TypeError: Assignment to Constant Variable in JavaScript
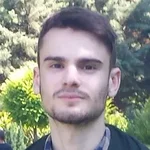
Last updated: Mar 2, 2024 Reading time · 3 min
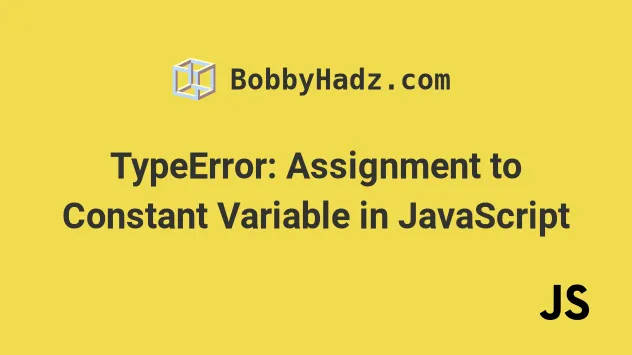

# TypeError: Assignment to Constant Variable in JavaScript
The "Assignment to constant variable" error occurs when trying to reassign or redeclare a variable declared using the const keyword.
When a variable is declared using const , it cannot be reassigned or redeclared.

Here is an example of how the error occurs.
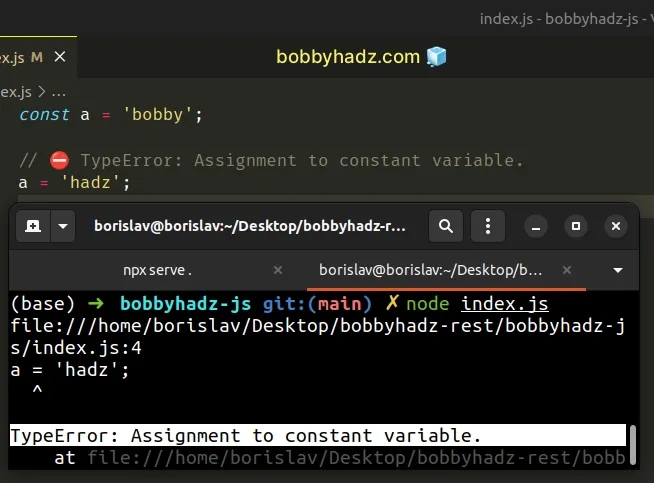
# Declare the variable using let instead of const
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const .
Variables declared using the let keyword can be reassigned.
We used the let keyword to declare the variable in the example.
Variables declared using let can be reassigned, as opposed to variables declared using const .
You can also use the var keyword in a similar way. However, using var in newer projects is discouraged.
# Pick a different name for the variable
Alternatively, you can declare a new variable using the const keyword and use a different name.
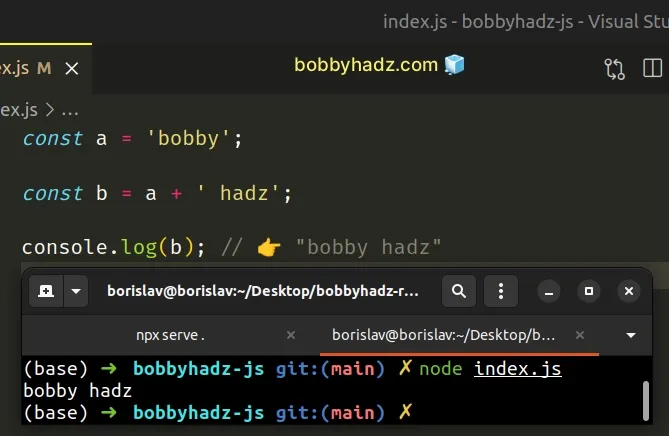
We declared a variable with a different name to resolve the issue.
The two variables no longer clash, so the "assignment to constant" variable error is no longer raised.
# Declaring a const variable with the same name in a different scope
You can also declare a const variable with the same name in a different scope, e.g. in a function or an if block.
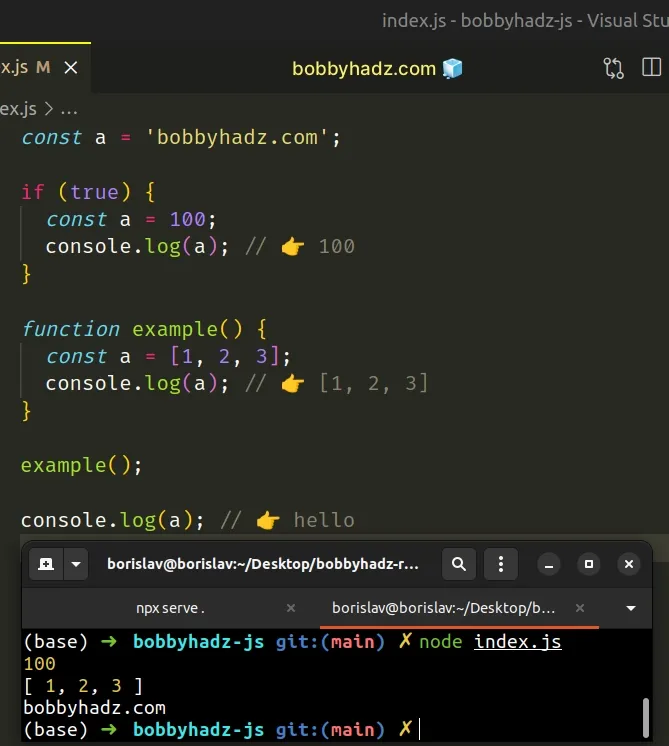
The if statement and the function have different scopes, so we can declare a variable with the same name in all 3 scopes.
However, this prevents us from accessing the variable from the outer scope.
# The const keyword doesn't make objects immutable
Note that the const keyword prevents us from reassigning or redeclaring a variable, but it doesn't make objects or arrays immutable.
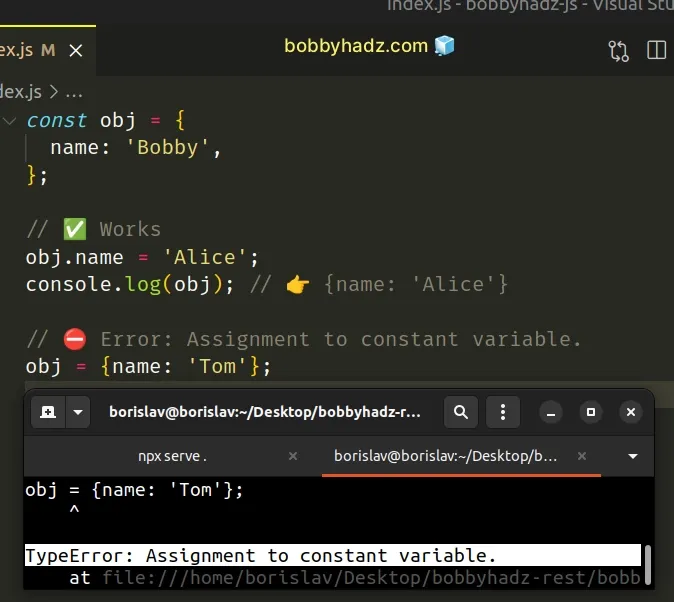
We declared an obj variable using the const keyword. The variable stores an object.
Notice that we are able to directly change the value of the name property even though the variable was declared using const .
The behavior is the same when working with arrays.
Even though we declared the arr variable using the const keyword, we are able to directly change the values of the array elements.
The const keyword prevents us from reassigning the variable, but it doesn't make objects and arrays immutable.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: Unterminated string constant in JavaScript
- TypeError (intermediate value)(...) is not a function in JS
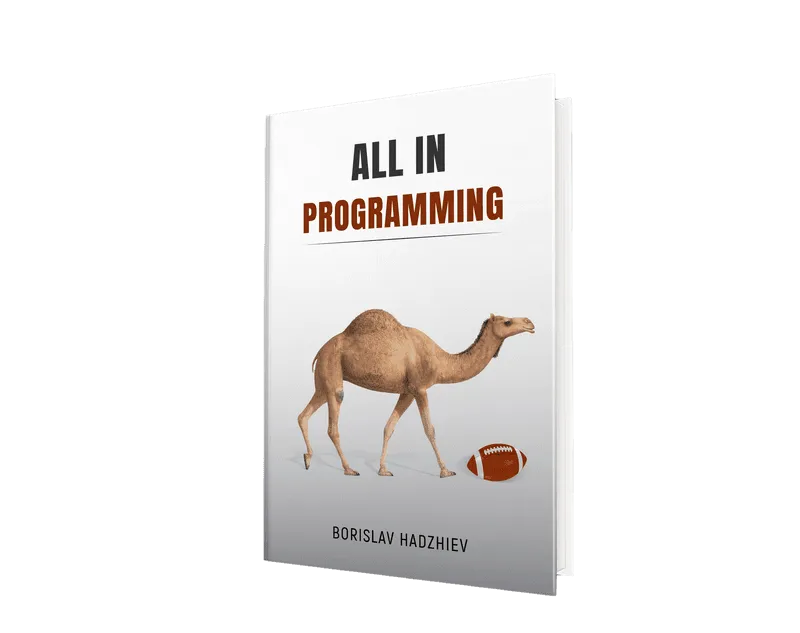
Borislav Hadzhiev
Web Developer
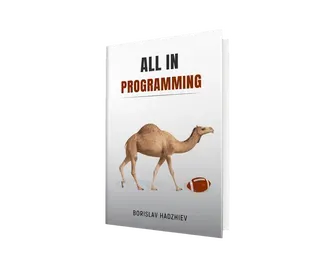
Copyright © 2024 Borislav Hadzhiev
- Skip to main content
- Select language
- Skip to search
TypeError: invalid assignment to const "x"
Const and immutability, what went wrong.
A constant is a value that cannot be altered by the program during normal execution. It cannot change through re-assignment, and it can't be redeclared. In JavaScript, constants are declared using the const keyword.
Invalid redeclaration
Assigning a value to the same constant name in the same block-scope will throw.
Fixing the error
There are multiple options to fix this error. Check what was intended to be achieved with the constant in question.
If you meant to declare another constant, pick another name and re-name. This constant name is already taken in this scope.
const , let or var ?
Do not use const if you weren't meaning to declare a constant. Maybe you meant to declare a block-scoped variable with let or global variable with var .
Check if you are in the correct scope. Should this constant appear in this scope or was is meant to appear in a function, for example?
The const declaration creates a read-only reference to a value. It does not mean the value it holds is immutable, just that the variable identifier cannot be reassigned. For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable:
But you can mutate the properties in a variable:
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy." href="Property_access_denied.html">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing variable name
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: cyclic object value
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting a property that has only a getter
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- SYEDHUSSIM.COM
SAP Business One
Difference between const, let and var.
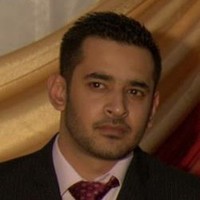
- Author Syed Hussim
- Published 12 July 2019
- Viewed 1798
Part 2 of A Javascript Implementation Of The Logo Programming Language. In the previous article I explained how to develop a simple lexer. In this part we develop a TokenCollection class to help traverse the array of tokens returned from the lexer.
In this four part article, I explain how to develop the iconic Logo programming language in JavaScript. In this part, we discuss how to take a source input and convert it into a series of tokens.
If you're building a REST API, chances are you're going to need to generate secure random API keys. In this article, I explain how to use the Node.js crypto module to generate random secure API keys.
A simple port scanner to scan a range of ports.
An introduction to sorting algorithms.
This article explains how to create a simple HTTP server using Node.js without using any dependencies.
Node.Js provides several ways to read user input from the terminal. This article explains how to use the process object to read user input.
SAP HANA has several functions to help query JSON data and in this article, we'll take a look at how to use the JSON_TABLE function to query JSON data stored in a regular column.
The hdbconnect package makes it easy to connect to a HANA database using Rust and in this article, we'll take a look at how to establish a connection and perform some queries.
In this last article on developing a command line program, we'll develop a program that counts the occurrence of different file types in a directory and its sub-directories. The program will accept a directory as an argument and recursively scan all sub-directories. We'll use a HashMap to keep track of the count for each file type.
In the previous article, we developed a simple command line program that counted how many times a word occurred in a text file. The article gave us a brief introduction to the env, collections, and fs modules. In this article, we'll take a look at the TcpStream struct from the net module to develop a simple port scanner.
The best way to learn any programming language is to develop small meaningful programs that are easy to write and serve a purpose. In this three part article, we'll develop several small command line programs. Each article will focus on a different part of the standard library.
In part 1, we developed a simple single-threaded HTTP server that processed requests synchronously. In part 2, we modified the server so that each request was handled in a separate thread, which improved concurrency but increased the load on the CPU and memory. In this article, we'll modify the server to use a ThreadPool which will help to improve concurrency while reducing the CPU and memory usage.
In part 1, we developed a simple single-threaded HTTP server that returned a static string. While single-threaded servers have their use cases, they lack scalability. The nature of a single-threaded server means it can only process one request at a time. If there's a request that's taking a long time to process, all other requests will be blocked until the slow request has been completed. If we want to handle multiple requests at the same time, we need to use threads.
NodeJs has built-in http modules that allow developers to build web applications without the need to configure and run a proprietary HTTP server such as Apache or Nginx. Other programming languages provide similar libraries that allow developers to bundle a server with an application. So in this article, we'll take a look at how easy it is to write a simple single-threaded HTTP server in Java. Once we're done, we can run a simple test using Apaches ab tool to determine how many requests per second the server can handle.
Batch managed items can be transferred between warehouses and bins by including the BatchNumbers property in the StockTransfers API post request. The BatchNumbers property is an array of batch numbers. Listing 1 below shows an example JSON object that contains an item with a single batch. Notice that the quantity specified for the batch number object is equal to the quantity at the item level.
Developing inventory management tools requires a good understanding of SAP Business One API's. Many API's in the Service Layer documentation lack payload samples leaving developers to do their own research. This article aims to help developers understand the payload data that is required to create a stock transfer.
In this article we continue developing the Logo Programming language further by writing a simple TokenCollection class to help traverse the array of tokens returned from the lexer we created in part 1. This class will be used in Part 3, when we develop the parser.
The Logo programming language was designed by Wally Feurzeig, Seymour Papert, and Cynthia Solomon in 1967 as an educational tool. The language was used to program a robotic turtle that would draw graphics given a series of instructions. In later years the physical robot was replaced by a virtual on-screen turtle. Over the years, various institutions and organizations have implemented the language with additional features. Notable major implementations are UCBLogo and MSWLogo.
If you're building a REST API, chances are you're going to need to generate secure random API keys. You may be tempted to use a third-party library but before you do, consider using the crypto package from Node.js. The crypto module can generate random bytes, which can then be used to create your API keys.
NodeJs has become one of my go to tools for developing small utilities and services extremely quickly. One such tool that I've developed, is a simple port scanner to help determine which ports are being used.
I don't often start an article asking a question, but I'll make an exception today. Can you take the following array [8,3,1,9,6,5,7,2,4] and sort it in ascending order? Fairly easy right? Many programming languages provide sorting functions that make tasks such as this a one liner.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript const.
The const keyword was introduced in ES6 (2015)
Variables defined with const cannot be Redeclared
Variables defined with const cannot be Reassigned
Variables defined with const have Block Scope
Cannot be Reassigned
A variable defined with the const keyword cannot be reassigned:
Must be Assigned
JavaScript const variables must be assigned a value when they are declared:
When to use JavaScript const?
Always declare a variable with const when you know that the value should not be changed.
Use const when you declare:
- A new Array
- A new Object
- A new Function
- A new RegExp
Constant Objects and Arrays
The keyword const is a little misleading.
It does not define a constant value. It defines a constant reference to a value.
Because of this you can NOT:
- Reassign a constant value
- Reassign a constant array
- Reassign a constant object
But you CAN:
- Change the elements of constant array
- Change the properties of constant object
Constant Arrays
You can change the elements of a constant array:
But you can NOT reassign the array:
Constant Objects
You can change the properties of a constant object:
But you can NOT reassign the object:
Difference Between var, let and const
What is good.
let and const have block scope .
let and const can not be redeclared .
let and const must be declared before use.
let and const does not bind to this .
let and const are not hoisted .
What is Not Good?
var does not have to be declared.
var is hoisted.
var binds to this.
Browser Support
The let and const keywords are not supported in Internet Explorer 11 or earlier.
The following table defines the first browser versions with full support:
Advertisement
Block Scope
Declaring a variable with const is similar to let when it comes to Block Scope .
The x declared in the block, in this example, is not the same as the x declared outside the block:
You can learn more about block scope in the chapter JavaScript Scope .
Redeclaring
Redeclaring a JavaScript var variable is allowed anywhere in a program:
Redeclaring an existing var or let variable to const , in the same scope, is not allowed:
Reassigning an existing const variable, in the same scope, is not allowed:
Redeclaring a variable with const , in another scope, or in another block, is allowed:
Variables defined with var are hoisted to the top and can be initialized at any time.
Meaning: You can use the variable before it is declared:
This is OK:
Variables defined with const are also hoisted to the top, but not initialized.
Meaning: Using a const variable before it is declared will result in a ReferenceError :

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- [email protected]
- 🇮🇳 +91 (630)-411-6234
- Reactjs Development Services
- Flutter App Development Services
- Mobile App Development Services
Web Development
Mobile app development, nodejs typeerror: assignment to constant variable.
Published By: Divya Mahi
Published On: November 17, 2023
Published In: Development
Grasping and Fixing the 'NodeJS TypeError: Assignment to Constant Variable' Issue
Introduction.
Node.js, a powerful platform for building server-side applications, is not immune to errors and exceptions. Among the common issues developers encounter is the “NodeJS TypeError: Assignment to Constant Variable.” This error can be a source of frustration, especially for those new to JavaScript’s nuances in Node.js. In this comprehensive guide, we’ll explore what this error means, its typical causes, and how to effectively resolve it.
Understanding the Error
In Node.js, the “TypeError: Assignment to Constant Variable” occurs when there’s an attempt to reassign a value to a variable declared with the const keyword. In JavaScript, const is used to declare a variable that cannot be reassigned after its initial assignment. This error is a safeguard in the language to ensure the immutability of variables declared as constants.
Diving Deeper
This TypeError is part of JavaScript’s efforts to help developers write more predictable code. Immutable variables can prevent bugs that are hard to trace, as they ensure that once a value is set, it cannot be inadvertently changed. However, it’s important to distinguish between reassigning a variable and modifying an object’s properties. The latter is allowed even with variables declared with const.
Common Scenarios and Fixes
Example 1: reassigning a constant variable.
Javascript:
Fix: Use let if you need to reassign the variable.
Example 2: Modifying an Object's Properties
Fix: Modify the property instead of reassigning the object.
Example 3: Array Reassignment
Fix: Modify the array’s contents without reassigning it.
Example 4: Within a Function Scope
Fix: Declare a new variable or use let if reassignment is needed.
Example 5: In Loops
Fix: Use let for variables that change within loops.
Example 6: Constant Function Parameters
Fix: Avoid reassigning function parameters directly; use another variable.
Example 7: Constants in Conditional Blocks
Fix: Use let if the variable needs to change.
Example 8: Reassigning Properties of a Constant Object
Fix: Modify only the properties of the object.
Strategies to Prevent Errors
Understand const vs let: Familiarize yourself with the differences between const and let. Use const for variables that should not be reassigned and let for those that might change.
Code Reviews: Regular code reviews can catch these issues before they make it into production. Peer reviews encourage adherence to best practices.
Linter Usage: Tools like ESLint can automatically detect attempts to reassign constants. Incorporating a linter into your development process can prevent such errors.
Best Practices
Immutability where Possible: Favor immutability in your code to reduce side effects and bugs. Normally use const to declare variables, and use let only if you need to change their values later .
Descriptive Variable Names: Use clear and descriptive names for your variables. This practice makes it easier to understand when a variable should be immutable.
Keep Functions Pure: Avoid reassigning or modifying function arguments. Keeping functions pure (not causing side effects) leads to more predictable and testable code.
The “NodeJS TypeError: Assignment to Constant Variable” error, while common, is easily avoidable. By understanding JavaScript’s variable declaration nuances and adopting coding practices that embrace immutability, developers can write more robust and maintainable Node.js applications. Remember, consistent coding standards and thorough code reviews are your best defense against common errors like these.
Related Articles
March 13, 2024
Expressjs Error: 405 Method Not Allowed
March 11, 2024
Expressjs Error: 502 Bad Gateway
I’m here to assist you.
Something isn’t Clear? Feel free to contact Us, and we will be more than happy to answer all of your questions.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Error Object Complete Reference
JS Range Error
- JavaScript RangeError - Invalid date
- JavaScript RangeError - Repeat count must be non-negative
JS Reference Error
- JavaScript ReferenceError - Can't access lexical declaration`variable' before initialization
- JavaScript ReferenceError - Invalid assignment left-hand side
- JavaScript ReferenceError - Assignment to undeclared variable
- JavaScript ReferenceError - Reference to undefined property "x"
- JavaScript ReferenceError - variable is not defined
- JavaScript ReferenceError Deprecated caller or arguments usage
JS Syntax Error
- JavaScript SyntaxError - Illegal character
- JavaScript SyntaxError - Identifier starts immediately after numeric literal
- JavaScript SyntaxError - Function statement requires a name
- JavaScript SyntaxError - Missing } after function body
- JavaScript SyntaxError - Missing } after property list
- JavaScript SyntaxError - Missing variable name
- JavaScript SyntaxError - Missing ] after element list
- JavaScript SyntaxError - Invalid regular expression flag "x"
- JavaScript SyntaxError "variable" is a reserved identifier
- JavaScript SyntaxError - Missing ':' after property id
- JavaScript SyntaxError - Missing ) after condition
- JavaScript SyntaxError - Missing formal parameter
- JavaScript SyntaxError - Missing ; before statement
- JavaScript SyntaxError - Missing = in const declaration
- JavaScript SyntaxError - Missing name after . operator
- JavaScript SyntaxError - Redeclaration of formal parameter "x"
- JavaScript SyntaxError - Missing ) after argument list
- JavaScript SyntaxError - Return not in function
- JavaScript SyntaxError: Unterminated string literal
- JavaScript SyntaxError - Applying the 'delete' operator to an unqualified name is deprecated
- JavaScript SyntaxError - Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- JavaScript SyntaxError - Malformed formal parameter
- JavaScript SyntaxError - "0"-prefixed octal literals and octal escape sequences are deprecated
- JavaScript SyntaxError - Test for equality (==) mistyped as assignment (=)?
- JavaScript SyntaxError - "x" is not a legal ECMA-262 octal constant
JS Type Error
- JavaScript TypeError - "X" is not a non-null object
- JavaScript TypeError - "X" is not a constructor
- JavaScript TypeError - "X" has no properties
- JavaScript TypeError - "X" is (not) "Y"
- JavaScript TypeError - "X" is not a function
- JavaScript TypeError - 'X' is not iterable
- JavaScript TypeError - More arguments needed
- JavaScript TypeError - "X" is read-only
- JavaScript TypeError - Reduce of empty array with no initial value
- JavaScript TypeError - Can't assign to property "X" on "Y": not an object
- JavaScript TypeError - Can't access property "X" of "Y"
- JavaScript TypeError - Can't define property "X": "Obj" is not extensible
- JavaScript TypeError - X.prototype.y called on incompatible type
- JavaScript TypeError - Invalid assignment to const "X"
- JavaScript TypeError - Property "X" is non-configurable and can't be deleted
- JavaScript TypeError - Can't redefine non-configurable property "x"
- JavaScript TypeError - Variable "x" redeclares argument
- JavaScript TypeError - Setting getter-only property "x"
- JavaScript TypeError - Invalid 'instanceof' operand 'x'
- JavaScript TypeError - Invalid Array.prototype.sort argument
- JavaScript TypeError - Cyclic object value
- JavaScript TypeError - Can't delete non-configurable array element
JS Other Errors
- JavaScript URIError | Malformed URI Sequence
- JavaScript Warning - Date.prototype.toLocaleFormat is deprecated
- Logging Script Errors in JavaScript
JS Error Instance
- JavaScript Error message Property
- JavaScript Error name Property
- JavaScript Error.prototype.toString() Method

JavaScript TypeError – Invalid assignment to const “X”
This JavaScript exception invalid assignment to const occurs if a user tries to change a constant value. Const declarations in JavaScript can not be re-assigned or re-declared.
Error Type:
Cause of Error: A const value in JavaScript is changed by the program which can not be altered during normal execution.
Example 1: In this example, the value of the variable(‘GFG’) is changed, So the error has occurred.
Output(in console):
Example 2: In this example, the value of the object(‘GFG_Obj’) is changed, So the error has occurred.
Please Login to comment...
Similar reads.
- JavaScript-Errors
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
11 Variables and assignment
11.1 let, 11.2.1 const and immutability, 11.2.2 const and loops.
- 11.3 Deciding between const and let
- 11.4.1 Shadowing variables
- 11.5 (Advanced)
- 11.6.1 Static phenomenon: scopes of variables
- 11.6.2 Dynamic phenomenon: function calls
11.7.1 globalThis [ES2020]
11.8.1 const and let : temporal dead zone.
- 11.8.2 Function declarations and early activation
- 11.8.3 Class declarations are not activated early
11.8.4 var : hoisting (partial early activation)
- 11.9.1 Bound variables vs. free variables
- 11.9.2 What is a closure?
- 11.9.3 Example: A factory for incrementors
- 11.9.4 Use cases for closures
These are JavaScript’s main ways of declaring variables:
- let declares mutable variables.
- const declares constants (immutable variables).
Before ES6, there was also var . But it has several quirks, so it’s best to avoid it in modern JavaScript. You can read more about it in Speaking JavaScript .
Variables declared via let are mutable:
You can also declare and assign at the same time:
11.2 const
Variables declared via const are immutable. You must always initialize immediately:
In JavaScript, const only means that the binding (the association between variable name and variable value) is immutable. The value itself may be mutable, like obj in the following example.
You can use const with for-of loops, where a fresh binding is created for each iteration:
In plain for loops, you must use let , however:
11.3 Deciding between const and let
I recommend the following rules to decide between const and let :
- const indicates an immutable binding and that a variable never changes its value. Prefer it.
- let indicates that the value of a variable changes. Use it only when you can’t use const .
exercises/variables-assignment/const_exrc.mjs
11.4 The scope of a variable
The scope of a variable is the region of a program where it can be accessed. Consider the following code.
- Scope A is the (direct) scope of x .
- Scopes B and C are inner scopes of scope A.
- Scope A is an outer scope of scope B and scope C.
Each variable is accessible in its direct scope and all scopes nested within that scope.
The variables declared via const and let are called block-scoped because their scopes are always the innermost surrounding blocks.
11.4.1 Shadowing variables
You can’t declare the same variable twice at the same level:
eval() delays parsing (and therefore the SyntaxError ), until the callback of assert.throws() is executed. If we didn’t use it, we’d already get an error when this code is parsed and assert.throws() wouldn’t even be executed.
You can, however, nest a block and use the same variable name x that you used outside the block:
Inside the block, the inner x is the only accessible variable with that name. The inner x is said to shadow the outer x . Once you leave the block, you can access the old value again.
See quiz app .
11.5 (Advanced)
All remaining sections are advanced.
11.6 Terminology: static vs. dynamic
These two adjectives describe phenomena in programming languages:
- Static means that something is related to source code and can be determined without executing code.
- Dynamic means at runtime.
Let’s look at examples for these two terms.
11.6.1 Static phenomenon: scopes of variables
Variable scopes are a static phenomenon. Consider the following code:
x is statically (or lexically ) scoped . That is, its scope is fixed and doesn’t change at runtime.
Variable scopes form a static tree (via static nesting).
11.6.2 Dynamic phenomenon: function calls
Function calls are a dynamic phenomenon. Consider the following code:
Whether or not the function call in line A happens, can only be decided at runtime.
Function calls form a dynamic tree (via dynamic calls).
11.7 Global variables and the global object
JavaScript’s variable scopes are nested. They form a tree:
- The outermost scope is the root of the tree.
- The scopes directly contained in that scope are the children of the root.
The root is also called the global scope . In web browsers, the only location where one is directly in that scope is at the top level of a script. The variables of the global scope are called global variables and accessible everywhere. There are two kinds of global variables:
- They can only be created while at the top level of a script, via const , let , and class declarations.
- They are created in the top level of a script, via var and function declarations.
- The global object can be accessed via the global variable globalThis . It can be used to create, read, and delete global object variables.
- Other than that, global object variables work like normal variables.
The following HTML fragment demonstrates globalThis and the two kinds of global variables.
Each ECMAScript module has its own scope. Therefore, variables that exist at the top level of a module are not global. Fig. 5 illustrates how the various scopes are related.
The global variable globalThis is the new standard way of accessing the global object. It got its name from the fact that it has the same value as this in global scope.
For example, in browsers, there is an indirection . That indirection is normally not noticable, but it is there and can be observed.
11.7.1.1 Alternatives to globalThis
Older ways of accessing the global object depend on the platform:
- Global variable window : is the classic way of referring to the global object. But it doesn’t work in Node.js and in Web Workers.
- Global variable self : is available in Web Workers and browsers in general. But it isn’t supported by Node.js.
- Global variable global : is only available in Node.js.
11.7.1.2 Use cases for globalThis
The global object is now considered a mistake that JavaScript can’t get rid of, due to backward compatibility. It affects performance negatively and is generally confusing.
ECMAScript 6 introduced several features that make it easier to avoid the global object – for example:
- const , let , and class declarations don’t create global object properties when used in global scope.
- Each ECMAScript module has its own local scope.
It is usually better to access global object variables via variables and not via properties of globalThis . The former has always worked the same on all JavaScript platforms.
Tutorials on the web occasionally access global variables globVar via window.globVar . But the prefix “ window. ” is not necessary and I recommend to omit it:
Therefore, there are relatively few use cases for globalThis – for example:
- Polyfills that add new features to old JavaScript engines.
- Feature detection, to find out what features a JavaScript engine supports.
11.8 Declarations: scope and activation
These are two key aspects of declarations:
- Scope: Where can a declared entity be seen? This is a static trait.
- Activation: When can I access an entity? This is a dynamic trait. Some entities can be accessed as soon as we enter their scopes. For others, we have to wait until execution reaches their declarations.
Tbl. 1 summarizes how various declarations handle these aspects.
import is described in §27.5 “ECMAScript modules” . The following sections describe the other constructs in more detail.
For JavaScript, TC39 needed to decide what happens if you access a constant in its direct scope, before its declaration:
Some possible approaches are:
- The name is resolved in the scope surrounding the current scope.
- You get undefined .
- There is an error.
Approach 1 was rejected because there is no precedent in the language for this approach. It would therefore not be intuitive to JavaScript programmers.
Approach 2 was rejected because then x wouldn’t be a constant – it would have different values before and after its declaration.
let uses the same approach 3 as const , so that both work similarly and it’s easy to switch between them.
The time between entering the scope of a variable and executing its declaration is called the temporal dead zone (TDZ) of that variable:
- During this time, the variable is considered to be uninitialized (as if that were a special value it has).
- If you access an uninitialized variable, you get a ReferenceError .
- Once you reach a variable declaration, the variable is set to either the value of the initializer (specified via the assignment symbol) or undefined – if there is no initializer.
The following code illustrates the temporal dead zone:
The next example shows that the temporal dead zone is truly temporal (related to time):
Even though func() is located before the declaration of myVar and uses that variable, we can call func() . But we have to wait until the temporal dead zone of myVar is over.
11.8.2 Function declarations and early activation
In this section, we are using functions – before we had a chance to learn them properly. Hopefully, everything still makes sense. Whenever it doesn’t, please see §25 “Callable values” .
A function declaration is always executed when entering its scope, regardless of where it is located within that scope. That enables you to call a function foo() before it is declared:
The early activation of foo() means that the previous code is equivalent to:
If you declare a function via const or let , then it is not activated early. In the following example, you can only use bar() after its declaration.
11.8.2.1 Calling ahead without early activation
Even if a function g() is not activated early, it can be called by a preceding function f() (in the same scope) if we adhere to the following rule: f() must be invoked after the declaration of g() .
The functions of a module are usually invoked after its complete body is executed. Therefore, in modules, you rarely need to worry about the order of functions.
Lastly, note how early activation automatically keeps the aforementioned rule: when entering a scope, all function declarations are executed first, before any calls are made.
11.8.2.2 A pitfall of early activation
If you rely on early activation to call a function before its declaration, then you need to be careful that it doesn’t access data that isn’t activated early.
The problem goes away if you make the call to funcDecl() after the declaration of MY_STR .
11.8.2.3 The pros and cons of early activation
We have seen that early activation has a pitfall and that you can get most of its benefits without using it. Therefore, it is better to avoid early activation. But I don’t feel strongly about this and, as mentioned before, often use function declarations because I like their syntax.
11.8.3 Class declarations are not activated early
Even though they are similar to function declarations in some ways, class declarations are not activated early:
Why is that? Consider the following class declaration:
The operand of extends is an expression. Therefore, you can do things like this:
Evaluating such an expression must be done at the location where it is mentioned. Anything else would be confusing. That explains why class declarations are not activated early.
var is an older way of declaring variables that predates const and let (which are preferred now). Consider the following var declaration.
This declaration has two parts:
- Declaration var x : The scope of a var -declared variable is the innermost surrounding function and not the innermost surrounding block, as for most other declarations. Such a variable is already active at the beginning of its scope and initialized with undefined .
- Assignment x = 123 : The assignment is always executed in place.
The following code demonstrates the effects of var :
11.9 Closures
Before we can explore closures, we need to learn about bound variables and free variables.
11.9.1 Bound variables vs. free variables
Per scope, there is a set of variables that are mentioned. Among these variables we distinguish:
- Bound variables are declared within the scope. They are parameters and local variables.
- Free variables are declared externally. They are also called non-local variables .
Consider the following code:
In the body of func() , x and y are bound variables. z is a free variable.
11.9.2 What is a closure?
What is a closure then?
A closure is a function plus a connection to the variables that exist at its “birth place”.
What is the point of keeping this connection? It provides the values for the free variables of the function – for example:
funcFactory returns a closure that is assigned to func . Because func has the connection to the variables at its birth place, it can still access the free variable value when it is called in line A (even though it “escaped” its scope).
Static scoping is supported via closures in JavaScript. Therefore, every function is a closure.
11.9.3 Example: A factory for incrementors
The following function returns incrementors (a name that I just made up). An incrementor is a function that internally stores a number. When it is called, it updates that number by adding the argument to it and returns the new value.
We can see that the function created in line A keeps its internal number in the free variable startValue . This time, we don’t just read from the birth scope, we use it to store data that we change and that persists across function calls.
We can create more storage slots in the birth scope, via local variables:
11.9.4 Use cases for closures
What are closures good for?
For starters, they are simply an implementation of static scoping. As such, they provide context data for callbacks.
They can also be used by functions to store state that persists across function calls. createInc() is an example of that.
And they can provide private data for objects (produced via literals or classes). The details of how that works are explained in Exploring ES6 .
Using Global Variables in Node.js
Hey guys, in today's article I want to talk about global variables in Node. This article is aimed at developers who are at a beginner to intermediate skill level working with Node. If you have never heard of global variables or worked with them, no need to worry. This article will get you up and running in no time with everything you need to know about global variables.
- What are Global Variables?
Global variables are very similar, if not identical, to regular variables. Global variables can be initialized with a value, that value can be changed, and they can even be cleared out like a regular variable. The difference between a regular variable and a global variable comes down to their scope. When you create a variable in a JavaScript file, that variable only exists in the scope that it was declared in. Now what do I mean by this? In the code below, you can see an example of two different variables with different scopes.
In the code snippet above, we can see that there are two variables, fileScope and localScope . The variable fileScope can be changed or called from anywhere within this file, whereas the localScope variable only exists inside the function doSomething() . I'm sure at this point you are wondering what this has to do with global variables. When we talk about global variables, they exist for all of the files in a program meaning they have global scope for the program.
The reason this is possible is because JavaScript programs share a global namespace between all of the files in the program. To put it another way, imagine that your program is a giant file or container that has "imported" all the other JavaScript files. You then declare a variable in this large container file, that variable now has scope throughout the whole program. If you are not sure what a namespace is or you want to find out more about them, check out this article to learn more.
- How to Declare and Use a Global Variable
Now that we have a better understanding of what a global variable in Node is, let's talk about how we actually set up and use a global variable. To set up a global variable, we need to create it on the global object. The global object is what gives us the scope of the entire project, rather than just the file (module) the variable was created in. In the code block below, we create a global variable called globalString and we give it a value. Next, we change the value of globalString , and then finally we set it to undefined.
What I have not talked about yet is another way that you can make a variable global. The reason I have excluded this is because it is not a proper way of setting up a variable. If you declare a variable in a file without using the keyword var and then assign a value to it, the global object will set a property for this variable. This process essentially turns it into a globally accessible variable. I strongly advise against using this method though as it is not the proper way to go about creating globals. It is also important to note that if you set the 'use strict' directive, Node will disable implicit globals and you will likely end up with an error at runtime rather than a working script.
- Practical Use Cases for Global Variables
Now, you might be thinking to yourself that you want to go off and create global variables now that you know more about them. I am going to strongly caution against creating global variables for a few very important reasons.
The first reason is that when you create a global variable, it exists throughout the lifetime of the application. When a variable persists through the lifetime of the app it means that it is there, in memory, occupying resources while the app is running.
Second, traditionally using global variables can cause concurrency issues. If multiple threads can access the same variable and there are no access modifiers or fail-safes in place, it can lead to some serious issues of two threads attempting to access and use the same variable. However , while this is the case in other languages, it is not necessarily the case for Node.js as it is strictly a single-threaded environment. While it is possible to cluster Node processes, there is no native way to communicate between them.
The last reason I am going to talk about is that using globals can cause implicit coupling between files or variables. Coupling is not a good thing when it comes to writing great code. When writing code, we want to make sure that it is as modular and reusable as possible, while also making sure it is easy to use and understand. Coupling pieces of your code together can lead to some major headaches down the road when you are trying to debug why something isn't working.
If you want to know more about why globals are not recommended, you can check out this great article called Global Variables Are Bad .
If you feel confused as to the purpose of global variables, fear not. We are going to take a look at a few of the global variables that are built into Node and try to get a better understanding of why they are global and how they are used. In fact, you have probably used a few of them already without even realizing that they are global objects!
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!
If you take a look at the above code block you will probably see at least one instance you have used before, console.log() . According to the Node documentation, the console object is a global that has a few methods allowing developers to do things such as printing a log or an error. Digging deeper into the docs we can see that console is really a global instance that is configured to write to process.stdout and process.stderr .
This brings us to the next statement that you see in the code block above, the process object. If you have put up a production build of a Node application, then you have likely had to set the port for the environment variable. The environment variable env is a part of the process object which is another global. You can access variables on the process object in any file in your project because it is global. If this object was not global, the console object would not be accessible from any file either, remember it is really an object that refers back to the process object.
setInterval is another function that you may have seen before if you ever had reason to delay an operation before executing it. setTimeout and setImmediate are similar in nature to setInterval and are both global functions as well. These three functions are a part of the timer module which exposes a global API allowing you to call these functions without requiring a timer in your files explicitly.
All of the above mentioned use cases are built into Node and are global for a reason. The process object is global because it provides information about the current running Node process and therefore should be available from any file without having to require it. The same can be said of the timer module which contains a number of functions that are important and should be accessible anywhere without having to require it. If you would like to learn more about the existing global objects built into Node, I encourage you to visit the official documentation on Globals .
Want to learn more about the fundamentals of Node.js? Personally, I'd recommend an online course, like Wes Bos' Learn Node.js since the videos are much easier to follow and you'll actually get to build a real-world application.
I know that was quite a bit of information, so thank you for sticking it out. All of the above information was found in the documentation on Node's website . Please feel free to ask questions and give comments in the comment section below. Until next time guys!
You might also like...
- Node.js Websocket Examples with Socket.io
- Neural Networks in JavaScript with Brain.js
- Publishing and Subscribing to AWS SNS Messages with Node.js
- Message Queueing in Node.js with AWS SQS
Improve your dev skills!
Get tutorials, guides, and dev jobs in your inbox.
No spam ever. Unsubscribe at any time. Read our Privacy Policy.
Sam is an iOS developer who loves working with Swift and is currently doing freelance iOS work. When not coding, he can be found playing video games on Steam or writing articles.
In this article
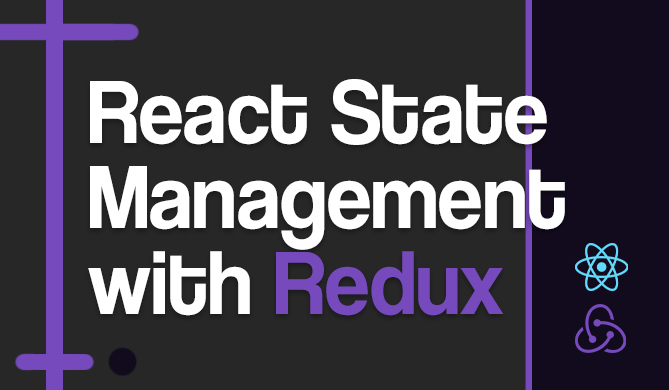
React State Management with Redux and Redux-Toolkit
Coordinating state and keeping components in sync can be tricky. If components rely on the same data but do not communicate with each other when...
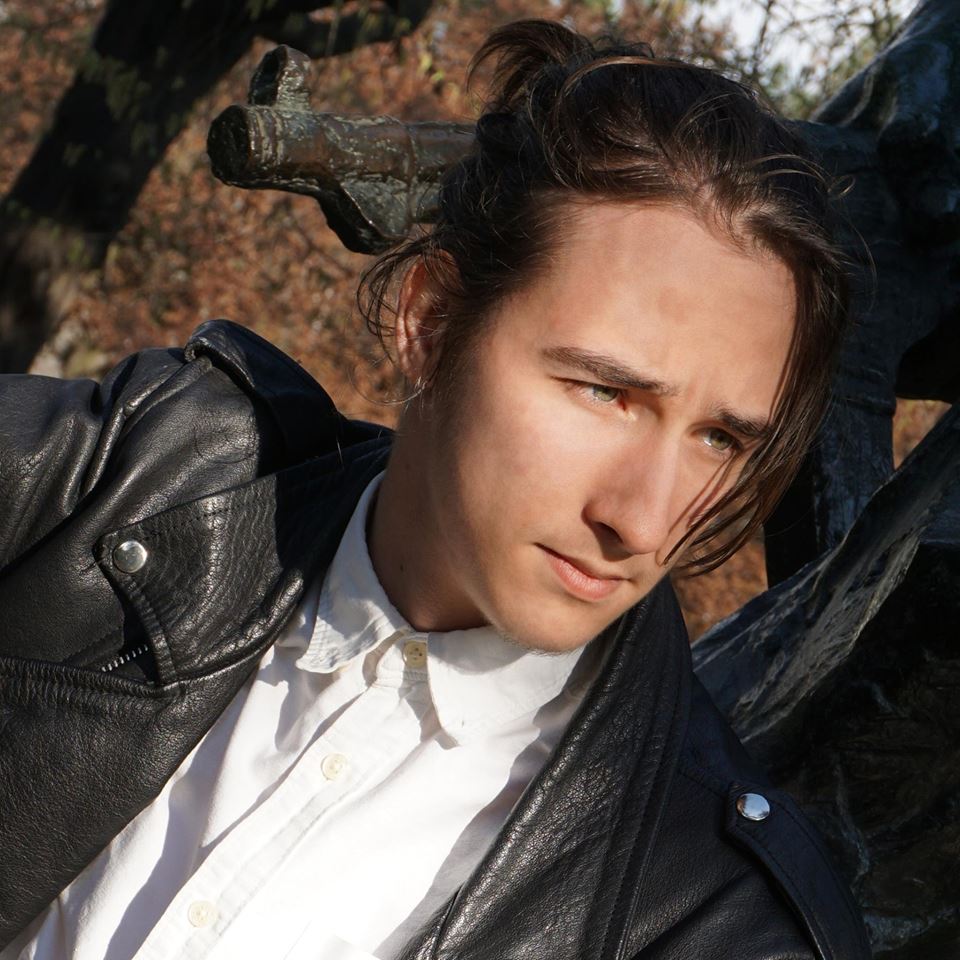
Getting Started with AWS in Node.js
Build the foundation you'll need to provision, deploy, and run Node.js applications in the AWS cloud. Learn Lambda, EC2, S3, SQS, and more!
© 2013- 2024 Stack Abuse. All rights reserved.

Constant Variables in JavaScript, or: When "const" Isn't Constant
ECMAScript 2015 introduced the let and const keywords as alternatives to var , which JavaScript has always had. Both let and const declare local variables with block scope rather than function scope . In addition, const provides some notion of constancy, which let doesn't.
Unfortunately, the name of the const keyword might be misleading. In JavaScript, const does not mean constant , but one-time assignment . It's a subtle yet important distinction. Let's see what one-time assignment means:
However, variables declared using the const keyword do not generally have a truly immutable value. Remember, const does not mean "constant", it means one-time assignment . The part that's constant is the reference to an object stored within the constant variable, not the object itself. The following example illustrates the difference:
Declaring a variable to be constant doesn't make the objects it references immutable, as the above example shows. Object properties can change or be deleted altogether. The same goes for arrays assigned to a constant variable; Elements can be added, removed, reordered, or modified:
For the sake of completeness, it is possible to create true constants in some cases. If a primitive value (such as a string, number, or boolean value) is assigned to a constant variable, that variable will be a true constant. Our PI constant is an example for this. There's no way to modify the value of the numeric literal 3.141592653589793 after it has been assigned.
To make an object truly immutable, you can pass it to the Object.freeze function to prevent any changes to its properties. Be aware that freeze is shallow, so you'll have to recursively call it for nested objects if you want the entire object tree to be frozen. If you need immutable data structures, it might be safer and more convenient to use a library such as Facebook's Immutable.js which is specifically made for this purpose.
Variable Scope in Modern JavaScript
It often surprises me when speaking to other JavaScript developers how many are unaware of how variable scope works in the language they code in. By scope we are talking about the visibility of variables in our code; or in other words which parts of the code can access and modify a variable. I regularly find people declaring variables with var in the middle of code, not knowing how JavaScript will scope these.
JavaScript has been going through some big changes over the last few years; these include the addition of a couple of interesting new keywords for declaring variables and deal with scope in new ways to what has come before. let and const were added as part of ES6 ( a.k.a. ES2015) which has been around for three years now; browser support is good and for everything else there’s Babel for transpiling ES6 into a more widely adopted JavaScript. So now is a good opportunity to look again at how we declare variables and gain some understanding of how they scope.
In this blog post I am going to run through a load of JavaScript examples to show how global , local and block scopes work. We will also look at let and const statements for anyone who is not already familiar with these.
Global Scope
Let’s begin by considering global scope . Globally declared variables can be accessed and modified anywhere in the code (well almost, but we will come to the exceptions later).
Global variables are declared in the code outside of any function definitions, in the top-level scope.
In this example, a is a global variable; it is therefore readily available in any function within our code. So here we can output the value of a from the method alpha . When we call alpha the value Fred Flinstone is written to the console.
When declaring global variables in a web browser they are also properties of the global window object. Take a look at this example:-
b can be accessed/modified as the property of the window object window.b . Of course it isn’t necessary to assign the new value to b via the window object, this is just to prove a point. We are more likely to write the above as:-
Be careful when using global variables. They can lead to unreadable code that is also difficult to test. I’ve seen many developers come unstuck with global variables trying to discover where the variable’s value is being changed within their codebase causing unexpected bugs. It is far better to pass variables as arguments to functions than rely on globals. Global variables should be used sparingly if at all.
If you really need to use the global scope it is a good idea to namespace your variables so that they become properties of a global object. For example, create a global object named something like globals or app .
If you are using NodeJS then the top-level scope is not the same as the global scope. If you use var foobar in a NodeJS module it is local to that module. To define a global variable in NodeJS we need to use the global namespace object , global .
It’s important to be aware that if you do not declare a variable using one of the keywords var , let or const in your codebase then the variable is given a global scope.
It’s a good idea to always initially declare variables with one of the variable keywords. This way we are in control of each variables scope within our code. Hopefully you can see the potential dangers of forgetting to use a keyword like in the above example.
Local Scope
Now we come to the local scope .
Variables declared within a function are scoped locally to that function. In the examples above both variables b and c are local to their respective methods. b is locally scoped to the function delta as it is being declared as an argument of the method, only the value of a is being passed to the function not the variable itself; c is declared as a local variable of epsilon inside the function. However, what if we have the following code; what does this write to the console?
The answer is 'Jerry' although at first this may not be obvious. This is one of those nasty job interview questions you might get asked if being grilled over your JavaScript abilities. Inside the function zeta we are declaring a new variable d that has a local scope. When using var to declare variables JavaScript initiates the variable at the top of the current scope regardless of where it has been included in the code. So the fact that we have declared d locally within the conditional is irrelevant. Essentially JavaScript is reinterpreting this code as:-
This is known as Hoisting . It’s a feature of JavaScript that is well worth being aware of as it can easily create bugs in your code if variables aren’t initiated at the top of a scope. Thankfully we now have let and const that will help avoid these issues going forward. So let’s take a look at how we can use let to block scope variables…
Block Scope
With the arrival of ES6 a few years ago came two new keywords for declaring variables: let and const . Both keywords allow us to scope to a block of code, that’s anything between two curly braces {} .
let is seen by many as a replacement to the existing var . However this isn’t strictly true as they scope variables differently. Whereas a var statement allows us to create a locally scoped variable, let scopes a variable to the block. Of course a block can be a function allowing us to use let pretty much as we would have used var previously.
Here a is block scoped to the function eta . We can also scope to conditional blocks and loops. The block scope includes any sub-blocks contained within the top-level block that the variable is defined.
In this example b is block scoped to the for loop (which includes the conditional block inside it). So it will output the odd numbers 1 and 3 then throw an error because we can’t access b outside the loop that it was scoped to.
What about our strange little function zeta from earlier where we saw JavaScript’s bizarre hoisting affect? If we rewrite the function to use let what happens?
This time zeta outputs 'Tom' because d is block scoped to the conditional, but does this mean hoisting is not happening here? No, when we use either let or const JavaScript will still hoist the variables to the top of the scope, but whereas with var the hoisted variables are intiated with undefined , let and const variables remain uninitiated, they exist in a temporal dead zone .
Let’s take a look what happens when we attempt to access a block scoped variable before it is initiated.
So calling theta will output undefined for the locally scoped variable e and throw an error for the block scoped variable f . We cannot use f until after it is initiated, in this case where we set its value as ‘Road Runner’.
There is one other important difference between let and var that we need to mention before moving on. When we use var in the very top level of our code it becomes a global variable and is added to the window object in browsers. With let whilst the variable will become global in the sense that it is block scoped to the entire codebase, it does not become a property of the window object.
I previously mentioned const in passing. This keyword was introduced alongside let as part of ES6. In terms of scope it works the same as let .
In this example a is scoped to the if statement so can be accessed inside the conditional, but is undefined outside of it.
Unlike let , variables defined by const cannot be changed through re-assignment.
However, when working with arrays or objects things are a little different. We still can’t re-assign, so the following will fail:-
But, we can modify a const array or object unless we use Object.freeze() on the variable to make it immutable.
Global + Local Scope
We kind of saw this earlier when looking at hoisting, but let’s take a look at what happens when we redeclare a variable in the local scope that already exists globally.
When we redeclare a global variable in the local scope JavaScript initiates a new local variable. In this example, we have a global variable a , but inside the function iota we create a new local variable a . The new local variable does not modify the global variable, but if we want to access the global value from within the function we’d need to use the global window object.
For me, this code would be much easier to read if we’d used a global namespace for our global variable and rewrite our function to use block scope:-
Local + Block Scope
Hopefully by now the following code should behave as you’d expect.
Code like this isn’t particularly readable, but the block scoped variable is a new variable accessible only within the block it is defined. Modifying the block variable will have no affect on the locally scoped variable outside of the block. The same will happen if we use let to declare all variants of the variable a , so we can rewrite the example like this:-
var, let or const?
I hope that this overview of scope has given you a better understanding of how JavaScript handles variables. Throughout this post I have shown examples using var , let and const statements for declaring variables. With the arrival of ES6 we can now use let and const where we once would have used var and for many this is the approach they are now taking.
Does that mean var is redundant? Well there is no right or wrong answer, but personally I still like to use var for defining global variables at the top-level. However, I use global variables sparingly and like to create a global namespace as discussed earlier for the few cases where these are needed. Otherwise, for any variables whose values will never change I’d use const and for everything else I go for let .
At the end of the day it is up to you how you declare your variables, but hopefully now you have a better comprehension of how they will scope in your code.

Typeerror assignment to constant variable
Doesn’t know how to solve the “Typeerror assignment to constant variable” error in Javascript?
Don’t worry because this article will help you to solve that problem
In this article, we will discuss the Typeerror assignment to constant variable , provide the possible causes of this error, and give solutions to resolve the error.
First, let us know what this error means.
What is Typeerror assignment to constant variable?
“Typeerror assignment to constant variable” is an error message that can occur in JavaScript code.
It means that you have tried to modify the value of a variable that has been declared as a constant.
In JavaScript, when you declare a variable using the const keyword, its value cannot be changed or reassigned.
Attempting to modify a constant variable, you will receive an error stating:
Here is an example code snippet that triggers the error:
In this example, we have declared a constant variable greeting and assigned it the value “Hello” .
When we try to reassign greeting to a different value (“Hi”) , we will get the error:
because we are trying to change the value of a constant variable.
Let us explore more about how this error occurs.
How does Typeerror assignment to constant variable occurs ?
This “ TypeError: Assignment to constant variable ” error occurs when you attempt to modify a variable that has been declared as a constant.
In JavaScript, constants are variables whose values cannot be changed once they have been assigned.
When you declare a variable using the const keyword, you are telling JavaScript that the value of the variable will remain constant throughout the program.
If you try to modify the value of a constant variable, you will get the error:
This error can occur in various situations, such as:
- Attempting to reassign a constant variable:
When you declare a variable using the const keyword, its value cannot be changed.
If you try to reassign the value of a constant variable, you will get this error.
Here is an example :
In this example, we declared a constant variable age and assigned it the value 30 .
When we try to reassign age to a different value ( 35 ), we will get the error:
- Attempting to modify a constant object:
If you declare an object using the const keyword, you can still modify the properties of the object.
However, you cannot reassign the entire object to a new value.
If you try to reassign a constant object, you will get the error.
For example:
In this example, we declared a constant object person with two properties ( name and age ).
We are able to modify the age property of the object without triggering an error.
However, when we try to reassign person to a new object, we will get the Typeerror.
- Using strict mode:
In strict mode, JavaScript throws more errors to help you write better code.
If you try to modify a constant variable in strict mode, you will get the error.
In this example, we declared a constant variable name and assigned it the value John .
However, because we are using strict mode, any attempt to modify the value of name will trigger the error.
Now let’s fix this error.
Typeerror assignment to constant variable – Solutions
Here are the alternative solutions that you can use to fix “Typeerror assignment to constant variable” :
Solution 1: Declare the variable using the let or var keyword:
If you need to modify the value of a variable, you should declare it using the let or var keyword instead of const .
Just like the example below:
Solution 2: Use an object or array instead of a constant variable:
If you need to modify the properties of a variable, you can use an object or array instead of a constant variable.
Solution 3: Declare the variable outside of strict mode:
If you are using strict mode and need to modify a variable, you can declare the variable outside of strict mode:
Solution 4: Use the const keyword and use a different name :
This allows you to keep the original constant variable intact and create a new variable with a different value.
Solution 5: Declare a const variable with the same name in a different scope :
This allows you to create a new constant variable with the same name as the original constant variable.
But with a different value, without modifying the original constant variable.
For Example:
You can create a new constant variable with the same name, without modifying the original constant variable.
By declaring a constant variable with the same name in a different scope.
This can be useful when you need to use the same variable name in multiple scopes without causing conflicts or errors.
So those are the alternative solutions that you can use to fix the TypeError.
By following those solutions, you can fix the “Typeerror assignment to constant variable” error in your JavaScript code.
Here are the other fixed errors that you can visit, you might encounter them in the future.
- typeerror unsupported operand type s for str and int
- typeerror: object of type int64 is not json serializable
- typeerror: bad operand type for unary -: str
In conclusion, in this article, we discussed “Typeerror assignment to constant variable” , provided its causes and give solutions that resolve the error.
By following the given solution, surely you can fix the error quickly and proceed to your coding project again.
I hope this article helps you to solve your problem regarding a Typeerror stating “assignment to constant variable” .
We’re happy to help you.
Happy coding! Have a Good day and God bless.


COMMENTS
Remember, const is appropriate for values that remain constant during execution, while let is more suitable for mutable variables. as an example I am giving an code. const pi = 3.14; // This variable cannot be reassigned a new value // To fix the error, use 'let' if you need to change the value let counter = 0; counter = 1; // This is valid ...
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const. Variables declared using the let keyword can be reassigned. The code for this article is available on GitHub. We used the let keyword to declare the variable in the example. Variables declared using let can be ...
For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable: js. const obj = { foo: "bar" }; obj = { foo: "baz" }; // TypeError: invalid assignment to const `obj'. But you can mutate the properties in a variable:
The const declaration creates an immutable reference to a value. It does not mean the value it holds is immutable — just that the variable identifier cannot be reassigned. For instance, in the case where the content is an object, this means the object's contents (e.g., its properties) can be altered. You should understand const declarations as "create a variable whose identity remains ...
A constant is a value that cannot be altered by the program during normal execution. It cannot change through re-assignment, and it can't be redeclared. In JavaScript, constants are declared using the const keyword. Examples Invalid redeclaration. Assigning a value to the same constant name in the same block-scope will throw. const COLUMNS = 80
In JavaScript, const is used to declare variables that are meant to remain constant and cannot be reassigned. Therefore, if you try to assign a new value to a constant variable, such as: 1 const myConstant = 10; 2 myConstant = 20; // Error: Assignment to constant variable 3. The above code will throw a "TypeError: Assignment to constant ...
Like the let keyword, var variables can be re-declared and re-assigned a value. The difference between var and let is that var variables are executed within the context they were defined. Either a function scope or a global scope. To understand this better, let's take a look at an example.
Constant Objects and Arrays. The keyword const is a little misleading. It does not define a constant value. It defines a constant reference to a value. Because of this you can NOT: Reassign a constant value; Reassign a constant array; Reassign a constant object; But you CAN: Change the elements of constant array; Change the properties of ...
In Node.js, the "TypeError: Assignment to Constant Variable" occurs when there's an attempt to reassign a value to a variable declared with the const keyword. In JavaScript, const is used to declare a variable that cannot be reassigned after its initial assignment.
NodeJS Tutorial; AngularJS Tutorial; ... This JavaScript exception invalid assignment to const occurs if a user tries to change a constant value. Const declarations in JavaScript can not be re-assigned or re-declared. ... invalid assignment to const "x" (Firefox) TypeError: Assignment to constant variable. (Chrome) TypeError: Assignment to ...
Scope A is the (direct) scope of x.; Scopes B and C are inner scopes of scope A.; Scope A is an outer scope of scope B and scope C.; Each variable is accessible in its direct scope and all scopes nested within that scope. The variables declared via const and let are called block-scoped because their scopes are always the innermost surrounding blocks.. 11.4.1 Shadowing variables
To set up a global variable, we need to create it on the global object. The global object is what gives us the scope of the entire project, rather than just the file (module) the variable was created in. In the code block below, we create a global variable called globalString and we give it a value.
Var is properly function-scoped, meaning that the issue doesn't happen in functions, but in blocks like if or for all bets are off and variables declared with var get hoisted to the parent scope. Const. With const you can define immutable variables (constants). Trying to re-assign a constant will raise an error:
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
However, variables declared using the const keyword do not generally have a truly immutable value. Remember, const does not mean "constant", it means one-time assignment. The part that's constant is the reference to an object stored within the constant variable, not the object itself. The following example illustrates the difference:
If you are using NodeJS then the top-level scope is not the same as the global scope. If you use var foobar in a NodeJS module it is local to that module. To define a global variable in NodeJS we need to use the global namespace object, global. global.foobar = 'Hello World!'; // This is a global variable in NodeJS
Could probably do it using eval (which is really the only way to define dynamically named variables in the local scope), but the actual programming problem is likely solved a much better way (properties on an object is the typical way). But, to help you with that more specifically, you'd have to describe the actual programming problem rather than your attempted solution.
You can create a new constant variable with the same name, without modifying the original constant variable. By declaring a constant variable with the same name in a different scope. This can be useful when you need to use the same variable name in multiple scopes without causing conflicts or errors.
So I have this problem right now. So before, my ping command worked, but now it's not, here is the error: (node:739) UnhandledPromiseRejectionWarning: TypeError ...