies to the default assignment operator ( = ) and the compound assignment operator ( += ).
Otherwise, all operators that begin with / , = , - , + , ! , * , % , < , > , & , | , ^ , ? , or ~ , or are one of the Unicode characters specified here are fair game.
Best Practices
While this language feature is extremely powerful and go a long way towards improving your code's legibility and friendliness, it can also take you in the opposite direction.
Let's take a moment to discuss some best practices around using this language feature.
Firstly, overloading operators in Swift should be done in a way that is consistent with how the operator is normally used. A new developer should be able to reason about the expected behavior of the operator without needing to check the implementation.
Next, in situations where the traditional operators don't make semantic sense, you may want to consider creating a custom operator instead.
Finally, and a more pragmatic point, they should be easy to remember and type on the keyboard - an obscure custom operator like .|. benefits no one as its meaning is neither intuitive nor is it convenient to type.
Ultimately, this is just a long-winded way of saying that custom operators, typealias , and all other forms of "syntactic sugar" can improve your code's clarity and your development speed when used with a bit of restraint and pragmatism.
If you're interested in more articles about iOS Development & Swift, check out my YouTube channel or follow me on Twitter .
Join the mailing list below to be notified when I release new articles!
Do you have an iOS Interview coming up?
Check out my book Ace The iOS Interview !
Further Reading
Want to take a deeper dive into operator overloading?
Check out these great resources:
- https://www.codingexplorer.com/custom-operators-swift/
- https://sarunw.com/posts/how-to-create-custom-operators-and-operators-overloading-in-swift/
- https://jayeshkawli.ghost.io/custom-operators-in-swift/
Subscribe to Digital Bunker
Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
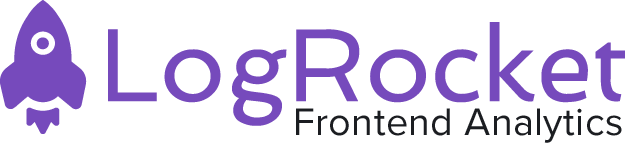
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
Creating custom operators in Swift
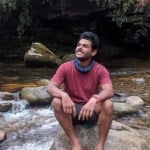
Table of Contents
What is an operator in swift, types of operators in swift, operator notation, operator precedence and associativity in swift, common operators in swift, defining a custom operator in swift, setting precedence of custom swift operators, specifying the notation of a custom operator in swift.
Operators are one of the basic constructs of any programming language. They are represented as symbols and have various associated properties, and understanding them is crucial to mastering any programming language.
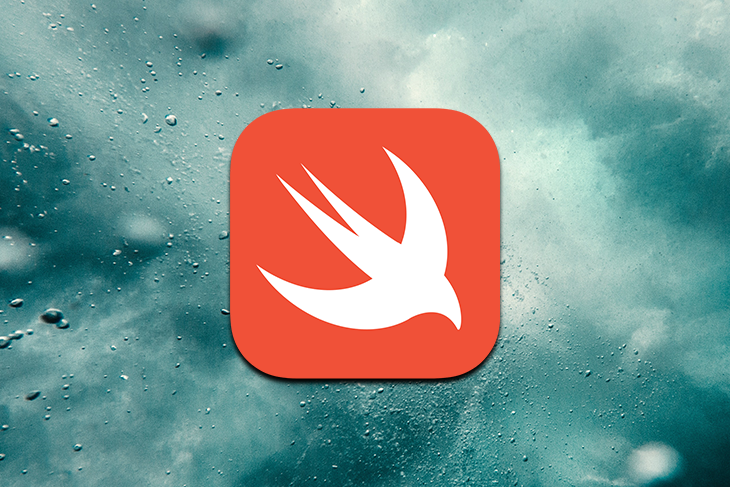
In this article, we’ll look at some of the operators that Swift ships with and also create our own operators.
Before we begin looking at the different operators that Swift provides us with and creating custom operators, let’s understand a few basic terms related to them. Here’s an example:
Here, we can see a few variables and constants being defined, along with a few symbols (e.g., = and + ). Let’s define two terms here:
- Operators: any symbol that is used to perform a logical, computational, or assignment operation. = is used to assign and + is used to add in the above example and are therefore operators
- Operands: variables on which operations are performed, e.g., on line 1 we have a as an operand, and on line 3, we have a and b as two operands on which the + operator is being applied
There are three different types of operators, which are defined by the number of operands they work on.
- Unary operators: operators that work on only one operand (e.g., = and ! )
- Binary operators: work on two operands, such as + , - , * , etc.
- Ternary operator: works on three operands, e.g., ?:
Notation defines the position of the operator when used with the operands. There are again three types:
- Infix : when the operators are used in between operands. Binary and ternary operators are always infixed
- Prefix : when the operators are used before an operand, e.g., ++ , -- , and !
- Postfix : when the operators are used after an operand, e.g., …
When working with operators in Swift, you should also know the priority order in which the operator is executed. Operator precedence only matters for infix operators, as they work on multiple operands.
For example, if an expression has multiple operators in it, Swift needs to know which operator needs to be executed first.
In the above example, * has more precedence compared to + , which is why 6*2 is executed before 4+6 .
In case you have two operators with the same precedence being used in an expression, they’ll fall back on their associativity. Associativity defines the direction in which the expression will start resolving in case the precedence of the operators are the same.
Associativity is of two types:
- left : this means that the expression to the left will be resolved first
- right : this means the expression to the right will be resolved first
For example, we have the following statement:
Because both + and - have the same precedence, Swift falls back to the associativity of the operators. + and - have associativity of left , so we resolve the expression from the left so that + is evaluated first.
Here’s a table of the precedence order that Swift follows. The table lists the precedence in the order of decreasing priority.
, , , | |
, , , , | |
, , , , , | |
, | |
, , , | |
, , , , , , , , , , , , , , | |
, | |
, , | |
, , , , , , , , , , , , , , , , , , |
Now that we know the basics of what Swift operators are and how they are used, let’s look at popular operators that ship with Swift.
Over 200k developers use LogRocket to create better digital experiences

The most common operators that Swift ships with fall under the following categories:
1. Assignment operator
Assignment operators are used to assign values to a constant or variable. The symbol is = .
2. Arithmetic operators
These operators are used to perform basic arithmetic operations, such as + , - , * , / , and % .
3. Logical operators
Logical operators are used to combine two conditions to give a single boolean value output, e.g., ! , && , and || .
4. Comparison operators
These operators are used to compare numbers and give out a true or false output: > , < , == , >= , <= , and != .
5. Ternary operator
This operator is a shorthand operator for writing if-else conditions. Even though it does the same job, it is not a good idea to consider it a replacement for if-else conditions because it hampers readability.
Therefore, when you have different code blocks to run for different conditions, it’s better to use if-else blocks. For shorter, simpler cases, use the ternary operator. The operator, ?: , is used like this:
6. Nil coalescing operator
This is a shorthand operator to return default values in case a particular variable is nil. The operator symbol is ?? and commonly used like this:
7. Range operators
These operators are used to define ranges, e.g., … and ..< . They are mainly used with array/strings to extract sub arrays/strings.
For a list of all the basic operators and their descriptions, read the Swift documentation .
Now that we’ve seen the operators that Swift ships with, let’s create our own operators. Custom operators are generally defined for three purposes:
- To define a completely new operator because you think the symbol’s meaning can express what the operator will do better than a function with a name can
- To define an operator that exists for basic data types like numbers or strings but does not exist for classes or structs that have been defined by you
- You want to override an operator that already exists for your classes and structs, but you need it to behave differently
Let’s look at all three purposes with examples.
Creating a new operator with a new symbol
Let’s say you want to define an operator with the △ symbol that calculates the hypotenuse of a right angle triangle given its two sides.
More great articles from LogRocket:
- Don't miss a moment with The Replay , a curated newsletter from LogRocket
- Learn how LogRocket's Galileo cuts through the noise to proactively resolve issues in your app
- Use React's useEffect to optimize your application's performance
- Switch between multiple versions of Node
- Discover how to use the React children prop with TypeScript
- Explore creating a custom mouse cursor with CSS
- Advisory boards aren’t just for executives. Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
Creating an operator for a custom class or struct
We know that the + operator is used to add two numbers, but let’s say we wanted to add two instances of our struct called Velocity , which is a two-dimensional entity:
If we were to run the following command, you’d get a compiler error:
In this case, it makes sense to define a + operator that takes care of adding two Velocity instances:
Now the earlier statement will execute properly.
Overriding a Swift operator that already exists for a class/struct
Let’s say you have a struct that is used to define an item in a supermarket.
When we need to compare two items, such that if two items have the same itemName , itemType , and itemPrice , we need to consider them equal regardless of what their id is.
If you run the following piece of code, you’ll see that the two variables are not equal:
The reason for this is that instances detergentA and detergentB have different ids . In this case, we need to override the == operator and custom logic to determine equality.
Now that we know the different scenarios in which we define custom operators, let’s define our custom operator.
A custom operator is defined just like a function is defined in Swift. There are two types of operators you can define:
Global operator
- Operator specific to a class/struct
The definition looks like the following:
There are different types of parameters you can define based on whether it is an infix or a prefix / postfix operator.
If it’s an infix operator, you can have two parameters, and, when it’s a prefix / postfix operator, you can have a single parameter.
With global operators, we first define the operator with the notation keyword ( infix , prefix or postfix ) and the operator keyword, like this:
Here’s an example:
Operator for class/struct
When we want to define an operator for a class or a struct, we need to define the operator function as a static function. The notation parameter is optional in case the operator is an infix operator (i.e., you have two parameters in the function signature), else you need to specify prefix or postfix :
Given that we’ve already looked at defining basic custom operators like + and == , let’s review some examples of how you can define custom compound operators or operators that mutate the parameters themselves.
We’ll do this by defining a custom compound arithmetic operator for the Velocity struct from earlier:
Now let’s execute the following statement:
Now, we’ll specify the notation of a custom operator.
In order to define the notation of a custom operator, you have three keywords for the corresponding notation:
When defining a global operator, you need to first define the operator’s notation, then define its functions. Note that this is not a compulsory step, but it’s necessary if you want to define global operators or hope to assign a precedence to it (which we’ll discuss in the next section).
Let’s look at the hypotenuse operator again, which is being defined as a global operator. You can see that we first define the operator with the notation and the symbol, then implement its logic:
When defining an operator for your own class/struct, you can directly use the notation keyword with the method definition. For example, let’s define a negation operator for Velocity as well, which negates both the xVelocity and yVelocity properties. This operator is going to be defined as a prefix operator:
You can also define the precedence of your operator using the precedence keywords, as mentioned in the precedence table above. This is usually done in the definition step:
This way, the compiler knows which operator needs to be executed first. In case no precedence is specified, it defaults to DefaultPrecedence , which is higher than TernaryPrecedence .
This brings us to the conclusion of what operators are in Swift and how you can create your own. Although they are pretty simple to use, understanding them is important because they are one of the most foundational constructs of any programming language.
You should be familiar with basic operators, as you will be using them frequently in your code, and, when it comes to custom operators, define them only if the symbol’s original meaning makes sense for you.
Get set up with LogRocket's modern error tracking in minutes:
- Visit https://logrocket.com/signup/ to get an app ID
Install LogRocket via npm or script tag. LogRocket.init() must be called client-side, not server-side
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
Hey there, want to help make our blog better?
Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
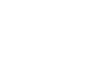
Stop guessing about your digital experience with LogRocket
Recent posts:.
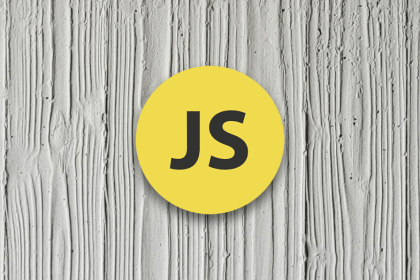
How to display notification badges on PWAs using the Badging API
Ding! You got a notification, but does it cause a little bump of dopamine or a slow drag of cortisol? […]
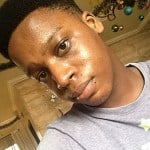
JWT authentication: Best practices and when to use it
A guide for using JWT authentication to prevent basic security issues while understanding the shortcomings of JWTs.
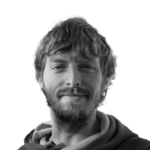
Auth.js adoption guide: Overview, examples, and alternatives
Auth.js makes adding authentication to web apps easier and more secure. Let’s discuss why you should use it in your projects.
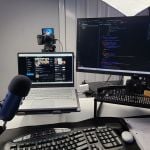
Lucia Auth: An Auth.js alternative for Next.js authentication
Compare Auth.js and Lucia Auth for Next.js authentication, exploring their features, session management differences, and design paradigms.
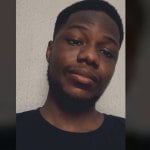
Leave a Reply Cancel reply
Overloading Custom Operators in Swift
In this Swift tutorial, you’ll learn how to create custom operators, overload existing operators and set operator precedence. By Owen L Brown.

Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Already a member of Kodeco? Sign in
Getting Started
Overloading the addition operator, other types of operators.
- Mixed Parameters? No Problem!
- Protocol Operators
- Creating Custom Operators
- Precedence Groups
- Dot Product Precedence
- Where to Go From Here?
Operators are the core building blocks of any programming language. Can you imagine programming without using + or = ?
Operators are so fundamental that most languages bake them in as part of their compiler (or interpreter). The Swift compiler, on the other hand, doesn’t hard code most operators, but instead provides libraries a way to create their own. It leaves the work up to the Swift Standard Library to provide all of the common ones you’d expect. This difference is subtle but opens the door for tremendous customization potential.
Swift operators are particularly powerful because you can alter them to suit your needs in two ways: assigning new functionality to existing operators (known as operator overloading ), and creating new custom operators.
Throughout this tutorial, you’ll use a simple Vector struct and build your own set of operators to help compose different vectors together.
Open Xcode and create a new playground by going to File ▶ New ▶ Playground . Pick the Blank template and name your playground CustomOperators . Delete all the default code so you can start with a blank slate.
Add the following code to your playground:
Here you define a new Vector type with three properties conforming to two protocols. The CustomStringConvertible protocol and the description computed property let you print a friendly String representation of the Vector .
At the bottom of your playground, add the following lines:
You just created two Vector s with simple Array s, and with no initializers! How did that happen?
The ExpressibleByArrayLiteral protocol provides a frictionless interface to initialize a Vector . The protocol requires a non-failable initializer with a variadic parameter: init(arrayLiteral: Int…) .
The variadic parameter arrayLiteral lets you pass in an unlimited number of values separated by commas. For example, you can create a Vector such as Vector(arrayLiteral: 0) or Vector(arrayLiteral: 5, 4, 3) .
The protocol takes convenience a step further and allows you to initialize with an array directly, as long as you define the type explicitly, which is what you’ve done for vectorA and vectorB .
The only caveat to this approach is that you have to accept arrays of any length. If you put this code into an app, keep in mind that it will crash if you pass in an array with a length other than exactly three. The assert at the top of the initializer will alert you in the console during development and internal testing if you ever try to initialize a Vector with less than or more than three values.
Vectors alone are nice, but it would be even better if you could do things with them. Just as you did in grade school, you’ll start your learning journey with addition .
A simple example of operator overloading is the addition operator. If you use it with two numbers, the following happens:
But if you use the same addition operator with strings, it has an entirely different behavior:
When + is used with two integers, it adds them arithmetically. But when it’s used with two strings, it concatenates them.
In order to overload an operator, you have to implement a function whose name is the operator symbol.
Add the following piece of code at the end of your playground:
This function takes two vectors as arguments and return their sum as a new vector. To add vectors, you simply need to add their individual components.
To test this function, add the following to the bottom of your playground:
You can see the resultant vector in the right-hand sidebar in the playground.
The addition operator is what is known as an infix operator, meaning that it is used between two different values. There are other types of operators as well:
- infix : Used between two values, like the addition operator (e.g., 1 + 1 )
- prefix : Added before a value, like the negation operator (e.g., -3 ).
- postfix : Added after a value, like the force-unwrap operator (e.g., mayBeNil! )
- ternary : Two symbols inserted between three values. In Swift, user defined ternary operators are not supported and there is only one built-in ternary operator which you can read about in Apple’s documentation .
The next operator you’ll want to overload is the negation sign, which will change the sign of each component of the Vector . For example, if you apply it to vectorA , which is (1, 3, 2) , it returns (-1, -3, -2) .
Add this code below the previous static function, inside the extension:
Operators are assumed to be infix , so if you want your operator to be a different type, you’ll need to specify the operator type in the function declaration. The negation operator is not infix , so you add the prefix modifier to the function declaration.
At the bottom of your playground, add the line:
Check for the correct result in the sidebar.
Next is subtraction, which I will leave to you to implement yourself. When you finish, check to make sure your code is similar to mine. Hint: subtraction is the same thing as adding a negative.
Give it a shot, and if you need help, check the solution below!
[spoiler title=”Solution”]
Test your new operator out by adding this code to the bottom of your playground:
All videos. All books. One low price.
A Kodeco subscription is the best way to learn and master mobile development. Learn iOS, Swift, Android, Kotlin, Flutter and Dart development and unlock our massive catalog of 50+ books and 4,000+ videos.
This page requires JavaScript.
Please turn on JavaScript in your browser and refresh the page to view its content.
Coding Explorer Blog
Exploring how to code for iOS in Swift and Objective-C
Operator Overloading — Tailor Swift To Your Needs
Last updated on August 12, 2020
Sorry, but I have been waiting for months to make this pun. So today, we are going to talk about Operator overloading in Swift. This tool is very useful, but quite dangerous as well. The “With great power comes great responsibility,” quote is very appropriate for operator overloading. It can make your code a lot more concise, making even a function call seem like a 3-hour long lecture. But with that, you can also make the code nigh-unreadable to anybody that is new to your code.
Be very careful with this tool, and use it where it make sense, such as how “adding” two Swift Strings together makes a new string with one string first, then the other afterwards. You can make the addition operator print to the screen, make a network request, play music, or whatever else you could write a function for, but doing any of those would be a terrible idea in production code. You should do only what is necessary for the operator and nothing else. If you need different things like those, make an appropriately named function that makes it obvious that it will make a network request.
Whenever you think of whether to use operator overloading, you have to ponder whether it helps your code anymore than a simple function call. The function call may be longer, but it is a whole lot more expressive as to what it does (if named appropriately). If it looks like you are adding, subtracting, checking equality, or whatever else the built-in operators do though, and you do it enough in your code, Swift’s operator overloading may be the right tool for the job.
Operator Overloading
You should only use operator overloading in a way that is consistent with how the operator is normally used in Swift. Here is an example I thought of that could make sense, though there are still some pitfalls with it. Let’s say we want to find the amount of time between two Date objects. It would make sense to get that via “laterDate – initialDate” right? So let’s do that:
In this case, I chose the Calendar.Components of year, month, day, hour, minute, and second as the components to use in this subtraction. Herein lies ones of the pitfalls. There are several other Components I could choose. If I just wanted to know the weeks between something, I could put that in instead for this. Nonetheless, using these units give us what we would want in most calendrical calculations. The next line just creates the DateComponents object using components:from:to: from the currentCalendar. We’re taking advantage of the fact that a single line of code that returns something can be used without explicitly writing return, because it is pretty clear to the compiler that a function that returns a date components being the only thing called in a function that also returns DateComponents would simply want to forward that return.
An operator used between two values is called an infix operator. There are two other overloadable types of operators known as prefix and postfix , like the old ++i and i++ operators of yore.
As you can see from the top, this is just a function in Swift, but instead of a text name, we have a symbol as the name. We specify the types of inputs for the left and right parts of the expression, and then specify the return type, which for this calculation should be an DateComponents object. Now we can use it like this:
There was a bit of setup there, but most of it should be pretty easy to understand. We first create an initial date, which has the value of the moment the Date object was created. Then there’s an DateComponents object that has its year, month, and minute values set. Then the laterDate constant is made, using Calendar’s dateByAddingComponents method, so that makes a value 1 year, 5 months, and 42 minutes in the future. Then we actually test our new capability for the ” – ” operator. When we look at the components of the result of our subtraction, you can see it has the correct value for the year, month, and minute value for exactly how far in the future it was.
I think that this function is a marginally okay use of operator overloading. It is using the subtraction operator how it is normally used in math. However, it hides several assumptions. I already mentioned that it uses certain Calendar.Components, but not all of them. Secondly, it uses Calendar’s current variable. I think this makes sense, but it is nonetheless hidden.
If we just use the normal function call that is behind our overloaded operator, you can clearly see what calendar it is using and which flags you want. That is why I only say that this is a marginally okay use of operator overloading. It makes sense, but it hides those assumptions from the reader, making them have to look up the operator overloading function to see what assumptions are made.
The Equatable Protocol
There are a few places in Swift where operator overloading is actually encouraged. If you want to be able to compare your custom class with the ” == ” operator, you will have to overload it. If you are going to implement this, your class should probably adopt the Equatable protocol. The Equatable protocol is used often with Generic functions (you can read more about them here: Generic Functions in Swift ), when it will need to check for equality inside. If you do, you only need to implement the ” == ” operator, you get the != for free (since it is basically just negating the ” == ” operator’s answer.
The way you overload the ” == ” operator is the same as with any other infix operator, like what we did above with the ” – ” operator. Let’s make a custom type and overload the ” == ” operator below:
Now we can compare Temperature instances with the ” == ” operator. When an operator is overloaded, it must be marked as static. Actually, if I had made this a struct or enum, I wouldn’t even need the initializer or the operator overloading! If the parts in it are hashable or equatable, structs and enums can generate those methods themselves, without me needing to explicitly write them. The same is not extended to classes, and really, this type should’ve been a struct, but I wanted to show the behavior for a type that didn’t automatically get those methods when they adopt the protocol.
Another place where operator overloading in Swift is encouraged is with the Comparable protocol. To conform to the Comparable protocol you must implement an operator function for the ” < ” operator, as well as conform to the Equatable protocol. With you implementing == and > operators, the compiler can figure out the !=, <, <=, and >= operators for you. You can see an example of this in my previous post Generic Functions in Swift . It also has another example of overloading the ” == ” operator.
I debated showing the Date subtraction example above, due to how it hides many assumptions about the code being called. I decided to share it though, because it also highlights what you have to think about when deciding to use operator overloading in Swift. If you are doing subtraction of dates a lot in your code, always with the same unitFlags, overloading the subtraction operator could make your code a lot more readable.
There are a few operators that you cannot overload, most notably the assignment operator ” = ” (just one equal sign). Even if you could, I’m not sure I would want to know the chaos that would be wrought by doing so, considering how much the assignment operator is used. You can read more about the operators you can’t overload at Tammo Freese ‘s article Facets of Swift, Part 5: Custom Operators .
Much like Custom Subscripts in Swift , this tool is very useful, but very dangerous. Use it wisely .
In updating this post, there are two very welcome updates to Swift that made the code for the “-” operator’s overload much nicer. Firstly, that you can use the most common Calendar.Components with their common names (.Year, .Month, etc.) instead of the longer original ones ( . YearCalendarUnit, .Month CalendarUnit, etc.). Secondly, the code used to use the OR operator ” | ” to combine the unit flags. Now, Swift lets you give it a Swift Set type for options of that sort, which itself can be created with a Swift Array literal.
I hope you found this article helpful. If you did, please don’t hesitate to share this post on Twitter or your social media of choice. The blog is still pretty new, and every share helps. Of course, if you have any questions, don’t hesitate to contact me on Twitter @CodingExplorer , and I’ll see what I can do. Thanks!
- The Swift Programming Language – Apple Inc.
- Facets of Swift, Part 5: Custom Operators — Swift Programming — Medium
- Terms Of Use
- Privacy Policy
- Affiliate Disclaimer
Subscribe to the Coding Explorer Newsletter
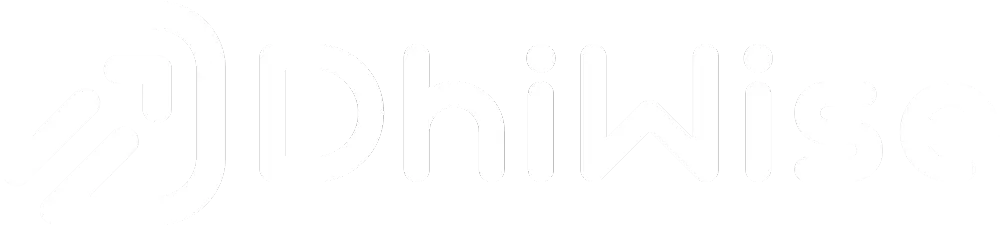
You’ve made it this far. Let’s build your first application
DhiWise is free to get started with.

Design to code
- Figma plugin
- Documentation
- DhiWise University
- DhiWise vs Anima
- DhiWise vs Appsmith
- DhiWise vs FlutterFlow
- DhiWise vs Monday Hero
- DhiWise vs Retool
- DhiWise vs Supernova
- DhiWise vs Amplication
- DhiWise vs Bubble
- DhiWise vs Figma Dev Mode
- Terms of Service
- Privacy Policy
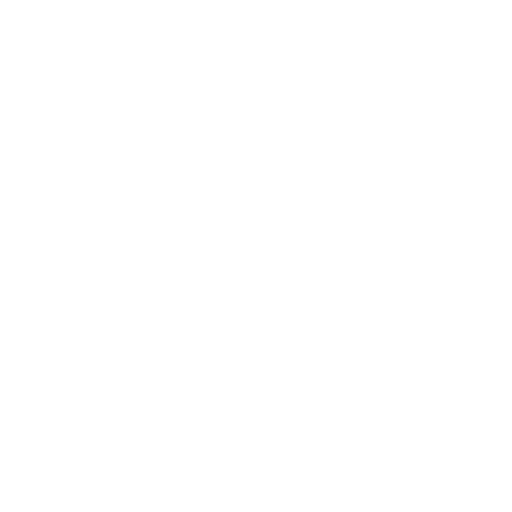
Inside Swift Operators: Your Guide to Effortless Coding with Swift
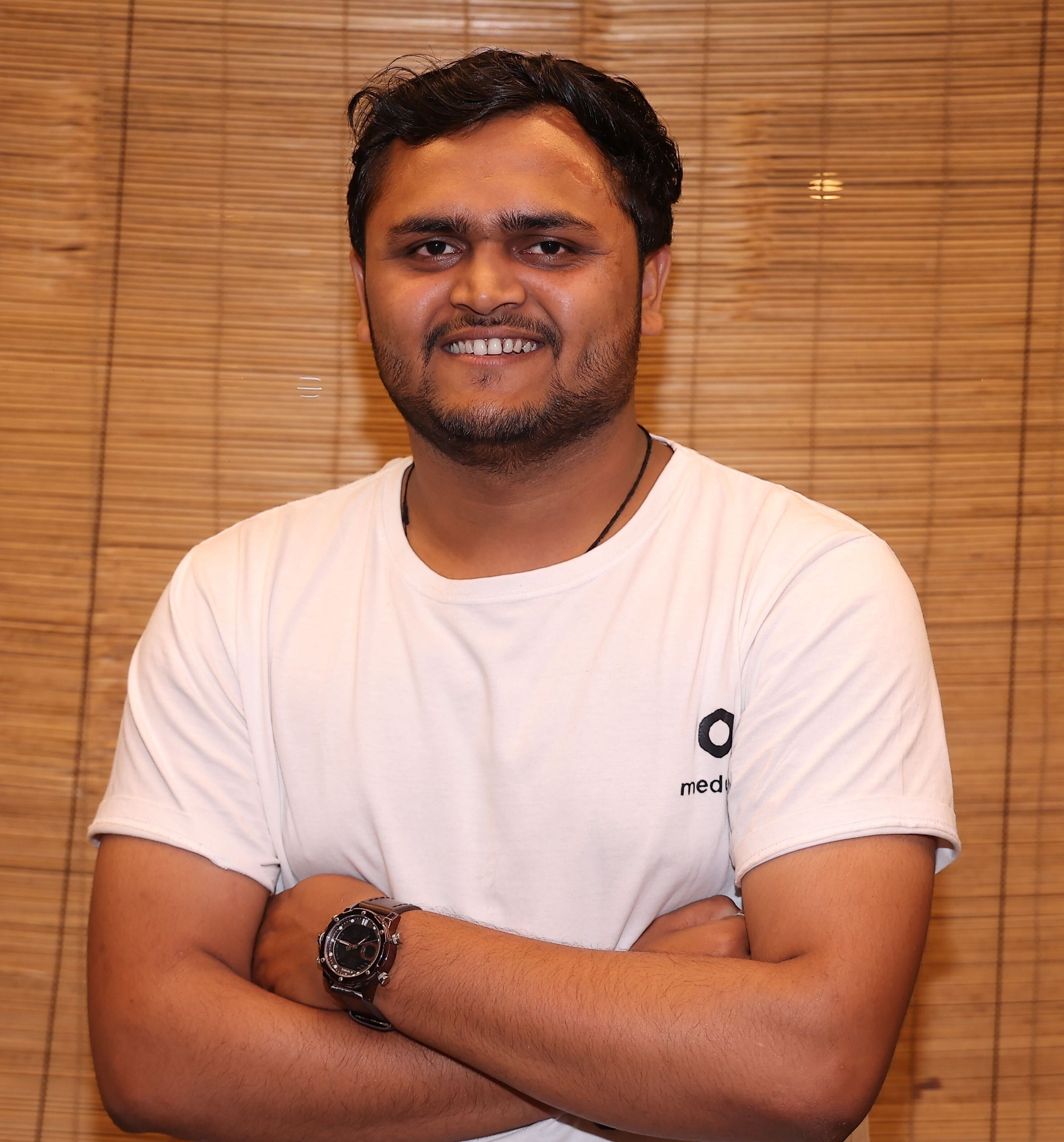
Parth Asodariya
Frequently asked questions, what is a swift operator, what is the precedence of operators in swift, how to use logical operators in swift, what is an example of a range operator in swift, is there operator overloading in swift.
Swift, Apple's powerful and intuitive programming language, has been a game-changer since its introduction. It simplifies the complexities of app development, making it accessible to a broader audience.
A fundamental aspect of Swift—and any programming language—is its operators. Operators in Swift perform various tasks, from basic arithmetic to logical comparisons and more.
In this blog, we will understand Swift operators, and how they impact your code, and cover everything from arithmetic to custom operators. Being fluent with operators is helpful to understand the grammar of a language—it allows you to write clearer, more efficient code and is essential for any developer looking to master Swift.
Basic Swift Operators
Operators are special symbols used within Swift to execute particular operations on values and variables—known as operands. They can manipulate number values, string values, boolean values, and other data types by performing tasks such as assignments, arithmetic calculations, comparisons, or logic.
Exploring Arithmetic Operators in Swift
Arithmetic operators are the most familiar operators, performing mathematical operations on numerical values. These include the addition (+), subtraction (-), multiplication (*), division (/), and remainder (%) operators. Arithmetic operators in Swift are used in the same way as in mathematics.
In the above example, sum, difference, product, and quotient all hold the final value after the respective operation is applied to two values. The remainder operator % provides the remainder of the division, signifying operations are not merely limited to elementary arithmetic but also accommodate modular arithmetic in Swift.
Usage of Arithmetic Operators With Examples
The ability to print consistent and accurate arithmetic operations remains a building block of Swift applications. For instance, a currency converter app performs arithmetic operations to determine exchange rates. Consider the following example:
The code sample uses an arithmetic operator to calculate a currency conversion from dollars to euros and outputs the result with the print function.
Logical Operators in Swift
Swift logical operators evaluate boolean value expressions, allowing code to make decisions. Logical operators include && (logical AND), || (logical OR), and ! (logical NOT). Here's a brief look at how these Swift logical operators control program flow:
• && returns true if both expressions are true.
• || returns true if at least one of the expressions is true.
• ! inverts the boolean value of an expression.
In the example, the front-page feature requirement depends on both having a high score and good reviews. The use of && ensures that both conditions must be met for the conditional branch to execute.
Swift Operator Overloading
Swift operator overloading allows developers to provide a custom implementation of existing operators for their types or create new operators with customized behavior. This feature is particularly powerful as it enables clean and intuitive usage of custom types, akin to Swift’s built-in types.
To overload an operator, you define a new implementation using the func keyword and specifying the operator's symbols:
Overloading the + operator allows adding two Vector2D instances, returning a new Vector2D that represents the sum of the original vectors.
Operators for Swift Ranges
A range in Swift represents a sequence of consecutive values, which can be numbers, characters, or another sequence type. Using range operators is essential when working with arrays, loops, or any context where you need to specify a span of values.
The Closed Range Operator
The closed range operator (...) includes all the values within the range, extending from the lower bound to the upper bound. This operator is extremely useful when you want to iterate over all the elements in a collection without excluding any.
Here's an example of a closed range in action:
In the above code, the loop iterates over a closed range that includes the numbers 1 through 5.
The Half-Open Range Operator
Conversely, the half-open range operator (..<) includes values from the lower bound up to, but not including, the upper bound value. The half-open range is especially useful when working with zero-based lists like arrays, where you wish to include all the elements up to the one before the last.
Consider this example using a half-open range:
Here we access the array index using the half-open range to access all the elements within the fruits array without exceeding its bounds.
Swift Range Operator Applications
Ranges in Swift are powerful tools that simplify code involving sequences of values. Whether for use in a loop to iterate over a set number of iterations or to slice off subsets from collections, range operators help streamline these operations.
Let's see how range operators manage swift ranges through a collection slicing example:
The example demonstrates the closed range operator by slicing an array to create a new passingScores array containing elements at indices 1 to 3 from the scores array.
Advanced Swift Operators
Beyond the basics, Swift provides a suite of advanced operators that perform more complex operations. These include bitwise operators for manipulating binary data, and compound assignment operators (such as += and *=) that combine assignment (=) with another operation.
Let's explore the bitwise and assignment operator used in Swift:
Bitwise Operators in Swift
Bitwise operators allow operations on individual bits of integers. They are used when performing low-level programming, such as graphics programming or device driver creation.
For example, the bitwise AND operator (&) compares the bits of two integers and returns a new integer with bits set to 1 only where both original integers had them at 1:
Compound Assignment Operators
Compound assignment operators provide a shorthand way to modify the value of variables. For instance, instead of writing x = x + 2, you can simply write x += 2.
In the above code, we first defined a score variable, and then used a compound assignment operator to subtract 5 from the current value of the score.
Swift Operator Precedence and Associativity
Understanding operator precedence and associativity is crucial in Swift, as it determines the order in which operations are executed. The operator's precedence gives some operations higher priority than others, whereas associativity defines the order operations of the same precedence are performed.
For example, the multiplication operator (*) has a higher precedence than the addition operator (+). Therefore, the multiplication is performed before addition in a non-parenthesized expression.
In Swift, operators with the same precedence level are evaluated according to their associativity. Most arithmetic operators are left associative, meaning they evaluate from left to right.
Custom Operators and Precedence
Swift allows you to define your operator with custom precedence and associativity. To create a new operator, use the operator keyword, and then specify the precedence group to dictate how it interacts with other operators.
In this example, a custom infix operator named ^^ is defined for exponentiation, with precedence higher than multiplication and right associativity.
In this post, we've taken an extensive look into Swift operators, solidifying them as indispensable tools in a developer's toolkit. From handling arithmetic calculations to manipulating swift ranges and customizing behavior through operator overloading, operators in Swift are versatile and powerful.
Recognizing the significance of operators in Swift will not only streamline your coding process but also open up a world of possibilities for creating efficient and readable code. As you continue on your journey with Swift, let the mastery of operators empower your development, enabling you to craft elegant solutions with precision and ease.
Keep practicing, and explore new ways to leverage operators in your projects, and soon you'll be using them to their full potential, just as effortlessly as you write a print statement. Happy coding!
Short on time? Speed things up with DhiWise!!
Tired of manually designing screens, coding on weekends, and technical debt? Let DhiWise handle it for you!
You can build an e-commerce store, healthcare app, portfolio, blogging website, social media or admin panel right away. Use our library of 40+ pre-built free templates to create your first application using DhiWise.
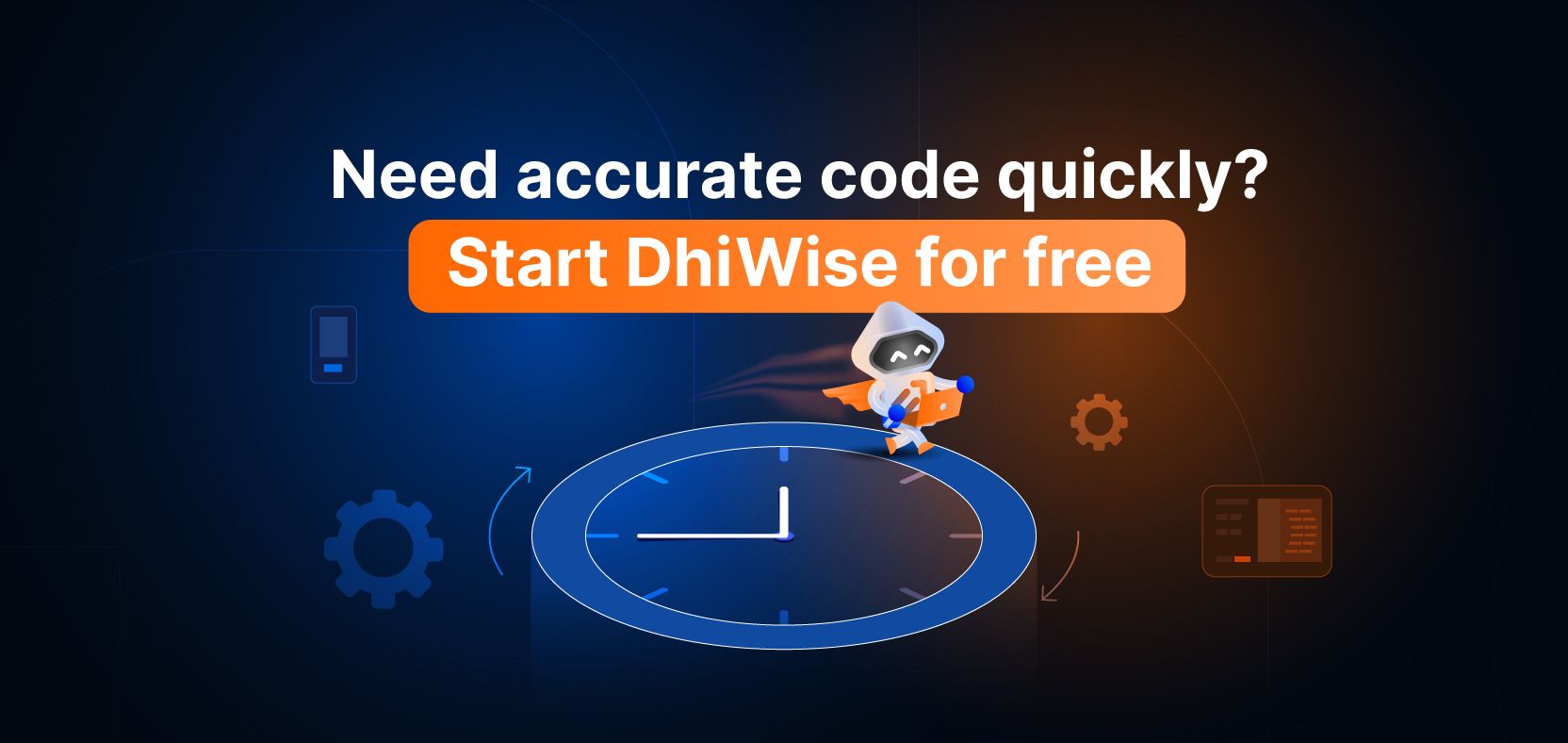
Custom assignment operator
Currently, swift's = operator does not return a value. This seems like a lapse to me, because, if it was othersise, then some usage in function builder would be simpler. But that's okay, since there is the ability to declare a custom one. Right?
But it produces a bunch of errors, from one suggesting that it is incorrectly used prefix unary func to the other saying to separate statements. All of this obviously indicates that I cannot properly work with lvalues. Can I?
That syntactic position cannot take an arbitrary operator. But more importantly, the operation done by var someView = expression is initialization, not assignment — so even if an arbitrary operator were allowed in that position, what you're trying to do wouldn't be allowed, because it's not okay to bind an inout parameter to an uninitialized variable.
It's also not clear why
aren't both better solutions than introducing a new operator.
To be able to reference objects by name in fb closure, rather than putting there ugly stuff, like a bunch of explicit returns. Currently, you have to ...
If I could make a custom assignment, then that would become
Nice and clean, which is the whole point of this function building stuff, no?
Why so? If there were such thing in swift as customizable assignment operator, then it would be not important if lvalue was initialized, because that what custom = would do.
There are much more applications to a custom assignment. What I have shown is the one among those missed opportunities for clean code simply because of yet another language limit.
What is point than to have a assignment field/property in precedence group declaration?
Please don't tell me that it is there because swift cannot deterministically resolve operators based on argument mutability
Leads to Ambiguous use of operator '=>
The problem with accepting uninitialized value for inout argument is that the function can READ inout arguments. The function signature says nothing about only writing to the said inout variable.
Mostly because, while being special, = is still an operator. It still needs to interact with other operators when parsing. Also, = is not the only operators of this class, *= and -= are normal operators that have AssignmentPrecedence .
It is to make assignment operators work “inside” of optional chaining:
It could be made such that operators with assignment wouldn't be able to read the value. Problem solved?
It might be better to propose implementing an out parameter which could take uninitialised variables. This could be somewhat useful in other situations like factoring out parts of initialisers. I'm not sure it's useful enough to be implemented, because it's not commonly needed and you can always just return the values instead and move the variables to the LHS, but it would be better than making a weird special case.
TEAM LICENSES: Save money and learn new skills through a Hacking with Swift+ team license >>
Why does Swift need operator overloading?
Paul Hudson @twostraws May 28th 2020
Updated for Xcode 16
Operator overloading allows the same operator – + , * , / , and so on – to do different things depending on what data you use it with. This allows us to use these symbols in various places where they would make sense: we can add two integers using +, we can append one string to another using +, we can join two arrays using +, and so on.
Of course, this doesn’t mean we can use all the operators in every place: we can subtract one integer from another, but what would it mean to subtract one string from another? Would it subtract from the start or the end? Would it subtract all instances of the string or just one?
When your skills grow you’ll find you can create your own custom operators if you want to, and even modify Swift’s existing operators.
SPONSORED Join a FREE crash course for mid/senior iOS devs who want to achieve an expert level of technical and practical skills – it’s the fast track to being a complete senior developer! Hurry up because it'll be available only until September 29th.
Click to save your spot
Sponsor Hacking with Swift and reach the world's largest Swift community!

Was this page useful? Let us know!
Average rating: 5.0/5

You are not logged in
Link copied to your pasteboard.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Swift: Overriding == in subclass results invocation of == in superclass only
I've got a class A , which conforms to Equatable protocol and implements == function. In subclass B I override == with more checks.
However, when I do comparison between two arrays of instances of B (which both have type Array<A> ), == for A is invoked. Of course if I change type of both arrays to Array<B> , == for B is invoked.
I came up with the following solution:
Which looks really ugly and must be extended for every subclass of A . Is there a way to make sure that == for subclass is invoked first?
2 Answers 2
The reason the equality for A is being invoked for an Array<A> that contains B is that overloading of free functions is resolved statically, not dynamically – that is, at compile time based on the type, not at runtime based on the pointed-to value.
This is not surprising given == is not declared inside the class and then overridden in the subclass. This might seem very limiting but honestly, defining polymorphic equality using traditional OO techniques is extremely (and deceptively) difficult. See this link and this paper for more info.
The naïve solution might be to define a dynamically dispatched function in A , then define == to just call that:
Then when you implement B , you’d override equalTo :
You still have to do one as? dance, because you need to determine if the right-hand argument is a B (if equalTo took a B directly, it wouldn’t be a legitimate override).
There’s also still some possibly surprising behaviour hidden in here:
That is, the order of the arguments changes the behaviour. This is not good – people expect equality to be symmetric. So really you’d need some of the techniques described in the links above to solve this properly.
At which point you might feel like all this is getting a bit unnecessary. And it probably is, especially given the following comment in the documentation for Equatable in the Swift standard library:
Equality implies substitutability . When x == y , x and y are interchangeable in any code that only depends on their values. Class instance identity as distinguished by triple-equals === is notably not part of an instance's value. Exposing other non-value aspects of Equatable types is discouraged, and any that are exposed should be explicitly pointed out in documentation.
Given this, you might seriously want to reconsider getting fancy with your Equatable implementation, if the way you’re implementing equality is not in a way where you’d be happy with two values being equal being substituted with each other. One way to avoid this is to consider object identity to be the measure of equality, and implement == in terms of === , which only needs to be done once for the superclass. Alternatively, you could ask yourself, do you really need implementation inheritance? And if not, consider ditching it and using value types instead, and then using protocols and generics to capture the polymorphic behaviour you’re looking for.
- Thanks a lot for detailed answer and your hard work! I'll take a look at links a bit later. – kovpas Commented Mar 1, 2015 at 13:58
- 1 Another problem: if the Equatable protocol conformance is declared in an extension , you cannot override it. – Kevin R Commented Nov 4, 2015 at 14:16
I ran into a similar issue because I wanted to use the difference(from:) on an MKPointAnnotation subclass (which inherits from NSObject). Even if I added func == to my annotation subclass, difference(from: would still call NSObject's implementation of == . I believe this just compares the memory location of the 2 objects which is not what I wanted. To make difference(from: work properly, I had to implement override func isEqual(_ object: Any?) -> Bool { for my annotation subclass. In the body I would make sure the object was the same type as my subclass and then do comparison there.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged swift overriding equality or ask your own question .
- The Overflow Blog
- One of the best ways to get value for AI coding tools: generating tests
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- How to NDSolve stiff ODE?
- Should I be careful about setting levels too high at one point in a track?
- Will "universal" SMPS work at any voltage in the range, even DC?
- 1950s comic book about bowling ball looking creatures that inhabit the underground of Earth
- O(nloglogn) Sorting Algorithm?
- How should I email HR after an unpleasant / annoying interview?
- Do I have to use a new background that's been republished under the 2024 rules?
- Fast leap year check
- Definition of annuity
- How do I completly remove "removed" disks from mdadm array
- Copyright Fair Use: Is using the phrase "Courtesy of" legally acceptable when no permission has been given?
- Existence of 2-fold branched covers
- Does the science work for why my trolls explode?
- What factors cause differences between dried herb/spice brands?
- Offset+Length vs 2 Offsets
- What does "иного толка" mean? Is it an obsolete meaning?
- Should I change advisors because mine doesn't object to publishing at MDPI?
- In Photoshop, when saving as PNG, why is the size of my output file bigger when I have more invisible layers in the original file?
- Looking for a short story on chess, maybe published in Playboy decades ago?
- What properties of the fundamental group functor are needed to uniquely determine it upto natural isomorphism?
- What would a planet need for rain drops to trigger explosions upon making contact with the ground?
- What is the oldest open math problem outside of number theory?
- How to easily detect new appended data with bpy?
- Getting a planet to be as volcanic as Io

IMAGES
VIDEO
COMMENTS
It is not possible to overload the default assignment operator (=). Only the compound assignment operators can be overloaded. Similarly, the ternary conditional operator (a ? b : c) cannot be overloaded. If that doesn't convince you, just change the operator to +=: func +=(left: inout CGFloat, right: Float) {. left += CGFloat(right) }
Swift's operator precedences and associativity rules are simpler and more predictable than those found in C and Objective-C. However, this means that they aren't exactly the same as in C-based languages. ... It isn't possible to overload the default assignment operator (=). Only the compound assignment operators can be overloaded ...
Not every type of operator can be overload. There are four restrictions that I know of: You can't overload and create a custom ternary operator. You can't overload the default assignment operator (=). You can overload other binary operators, including compound assignment operators such as a += 2. Only a subset of the ASCII characters are supported.
Overloading & Creating New Operators In Swift 5. We'll cover everything you need to know about operator overloading and creating custom operators with unique precedence and associativity behavior. Operator overloading allows you to change how existing operators (e.g. +, -, *, /, etc.) interact with custom types in your codebase.
Basically, I'm looking to overload the "+" operator so that it knows which "version" of the "+" to use depending of the type. swift; operator-overloading; operators; ... how to overload an assignment operator in swift. 1. Declare overloaded += operator as mutating? 2. Swift ambiguous overloaded + operator.
The overloading operators must be marked with the static keyword and can be defined within class, struct, or enum types to perform operations that involve their instances. Swift provides a comprehensive set of operators, which are categorized as unary, binary, and ternary: • Unary operators operate on a single target.
Enter the following in your playground to confirm Swift operators follow these same rules: var sumWithMultiplication = 1 + 3 - 3 * 2. You'll see the following result: -2. In cases where arithmetic operators have the same precedence, Swift evaluates the operators from left to right.
Operator overloading is the practice of adding new operators and modifying existing ones to do different things. Operators are those little symbols like +, *, and /, and Swift uses them in a variety of ways depending on context - a string plus another string equals a combined string, for example, whereas an integer plus another integer equals a summed integer.
Operator overloading. Swift supports operator overloading, which is a fancy way of saying that what an operator does depends on the values you use it with. For example, + sums integers like this: let meaningOfLife = 42 let doubleMeaning = 42 + 42. But + also joins strings, like this:
Common operators in Swift. The most common operators that Swift ships with fall under the following categories: 1. Assignment operator. Assignment operators are used to assign values to a constant or variable. The symbol is =. 2. Arithmetic operators. These operators are used to perform basic arithmetic operations, such as +, -, *, /, and %. 3.
Swift has shorthand operators that combine one operator with an assignment, so you can change a variable in place. These look like the existing operators you know - +, -, *, and /, but they have an = on the end because they assign the result back to whatever variable you were using. For example, if someone scored 95 in an exam but needs to be ...
Swift operators are particularly powerful because you can alter them to suit your needs in two ways: assigning new functionality to existing operators (known as operator overloading), and creating new custom operators. Throughout this tutorial, you'll use a simple Vector struct and build your own set of operators to help compose different ...
Basic Operators. Perform operations like assignment, arithmetic, and comparison. An operator is a special symbol or phrase that you use to check, change, or combine values. For example, the addition operator (+) adds two numbers, as in let i = 1 + 2, and the logical AND operator (&&) combines two Boolean values, as in if enteredDoorCode ...
Another place where operator overloading in Swift is encouraged is with the Comparable protocol. To conform to the Comparable protocol you must implement an operator function for the " < " operator, as well as conform to the Equatable protocol. ... There are a few operators that you cannot overload, most notably the assignment operator ...
Swift Operator Overloading. Swift operator overloading allows developers to provide a custom implementation of existing operators for their types or create new operators with customized behavior. This feature is particularly powerful as it enables clean and intuitive usage of custom types, akin to Swift's built-in types.
Declaring the Operator. To define the function of an operator, itself has to be defined first. If we don't want to override or reuse an existing modifier, we must define its precedence and associativity ourselves.. The order of operations, also known as "operator precedence", defines the rules between different operators in expressions.
That syntactic position cannot take an arbitrary operator. But more importantly, the operation done by var someView = expression is initialization, not assignment — so even if an arbitrary operator were allowed in that position, what you're trying to do wouldn't be allowed, because it's not okay to bind an inout parameter to an uninitialized variable.
Updated for Xcode 16. Operator overloading allows the same operator - +, *, /, and so on - to do different things depending on what data you use it with. This allows us to use these symbols in various places where they would make sense: we can add two integers using +, we can append one string to another using +, we can join two arrays ...
note that the above operators won't get fired if one of the operands is an optional. also, if both the operator's parameters are optionals, and both the operands are non-optionals, it won't get fired. make both the operator's parameters implicitly unwrapped optionals to ensure it will always be fired for your custom class; but ensure nil cases are handled, otherwise your program may crash
In subclass B I override == with more checks. However, when I do comparison between two arrays of instances of B (which both have type Array<A>), == for A is invoked. Of course if I change type of both arrays to Array<B>, == for B is invoked. I came up with the following solution: if lhs is B && rhs is B {. return lhs as!