Home » Variables
Summary : in this tutorial, you will learn about variables including declaring variables, setting their values, and assigning value fields of a record to variables.

What is a variable
A variable is an object that holds a single value of a specific type e.g., integer , date , or varying character string .
We typically use variables in the following cases:
- As a loop counter to count the number of times a loop is performed.
- To hold a value to be tested by a control-of-flow statement such as WHILE .
- To store the value returned by a stored procedure or a function
Declaring a variable
To declare a variable, you use the DECLARE statement. For example, the following statement declares a variable named @model_year :
The DECLARE statement initializes a variable by assigning it a name and a data type. The variable name must start with the @ sign. In this example, the data type of the @model_year variable is SMALLINT .
By default, when a variable is declared, its value is set to NULL .
Between the variable name and data type, you can use the optional AS keyword as follows:
To declare multiple variables, you separate variables by commas:
Assigning a value to a variable
To assign a value to a variable, you use the SET statement. For example, the following statement assigns 2018 to the @model_year variable:
Using variables in a query
The following SELECT statement uses the @model_year variable in the WHERE clause to find the products of a specific model year:
Now, you can put everything together and execute the following code block to get a list of products whose model year is 2018:
Note that to execute the code, you click the Execute button as shown in the following picture:
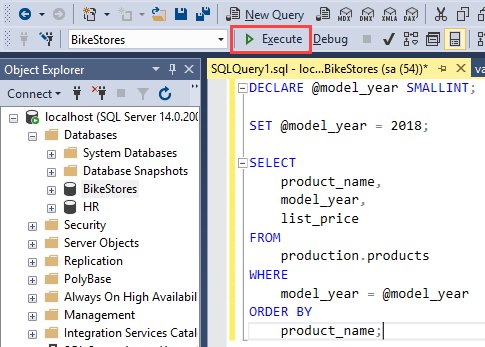
The following picture shows the output:
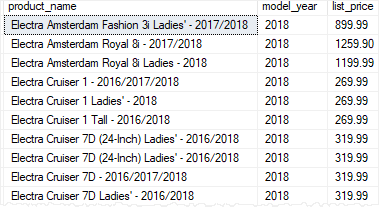
Storing query result in a variable
The following steps describe how to store the query result in a variable:
First, declare a variable named @product_count with the integer data type:
Second, use the SET statement to assign the query’s result set to the variable:
Third, output the content of the @product_count variable:
Or you can use the PRINT statement to print out the content of a variable:
The output in the messages tab is as follows:
To hide the number of rows affected messages, you use the following statement:
Selecting a record into variables
The following steps illustrate how to declare two variables, assign a record to them, and output the contents of the variables:
First, declare variables that hold the product name and list price:
Second, assign the column names to the corresponding variables:
Third, output the content of the variables:

Accumulating values into a variable
The following stored procedure takes one parameter and returns a list of products as a string:
In this stored procedure:
- First, we declared a variable named @product_list with varying character string type and set its value to blank.
- Second, we selected the product name list from the products table based on the input @model_year . In the select list, we accumulated the product names to the @product_list variable. Note that the CHAR(10) returns the line feed character.
- Third, we used the PRINT statement to print out the product list.
The following statement executes the uspGetProductList stored procedure:
The following picture shows the partial output:
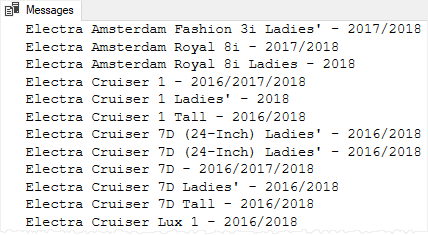
In this tutorial, you have learned about variables including declaring variables, setting their values, and assigning value fields of a record to the variables.
- SQL Server training
- Write for us!

SQL Variables: Basics and usage
In this article, we will learn the notions and usage details of the SQL variable. In SQL Server, local variables are used to store data during the batch execution period. The local variables can be created for different data types and can also be assigned values. Additionally, variable assigned values can be changed during the execution period. The life cycle of the variable starts from the point where it is declared and has to end at the end of the batch. On the other hand, If a variable is being used in a stored procedure, the scope of the variable is limited to the current stored procedure. In the next sections, we will reinforce this theoretical information with various examples
Note: In this article examples, the sample AdventureWorks database is used.
SQL Variable declaration
The following syntax defines how to declare a variable:
Now, let’s interpret the above syntax.
Firstly, if we want to use a variable in SQL Server, we have to declare it. The DECLARE statement is used to declare a variable in SQL Server. In the second step, we have to specify the name of the variable. Local variable names have to start with an at (@) sign because this rule is a syntax necessity. Finally, we defined the data type of the variable. The value argument which is indicated in the syntax is an optional parameter that helps to assign an initial value to a variable during the declaration. On the other hand, we can assign or replace the value of the variable on the next steps of the batch. If we don’t make any initial value assigned to a variable, it is initialized as NULL.
The following example will declare a variable whose name will be @VarValue and the data type will be varchar. At the same time, we will assign an initial value which is ‘Save Our Planet’:
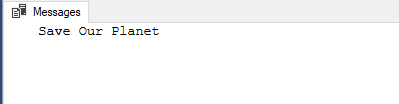
Assigning a value to SQL Variable
SQL Server offers two different methods to assign values into variables except for initial value assignment. The first option is to use the SET statement and the second one is to use the SELECT statement. In the following example, we will declare a variable and then assign a value with the help of the SET statement:
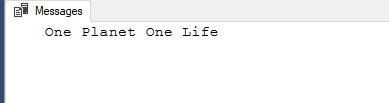
In the following example, we will use the SELECT statement in order to assign a value to a variable:
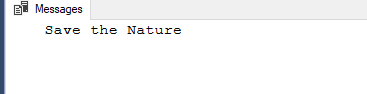
Additionally, the SELECT statement can be used to assign a value to a variable from table, view or scalar-valued functions. Now, we will take a glance at this usage concept through the following example:
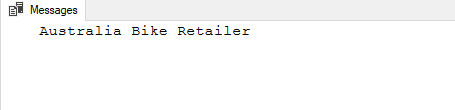
As can be seen, the @PurchaseName value has been assigned from the Vendor table.
Now, we will assign a value to variable from a scalar-valued function:
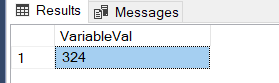
Multiple SQL Variables
For different cases, we may need to declare more than one variable. In fact, we can do this by declaring each variable individually and assigned a value for every parameter:
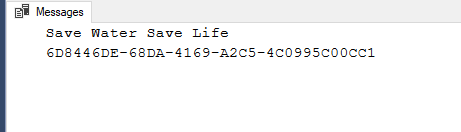
This way is tedious and inconvenient. However, we have a more efficient way to declare multiple variables in one statement. We can use the DECLARE statement in the following form so that we can assign values to these variables in one SELECT statement:
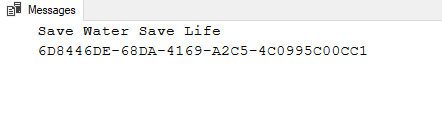
Also, we can use a SELECT statement in order to assign values from tables to multiple variables:
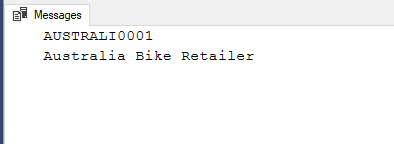
Useful tips about the SQL Variables
Tip 1: As we mentioned before, the local variable scope expires at the end of the batch. Now, we will analyze the following example of this issue:

The above script generated an error because of the GO statement. GO statement determines the end of the batch in SQL Server thus @TestVariable lifecycle ends with GO statement line. The variable which is declared above the GO statement line can not be accessed under the GO statement. However, we can overcome this issue by carrying the variable value with the help of the temporary tables:
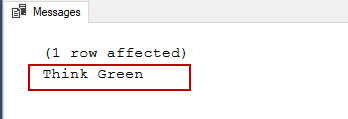
Tip 2: Assume that, we assigned a value from table to a variable and the result set of the SELECT statement returns more than one row. The main issue at this point will be which row value is assigned to the variable. In this circumstance, the assigned value to the variable will be the last row of the resultset. In the following example, the last row of the resultset will be assigned to the variable:
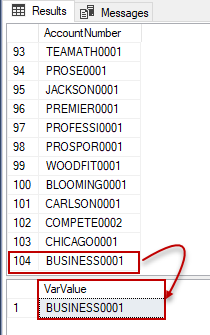
Tip 3: If the variable declared data types and assigned value data types are not matched, SQL Server makes an implicit conversion in the value assignment process, if it is possible. The lower precedence data type is converted to the higher precedence data type by the SQL Server but this operation may lead to data loss. For the following example, we will assign a float value to the variable but this variable data type has declared as an integer:
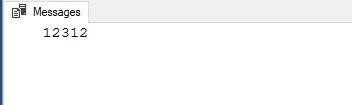
In this article, we have explored the concept of SQL variables from different perspectives, and we also learned how to define a variable and how to assign a value(s) to it.
- Recent Posts

- SQL Performance Tuning tips for newbies - April 15, 2024
- SQL Unit Testing reference guide for beginners - August 11, 2023
- SQL Cheat Sheet for Newbies - February 21, 2023
Related posts:
- SQL Server PRINT and SQL Server RAISERROR statements
- What to choose when assigning values to SQL Server variables: SET vs SELECT T-SQL statements
- SQL varchar data type deep dive
- SQL Convert Function
- What is causing database slowdowns?
The Basics of SQL Server Variables
By: Aubrey Love | Updated: 2022-12-21 | Comments (2) | Related: > TSQL
I see many people using SQL variables in Microsoft SQL Server, but haven't fully grasped the concept with the T-SQL language. In this tutorial, we take a look at declaring and using variables in SQL statements.
Let's start at the beginning with the understanding that SQL variables are not created, but rather, they are declared. Yes, there is a difference. To create means that you have created an object in SQL Server that will remain after you close your SQL instance. When you declare a variable in SQL Server, it's a temporary object and will only be usable during the script execution. SQL variables don't exist as an entity but as an object value inside the script.
SQL Variable Declaration
As mentioned earlier, we "declare" variables in SQL Server and assign them a datatype and value in a SQL query. We can later use that value to return a result set. Hence, we actually use the word "DECLARE" when setting a SQL variable and must use an @ symbol preceding the variable name.
The basic syntax to declare a variable, the data type and the variable value is as follows:
We can also declare a variable first and use a set statement to define its value later:
An excellent point to remember is that variables are just as they sound, something that varies. This means that you can change the value of a variable at any time. However, you cannot change the datatype or name of a variable.
We will create a variable in the code sample below, set its value to 12 and run a SELECT script calling that variable. Next, we will re-assign the value of that variable and again run a SELECT script to see the difference.
Query results:
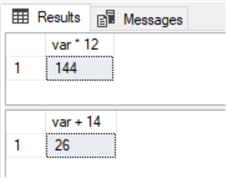
Assigning Values to a SQL Variable
Transact-SQL has two options are available to assign a value to a variable: set and select. We used the SET command in the previous section to assign a value. This section will use the SELECT statement to assign our values. We can select a string value or pull a value from a table to use as the value of our variables.
In this sample, we assign a string value to a variable that we will call "testvalue1":

In the following example, we are using a value in the "FirstName" column of the Person.Person table in the AdventureWorks2019 sample database. You can get a free copy of the sample database here .
In our sample code, we treat it like any other SELECT statement, but our desired value is being called by referencing the value of our variable. In other words, we assign our variable the first name in the Person.Person table that has a BusinessEntityID of 8.

You can also declare multiple variables at the same time. Below, we will expand on the previous sample code by adding the "LastName" column value from our Person.Person table.
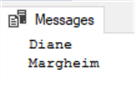
We can also assign a value to a SQL variable from a scalar-valued function.

It's important to remember that variables are only available during a query execution. If a batch is terminated with the "GO" batch separator, then you can no longer reference the variable.
Example: Referring to our original sample, if we terminate the batch and then try to print the value of our variable, we will get an error.

As you can see from the result set above, the print statement inside the batch ran successfully, but the print statement outside the batch did not. That's because the variable no longer exists once the batch has been terminated.
DECLARE, DECLARE, DECLARE. Can I clean up the code if I need to declare more than one variable in a batch? Yes, there is. When declaring variables and setting their values, you can comma separate them without having to state DECLARE or SET for each variable.
In the sample below, we will declare two variables with one DECLARE statement, set the two values with one SELECT statement, and print both values with one PRINT statement.
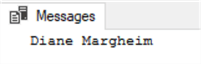
Wrapping Up
In this tutorial, we looked at some of the fundamentals of SQL variables. These are the basic building blocks that every DBA and database developer needs to start with when learning about SQL variables. We learned what a variable is and is not, how to create one or more variables, and how to use those variables to our advantage.
Click the links below to continue reading about SQL variables:
- SQL Server INSERT command with variables
SQL Variables in Scripts, Functions, Stored Procedures, SQLCMD and More
- SQL Variables for Queries and Stored Procedures in SQL Server, Oracle and PostgreSQL
- Some Tricky Situations When Working with SQL Server NULL
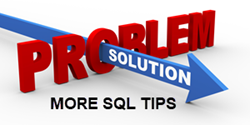
About the author
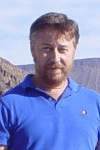
Comments For This Article
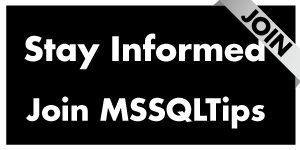
Related Content
SQL Declare Variable to Define and Use Variables in SQL Server code
How to use @@ROWCOUNT in SQL Server
When to use SET vs SELECT when assigning values to variables in SQL Server
Using SQL Variables in SQL Server Code and Queries
Nullability settings with select into and variables
SQL Server 2008 Inline variable initialization and Compound assignment
Related Categories
SQL Reference Guide
Change Data Capture
Common Table Expressions
Dynamic SQL
Error Handling
Stored Procedures
Transactions
Development
Date Functions
System Functions
JOIN Tables
SQL Server Management Studio
Database Administration
Performance
Performance Tuning
Locking and Blocking
Data Analytics \ ETL
Microsoft Fabric
Azure Data Factory
Integration Services
Popular Articles
Date and Time Conversions Using SQL Server
Format SQL Server Dates with FORMAT Function
SQL Server CROSS APPLY and OUTER APPLY
SQL Server Cursor Example
SQL CASE Statement in Where Clause to Filter Based on a Condition or Expression
DROP TABLE IF EXISTS Examples for SQL Server
SQL Convert Date to YYYYMMDD
Rolling up multiple rows into a single row and column for SQL Server data
SQL NOT IN Operator
Resolving could not open a connection to SQL Server errors
Format numbers in SQL Server
SQL Server PIVOT and UNPIVOT Examples
Script to retrieve SQL Server database backup history and no backups
How to install SQL Server 2022 step by step
An Introduction to SQL Triggers
Using MERGE in SQL Server to insert, update and delete at the same time
How to monitor backup and restore progress in SQL Server
List SQL Server Login and User Permissions with fn_my_permissions
SQL Server Loop through Table Rows without Cursor
SQL Server Database Stuck in Restoring State
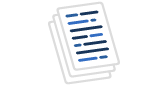
Combining Silver Tables into a Model – Data Engineering with Fabric
John Miner , 2024-05-15
Data Presentation Layer
Microsoft Fabric allows the developer to create delta tables in the lake house. The bronze tables contain multiple versions of the truth, and the silver tables are a single version of the truth. How can we combine the silver tables into a model for consumption from the gold layer?
Business Problem
Our manager at Adventure Works has asked us to use a metadata-driven solution to ingest CSV files from external storage into Microsoft Fabric. A typical medallion architecture will be used in the design. The final goal of the project is to have end users access the gold layer tables using their favorite SQL tool.
Technical Solution
Microsoft Fabric supports two data warehouse components : SQL Analytics Endpoint and Synapse Data Warehouse. The image below shows that the endpoint is read-only while the warehouse supports both read and write operations. It makes sense to use the SQL Analytics Endpoint since we do not have to write to the tables.

The following topics will be covered in this article (thread).
- create new lake house
- create + populate metadata table
- import + update child pipeline
- create + execute parent pipeline
- review bronze + silver tables
- use warehouse view to combine tables
- use warehouse view to aggregate table
- connect to warehouse via SSMS
Architectural Overview
The architectural diagram for the metadata driven design is shown below. This is the child pipeline that I have been talking about. Please remember that the data pipeline uses a full refresh pattern. The bronze tables will contain all processed files and the silver tables will contained the most recent file (data).

The parent pipeline will call the child pipeline for each meta data entry. At the end of a successful execution, the lake house has been created for all saleslt tables for both the bronze and silver zones. We will be working on the creating parent pipeline in this article.

The above diagram shows how the presentation layer works. By default, all tables in the lake house (spark engine) are available to the SQL Analytics Endpoint as read only. To date, spark views are not supported. Do not fret. We can combine and aggregate data using SQL views in the data warehouse. After completing the warehouse or gold layer, we will retrieve data using my favorite tool (SQL Server Management Studio).
Meta Data Tables
A meta data driven design allows the developer to automate the copying of data into the lake (raw files) as well as building the bronze and silver tables. The image below shows the meta data table viewed from the Lake House explorer. Please note, only the meta data table exists currently, and the local raw folder is empty.

Let us review the meta data table right now with the following fields.
- pipeline id – just an identifier
- container name – same path in source and destination storage
- file name – same file in source and destination storage
- header flag – does the data file have a header line
- delimiter string – how is the tuple divided into columns
- table name – the root name of the table
- schema string – infer schema or use this definition
Each piece of information in the delta table is used by either the pipeline activities or spark notebooks to dictate which action to perform. Of course, there is a one-to-one mapping from source data files to rows of data in the meta data table. Please see the folder listing of the source storage account as shown below.

Please look at the nb-create-meta-data notebook for more details on how to reload the meta data table from scratch. Just download the zip file at the end of the article and find the file under the code folder.
Parent Pipeline
If you did not notice, I created a new lake house, named lh_adv_wrks. Since this task is so easy, I did not include any instructions. See the MS Learn webpage for more details.
The data pipeline, named pl-refresh-advwrks, is the parent pipeline we are building out today. It calls the child pipeline, named pl-delimited-full-load, with the pipeline id ranging from 1 to 11.

To write a while loop, we usually need two variables in a programming language like Python. The first variable is a counter and the second is the dynamic limit.
Data Factory does not allowed the developer to set a variable to itself. It is just a limitation of tool. The image below shows the error when trying to set the variable, named var_cnt, to itself plus one. The solution to this problem is to use another variable.

The image below shows the test condition for the until loop .

The variable, named var_cur, is used as a temporary variable since we cannot use self-assignment. Thus, we set the current value equal to the counter plus one.

The last step of the process is to set the counter to the current value. See the assignment of the var_cnt variable below.

After successful execution of the parent pipeline, the lake house will contain refreshed data.
System Review
My workspace is where all the personal objects in Microsoft Fabric are listed.

The table below shows the objects that are part of the Adventure Works lake house solution.
- nb-create-meta-data – this delta table describes how to load the system
- nb-delimited-full-load – read raw files, refresh bronze + silver tables
- nb-test-schema – this Spark SQL code tests the schema used in meta data table
- pl-delimited-full-load – copy file from source to raw, execute spark notebook
- pl-refresh-advwrks – call the full load pipeline for all source files/li>
- SQL analytics endpoint – external programs can interact with lake via TDS
- semantic model – the default Power BI data model
The One Lake is where all the action happens. If we right click on the lake house object and select open, the lake house explorer will be shown. The image below shows the versioning of data files in the raw zone for the adventure works dataset. Each time we execute the pipeline, a CSV file is copied to a sub-folder that has today’s date in sortable format.

The image below shows a bronze and silver table for each of the source files. There are a total of twenty-three delta tables shown by the explorer.

In the next section, we will be using the SQL analytics endpoint to create views for our presentation layer.
Data Warehouse
Make sure the key word warehouses is at the top of the explorer. In the lake house explorer, we have only tables and files. In the warehouse explorer, we can create other objects such as views, functions, and stored procedures. Today, we are focusing on views for our presentation layer.

The first view we are going to create is named silver_dim_products. The SQL code below creates the view that combines the three product related tables into one dataset. It is a coin toss on what prefix to use on the table. I chose to use silver since we did not perform any calculations.
The output from previewing the SQL View is show below.

The view, named gold_report_data, combines data from six tables into one flattened dataset ready for reporting. See the code below for details. This table is truly a gold layer object.
The image below shows sample data from the table.

The last step is to use this flattened data in the gold view to figure out what sold best in December 2010 in North America. The bike model named “Road-150” was the top seller.

The SQL query seen below was used to generate the previous result set.
What I did not cover is the fact that any SQL scripts that are created by hand or by right click are stored in the section called My Queries. These queries can be pulled up and re-executed any time you want. Just like a SQL database, an object must be dropped before being created again.
Using the SQL endpoint
We need to retrieve the fully qualified name of the SQL analytics endpoint. Use the copy icon to retrieve this long string.

Fabric only supports Microsoft Entra, formally known as Azure Active Directory with multi-factory authentication (MFA). The image below shows the most recent SQL Server Management Studio making a connection to the SQL endpoint. The user named [email protected] is signing into the service.

The login action from SSMS will open a web browser to allow the end user to enter credentials. Since I work with many different accounts, I must choose the correct username.

The authenticator application is used to verify that the correct user is signing into the system.

Once authentication is complete, we can close the web browser window.

The last step is to make sure the result set that was created in our Fabric Data Warehouse matches the result set retrieved by SSMS using the SQL end point.

The real power of the One Lake is the ability to mesh data sets from a variety of locations into one place. The SQL analytics end point allows users to leverage existing reports and/or developer tools to look at the data in the warehouse.
One thing I really like about Microsoft Fabric is the fact that all the services are in one place. Today, we covered the parent/child pipeline design. The parent pipeline retrieves the list of meta data from the delta table. For each row, data is copied from the source location to the raw layer. Additionally, a bronze table showing history is created as well as a silver table showing the most recent data. This is a full refresh load pattern with history for auditing.
Once the data is in the lake house, we can use the data warehouse section of Fabric to create views that combine tables, aggregate rows and calculate values. This data engineering is used to clean the data for reporting or machine learning.
Finally, there are two ways to use the data. The semantic model is used by Power BI reports that a built into Fabric. The SQL analytics endpoint is used for external reporting and/or developer tools to access the data. The steps to access the warehouse are pretty like any relational database management system that we might access.
Next time, I will be talking about how end users can update files in the Lakehouse using the one lake explorer. Enclosed is the zip file with the CSV data files, Data Pipelines in JSON format, Spark notebooks and SQL notebooks.
Log in or register to rate
You rated this post out of 5. Change rating
- Uncategorized
- Adventure Works Schema
- Apache Spark
- data engineering
- Data Factory
- Data Warehouses
- External SQL Tools
- Full Load Code
- John F. Miner III
- Meta Data Driven Design
- Microsoft Fabric
- SQL Analyhtics Endpoint
Join the discussion and add your comment
Related content
Metadata driven pipelines – data engineering with fabric.
- by John Miner
- SQLServerCentral
- Microsoft Fabric (Azure Synapse, Data Engineering, etc.)
This article explains metadata driven pipelines and shows an example in Microsoft Fabric.
2,039 reads

Full vs. Incremental Loads – Data Engineering with Fabric
Learn how to perform full and incremental loads in Fabric with a little SparkSQL.
3,327 reads
Managing Files and Folders with Python – Data Engineering with Fabric
In this article, learn how you can manage files and folders for both full and incremental loading situations.
2,358 reads
Managed Vs Unmanaged Tables – Data Engineering with Fabric
Learn how to get started with Microsoft Fabric along with the differences between managed and unmanaged tables.
2,656 reads
Evolving Role of Data Professionals in Artificial Intelligence Era
- by Amit Rai
Generative Al tools like Gemini and GPT promise to automate and augment knowledge-based work. Data professionals must adapt to this transformation by acquiring new skills and playing a central role in their organization's AI-driven future. Data preparation, curation, ethical sourcing and labeling, and collecting user feedback become crucial as high-quality data is essential for effective LLM based application.
2,413 reads
TechRepublic
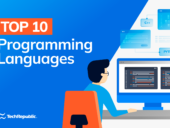
TIOBE Index for May 2024: Top 10 Most Popular Programming Languages
Fortran is in the spotlight again in part due to the increased interest in artificial intelligence.
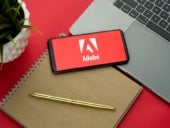
Adobe Adds Firefly and Content Credentials to Bug Bounty Program
Security researchers can earn up to $10,000 for critical vulnerabilities in the generative AI products.
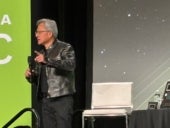
NVIDIA GTC 2024: CEO Jensen Huang’s Predictions About Prompt Engineering
"The job of the computer is to not require C++ to be useful," said Huang at NVIDIA GTC 2024.
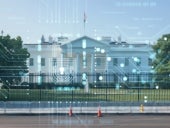
White House Recommends Memory-Safe Programming Languages and Security-by-Design
A new report promotes preventing cyberattacks by using memory-safe languages and the development of software safety standards.
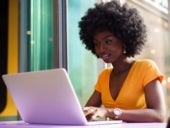
How to Hire a Python Developer
Spend less time researching and more time recruiting the ideal Python developer. Find out how in this article.
Latest Articles
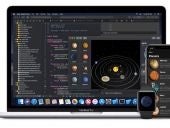
The Apple Developer Program: What Professionals Need to Know
If you want to develop software for macOS, iOS, tvOS, watchOS or visionOS, read this overview of Apple's Developer Program.
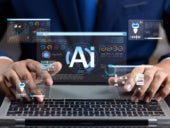
U.K.’s AI Safety Institute Launches Open-Source Testing Platform
Inspect is the first AI safety testing platform created by a state-backed body to be made freely available to the global AI community.
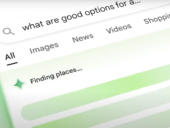
Google I/O 2024: Google Search’s AI Overviews Are Generally Available This Week
Plus, Google reveals plans to unleash Gemini across Workspace to make interpreting long email threads or creating spreadsheets easier.
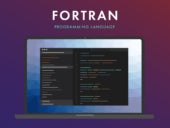
TIOBE Index News (May 2024): Why is Fortran Popular Again?
The AI boom is starting to show up on the TIOBE Index by bringing back a formative programming language.
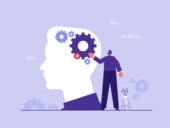
Udemy Report: Which IT Skills Are Most in Demand in Q1 2024?
Informatica PowerCenter, Microsoft Playwright and Oracle Database SQL top Udemy’s list of most popular tech courses.
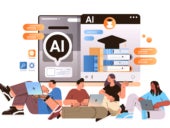
The 10 Best AI Courses in 2024
Today’s options for best AI courses offer a wide variety of hands-on experience with generative AI, machine learning and AI algorithms.
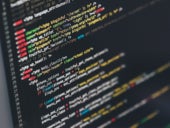
Learn Windows PowerShell for just $17
Streamline your workflow, automate tasks and more with The 2024 Windows PowerShell Certification Bundle.
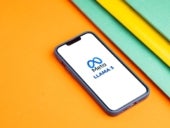
Llama 3 Cheat Sheet: A Complete Guide for 2024
Learn how to access Meta’s new AI model Llama 3, which sets itself apart by being open to use under a license agreement.
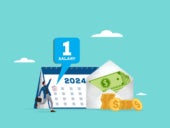
How Are APAC Tech Salaries Faring in 2024?
The year 2024 is bringing a return to stable tech salary growth in APAC, with AI and data jobs leading the way. This follows downward salary pressure in 2023, after steep increases in previous years.
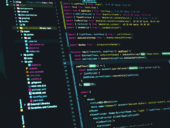
Learn Python for Just $16 Through 5/5
One of our best-selling Python bundles is discounted even further to just $15.97 through May 5. Now's the time to learn the popular programming language.
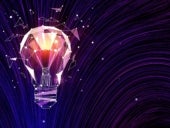
TechRepublic Premium Editorial Calendar: Policies, Checklists, Hiring Kits and Glossaries for Download
TechRepublic Premium content helps you solve your toughest IT issues and jump-start your career or next project.
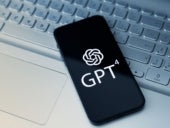
OpenAI’s GPT-4 Can Autonomously Exploit 87% of One-Day Vulnerabilities, Study Finds
Researchers from the University of Illinois Urbana-Champaign found that OpenAI’s GPT-4 is able to exploit 87% of a list of vulnerabilities when provided with their NIST descriptions.
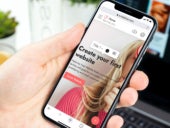
Create Easy, Professional Websites with Mobirise – Now Just $80 Through 4/21
Most user-friendly website builders create basic, non-professional or ineffective websites. Not anymore! With Mobirise, new users can get a year of easily built beautiful sites. Get a year’s access for only $79.97 through April 21.
Create a TechRepublic Account
Get the web's best business technology news, tutorials, reviews, trends, and analysis—in your inbox. Let's start with the basics.
* - indicates required fields
Sign in to TechRepublic
Lost your password? Request a new password
Reset Password
Please enter your email adress. You will receive an email message with instructions on how to reset your password.
Check your email for a password reset link. If you didn't receive an email don't forgot to check your spam folder, otherwise contact support .
Welcome. Tell us a little bit about you.
This will help us provide you with customized content.
Want to receive more TechRepublic news?
You're all set.
Thanks for signing up! Keep an eye out for a confirmation email from our team. To ensure any newsletters you subscribed to hit your inbox, make sure to add [email protected] to your contacts list.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
= (Assignment Operator) (Transact-SQL)
- 11 contributors
The equal sign (=) is the only Transact-SQL assignment operator. In the following example, the @MyCounter variable is created, and then the assignment operator sets @MyCounter to a value returned by an expression.
The assignment operator can also be used to establish the relationship between a column heading and the expression that defines the values for the column. The following example displays the column headings FirstColumnHeading and SecondColumnHeading . The string xyz is displayed in the FirstColumnHeading column heading for all rows. Then, each product ID from the Product table is listed in the SecondColumnHeading column heading.
Operators (Transact-SQL) Compound Operators (Transact-SQL) Expressions (Transact-SQL)
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources

IMAGES
VIDEO
COMMENTS
SQL Server 2005 actually allows us to parameterize the TOP clause, using a variable, expression or statement. So you can do things like: SELECT TOP (@foo) a FROM table ORDER BY a. SELECT TOP (SELECT COUNT(*) FROM somewhere else) a FROM table ORDER BY a. SELECT TOP (@foo + 5 * 4 / 2) a FROM table ORDER BY a. Source.
The syntax "select top (@var) ..." only works in SQL SERVER 2005+. For SQL 2000, you can do: set rowcount @top. select * from sometable. set rowcount 0. Hope this helps. Oisin. (edited to replace @@rowcount with rowcount - thanks augustlights)
Set a value in a Transact-SQL variable. When a variable is first declared, its value is set to NULL. To assign a value to a variable, use the SET statement. This is the preferred method of assigning a value to a variable. A variable can also have a value assigned by being referenced in the select list of a SELECT statement.
Limits the rows returned in a query result set to a specified number of rows or percentage of rows in SQL Server. When you use TOP with the ORDER BY clause, the result set is limited to the first N number of ordered rows. Otherwise, TOP returns the first N number of rows in an undefined order. Use this clause to specify the number of rows ...
SQL Server provides us with two methods in T-SQL to assign a value to a previously created local SQL variable. The first method is the SET statement, the ANSI standard statement that is commonly used for variable value assignment. The second statement is the SELECT statement. In addition to its main usage to form the logic that is used to ...
Returning values through a query. Whenever you are assigning a query returned value to a variable, SET will accept and assign a scalar (single) value from a query. While SELECT could accept multiple returned values. But after accepting multiple values through a SELECT command you have no way to track which value is present in the variable.
SELECT @ local_variable is typically used to return a single value into the variable. However, when expression is the name of a column, it can return multiple values. If the SELECT statement returns more than one value, the variable is assigned the last value that is returned. If the SELECT statement returns no rows, the variable retains its ...
I am building a report on server hardware projections, and I'm getting stuck here. I have a query that correctly returns the number of HDD's installed on the server, and am storing that number into a variable called @OtherHDDs, and it is an INT.. I need to now do this...
It is helpful to manipulate data within a stored procedure, function, or batch of SQL statements. The variable is beneficial to keep the temporary data for the query batch. You can assign a static value or define dynamic values using SQL query. Declaring a Single SQL Variable. The T-SQL syntax for declaring a variable in SQL Server is as follows:
The SQL variable syntax above requires the following: @local_variable: Provide a variable name, which must start with an "@" sign.; data_type: Define the data type (int, char, varchar, decimal, numeric, datetime, etc.) for the declared variable.You cannot assign the data types to be a "text", "ntext", or "image" types.= value: This is optional, as you can set a variable value in another way.
SELECT TOP into variable Forum - Learn more on SQLServerCentral ... Microsoft Certified Master: SQL Server, MVP, M.Sc (Comp Sci) ... The TOP can go to the right of the assignment too. like.
Storing query result in a variable. The following steps describe how to store the query result in a variable: First, declare a variable named @product_count with the integer data type:. DECLARE @product_count INT; Code language: SQL (Structured Query Language) (sql). Second, use the SET statement to assign the query's result set to the variable:. SET @product_count = ( SELECT COUNT (*) FROM ...
Tip 3: If the variable declared data types and assigned value data types are not matched, SQL Server makes an implicit conversion in the value assignment process, if it is possible.The lower precedence data type is converted to the higher precedence data type by the SQL Server but this operation may lead to data loss.
SQL Server SELECT @VARIABLE = TOP 1. Ask Question Asked 8 years ago. Modified 8 years ago. Viewed 14k times ... If you want to use your actual variable for the TOP statement (i.e. retrieve the top @TICKET_AGE rows), then you would could use : SELECT TOP @TICKET_AGE DATEDIFF(second, DATE_ENTERED, GETDATE()) / 60 FROM TICKETS ...
The SET statement that assigns a value to the variable returns a single value. When you initialize multiple variables, use a separate SET statement for each local variable. You can use variables only in expressions, not instead of object names or keywords. To construct dynamic Transact-SQL statements, use EXECUTE.
To ASSIGN variables using a SQL select the best practice is as shown below->DECLARE co_id INT ; ->DECLARE sname VARCHAR(10) ; ->SELECT course_id INTO co_id FROM course_details ; ->SELECT student_name INTO sname FROM course_details; IF you have to assign more than one variable in a single line you can use this same SELECT INTO
SQL Variable Declaration. As mentioned earlier, we "declare" variables in SQL Server and assign them a datatype and value in a SQL query. We can later use that value to return a result set. Hence, we actually use the word "DECLARE" when setting a SQL variable and must use an @ symbol preceding the variable name.
See the assignment of the var_cnt variable below. ... Make sure the key word warehouses is at the top of the explorer. ... The image below shows the most recent SQL Server Management Studio making ...
Developer TR Academy Create Easy, Professional Websites with Mobirise - Now Just $80 Through 4/21 . Most user-friendly website builders create basic, non-professional or ineffective websites.
A cursor variable: Can be the target of either a cursor type or another cursor variable. For more information, see SET @local_variable (Transact-SQL). Can be referenced as the target of an output cursor parameter in an EXECUTE statement if the cursor variable doesn't have a cursor currently assigned to it.
SET is the command for setting options (SET ANSI_QUOTES ON, SET IDENTITY_INSERT MyTable OFF). SELECT is the command for all queries, and queries are more than capable of assigning values to variables (if there is only one row). You could use SET @myvar = (SELECT TOP 1 MyCol FROM MyTable), but what's the point when you could also do SELECT TOP 1 ...
In this article. Applies to: SQL Server Azure SQL Database Azure SQL Managed Instance Azure Synapse Analytics Analytics Platform System (PDW) SQL analytics endpoint in Microsoft Fabric Warehouse in Microsoft Fabric The equal sign (=) is the only Transact-SQL assignment operator. In the following example, the @MyCounter variable is created, and then the assignment operator sets @MyCounter to a ...