- PHP History
- Install PHP
- Hello World
- PHP Constant
- Predefined Constants
- PHP Comments
- Parameters and Arguments
- Anonymous Functions

Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
- If Condition
- If-else Block
- Break Statement
- Operator Precedence
- PHP Arithmetic Operators
- Assignment Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- PHP String Operators
- Array Operators
- Conditional Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
- Elvis Operator
- Null Coalescing Operator
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
- Programming
- PHP Tutorial
PHP variable function is a variable that is used to assign a function name with parentheses “()”. However, the PHP interpreter checks if it contains a callback or a function which should exist in the PHP project. If it doesn’t exist, the interpreter will produce an error.
How to Assign a Function to Variable using PHP
Assign a php function of class as a variable, variable function and arguments, wrapping up.
Let’s see how to do that.
According to this concept, PHP allow us to write a string inside a variable which can be called later as a function callback. Here is an example.
In this example, I assigned a string value to the variable “$func”, which contains the function name “codedtag”. Then, I defined the function with the same name as this string.
In the following line, I called the variable as a function “$func()”. However, when the interpreter reads it, it will connect the parentheses “()” with the string inside the variable and consider it as a callback.
Additionally, the PHP interpreter will consider this as a variable function, so if the function is not found during execution, the interpreter will stop executing the code immediately and show you an error message.
Here’s an example to demonstrate this scenario.
In this example, I called an undefined function, which is “calling_func”. That means PHP has to execute this function, “calling_func()”. But it is not defined yet in the document. So, it will show you the following error.
Fatal error: Uncaught Error: Call to undefined function calling_func() in /tmp/index.php:4 Stack trace: #0 {main} thrown in /tmp/index.php on line ..
Anyway, let’s focus on another aspect of PHP variable functions, which involves the functions inside a class.
In PHP, you can also use the methods of a class as variables with parentheses to call them once an instance of the class is created.
Here is an example:
In this example, we can see that the “$group” variable stores the name of the method, which can be called by using parentheses.
But, what if the method inside the class contains a static property? Let’s see how it works with the example below.
One way to call the function using the variable name as a function name is by using the scope resolution operator like this: $obj::$group() .
Moreover, there is another method that works on PHP 5.4 and above. It allows us to use a complex callback. For example:
Let’s see how it works with the static method.
In both of these examples, the array acts as a function by implementing the class object, with the function being callable once it finds that the main variable has parentheses.
You learned how to assign a function to a PHP variable, but how to pass arguments within? Let’s focus on this in the following section.
You can follow the same approach and pass the arguments into the parentheses of the variable function.
In the above example, the $func variable contains the string name “count”. When invoked with parentheses, PHP looks for the count() function, which requires a countable element as an argument.
However, some PHP predefined callbacks, such as language constructs (e.g., require, empty, include, isset, print, unset, and echo), will not work with variables.
This will show you a fetal error in PHP like the below.
Fatal error: Uncaught Error: Call to undefined function empty()
Anyway, Let’s summarize it.
During this tutorial, you learned what variable functions are and how to use them with a class, static methods, and more. Here is the explanation in a few points:
- The variable functions in PHP lets you use a variable as a function name. You assign the name of a function to a variable. And then, you call it with parentheses.
- Assign "codedtag" to a variable $func . Define a function codedtag() . Call $func() to execute codedtag() .
- If the assigned function doesn’t exist, PHP stops. It shows a fatal error. This means the function is undefined.
- You can assign methods from a class to variables. Call these methods through the variable. Use the scope resolution operator ( :: ) for static methods.
- Variable functions can take arguments. Include them within the calling parentheses.
- Some PHP constructs like require and empty can’t be variable functions. Trying this results in a fatal error.
Thank you for reading. Happy Coding!
Did you find this tutorial useful?
Your feedback helps us improve our tutorials.
PHP: Assign a function to a variable.
This is a short guide on how to assign an anonymous function to a variable in PHP and then call it. Note that these kind of functions are sometimes referred to as lambda functions .
Let’s start off with a simple example with no parameters:
In the code above, we created a very basic anonymous PHP function and assigned it to a variable called $myAnonymousFunction . As a result, we were then able to call the function in question by referencing $myAnonymousFunction .
Anonymous function with parameters.
Now, let’s create an anonymous PHP function that takes in parameters:
As you can see, this example doesn’t differ too much from our first one. The only difference is that it takes in a parameter called $name and prints it out.
Passing one anonymous function into another.
You can also pass one anonymous function in as a parameter to another anonymous function:
In the code above, we created two functions and assigned them to PHP variables. The first function prints out a string, whereas the second one takes in a function as a parameter before calling it. Furthermore, we passed the first function into the second function.
As a result, the script above will print the words “Hi everybody” to the browser.
Crazy, right?
Oh, and by the way, I am not sorry for putting Dr. Nick’s voice in your head.
Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Operator | Example | Equivalent | Operation |
---|---|---|---|
+= | $x += $y | $x = $x + $y | Addition |
-= | $x -= $y | $x = $x – $y | Subtraction |
*= | $x *= $y | $x = $x * $y | Multiplication |
/= | $x /= $y | $x = $x / $y | Division |
%= | $x %= $y | $x = $x % $y | Modulus |
**= | $z **= $y | $x = $x ** $y | Exponentiation |
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
- Variable Assignment, Expressions, and Operators
Variables are containers for storing information, such as numbers or text so that they can be used multiple times in the code. Variables in PHP are identified by a dollar sign ($) followed by the variable name. A variable name must begin with a letter or the underscore character and only contain alphanumeric characters and underscores. A variable name cannot contain spaces. Finally, variable names in PHP are case-sensitive.
- Post author By BrainBell
- Post date May 12, 2022
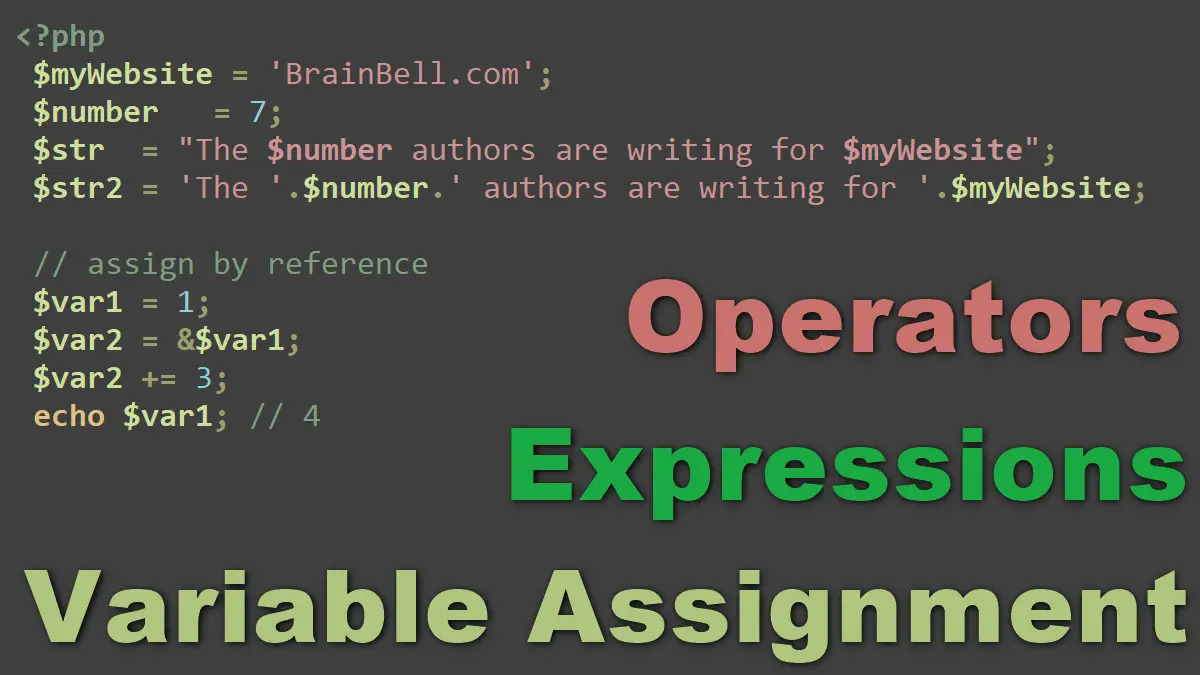
This tutorial covers the following topics:
- Define a variable
- Assign a variable by reference
- Assign a string value to a variable
Assignment Operators
- Arithmetic Operators (See Comparison Operators and Logical Operators on Conditional Expression Tutorial).
Operator precedence
Expressions, define a variable.
PHP uses the = symbol as an assignment operator. The variable goes on the left of the equal sign, and the value goes on the right. Because it assigns a value, the equal sign is called the assignment operator .
$ variableName = 'Assigned Value' ;
You can break the above example into the following parts:
- A dollar sign $ prefix
- Variable name
- The assignment operator (equal sign = )
- Assigned value
- Semicolon to terminate the statement
A PHP variable must be defined before it can be used. Attempting to use an undefined variable will trigger an error exception:
In PHP, you do not need to declare a variable separately before using it. Just assign value to a variable by using the assignment operator (equals sign = ) to make it defined or initialized:
A defined variable can be used by referencing its name, for example, use the print or echo command (followed by the variable’s name) to display the value of the variable on the web page:
Variable names are case-sensitive in PHP, so $Variable , $variable , $VAriable , and $VARIABLE are all different variables.
Text requires quotes
If you look closely at the PHP code block in the above example, you’ll notice that the value assigned to the second variable isn’t enclosed in quotes. It looks like this:
Then the ‘BrainBell.com’ did use quotes, like this:
The simple rules are as follows:
- The text requires quotes (single or double)
- No quotes are required for numbers, True , False and Null
Assign a string value to a variable:
Concatenate two strings together to produce “test string”:
Add a string to the end of another to produce “test string”:
Here is a shortcut to adding a string to the end of another:
Assign by reference
By default, PHP assigns all variables other than objects by value and not by reference. PHP has optimizations to make assignment by value faster than assigning by reference, but if you want to assign by reference you can use the & operator as follows:
The assignment operator (equal = ) can be combined with other operators to make it easier to write certain expressions. See the following table:
Operator | Example | Same as |
---|---|---|
= | $a = 2; | |
+= | $a += 2; | $a = $a + 2; |
-= | $a -= 2; | $a = $a – 2; |
/= | $a /= 2; | $a = $a / 2; |
%= | $a %= 2; | $a = $a % 2; |
.= | $a .= $b; | $a = $a . $b; |
*= | $a *= 2; | $a = $a * 2; |
These operators assign values to variables. They start with the assignment operator = and move on to += , -= , etc.(see above table). The operator += adds the value on the right side to the variable on the left:
Arithmetic Operators
Using an operator, you can manipulate the contents of one or more variables or constants to produce a new value. For example, this code uses the addition operator ( + ) to add the values of $x and $y together to produce a new value:
So an operator is a symbol that manipulates one or more values, usually producing a new value in the process. The following list describes the types of arithmetic operators:
Operator | Description |
---|---|
sum or addition | |
subtraction | |
division | |
multiplication | |
modulus | |
exponentiation (PHP 5.6 and above) | |
add 1 | |
subtract 1 |
Sum integers to produce an integer:
The values and variables that are used with an operator are known as operands.
Subtraction, multiplication, and division might have a result that is a float or an integer, depending on the initial value of $var :
Multiply to double a value:
Halve a value:
These work with float types too:
Get the remainder of dividing 5 by 4:
4 exponent (or power) of 2:
These all add 1 to $var:
And these all subtract 1 from $var:
If the -- or ++ operator appears before the variable then the interpreter will first evaluate it and then return the changed variable:
If the -- or ++ operator appears after the variable then the interpreter will return the variable as it was before the statement run and then increment the variable:
There are many mathematical functions available in the math library of PHP for more complex tasks. We introduce some of these in the next pages.
The precedence of operators in an expression is similar to the precedence defined in any other language. Multiplication and division occur before subtraction and addition, and so on. However, reliance on evaluation orders leads to unreadable, confusing code. Rather than memorize the rules, we recommend you construct unambiguous expressions with parentheses because parentheses have the highest precedence in evaluation.
For example, in the following fragment $variable is assigned a value of 32 because of the precedence of multiplication over addition:
The result is much clearer if parentheses are used:
But the following example displays a different result because parentheses have the highest precedence in evaluation.
An expression in PHP is anything that evaluates a value; it is a combination of values, variables, operators, and functions that results in a value. Here are some examples of expressions:
An expression has a value and a type; for example, the expression 4 + 7 has the value 11 and the type integer, and the expression "abcdef" has the value abcdef and the type string . PHP automatically converts types when combining values in an expression. For example, the expression 4 + 7.0 contains an integer and a float; in this case, PHP considers the integer as a floating-point number, and the result is a float. The type conversions are largely straightforward; however, there are some traps, which are discussed later in this section.
Getting Started with PHP:
- Introducing PHP
- PHP Development Environment
- Delimiting Strings
- Variable Substitution
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php functions.
The real power of PHP comes from its functions.
PHP has more than 1000 built-in functions, and in addition you can create your own custom functions.
PHP Built-in Functions
PHP has over 1000 built-in functions that can be called directly, from within a script, to perform a specific task.
Please check out our PHP reference for a complete overview of the PHP built-in functions .
PHP User Defined Functions
Besides the built-in PHP functions, it is possible to create your own functions.
- A function is a block of statements that can be used repeatedly in a program.
- A function will not execute automatically when a page loads.
- A function will be executed by a call to the function.
Create a Function
A user-defined function declaration starts with the keyword function , followed by the name of the function:
Note: A function name must start with a letter or an underscore. Function names are NOT case-sensitive.
Tip: Give the function a name that reflects what the function does!

Call a Function
To call the function, just write its name followed by parentheses () :
In our example, we create a function named myMessage() .
The opening curly brace { indicates the beginning of the function code, and the closing curly brace } indicates the end of the function.
The function outputs "Hello world!".
Advertisement
PHP Function Arguments
Information can be passed to functions through arguments. An argument is just like a variable.
Arguments are specified after the function name, inside the parentheses. You can add as many arguments as you want, just separate them with a comma.
The following example has a function with one argument ($fname) . When the familyName() function is called, we also pass along a name, e.g. ("Jani") , and the name is used inside the function, which outputs several different first names, but an equal last name:
The following example has a function with two arguments ($fname, $year) :
PHP Default Argument Value
The following example shows how to use a default parameter. If we call the function setHeight() without arguments it takes the default value as argument:
PHP Functions - Returning values
To let a function return a value, use the return statement:
Passing Arguments by Reference
In PHP, arguments are usually passed by value, which means that a copy of the value is used in the function and the variable that was passed into the function cannot be changed.
When a function argument is passed by reference, changes to the argument also change the variable that was passed in. To turn a function argument into a reference, the & operator is used:
Use a pass-by-reference argument to update a variable:
Variable Number of Arguments
By using the ... operator in front of the function parameter, the function accepts an unknown number of arguments. This is also called a variadic function.
The variadic function argument becomes an array.
A function that do not know how many arguments it will get:
You can only have one argument with variable length, and it has to be the last argument.
The variadic argument must be the last argument:
If the variadic argument is not the last argument, you will get an error.
Having the ... operator on the first of two arguments, will raise an error:
PHP is a Loosely Typed Language
In the examples above, notice that we did not have to tell PHP which data type the variable is.
PHP automatically associates a data type to the variable, depending on its value. Since the data types are not set in a strict sense, you can do things like adding a string to an integer without causing an error.
In PHP 7, type declarations were added. This gives us an option to specify the expected data type when declaring a function, and by adding the strict declaration, it will throw a "Fatal Error" if the data type mismatches.
In the following example we try to send both a number and a string to the function without using strict :
To specify strict we need to set declare(strict_types=1); . This must be on the very first line of the PHP file.
In the following example we try to send both a number and a string to the function, but here we have added the strict declaration:
The strict declaration forces things to be used in the intended way.
PHP Return Type Declarations
PHP 7 also supports Type Declarations for the return statement. Like with the type declaration for function arguments, by enabling the strict requirement, it will throw a "Fatal Error" on a type mismatch.
To declare a type for the function return, add a colon ( : ) and the type right before the opening curly ( { )bracket when declaring the function.
In the following example we specify the return type for the function:
You can specify a different return type, than the argument types, but make sure the return is the correct type:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
PHP - assigning function return to variable
I have a PHP script that returns a random password. How do I echo out the password that is generated?
- 3 do you wrote all that code and you don't know about echo? – dynamic Commented Feb 20, 2011 at 23:32
2 Answers 2
As prodigitalson mentioned, you can directly echo out the return value or assign it to a variable, eg
err.. change
Or.. better yet:
- 7 Functions that echo are so Wordpress. Also, "Wordpress" is my new vernacular for "bad", hopefully it catches on ;) – Phil Commented Feb 20, 2011 at 23:36
- Hahaha, I agree on that (function that echo are bad). But, I don't want to start 'religion' wars ;) – ariefbayu Commented Feb 20, 2011 at 23:55
- @Phil Or you could just use the right function. get_ in front of the function name will return the output instead of echo'ing. – Archonic Commented Oct 24, 2014 at 3:39
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php or ask your own question .
- The Overflow Blog
- Where developers feel AI coding tools are working—and where they’re missing...
- He sold his first company for billions. Now he’s building a better developer...
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Should low-scoring meta questions no longer be hidden on the Meta.SO home...
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- How long have people been predicting the collapse of the family?
- Print 4 billion if statements
- How can I draw a wedge of a cylinder?
- How do you tell someone to offer something to everyone in a room by taking it physically to everyone in the room so everyone can have it?
- Is there any reason _not_ to add a flyback diode?
- What is "illegal, immoral or improper" use in CPOL?
- On a glassed landmass, how long would it take for plants to grow?
- Can a floppy disk be wiped securely using the Windows format command with the passes-parameter?
- Boon of the Night Spirit when leaving dim light
- Can I breed fish in Minecraft?
- Tikz template for organization chart
- What is an ellipse in infinite-dimensional spaces?
- Does the Rogue's Evasion cost a reaction?
- Is Mankiw 's answer really correct? The expected benefit from installing the traffic light. ("Principles of Economics 9e" by Mankiw.)
- Model files not opening with Cura after removing old version
- How do I avoid getting depressed after receiving edits?
- Asymptotics of an entire function with real zeroes on the real line
- How is the universe able to run physics so smoothly?
- What should you list as location in job application?
- Would "deferring to scientists" in the case of philosophical grounding theory, mean adapting metaphysical grounding talk to mathematical such talk?
- How do I link a heading containing spaces in Markdown?
- Can a US president put a substantial tariff on cars made in Mexico, within the confines of USMCA?
- A military space Saga with a woman who is a brilliant tactician and strategist
- Why did the Apollo 13 tank #2 have a heater and a vacuum?
- Language Reference
Variables in PHP are represented by a dollar sign followed by the name of the variable. The variable name is case-sensitive.
Variable names follow the same rules as other labels in PHP. A valid variable name starts with a letter or underscore, followed by any number of letters, numbers, or underscores. As a regular expression, it would be expressed thus: ^[a-zA-Z_\x80-\xff][a-zA-Z0-9_\x80-\xff]*$
Note : For our purposes here, a letter is a-z, A-Z, and the bytes from 128 through 255 ( 0x80-0xff ).
Note : $this is a special variable that can't be assigned. Prior to PHP 7.1.0, indirect assignment (e.g. by using variable variables ) was possible.
See also the Userland Naming Guide .
For information on variable related functions, see the Variable Functions Reference .
<?php $var = 'Bob' ; $Var = 'Joe' ; echo " $var , $Var " ; // outputs "Bob, Joe" $ 4site = 'not yet' ; // invalid; starts with a number $_4site = 'not yet' ; // valid; starts with an underscore $täyte = 'mansikka' ; // valid; 'ä' is (Extended) ASCII 228. ?>
By default, variables are always assigned by value. That is to say, when you assign an expression to a variable, the entire value of the original expression is copied into the destination variable. This means, for instance, that after assigning one variable's value to another, changing one of those variables will have no effect on the other. For more information on this kind of assignment, see the chapter on Expressions .
PHP also offers another way to assign values to variables: assign by reference . This means that the new variable simply references (in other words, "becomes an alias for" or "points to") the original variable. Changes to the new variable affect the original, and vice versa.
To assign by reference, simply prepend an ampersand (&) to the beginning of the variable which is being assigned (the source variable). For instance, the following code snippet outputs ' My name is Bob ' twice: <?php $foo = 'Bob' ; // Assign the value 'Bob' to $foo $bar = & $foo ; // Reference $foo via $bar. $bar = "My name is $bar " ; // Alter $bar... echo $bar ; echo $foo ; // $foo is altered too. ?>
One important thing to note is that only named variables may be assigned by reference. <?php $foo = 25 ; $bar = & $foo ; // This is a valid assignment. $bar = &( 24 * 7 ); // Invalid; references an unnamed expression. function test () { return 25 ; } $bar = & test (); // Invalid. ?>
It is not necessary to initialize variables in PHP however it is a very good practice. Uninitialized variables have a default value of their type depending on the context in which they are used - booleans default to false , integers and floats default to zero, strings (e.g. used in echo ) are set as an empty string and arrays become to an empty array.
Example #1 Default values of uninitialized variables
Relying on the default value of an uninitialized variable is problematic in the case of including one file into another which uses the same variable name. E_WARNING (prior to PHP 8.0.0, E_NOTICE ) level error is issued in case of working with uninitialized variables, however not in the case of appending elements to the uninitialized array. isset() language construct can be used to detect if a variable has been already initialized.
Improve This Page
User contributed notes 2 notes.


COMMENTS
Variable functions. ¶. PHP supports the concept of variable functions. This means that if a variable name has parentheses appended to it, PHP will look for a function with the same name as whatever the variable evaluates to, and will attempt to execute it. Among other things, this can be used to implement callbacks, function tables, and so forth.
This question is about assigning a function/method to a variable so the variable behaves like a Closure. The "php call class function by string name" is about using a variable string in place of the actual function/method name. The variable string does not behave like a Closure. -
PHP - Variable assignment in function call. Ask Question Asked 11 years, 8 months ago. Modified 7 years, 6 months ago. Viewed 95 times ... Variable assignment and functions - this should work right? 4. Multiple assignments to the same variable. 1. variable inside variable in a PHP function? 1.
This way, any changes made to the variable in one function are reflected when accessed in another function. The usage of global variables should be handled with caution to avoid creating dependencies and potential conflicts, but in some cases, it can be a practical solution for sharing variables between functions.
Function Reference Affecting PHP's Behaviour Audio Formats Manipulation ... Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference.
During this tutorial, you learned what variable functions are and how to use them with a class, static methods, and more. Here is the explanation in a few points: The variable functions in PHP lets you use a variable as a function name. You assign the name of a function to a variable. And then, you call it with parentheses.
In PHP, variables can be declared anywhere in the script. ... Then, each time the function is called, that variable will still have the information it contained from the last time the function was called. Note: The variable is still local to the function. Previous Next ...
Summary: in this tutorial, you will learn about the PHP variable functions and how to use them to call a function, a method of an object, and a class's static method. Introduction to PHP variable functions. Variable functions allow you to use a variable like a function.
As of PHP 8.0.0, the list of scope-inherited variables may include a trailing comma, which will be ignored. Inheriting variables from the parent scope is not the same as using global variables. Global variables exist in the global scope, which is the same no matter what function is executing.
This is a short guide on how to assign an anonymous function to a variable in PHP and then call it. Note that these kind of functions are sometimes referred to as lambda functions. Let's start off with a simple example with no parameters: //Create an anonymous function and assign it to a PHP variable.
In PHP global variables must be declared global inside a function if they are going to be used in that function. The global ... I had to add the following code to my safeinclude function (before variables are used or file is included) ... When you assign some variable value by reference you in fact write address of source variable to recepient ...
Rules for PHP variables: A variable starts with the $ sign, followed by the name of the variable. A variable name must start with a letter or the underscore character. A variable name cannot start with a number. A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
Use PHP assignment operator (=) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator (.=)to concatenate strings and assign the result to a variable in a single statement.
Variables in PHP are identified by a dollar sign ($) followed by the variable name. ... These operators assign values to variables. They start with the assignment operator = and move on to +=, -=, etc. ... There are many mathematical functions available in the math library of PHP for more complex tasks. We introduce some of these in the next pages.
PHP User Defined Functions. Besides the built-in PHP functions, it is possible to create your own functions. A function is a block of statements that can be used repeatedly in a program. A function will not execute automatically when a page loads. A function will be executed by a call to the function.
In addition, it is possible to use associative array to secure name of variables available to be used within a function (or class / not tested). This way the variable variable feature is useful to validate variables; define, output and manage only within the function that receives as parameter an associative array :
Function arguments. ¶. Information may be passed to functions via the argument list, which is a comma-delimited list of expressions. The arguments are evaluated from left to right, before the function is actually called (eager evaluation). PHP supports passing arguments by value (the default), passing by reference, and default argument values.
Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand; OverflowAI GenAI features for Teams; OverflowAPI Train & fine-tune LLMs; Labs The future of collective knowledge sharing; About the company Visit the blog
Basics. ¶. Variables in PHP are represented by a dollar sign followed by the name of the variable. The variable name is case-sensitive. Variable names follow the same rules as other labels in PHP. A valid variable name starts with a letter or underscore, followed by any number of letters, numbers, or underscores.