- Español – América Latina
- Português – Brasil
- Tiếng Việt

Create and use variables in Kotlin
1. before you begin.
In the apps that you use on your phone, notice that some parts of the app stay the same, while other parts change (or are variable).
For example, the names of the categories within the Settings app stay the same – Network & internet, Connected devices, Apps, and more.
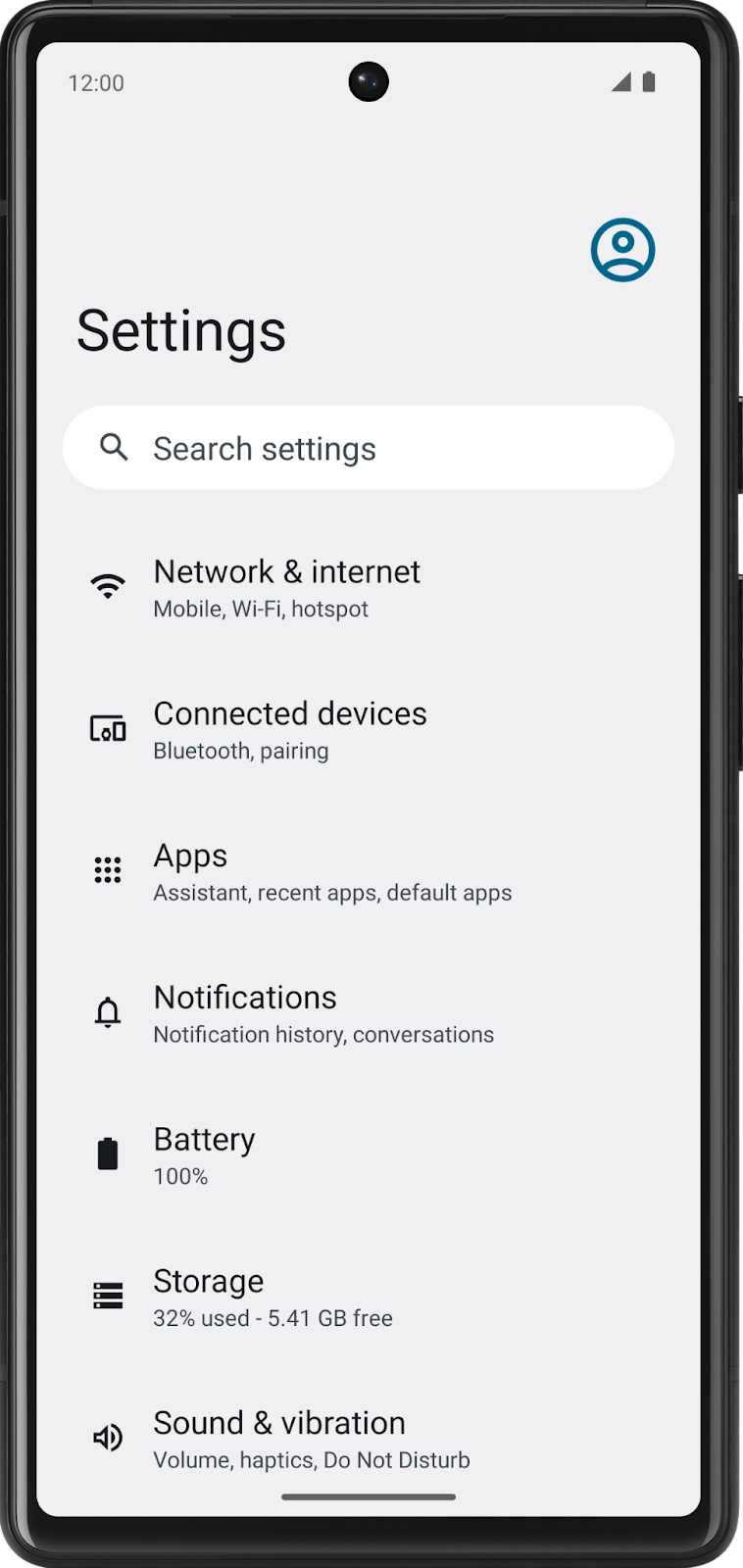
On the other hand, if you look at a news app, the articles will change often. The article name, source, time posted, and images change.
How do you write your code so that content changes over time? You can't rewrite the code in your app every time there are new articles to post, which happens every day, every hour, and every minute!
In this codelab, you learn how to write code that uses variables so that certain parts of your program can change without having to write a whole new set of instructions. You will use the Kotlin Playground as you did in the previous codelab.
What you'll build
- Short programs in Kotlin that use variables.
What you'll learn
- How to define a variable and update its value.
- How to select an appropriate data type for a variable from the basic data types in Kotlin.
- How to add comments to your code.
What you'll need
- A computer with internet access and a web browser.
2. Variables and data types
In computer programming, there's the concept of a variable , which is a container for a single piece of data. You can envision it as a box that contains a value. The box has a label, which is the name of the variable. By referring to the box by its name, you have access to the value it holds.
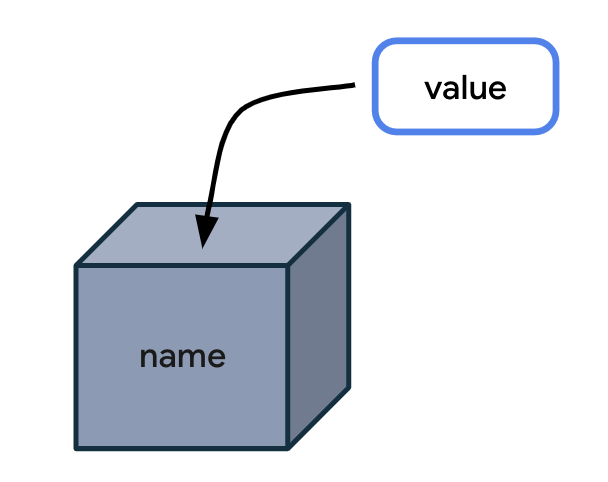
Why store the value in a box and reference the box by its name when you can simply use the value directly? The problem is that when your code uses values directly in all the instructions, your program will only work for that specific case.
Here's an analogy that can make it easier to understand why variables are useful. The following is a letter for someone you recently met.
Dear Lauren,
It was great meeting you today at the office. I look forward to seeing you on Friday.
Have a nice day!
This letter is great, but it only works for your specific situation with Lauren. What if you find yourself writing the same letter many times but with slight variations for different people? It would be more efficient to create a single letter template, leaving blanks for the parts that can change.
Dear ____ ,
It was great meeting you today at _____. I look forward to seeing you on ____ .
You can also specify the type of information that goes in each blank space. This ensures that the letter template will be used as you expected.
Dear { name } ,
It was great meeting you today at { location } . I look forward to seeing you on { date } .
Conceptually, building an app is similar. You have placeholders for some data, while other parts of the app stay the same.
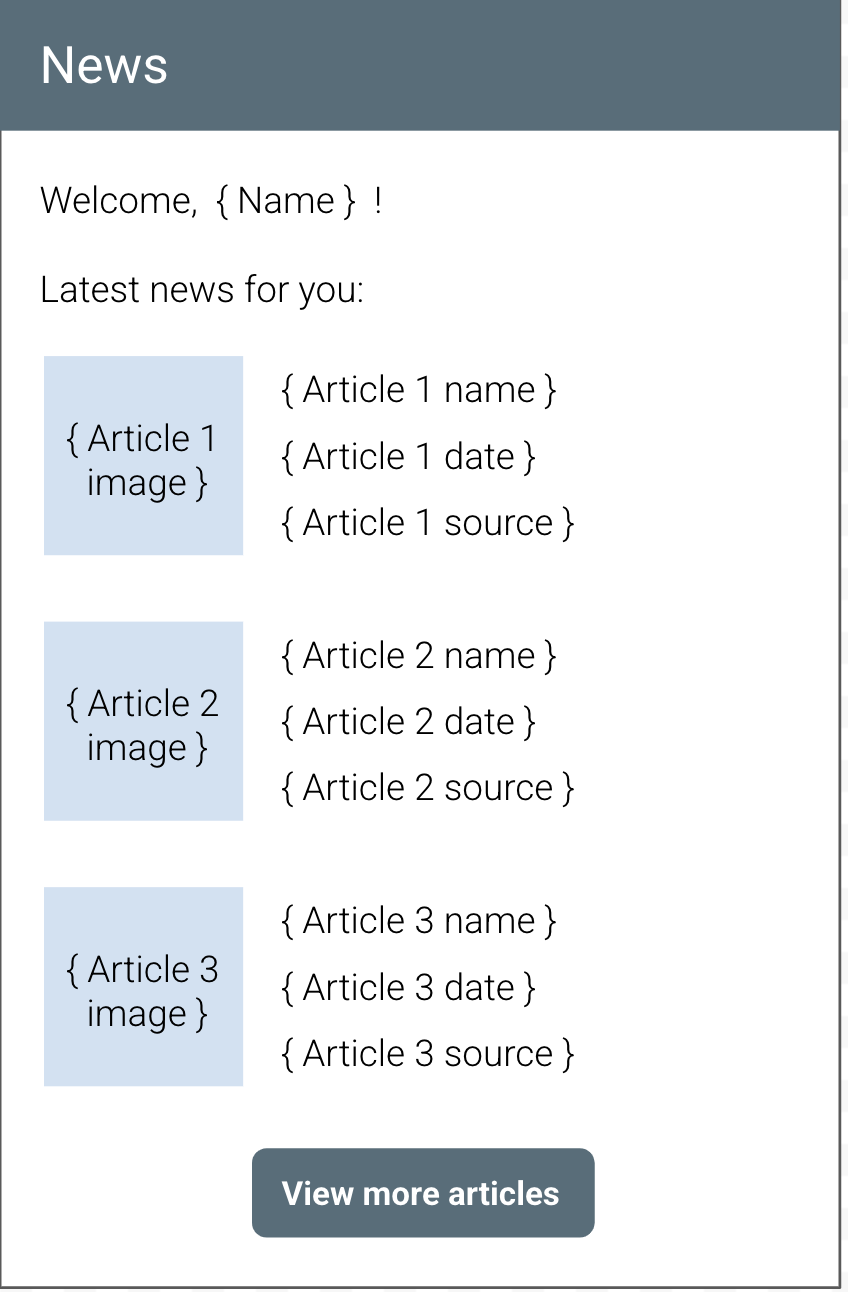
In the above illustration of a news app, the "Welcome" text, the "Latest news for you" heading, and the "View more articles" button text always stay the same. Conversely, the name of the user and the contents of each article will change, so that would be a great opportunity to use variables to hold each piece of information.
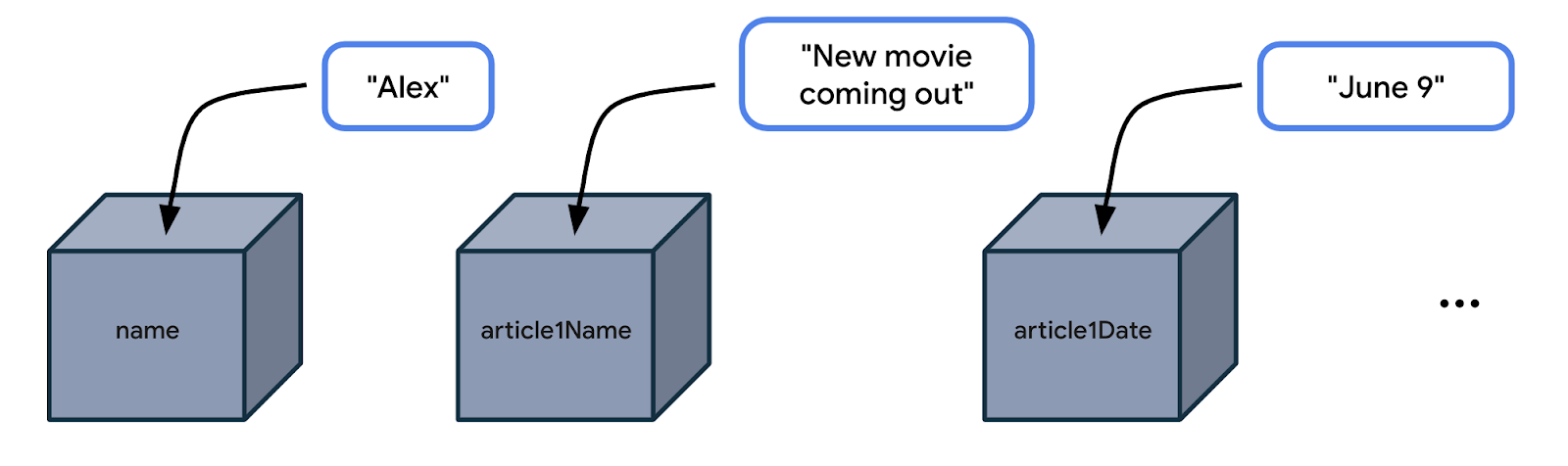
You don't want to write the code (or instructions) in your news app to only work for a user named Alex, or for a news article that always has the same title and publication date. Instead, you want a more flexible app, so you should write your code by referencing variable names like name , article1Name , article1Date , and so on. Then your code becomes general enough to work for many different use cases where the user's name could be different and the article details could be different.
Example app with variables
Let's look at an app example to see where it may use variables.
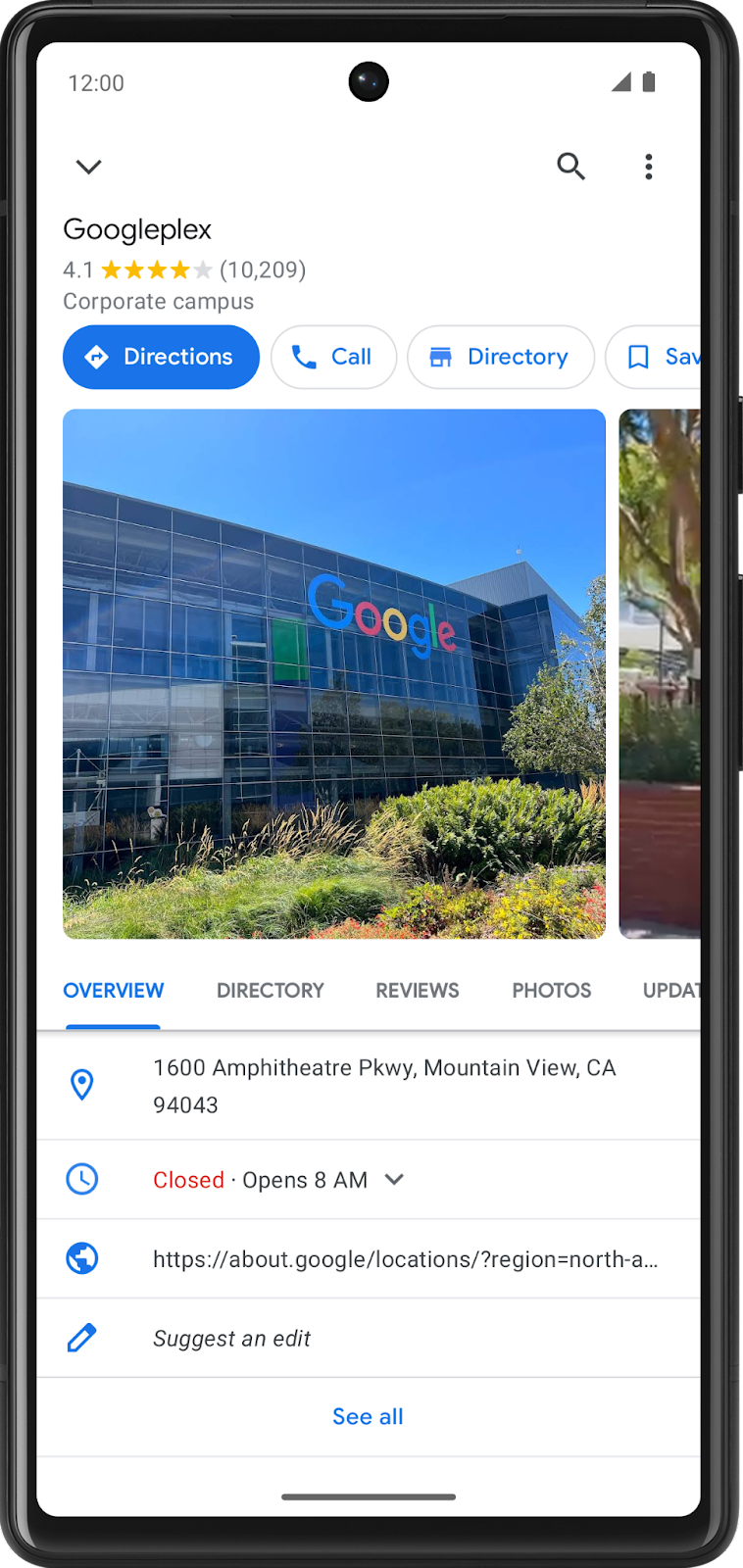
In a maps app, you may find a details screen for each location, such as a restaurant or business. The above screenshot from the Google Maps app shows the details for Google's company headquarters, which is called the Googleplex. Which pieces of data do you think are stored as variables in the app?
- Name of the location
- Star rating of the location
- Number of reviews of the location
- Whether the user saved (or bookmarked) this location
- Address of the location
Change the data that's stored in these variables and you have a maps app that's flexible enough to display the details of other locations too.
When you decide what aspects of your app can be variable, it's important to specify what type of data can be stored in those variables. In Kotlin, there are some common basic data types. The table below shows a different data type in each row. For each data type, there's a description of what kind of data it can hold and example values.
Now that you are aware of some common Kotlin data types, which data type would be appropriate for each of the variables identified in the location detail page you saw earlier?
- Name of the location is text, so it can be stored in a variable whose data type is String .
- Star rating of the location is a decimal number (such as 4.2 stars), so it can be stored as a Double .
- Number of reviews of the location is a whole number, so it should be stored as an Int .
- Whether the user saved this location only has two possible values (saved or not saved), so it's stored as a Boolean , where true and false can represent each of those states.
- Address of the location is text, so it should be a String .
Practice on two more scenarios below. Identify the use of variables and their data types in the following apps.
- In an app for watching videos, such as the YouTube app, there's a video details screen. Where are variables likely used? What's the data type of those variables?
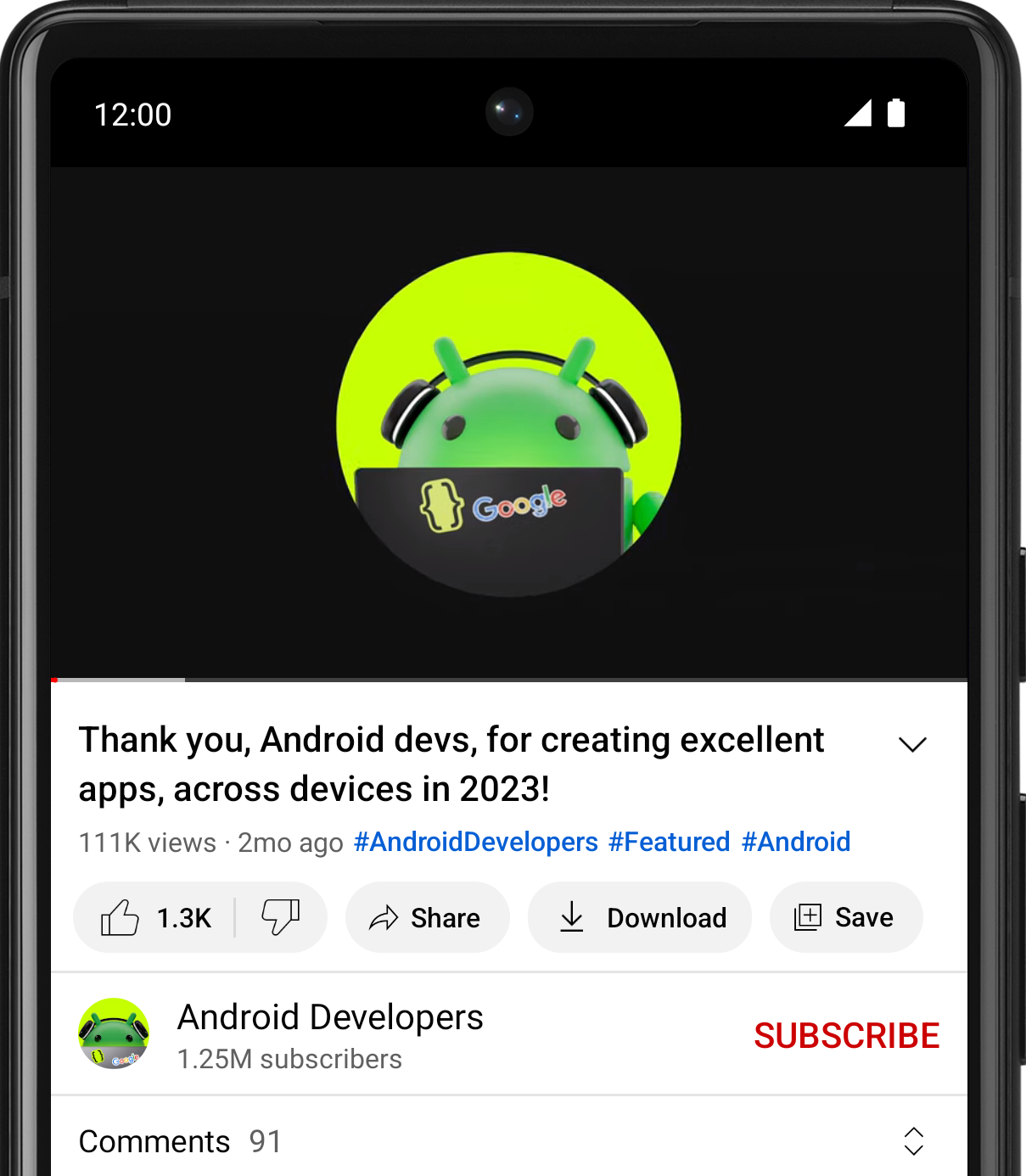
There isn't a single correct answer, but in a video-watching app, variables can be used for the following pieces of data:
- Name of the video ( String )
- Name of the channel ( String )
- Number of views on the video ( Int )
- Number of likes on the video ( Int )
- Number of comments on the video ( Int )
- In an app like Messages, the screen lists the most recent text messages received. Where are variables likely used? What's the data type of those variables?
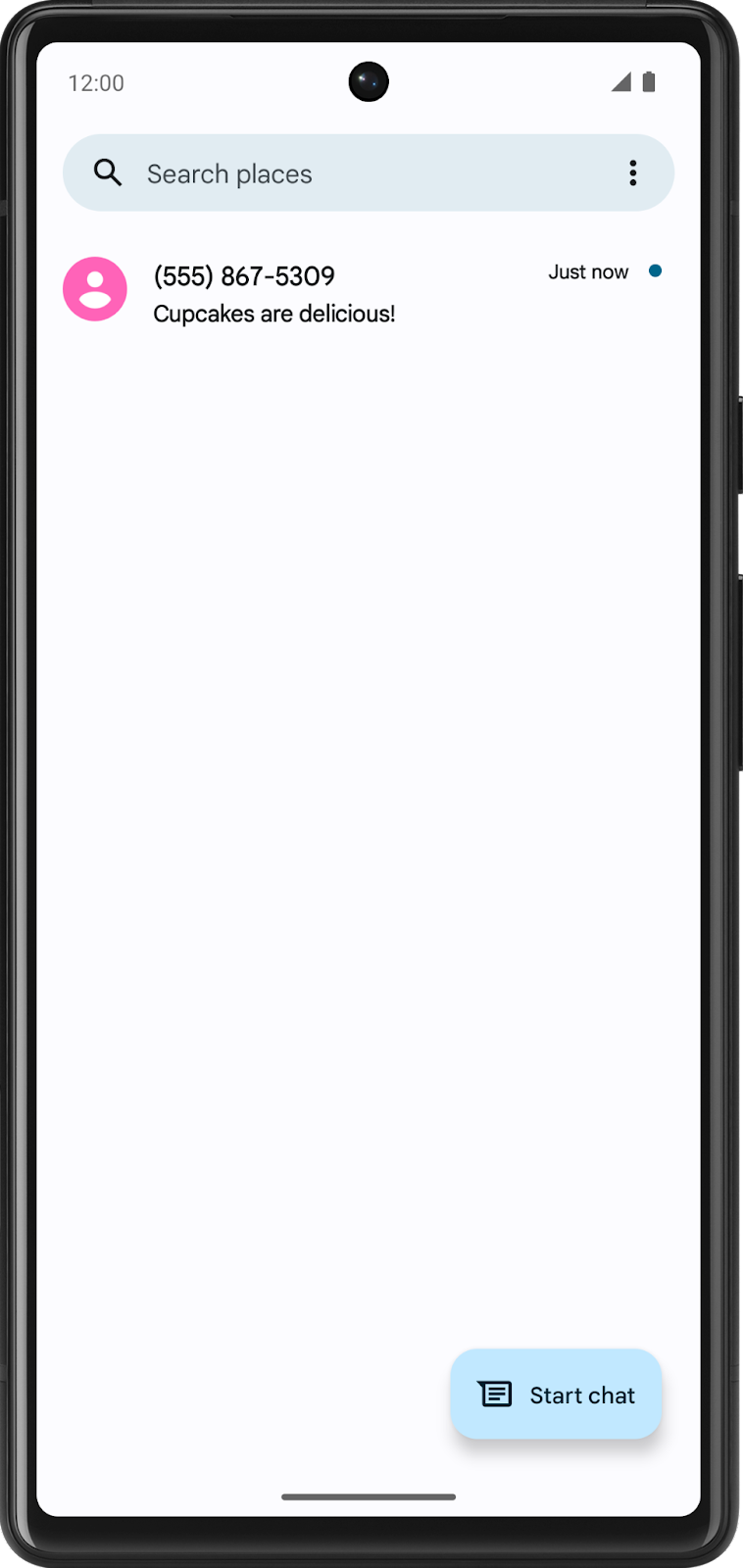
Again, there isn't a single correct answer. In an text messaging app, variables can be used for the following pieces of data:
- Phone number of the sender ( String )
- Timestamp of the message ( String )
- Preview of the message contents ( String )
- Whether the text message is unread ( Boolean )
- Open your favorite app on your phone.
- Identify where you think variables are used in the app on that particular screen.
- Guess what data type those variables are.
- Share your answers on social media with a screenshot of the app, an explanation of where you think variables are used, and the hashtag #AndroidBasics.
Great work in this codelab so far! Move onto the next section to learn more about how variables and data types are used in your code.
3. Define and use variables
Define versus use a variable.
You must define a variable first in your code before you can use the variable. This is similar to what you learned in the last codelab about defining functions before calling them.
When you define a variable, you assign a name to uniquely identify it. You also decide what type of data it can hold by specifying the data type. Lastly, you can provide an initial value that will be stored in the variable, but this is optional.
Once you define a variable, you can use that variable in your program. To use a variable, type out the variable name in your code, which tells the Kotlin compiler that you want to use the variable's value at that point in the code.
For example, define a variable for the number of unread messages in a user's inbox. The variable can have the name count . Store a value such as the number 2 inside the variable, representing 2 unread messages in the user's inbox. (You can pick a different number to store in the variable, but for the purpose of this example, use the number 2 .)
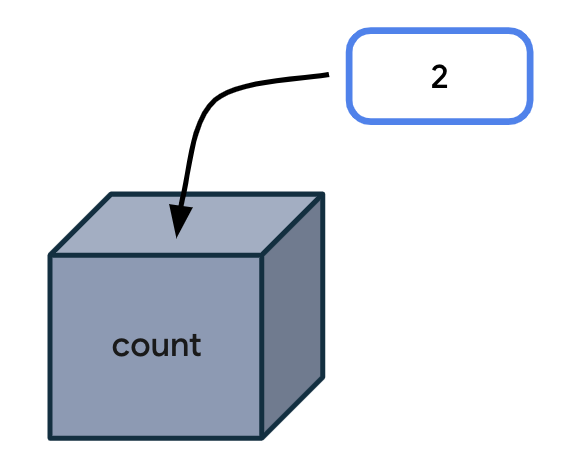
Every time your code needs to access the number of unread messages, type count in your code. When executing your instructions, the Kotlin compiler sees the variable name in your code and uses the variable value in its place.
Technically, there are more specific vocabulary words to describe this process:
An expression is a small unit of code that evaluates to a value. An expression can be made up of variables, function calls, and more. In the following case, the expression is made up of one variable: the count variable. This expression evaluates to 2 .
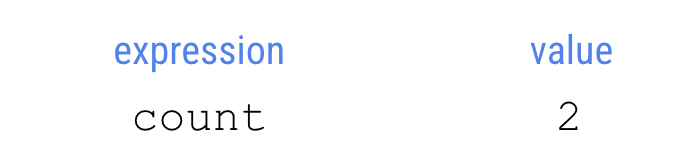
Evaluate means determining the value of an expression. In this case, the expression evaluates to 2 . The compiler evaluates expressions in the code and uses those values when executing the instructions in the program.
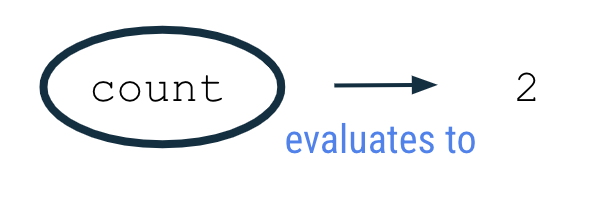
To observe this behavior in the Kotlin Playground, run the program in the next section.
- Open the Kotlin Playground in a web browser.
- Replace the existing code in the Kotlin Playground with the following program.
This program creates a variable called count with an initial value of 2 and uses it by printing out the value of the count variable to the output. Don't worry if you don't understand all aspects of the code syntax yet. It will be explained in more detail in upcoming sections.
- Run the program and the output should be:
Variable declaration
In the program you ran, the second line of code says:
This statement creates an integer variable called count that holds the number 2 .
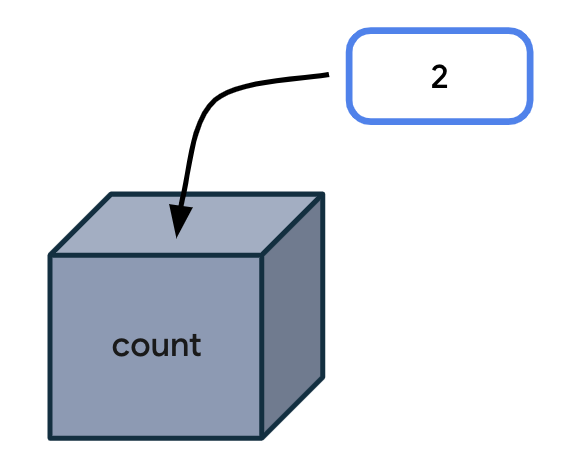
It can take a while to become familiar with reading the syntax (or format) for declaring a variable in Kotlin. The following diagram shows where each detail about the variable should be located, as well as the location of spaces and symbols.

In the context of the count variable example, you can see that the variable declaration starts with the word val . The name of the variable is count . The data type is Int , and the initial value is 2 .
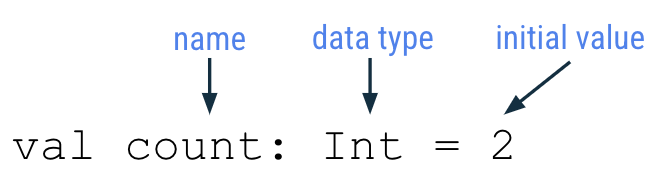
Each part of the variable declaration is explained in more detail below.
Keyword to define new variable
To define a new variable, start with the Kotlin keyword val (which stands for value). Then the Kotlin compiler knows that a variable declaration is in this statement.
Variable name
Just like you name a function, you also name a variable. In the variable declaration, the variable name follows the val keyword.

You can choose any variable name you want, but as a best practice, avoid using Kotlin keywords as a variable name.
It's best to choose a name that describes the data that the variable holds so that your code is easier to read.
Variable names should follow the camel case convention, as you learned with function names. The first word of the variable name is all lower case. If there are multiple words in the name, there are no spaces between words, and all other words should begin with a capital letter.
Example variable names:
- numberOfEmails
- bookPublicationDate
For the code example shown earlier, count is the name of the variable.
Variable data type
After the variable name, you add a colon, a space, and then the data type of the variable. As mentioned earlier, String , Int , Double , Float, and Boolean are some of the basic Kotlin data types. You'll learn more data types later in this course. Remember to spell data types exactly as shown and begin each with a capital letter.

For the count variable example, Int is the data type of the variable.
Assignment operator
In the variable declaration, the equal sign symbol ( = ) follows the data type. The equal sign symbol is called the assignment operator . The assignment operator assigns a value to the variable. In other words, the value on the right-hand side of the equal sign gets stored in the variable on the left-hand side of the equal sign.
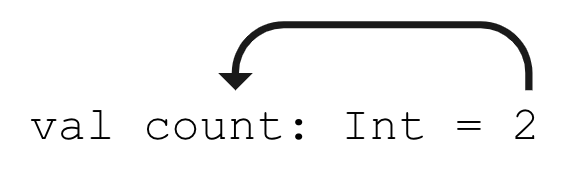
Variable initial value
The variable value is the actual data that's stored in the variable.

For the count variable example, the number 2 is the initial value of the variable.
You may also hear the phrase: "the count variable is initialized to 2 ." This means that 2 is the first value stored in the variable when the variable is declared.
The initial value will be different depending on the data type declared for the variable.
Refer to the following table which you may recognize from earlier in the codelab. The third column shows example values that can be stored in a variable of each corresponding type. These values are called literals because they are fixed or constant values (the value is constantly the same). As an example, the integer 32 is always going to have the value of 32. In contrast, a variable is not a literal because its value can change. You may hear these literal values referred to based on their type: string literal, integer literal, boolean literal, etc.
It's important to provide an appropriate and valid value according to the data type of the variable. For instance, you can't store a string literal like "Hello" inside a variable of type Int because the Kotlin compiler will give you an error.
Use a variable
The following is the original program you ran in the Kotlin Playground. So far you learned that the second line of code creates a new integer variable called count with a value of 2 .
Now look at the third line of code. You are printing the count variable to the output:
Notice there are no quotation marks around the word count . It is a variable name, not a string literal. (You would find quotation marks around the word if it was a string literal.) When you run the program, the Kotlin compiler evaluates the expression inside the parentheses, which is count , for the println() instruction. Since the expression evaluates to 2 , then the println() method is called with 2 as the input: println(2) .
Hence the output of the program is:
The number by itself in the output is not very useful. It would be more helpful to have a more detailed message printed in the output to explain what the 2 represents.
String template
A more helpful message to display in the output is:
Follow these steps so that the program outputs a more helpful message.
- Update your program in the Kotlin Playground with the following code. For the println() call, pass in a string literal that contains the count variable name. Remember to surround the text with quotation marks. Note that this will not give you the results you expect. You'll fix the issue in a later step.
- Run the program and the output should display:
That sentence doesn't make sense! You want the value of the count variable to be displayed in the message, not the variable name.
- To fix the output, you need a string template. This is a string template because it contains a template expression , which is a dollar sign ( $ ) followed by a variable name. A template expression is evaluated, and its value gets substituted into the string.

Add a dollar sign $ before the count variable. In this case, the template expression $count evaluates to 2 , and the 2 gets substituted into the string where the expression was located.
- When you run the program, the output matches the desired goal:
That sentence makes much more sense to the user!
- Now change the initial value of the count variable to a different integer literal. For example, you could choose the number 10 . Leave the rest of the code in the program unchanged.
- Run the program. Notice that the output changes accordingly and you didn't even need to change the println() statement in your program.
You can see how useful a string template can be. You only wrote the string template once in your code ( "You have $count unread messages." ). If you change the initial value of the count variable, the println() statement still works. Your code is more flexible now!
To further emphasize this point, compare the following two programs. The first program uses a string literal, with the exact number of unread messages directly in the string. This program only works when your user has 10 unread messages.
By using a variable and a string template in the second program, your code can adapt to more scenarios. This second program is more flexible!
Type inference
Here's a tip that allows you to write less code when declaring variables.
Type inference is when the Kotlin compiler can infer (or determine) what data type a variable should be, without the type being explicitly written in the code. That means you can omit the data type in a variable declaration, if you provide an initial value for the variable. The Kotlin compiler looks at the data type of the initial value, and assumes that you want the variable to hold data of that type.
The following syntax is for a variable declaration that uses type inference:

Returning to the count example, the program initially contained this line of code:
However, this line of code can also be written as follows. Notice that the colon symbol ( : ) and Int data type are omitted. The updated syntax has fewer words to type, and it achieves the same outcome of creating an Int variable called count with a value of 2 .
The Kotlin compiler knows that you want to store 2 (a whole number integer) into the variable count , so it can infer that the count variable is of type Int . Convenient, right? This is one example of how writing Kotlin code is more concise!
Even though this example only discusses a variable of type Int , the concept of type inference applies to all data types in Kotlin.
Basic math operations with integers
What is the difference between an Int variable with value 2 and a String variable with value "2" ? When they both get printed to the output, they look the same.
The advantage to storing integers as an Int (as opposed to a String ) is that you can perform math operations with Int variables, such as addition, subtraction, division, and multiplication (see other operators ). For example, two integer variables can be added together to get their sum. There are certainly cases where it's reasonable to have integers stored as Strings, but the purpose of this section is to show you what you can do with Int variables.
- Return to the Kotlin Playground and remove all the code in the code editor.
- Create a new program where you define an integer variable for the number of unread emails in an inbox, and initialize it to a value such as 5 . You can pick a different number if you'd like. Define a second integer variable for the number of read emails in an inbox. Initialize it to a value such as 100 . You can pick a different number if you'd like. Then print out the total number of messages in the inbox by adding the two integer numbers together.
- Run the program and it should display the total number of messages in the inbox:
For a string template, you learned that you can put the $ symbol before a single variable name. However, if you have a more complex expression, you must enclose the expression in curly braces with the $ symbol before the curly braces: ${unreadCount + readCount} . The expression within the curly braces, unreadCount + readCount , evaluates to 105 . Then the value 105 is substituted within the string literal.

- To explore this topic further, create variables with different names and different initial values, and use template expressions to print messages to the output.
For example, modify your program to print this out:
Here's one way that you can write your program, though there are other correct ways to write it too!
4. Update variables
When an app is running, the value of a variable may need to be updated. For example, in a shopping app, as the user adds items to the shopping cart, the cart total increases.
Let's simplify the shopping use case into a simple program. The logic is written out below with human language, not in Kotlin. This is called pseudocode because it describes the key points of how the code will be written, but it doesn't contain all the details of the code.
In the main function of a program:
- Create an integer cartTotal variable that starts at the value 0 .
- The user adds a sweater that costs $20 to their shopping cart.
- Update the cartTotal variable to 20 , which is the current cost of the items in their shopping cart.
- Print the total cost of the items in their cart, which is the cartTotal variable, to the output.
To further simplify the code, you don't need to write the code for when the user adds items to the shopping cart. (You haven't learned about how a program can respond to user input yet. That will come in a later unit.) Hence, focus on the parts where you create, update, and print out the cartTotal variable.
- Replace the existing code in the Kotlin Playground with the below program. In line 2 of the program, you initialize the cartTotal variable to the value 0 . Since you provide an initial value, there's no need to specify the Int data type due to type inference. In line 3 of the program, you attempt to update the cartTotal variable to 20 with the assignment operator ( = ). In line 4 of the program, you print out the cartTotal variable using a string template.
- Run the program, and you will get a compile error.
- Notice the error says that the val can't be reassigned. The error is on the third line of the program, which tries to change the value of the cartTotal variable to 20. The val cartTotal can't be reassigned to another value ( 20 ) after it's been assigned an initial value ( 0 ).
If you need to update the value of a variable, declare the variable with the Kotlin keyword var , instead of val .
- val keyword - Use when you expect the variable value will not change.
- var keyword - Use when you expect the variable value can change.
With val , the variable is read-only , which means you can only read, or access, the value of the variable. Once the value is set, you cannot edit or modify its value. With var , the variable is mutable , which means the value can be changed or modified. The value can be mutated.
To remember the difference, think of val as a fixed value and var as variable . In Kotlin, it's recommended to use the val keyword over the var keyword when possible.
- Update the variable declaration for cartTotal on line 2 of the program to use var instead of val . This is how the code should look:
- Notice the syntax of the code on line 3 of the program which updates the variable.
Use the assignment operator ( = ) to assign a new value ( 20 ) to the existing variable ( cartTotal ). You don't need to use the var keyword again because the variable is already defined.
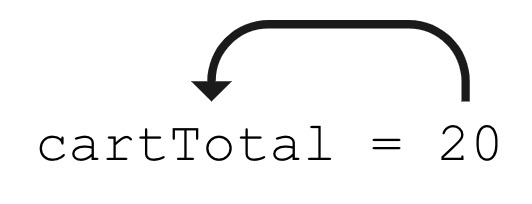
Using the box analogy, picture the value 20 being stored in the box labeled cartTotal .
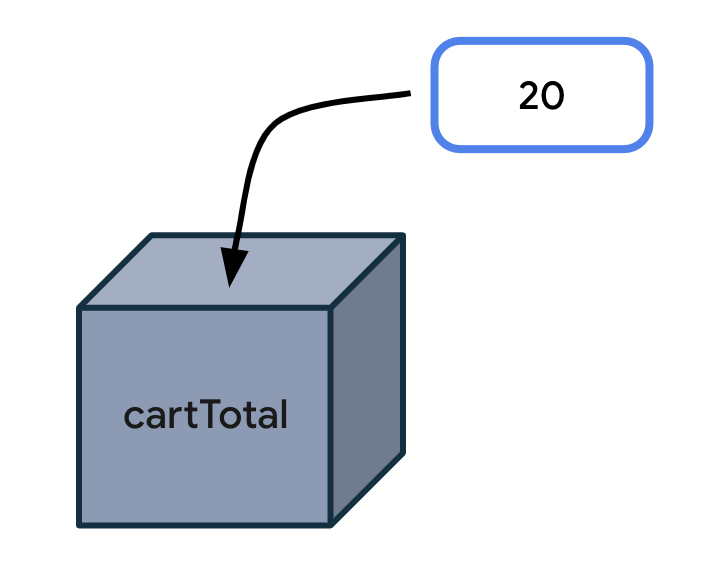
Here's a diagram for the general syntax for updating a variable, which has already been declared on an earlier line of code. Start the statement with the name of the variable you want to update. Add a space, the equal sign, followed by another space. Then write out the updated value for the variable.
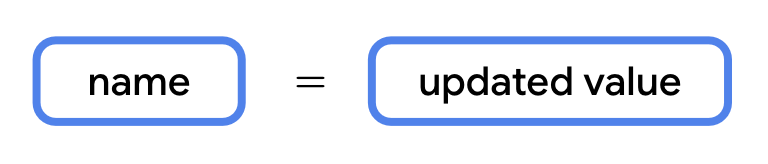
- Run your program and the code should successfully compile. It should print this output :
- To see how the variable value changes while the program is running, print the cartTotal variable to the output after the variable is initially declared. See the code changes below. Line 3 has a new println() statement. There's also a blank line added on line 4 of the code. Blank lines do not have any impact on how the compiler understands the code. Add a blank line where it would make it easier to read your code by separating out related blocks of code.
- Run the program again and the output should be:
You can see that initially the shopping cart total is 0 . Then it updates to 20 . You successfully updated a variable! This was possible because you changed cartTotal from a read-only variable (with val ) to a mutable variable (with var ).
Remember that you should only use var to declare a variable if you expect the value to change. Otherwise you should default to using val to declare a variable. This practice makes your code safer. Using val ensures that variables won't get updated in your program if you don't expect them to. Once a val is assigned a value, it always stays that value.
Increment and decrement operators
Now you know that a variable must be declared as a var in order to update its value. Apply this knowledge to the email message example below that should look familiar.
- Replace the code in the Kotlin Playground with this program:
- Run the program. It should print out:
- Replace the val keyword with the var keyword to make the count variable a mutable variable. There should be no change in the output when you run the program.
- However, now you can update the count to a different value. For example, when one new email arrives in the user's inbox, you can increase count by 1. (You don't need to write the code for the arrival of an email. Getting data from the internet is a more advanced topic for a much later unit.) For now, focus on the count variable increasing by 1 with this line of code:
The expression to the right of the equal sign is count + 1 and evaluates to 11 . That is because the current value of count is 10 (which is on line 2 of the program) and 10 + 1 equals 11 . Then with the assignment operator, the value 11 gets assigned or stored in the count variable.
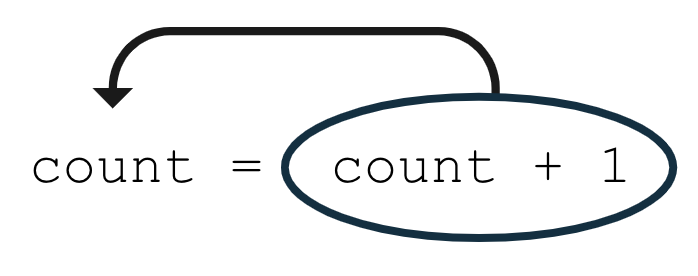
Add this line of code to your program at the bottom of the main() function. Your code should look like the following:
If you run the program now, the output is the same as before because you haven't added any code to use the count variable after you updated it.
- Add another print statement that prints out the number of unread messages after the variable has been updated.
- Run the program. The second message should display the updated count of 11 messages.
- For shorthand, if you want to increase a variable by 1 , you can use the increment operator ( ++ ) which is made up of two plus symbols. By using these symbols directly after a variable name, you tell the compiler that you want to add 1 to the current value of the variable, and then store the new value in the variable. The following two lines of code are equivalent, but using the ++ increment operator involves less typing.
Make this modification to your code and then run your program. There should be no spaces between the variable name and the increment operator.
- Run the program. The output is the same, but now you learned about a new operator!
- Now modify line 4 of your program to use the decrement operator ( -- ) after the count variable name. The decrement operator is made up of two minus symbols. By placing the decrement operator after the variable name, you tell the compiler that you want to decrease the value of the variable by 1 and store the new value into the variable.
- Run the program. It should print this output:
In this section, you learned how to update a mutable variable using the increment operator ( ++ ) and the decrement operator ( -- ). More specifically, count++ is the same as count = count + 1 and count-- is the same as count = count - 1 .
5. Explore other data types
Earlier in the codelab, you were introduced to some common basic data types: String , Int , Double , and Boolean . You just used the Int data type, now you will explore other data types.
Try these programs in the Kotlin Playground to see what the output is.
When you need a variable with a decimal value, use a Double variable. To learn about its valid range, refer to this table and look at the decimal digits it can store for example.
Imagine you're navigating to a destination, and your trip is split into three separate parts because you need to make stops along the way. This program displays the total distance left to reach your destination.
- Enter this code in the Kotlin Playground. Can you understand what is happening on each line of code?
Three variables called trip1 , trip2 , and trip3 are declared to represent the distance of each part of the trip. All of them are Double variables because they store decimal values. Use val to declare each variable because their values do not change over the course of the program. The program also creates a fourth variable called totalTripLength which is currently initialized to 0.0 . The last line of the program prints a message with the value of the totalTripLength variable.
- Fix the code so that the totalTripLength variable is the sum of all three trip lengths.
The expression on the right of the equal sign evaluates to 9.02 because 3.20 + 4.10 + 1.72 equals 9.02 . The value of 9.02 gets stored into the totalTripLength variable.

Your whole program should look like the below code:
- Run the program. It should print this out:
- Update your code to remove the unnecessary Double data type from the variable declarations because of type inference. The Kotlin compiler can infer that these variables are Double data types based on the decimal numbers provided as initial values.
- Run your code again to ensure that your code still compiles. The output should be the same, but now your code is simpler!
When you need a variable that can store text, use a String variable. Remember to use quotation marks around String literal values, such as "Hello Kotlin" , whereas the Int and Double literal values do not have quotes around them.
- Copy and paste this program into the Kotlin Playground.
Notice that there are two String variables declared, a nextMeeting variable and a date variable. Then a third String variable called reminder is declared, which is set equal to the nextMeeting variable plus the date variable.
With the + symbol, you can add two strings together, which is called concatenation . The two strings are combined together, one after the other. The result of the expression, nextMeeting + date is "Next meeting:January 1" as shown in the diagram below.

The value "Next meeting:January 1" is then stored into the reminder variable using the assignment operator on line 4 of the program.
- Run your program. It should print this out:
When you concatenate two strings together, there are no extra spaces added between the strings. If you want a space after the colon in the resulting string, you need to add the space to one string or the other.
- Update your nextMeeting variable to have an extra space at the end of the string before the closing quotation mark. (Alternatively, you could have added an extra space to the beginning of the date variable). Your program should look like the following:
- Run your program again and now there should be a space after the colon in the output message.
- Modify the code so that you concatenate - or add - another piece of text to the expression that gets stored in the reminder variable.
Use the + symbol to add the string literal " at work" to the end of the reminder string.
- Run the program.
It should print this output:
The code below shows you one way that you could implement the behavior.
Notice that there are no quotation marks around nextMeeting and date because they are names of existing string variables (where their respective values are text with quotes around them). Conversely, the literal " at work" is not previously defined in any variable, so use quotations around this text in order for the compiler to know that this is a string that should be concatenated onto the other strings.
Technically, you can achieve the same output by declaring a single String variable with the full text instead of using separate variables. However, the purpose of this exercise is to demonstrate how you can declare and manipulate String variables, especially how to concatenate separate strings.
- When reading code that contains strings, you may come across escape sequences . Escape sequences are characters that are preceded with a backslash symbol ( \ ), which is also called an escaping backslash.
An example is seeing \" within a string literal like in the below example. Copy and paste this code into the Kotlin Playground.
You learned earlier to use double quotation marks around a string literal. But what if you want to use the " symbol in your string? Then you need to add the backslash symbol before the double quotation mark as \" within your string. Remember that there should still be double quotation marks around the whole string.
- Run the program to see the output. It should show:
In the output, there are quotation marks displayed around hello because we added \" before and after hello in the println() statement.
For other escape sequences supported in Kotlin, refer to the documentation page on escape sequences . For example, if you want a new line in your string, use the \ symbol before the character n as in \n .
Now you've learned about concatenating strings and also escape sequences within strings. Move onto the last data type that this codelab covers.
The Boolean data type is useful when your variable only has two possible values represented by true or false .
An example is a variable that represents whether a device's airplane mode is on or off, or whether an app's notifications are enabled or disabled.
- Enter this code in the Kotlin Playground. In line 2 of this program, you declare a Boolean variable called notificationsEnabled and initialize it to true . Technically, you can omit : Boolean in the declaration, so you can remove it if you'd like. In line 3 of the program, you print out the value of the notificationsEnabled variable.
Run the program, and it should print this out:
- Change the initial value of the Boolean to false on line 2 of the program.
- Other data types can be concatenated to Strings . For example, you can concatenate Booleans to Strings . Use the + symbol to concatenate (or append) the value of the notificationsEnabled boolean variable onto the end of the "Are notifications enabled? " string.
Run the program to see the result of the concatenation. The program should print this output:
You can see that it's possible to set the Boolean variable to a true or false value. Boolean variables enable you to code more interesting scenarios in which you execute some set of instructions when a Boolean variable has a true value. Or if the Boolean has a false value, you skip those instructions. You learn more about Booleans in a future codelab.
6. Coding conventions
In the previous codelab, you were introduced to the Kotlin style guide for writing Android code in a consistent way as recommended by Google and followed by other professional developers.
Here are a couple of other formatting and coding conventions for you to follow based on the new topics you learned:
- Variable names should be in camel case and start with a lowercase letter.
- In a variable declaration, there should be a space after a colon when you specify the data type.
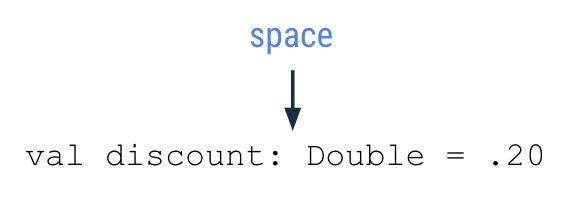
- There should be a space before and after an operator like the assignment ( = ), addition ( + ), subtraction ( - ), multiplication ( * ), division ( / ) operators and more.
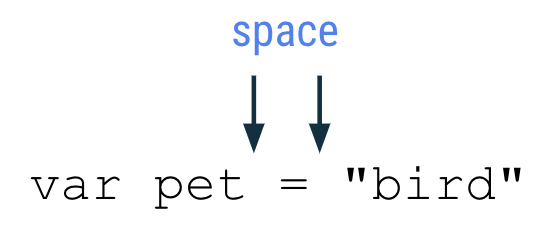
- As you write more complex programs, there is a recommended limit of 100 characters per line . That ensures that you can read all the code in a program easily on your computer screen, without needing to scroll horizontally when reading code.
7. Commenting in your code
When coding, another good practice to follow is to add comments that describe what the code is intended to do. Comments can help people who read your code follow it more easily. Two forward slash symbols, or // , indicate that the text after it on the rest of the line is considered a comment, so it isn't interpreted as code. It's common practice to add a space after the two forward slash symbols.
A comment can also start in the middle of a line of code. In this example, height = 1 is a normal coding statement. // Assume the height is 1 to start with is interpreted as a comment and not considered part of the code.
If you want to describe the code in more detail with a long comment that exceeds 100 characters on a line, use a multi-line comment. Start the multi-line comment with a forward slash ( / ) and an asterisk symbol ( * ) as /* . Add an asterisk at the beginning of each new line of the comment. Then finally end the comment with an asterisk and forward slash symbol */ .
This program contains single-line and multi-line comments that describe what's happening:
As mentioned earlier, you can add blank empty lines to your code to group related statements together and make the code easier to read.
- Add some comments to an earlier code snippet that you used.
- Run the program to ensure that the behavior didn't change because comments shouldn't affect the output.
8. Conclusion
Excellent work! You learned about variables in Kotlin, why variables are useful in programming, and how to create, update, and use them. You experimented with different basic data types in Kotlin, including the Int , Double , String , and Boolean data types. You also learned about the difference between the val and var keywords.
All of these concepts are critical building blocks on your journey to become a developer.
See you in the next codelab!
- A variable is a container for a single piece of data.
- You must declare a variable first before you use it.
- Use the val keyword to define a variable that is read-only where the value cannot change once it's been assigned.
- Use the var keyword to define a variable that is mutable or changeable.
- In Kotlin, it's preferred to use val over var when possible.
- To declare a variable, start with the val or var keyword. Then specify the variable name, data type, and initial value. For example: val count: Int = 2 .
- With type inference, omit the data type in the variable declaration if an initial value is provided.
- Some common basic Kotlin data types include: Int , String , Boolean , Float , and Double .
- Use the assignment operator ( = ) to assign a value to a variable either during declaration of the variable or updating the variable.
- You can only update a variable that has been declared as a mutable variable (with var ).
- Use the increment operator ( ++ ) or decrement operator ( -- ) to increase or decrease the value of an integer variable by 1, respectively.
- Use the + symbol to concatenate strings together. You can also concatenate variables of other data types like Int and Boolean to Strings .
- Basic types
- String templates
- Keywords and operators
- Basic syntax
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Kotlin Tutorial
Kotlin classes, kotlin examples, kotlin variables.
Variables are containers for storing data values.
To create a variable, use var or val , and assign a value to it with the equal sign ( = ):
The difference between var and val is that variables declared with the var keyword can be changed/modified , while val variables cannot .
Variable Type
Unlike many other programming languages, variables in Kotlin do not need to be declared with a specified type (like "String" for text or "Int" for numbers, if you are familiar with those).
To create a variable in Kotlin that should store text and another that should store a number, look at the following example:
Kotlin is smart enough to understand that "John" is a String (text), and that 1975 is an Int (number) variable.
However, it is possible to specify the type if you insist:
You can also declare a variable without assigning the value, and assign the value later. However , this is only possible when you specify the type:
This works fine:
This will generate an error:
Note: You will learn more about Data Types in the next chapter .
Advertisement
Notes on val
When you create a variable with the val keyword, the value cannot be changed/reassigned.
The following example will generate an error:
When using var , you can change the value whenever you want:
So When To Use val ?
The val keyword is useful when you want a variable to always store the same value, like PI (3.14159...):
Tip: Keep in mind that it is much safer to create variables that can't be changed, as they can't be overwritten by other programs or people.
Display Variables
Like you have seen with the examples above, the println() method is often used to display variables.
To combine both text and a variable, use the + character:
You can also use the + character to add a variable to another variable:
For numeric values, the + character works as a mathematical operator:
From the example above, you can expect:
- x stores the value 5
- y stores the value 6
- Then we use the println() method to display the value of x + y, which is 11
Variable Names
A variable can have a short name (like x and y) or more descriptive names (age, sum, totalVolume).
The general rule for Kotlin variables are:
- Names can contain letters, digits, underscores, and dollar signs
- Names should start with a letter
- Names can also begin with $ and _ (but we will not use it in this tutorial)
- Names are case sensitive ("myVar" and "myvar" are different variables)
- Names should start with a lowercase letter and it cannot contain whitespace
- Reserved words (like Kotlin keywords, such as var or String ) cannot be used as names
camelCase variables
You might notice that we used firstName and lastName as variable names in the example above, instead of firstname and lastname. This is called "camelCase", and it is considered as good practice as it makes it easier to read when you have a variable name with different words in it, for example "myFavoriteFood", "rateActionMovies" etc.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, kotlin introduction.
- Kotlin Hello World - Your First Kotlin Program
- Kotlin Comments
Kotlin Fundamentals
Kotlin variables and basic types.
Kotlin Type Conversion
- Kotlin Operators: Arithmetic, Assignment Operator and More
Kotlin Expression, Statements and Blocks
- Kotlin Basic Input/Output
Kotlin Flow Control
- Kotlin if...else Expression
- Kotlin when Expression
- Kotlin while and do...while Loop
Kotlin for Loop
- Kotlin break
- Kotlin continue
Kotlin Functions
- Kotlin Function (With Example)
- Kotlin Function Call Using Infix Notation
- Kotlin Default and Named Arguments
- Kotlin Recursion and Tail Recursive Function
- Kotlin Class and Objects
- Kotlin Constructors and Initializers
- Kotlin Getters and Setters (With Example)
- Kotlin Inheritance
- Kotlin Visibility Modifiers
- Kotlin Abstract Class and Abstract Members
- Kotlin Interfaces
- Kotlin Nested and Inner Class
- Kotlin Data Class
- Kotlin Sealed Classes
- Kotlin Object Declarations and Expressions
- Kotlin Companion Objects
- Kotlin Extension Function
- Kotlin Operator Overloading
Additional Topics
Kotlin Keywords and Identifiers
- Kotlin String and String Templates
- Kotlin Lambdas
- Kotlin Bitwise and Bitshift Operations
Kotlin Tutorials
As you know, a variable is a location in memory (storage area) to hold data.
To indicate the storage area, each variable should be given a unique name (identifier). Learn more about How to name a variable in Kotlin?
- How to declare a variable in Kotlin?
To declare a variable in Kotlin, either var or val keyword is used. Here is an example:
The difference in using var and val is discussed later in the article. For now, let's focus on variable declaration.
Here, language is a variable of type String , and score is a variable of type Int . You don't have to specify the type of variables; Kotlin implicitly does that for you. The compiler knows this by initializer expression ( "French" is a String , and 95 is an integer value in the above program). This is called type inference in programming.
However, you can explicitly specify the type if you want to:
We have initialized variable during declaration in above examples. However, it's not necessary. You can declare variable and specify its type in one statement, and initialize the variable in another statement later in the program.
Here are few examples that results into error.
Here, the type of language variable is not explicitly specified, nor the variable is initialized during declaration.
Here, we are trying to assign 14 (integer value) to variable of different type ( String ).
- Difference Between var and val
- val (Immutable reference) - The variable declared using val keyword cannot be changed once the value is assigned. It is similar to final variable in Java .
- var (Mutable reference) - The variable declared using var keyword can be changed later in the program. It corresponds to regular Java variable.
Here are few examples:
Here, language variable is reassigned to German . Since, the variable is declared using var , this code work perfectly.
You cannot reassign language variable to German in the above example because the variable is declared using val .
Now, you know what Kotlin variables are, it's time to learn different values a Kotlin variable can take.
Kotlin Basic Types
Kotlin is a statically typed language like Java. That is, the type of a variable is known during the compile time. For example,
Here, the compiler knows that language is of type Int , and marks is of type Double before the compile time.
The built-in types in Kotlin can be categorized as:
Number Type
Numbers in Kotlin are similar to Java. There are 6 built-in types representing numbers.
- The Byte data type can have values from -128 to 127 (8-bit signed two's complement integer).
- It is used instead of Int or other integer data types to save memory if it's certain that the value of a variable will be within [-128, 127]
- Example: fun main(args : Array<String>) { val range: Byte = 112 println("$range") // The code below gives error. Why? // val range1: Byte = 200 }
When you run the program, the output will be:
- The Short data type can have values from -32768 to 32767 (16-bit signed two's complement integer).
- It is used instead of other integer data types to save memory if it's certain that the value of the variable will be within [-32768, 32767].
- The Int data type can have values from -2 31 to 2 31 -1 (32-bit signed two's complement integer).
If you assign an integer between -2 31 to 2 31 -1 to a variable without explicitly specifying its type, the variable will be of Int type. For example,
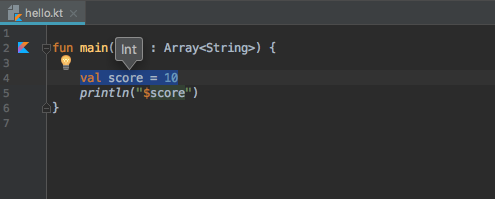
- The Long data type can have values from -2 63 to 2 63 -1 (64-bit signed two's complement integer).
If you assign an integer value greater than 2 31 -1 or less than -2 31 to a variable (without explicitly specifying its type), the variable will be of Long type. For example,
Similarly, you can use capital letter L to specify that the variable is of type Long . For example,
- The Double type is a double-precision 64-bit floating point.
- The Float data type is a single-precision 32-bit floating point. Learn more about single precision and double precision floating point if you are interested.
Notice that, we have used 19.5F instead of 19.5 in the above program. It is because 19.5 is a Double literal, and you cannot assign Double value to a variable of type Float .
To tell compiler to treat 19.5 as Float , you need to use F at the end.
If you are not sure what number value a variable will be assigned in the program, you can specify it as Number type. This allows you to assign both integer and floating-point value to the variable (one at a time). For example:
To learn more, visit: Kotlin smart casts
To represent a character in Kotlin, Char types are used.
Unlike Java, Char types cannot be treated as numbers. Visit this page to learn more about Java char Type .
In Java, you can do something like:
However, the following code gives error in Kotlin.
- The Boolean data type has two possible values, either true or false .
Booleans are used in decision making statements (will be discussed in later chapter).
Kotlin Arrays
An array is a container that holds data (values) of one single type. For example, you can create an array that can hold 100 values of Int type.
In Kotlin, arrays are represented by the Array class. The class has get and set functions, size property, and a few other useful member functions.
To learn in detail about arrays, visit: Kotlin Arrays
Kotlin Strings
In Kotlin, strings are represented by the String class. The string literals such as "this is a string" is implemented as an instance of this class.
To learn in detail about strings, vist: Kotlin Strings
Recommended Readings
- Type conversion in Kotlin
- Smart casts in Kotlin
- Kotlin nullable types

Table of Contents
- Kotlin Basic Types
Sorry about that.
Related Tutorials
Kotlin Tutorial
Kotlin Android
Kotlin basics.
- Kotlin Hello World
- Kotlin Ranges
- Kotlin Arrays
- Kotlin Programs
- LinearLayout
Android Compose
- Kotlin Tutorials
- Kotlin Android Tutorials
- Android Compose Tutorials
- Kotlin Android Home
- → Kotlin Tutorials
- →→ Kotlin Variables
- Introduction to Kotlin language
Kotlin Variables
- Kotlin Comments
- Kotlin if-else
- Kotlin While loop
- Kotlin Do..While loop
- Kotlin For loop
- Kotlin break
- Kotlin continue
- Kotlin Arrays Tutorials
- Kotlin String Tutorials
In Kotlin, we can define a variable using var keyword.
- var is the keyword
- x is the variable name
- = is the assignment operator
- "apple" is the value
If we do not initialise the variable, we have to specify the data type, as shown in the following.
Once a variable is defined, or initialled, we can reassign another value of the same datatype.
If we do not want the value to be changed, then we can declare the variable using val keyword. val keyword makes the variable immutable.
Once a value is assigned, we cannot reassign a new value to the variable.
Introduction to Variables, Types, and Literals
Understand variables by exploring concepts such as declaration, initialization, types, and literals.
Variable declaration
Implicit variable type, explicit variable type, variable initialization, basic types, their literals and operations.
To declare a variable in Kotlin, we start with the val or var keyword, then a variable name, the equality sign, and an initial value.
The keyword var (which stands for variable) represents read-write variables and is used to define variables whose values can be reassigned after initialization. This means that if we use var , we can always assign a new value to this variable.
The keyword val (which stands for value) represents read-only variables and is used to define values that cannot be reassigned. This means that if we use val , we cannot assign a new value to this variable once it is initialized.
Variable naming conventions
We can name variables using characters, underscore ( _ ), and numbers, but numbers are not allowed at the first position. By convention, we name variables with the camelCase convention; this means the variable name starts with a lowercase letter, and then (instead of using spaces) each next word starts with a capital letter.
What kind of Experience do you want to share?

You’ve made it this far. Let’s build your first application
DhiWise is free to get started with.

Design to code
- Figma plugin
- Screen Library
- Documentation
- DhiWise University
- DhiWise vs Anima
- DhiWise vs Appsmith
- DhiWise vs FlutterFlow
- DhiWise vs Monday Hero
- DhiWise vs Retool
- DhiWise vs Supernova
- DhiWise vs Amplication
- DhiWise vs Bubble
- DhiWise vs Figma Dev Mode
- Terms of Service
- Privacy Policy
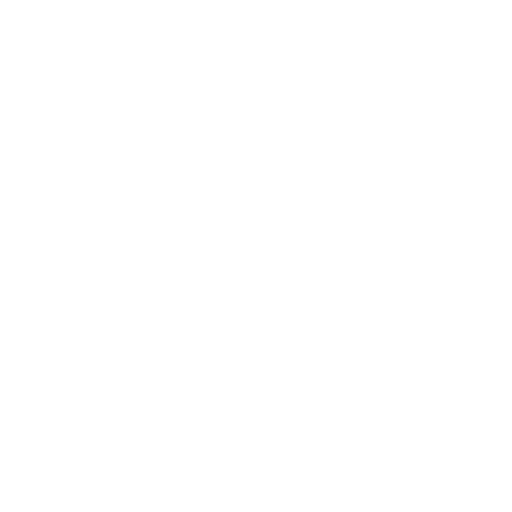
Kotlin Control Flow Explained: Crafting Elegant Logic in Your Code
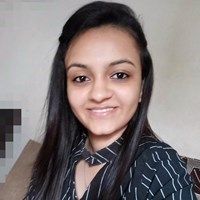
Nidhi Sorathiya
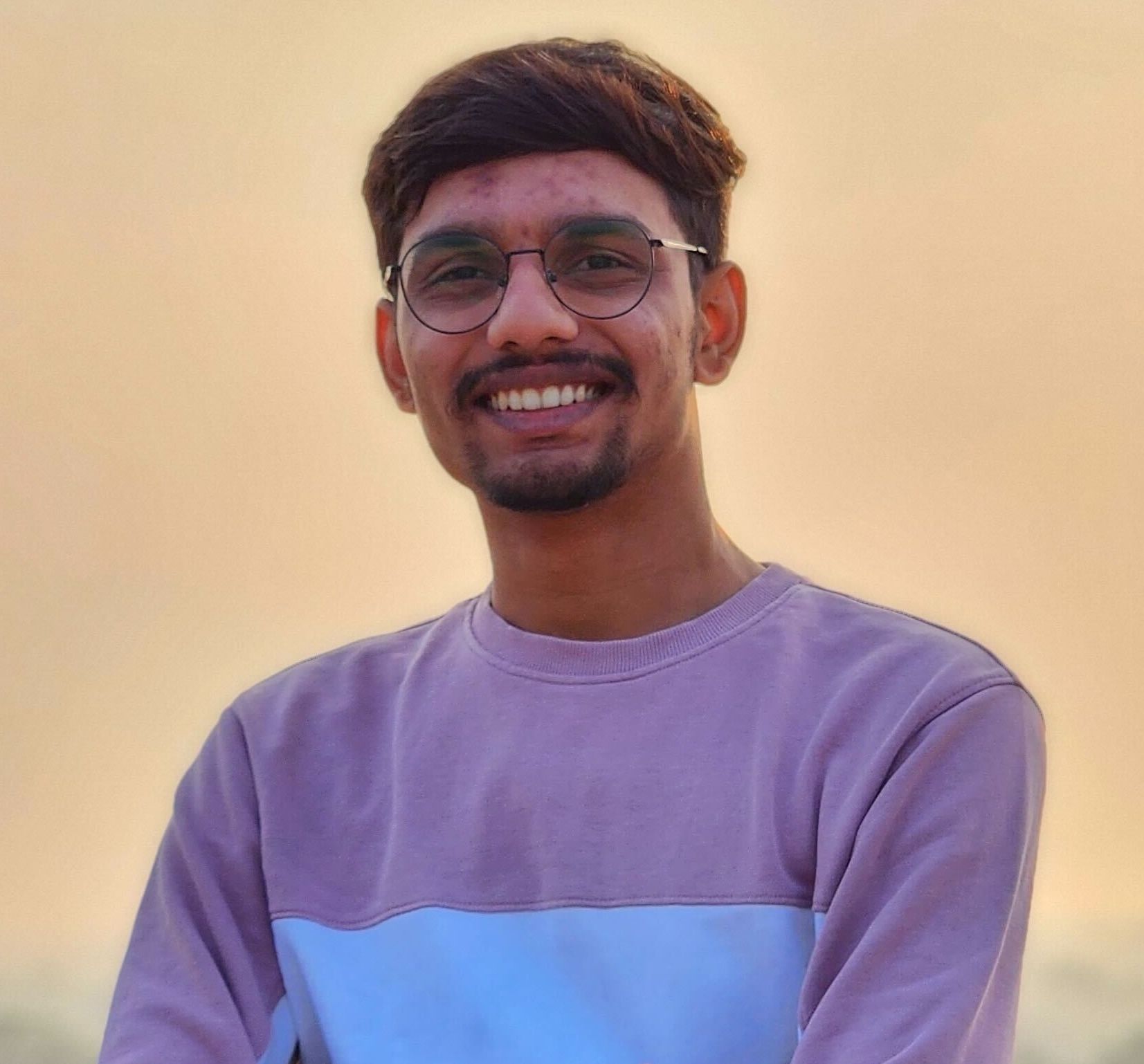
Keyur Kanjiya
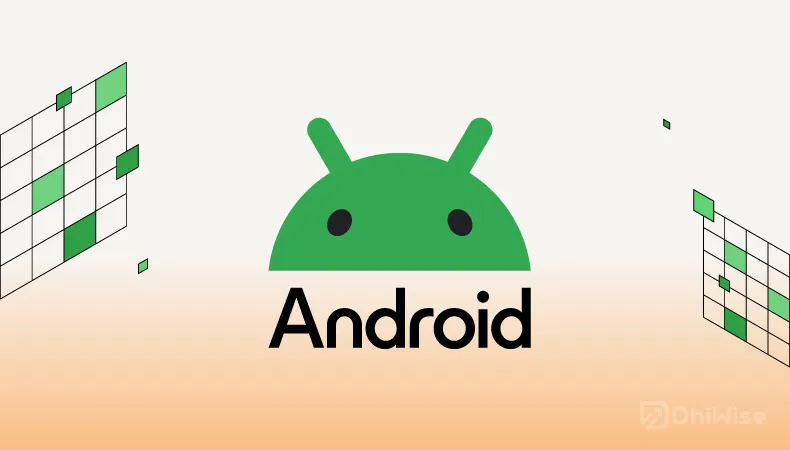
Frequently asked questions
What is control flow in kotlin, is there an else if in kotlin, what is the alternative to switch case in kotlin, does kotlin have a switch or when, how does the try {} catch {} finally {} work in kotlin.
In any programming language, including Kotlin, controlling the flow of execution is fundamental to building functional programs. Effective use of Kotlin control flow mechanisms allows a program to react differently under various conditions, making decisions, and repeating execution of code blocks. Kotlin simplifies control flow with clear syntax and powerful constructs, continuing its reputation for concise and expressive coding norms established by languages like Java.
Consider this simple example: the decision-making process in a program may need to utilize if statements, enabling the program to execute a certain block of code among multiple alternatives based on the provided conditions. Kotlin’s control flow tools, such as if...else structures, looping constructs like the Kotlin for loop , and Kotlin while loop, as well as the Kotlin try-catch mechanism for exception handling, make these operations straightforward and readable.
Let’s embark on a journey to explore Kotlin's control flow constructs and learn how to use them effectively in our code.
Kotlin If...Else Statements
Kotlin's decision-making prowess begins with the Kotlin if else statement. Implementing clear decision paths within a program, Kotlin enables you to conditionally execute blocks of code. An else-if Kotlin construct may follow the initial if statement to check multiple conditions sequentially, providing alternative execution paths.
Here's an example of a Kotlin conditional assignment:
In the above program, we evaluate whether the integer variable number is even or odd. The last expression of the if...else construct provides the value for the conditional assignment, a feature that sets Kotlin apart from many other programming languages.
Exploring Loops in Kotlin
Iteration in Kotlin enables programs to execute a block of code repeatedly. Kotlin provides several looping constructs, each with its unique application.
The Kotlin For Loop
The Kotlin for loop is versatile, perfect for iterating through ranges, arrays, or collections. The syntax uses a range of values followed by the keyword and encapsulates the execution block within curly braces. Here's an example showcasing the kotlin for loop:
Kotlin For Each
For more idiomatic iteration over collections, Kotlin offers the Kotlin for each construct, allowing concise and direct traversals. This improves readability and reduces the chance of errors commonly associated with traditional indexed loops.
Consider this kotlin for each example as we iterate over a list:
Kotlin While Loop
When the number of iterations is not known beforehand, the kotlin while loop comes into play. The condition is evaluated before each execution of the loop's body:
Do While Loop in Kotlin
The do-while loop in kotlin guarantees that the block inside will execute at least once. The condition check happens after the block executes:
In the next section, we'll explore Kotlin's approach to switching between multiple cases, emphasizing its use of the when expression as a versatile substitute for the traditional switch case statement found in C-like languages.
Branching with Kotlin Switch Statement
In traditional programming languages, the switch case statement offers a way to execute different parts of code based on the value of an expression. However, Kotlin takes it a step further with its when expression, which serves as a powerful replacement for the switch case approach. The when expression simplifies complex branching paths and can handle more than just constant values.
Traditional Switching Mechanism
Here’s how you might use a switch case in Kotlin, which is syntactically similar to Java:
In the above program, when the expression evaluates day compares it to multiple cases and executes the block associated with the matching case. If none of the cases match, the other branch is executed.
Enhanced Switching
The Kotlin switch case can also be used to match ranges or even types. Moreover, it can be used with arbitrary conditions, making it much more versatile:
The above code exemplifies the flexibility of Kotlin's when expression, efficiently replacing multiple if...else-if statements that would be used in other programming languages.
Following up, we'll navigate our way through Kotlin's error handling paradigm, examining the kotlin try-catch construct, and understanding how exception handling is a critical part of robust Kotlin control flow.
Exception Handling in Kotlin
In Kotlin, exception handling is a structured process that ensures your program can account for, and react to, unexpected events during execution. This is where the kotlin try-catch block, alongside the finally block, comes into play. Exception handling in Kotlin follows the try-catch-finally pattern common in Java and other programming languages.
Basic Try-Catch Blocks
A try block encloses code that might throw an exception. If an exception occurs, control is transferred to the catch block that can handle it. Here's an example:
In the example, dividing by zero throws an ArithmeticException which is caught in the catch block. The catch can handle multiple exception classes if needed.
Catching Multiple Exceptions
You can handle different exceptions separately by following the main catch with either catch block:
In this example, ArrayIndexOutOfBoundsException is specifically caught in the first catch block, while all other exceptions fall to the second catch block.
The Finally Block
The finally block encloses code that will execute whether an exception is thrown or not, making it the perfect place for resource management or cleanup tasks:
In the above code, attempting to calculate the length of a null string results in a Kotlin NullPointerException, which is caught and handled in the catch block. Regardless of the exception, the finally block is executed, showcasing an important statement about the robustness of the Kotlin control flow with exception handling.
Kotlin Conditional Assignment Techniques
Kotlin promotes elegant and expressive code, and this includes how values are conditionally assigned to variables. Instead of the verbose syntax found in Java and other programming languages, Kotlin uses the power of expressions for assignments, often resulting in one-liners that are both readable and concise.
Consider an example where you need to assign a value to a variable based on a condition:
In the example, we assign the category based on the age using a when expression. The overall expression evaluates and assigns the result directly to the category variable without the need for additional if...else blocks.
Kotlin also allows conditional assignment within expressions using the if statement:
The last expression in the block is the value returned and assigned to the result. It not only improves readability but simplifies the control flow by reducing the need for verbose code.
Conclusion: Kotlin Control Flow Best Practices
Embracing Kotlin control flow means writing more efficient and cleaner code. Throughout this exploration, we have seen kotlin if-else, the looping constructs kotlin for loop and kotlin while loop, and touched on the elegance of the kotlin try-catch block for exception handling.
Following best practices in programming, notably Kotlin’s approach to control flow can immensely improve the readability and maintainability of code. Utilize the if statement for simple conditional checks, leverage the power of the for and while loops for iteration, and always ensure robustness in your program with proper exception handling using try-catch kotlin constructs.
Conditional assignment with if and when expressions exemplify Kotlin’s emphasis on expressiveness and conciseness. They allow developers to write code that conveys the flow of execution, which is essential in a well-designed program.
Use this guide as a stepping stone in your Kotlin journey, and continue practicing with real-world examples to deepen your understanding and finesize your control flow mastery.
Assignment to variable inside when block
Say, I have multiple functions, each returns a sealed class and I want to chain-check the results using when block:
Now, I know I can assign the result of a function to a variable and then use it in when block, like so:
But the above, first of all, is not very nice looking - I don’t use the variables anywhere else but inside when.
What’s more important, is that I know that execution of when’s branches is lazy, so if one matches, the other ones are not executed. Meaning, if I would match on getResult() in my first example, the getDifferentResult() would not be called, which is excellent. Sadly, I can’t achieve the same using the second example. I probably could do something like val output01: Handled by lazy {} but that’s even less readable.
Is there some other way to achieve what I want? In my mind, being able to assign the result to a variable inside the block would solve the problem, similarly to how it’s possible when matching cases in Swift:
I think you would better add one more layer of abstraction and move the when block to a function that you’d call for each result:
This prints:
But yours does something totally different from what I was trying to achieve. Your code runs all the functions and prints all their outputs. Mine would only print the one that matched, not even calling anything down the line from the matched branch.
I used a very simplified example, in reality, each function could be doing something expensive, so I would want to find the first function output that matches my condition, do something with the result of that match and not even call the other functions
Something like this:
In the above getUnhandled() would never be called, as the first branch matches the condition
Now I understand …
What do you think about this approach using a sequence of function references:
This prints only
This is very nice indeed!
I can see myself using something like in some of the situations, but definitely not all - as I tried to show in the code comments in my last message, the handling of the result can be totally different, depending on which branch has matched. In your example, the result is always handled the same (by printResult).
Oh, and, of course, the functions I call - they can use completely different arguments: getResult(someStringArg) , getDifferentResult(someIntArg)
Also, the sealed class can be much more complex. I have Yes No, but it can have many different states and completely different associated values.:
May it’s best to use the good, old if .
But I’m wondering if it could make sense to combine the action and the handler in an object. Something like this:
However it is just a glorified if at the moment, but maybe it could bring you to an idea …
Even with if/else in Swift it looks so much more elegant:
Ability to assign the result of matched case during the matching is something I really would love to see in Kotlin - especially in the when expressions.
There is feature request about when statement: https://youtrack.jetbrains.com/issue/KT-4895
A rather nasty “hack” would make this work:
Instead of the when you could also just use a shortcircuit or expression. The key is that the inline function provides the action. This inline function could even return from the function (using a helper function that way is probably the cleanest way to allow a return value from the chain).
Related Topics

IMAGES
VIDEO
COMMENTS
Kotlin supports type inference and automatically identifies the data type of a declared variable. When declaring a variable, you can omit the type after the variable name: fun main () { //sampleStart // Declares the variable x with the value of 5;`Int` type is inferred val x = 5 // 5 //sampleEnd println (x) } xxxxxxxxxx.
Once you define a variable, you can that variable in your program. To use a variable, type out the variable name in your code, which tells the Kotlin compiler that you want to use the variable's value at that point in the code. For example, define a variable for the number of unread messages in a user's inbox.
Kotlin Variables. Variables are containers for storing data values. To create a variable, use var or val, and assign a value to it with the equal sign (=): ... You can also declare a variable without assigning the value, and assign the value later. However, this is only possible when you specify the type:
In Kotlin, as in many other programming languages, loops are a fundamental control structure that allows for the repeated execution of a block of code. The while loop is particularly useful for executing code as long as a certain condition remains true. However, Kotlin differs from some languages in how it treats variable assignment within the condition of a while loop.
In Java, we can declare and assign multiple variables only in the same type at once, such as: // Java code String str1 = "Hello World!", str2 = "Hi there", str3 = "See you later"; Copy. As the example above shows, we've declared three String variables in one single line separated by commas.
Kotlin Assignment Operator assigns the right operand, usually a value or an expression, to the left operand, usually a variable or constant. In this tutorial, you will learn how to use Assignment operator, with syntax and examples. ... Then we assign a new value, say "Hello User" to the message variable using Assignment operator. Kotlin Program.
Kotlin Basic Types. Kotlin is a statically typed language like Java. That is, the type of a variable is known during the compile time. For example, val language: Int. val marks = 12.3. Here, the compiler knows that language is of type Int, and marks is of type Double before the compile time. The built-in types in Kotlin can be categorized as ...
Kotlin is a type inferred language i.e Kotlin compiler automatically infers the type of variable if we declare and assign the value of variable together. val a = 10. In the above code, Kotlin ...
Kotlin Variables. In Kotlin, we can define a variable using var keyword. var x = "apple". where. var is the keyword. x is the variable name. = is the assignment operator. "apple" is the value. If we do not initialise the variable, we have to specify the data type, as shown in the following.
Variable declaration. To declare a variable in Kotlin, we start with the val or var keyword, then a variable name, the equality sign, and an initial value.. The keyword var (which stands for variable) represents read-write variables and is used to define variables whose values can be reassigned after initialization. This means that if we use var, we can always assign a new value to this variable.
In Kotlin, as in many other languages, there is a distinction between an assignment and an expression. Let's break down the difference: Assignment: It's an operation that gives a value to a variable. In Kotlin, assignment uses the = operator. For instance: val x = 10 or var y: Int; y = 20. Assignments in Kotlin do not evaluate to a value.
In Kotlin, let () is a pretty convenient scope function. It allows us to transform the given variable to a value in another type. In this tutorial, we'll explore how to apply let -like operations on multiple variables. 2. Introduction to the Problem. First of all, let's look at a simple let example:
You can not access the variable outside the loop. Same variable can be declared inside the nested loop - so if a function contains an argument x and we declare a new variable x inside the same loop, then x inside the loop is different than the argument. Naming Convention - Every variable should be named using lowerCamelCase.
3. Kotlin If-Else Expression. An expression is a combination of one or more values, variables, operators, and functions that execute to produce another value. val number: Int = 25. val result: Int = 50 + number. Copy. Here, 50 + number is an expression that returns an integer value.
Kotlin Conditional Assignment Techniques. Kotlin promotes elegant and expressive code, and this includes how values are conditionally assigned to variables. Instead of the verbose syntax found in Java and other programming languages, Kotlin uses the power of expressions for assignments, often resulting in one-liners that are both readable and ...
Say, I have multiple functions, each returns a sealed class and I want to chain-check the results using when block: sealed class Handled { data class Yes(val result: Boolean) : Handled() object No : Handled() } fun getResult(): Handled { // some logic to get the result } fun getDifferentResult(): Handled { // some other logic but output is of Handled type } when { getResult() is Handled.Yes ...
Kotlin - Assign value to variable or break and return function. 0. Kotlin - How to return object based on condition. 0. refactor if to takeIf and return "return" without label kotlin. Hot Network Questions Is a dome really the most efficient way to contain gas in a vacuum?
1. If you pass a lambda as a parameter of a function it will be stored in a variable. The calling application might need to save that (e.g. event listener for later use). Therefore you need to be able to store it as a variable as well. As said in the answer however, you should do this only when needed!
It has nothing to do with the local variable named verticesCount declared just above, so the compiler thinks you've still to initialize that variable. In Kotlin, the assignment to a variable (a = b) is not an expression, so it cannot be used as a value in other expressions. You have to split the assignment and the while-loop condition to ...