Sequential statement | ---- used in ----> | Process Procedure |
Syntax |
Rules and Examples |
A sequential signal assignment takes effect only when the process suspends. If there is more than one assignment to the same signal before suspension, the last one executed takes effect: | |
An equivalent process: |
If a signal which has assignments to it within a process is also in the sensitivity list, it may cause the process to be reactivated: In this architecture, the signals Y and Z will both get the same value (2*A + B) because even though two assignments to the signal M are executed, the first will always be superceded by the second |
A sequential signal assignment may have a delay: | |
The rules about what happpens when a delayed signal assignment is subsequently overridden are complex: see the LRM section 8.3.1 or "A Primer" by Jayaram Bhasker, section 4.14 | A delayed sequential signal assignment does suspend the process or procedure for the time specified. The assignment is to occur after the specified time, and any further sequential statements are executed immediately |
Synthesis Issues |
Sequential signal assignments are generally synthesisable, providing they use types and operators acceptable to the synthesis tool. Delays are usually ignored. All signals with assignments to them within a "clocked process" will become the outputs of registers in the synthesised design. Signals driven by a "combinational process" will be inferred as the outputs of combinational logic a signal which is assigned only under certain conditions may infer a latch. Assignment to 'Z' will normally generate tri-state drivers. assignment to 'X' may not be supported. |
Whats New in '93 |
In VHDL -93, any signal assignment statement may have an optional label:

Sequential Signal Assignment
Reference manual.
- Section 8.3
Rules and Examples
A sequential signal assignment takes effect only when the process suspends. If there is more than one assignment to the same signal before suspension, the last one executed takes effect:
An equivalent process:
In this architecture, the signals Y and Z will both get the same value (2*A + B) because even though two assignments to the signal M are executed, the first will always be superseded by the second
If a signal which has assignments to it within a process is also in the sensitivity list, it may cause the process to be reactivated.
A sequential signal assignment may have a delay:
The rules about what happens when a delayed signal assignment is subsequently overridden are complex: see the reference manual.
A delayed sequential signal assignment does not suspend the process or procedure for the time specified. The assignment is scheduled to occur after the specified time, and any further sequential statements are executed immediately.
Any signal assignment statement may have an optional label:
A delayed signal assignment with inertial delay may be explicitly preceded by the keyword inertial . It may also have a reject time specified. This is the minimum “pulse width” to be propagated, if different from the inertial delay:
Synthesis Issues
Sequential signal assignments are generally synthesizable, providing they use types and operators acceptable to the synthesis tool. Delays are usually ignored.
All signals with assignments to them within a “clocked process” will become the outputs of registers in the synthesized design.
Signals driven by a “combinational process” will be inferred as the outputs of combinational logic but a signal which is assigned only under certain conditions may infer a latch. Assignment to ‘Z’ will normally generate tri-state drivers. assignment to ‘X’ may not be supported.
VHDL Logical Operators and Signal Assignments for Combinational Logic
In this post, we discuss the VHDL logical operators, when-else statements , with-select statements and instantiation . These basic techniques allow us to model simple digital circuits.
In a previous post in this series, we looked at the way we use the VHDL entity, architecture and library keywords. These are important concepts which provide structure to our code and allow us to define the inputs and outputs of a component.
However, we can't do anything more than define inputs and outputs using this technique. In order to model digital circuits in VHDL, we need to take a closer look at the syntax of the language.
There are two main classes of digital circuit we can model in VHDL – combinational and sequential .
Combinational logic is the simplest of the two, consisting primarily of basic logic gates , such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
Sequential circuits use a clock and require storage elements such as flip flops . As a result, changes in the output are synchronised to the circuit clock and are not immediate. We talk more specifically about modelling combinational logic in this post, whilst sequential logic is discussed in the next post.
Combinational Logic
The simplest elements to model in VHDL are the basic logic gates – AND, OR, NOR, NAND, NOT and XOR.
Each of these type of gates has a corresponding operator which implements their functionality. Collectively, these are known as logical operators in VHDL.
To demonstrate this concept, let us consider a simple two input AND gate such as that shown below.
The VHDL code shown below uses one of the logical operators to implement this basic circuit.
Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals. This is roughly equivalent to the = operator in most other programming languages.
In addition to signals, we can also define variables which we use inside of processes. In this case, we would have to use a different assignment operator (:=).
It is not important to understand variables in any detail to model combinational logic but we talk about them in the post on the VHDL process block .
The type of signal used is another important consideration. We talked about the most basic and common VHDL data types in a previous post.
As they represent some quantity or number, types such as real, time or integer are known as scalar types. We can't use the VHDL logical operators with these types and we most commonly use them with std_logic or std_logic_vectors.
Despite these considerations, this code example demonstrates how simple it is to model basic logic gates.
We can change the functionality of this circuit by replacing the AND operator with one of the other VHDL logical operators.
As an example, the VHDL code below models a three input XOR gate.
The NOT operator is slightly different to the other VHDL logical operators as it only has one input. The code snippet below shows the basic syntax for a NOT gate.
- Mixing VHDL Logical Operators
Combinational logic circuits almost always feature more than one type of gate. As a result of this, VHDL allows us to mix logical operators in order to create models of more complex circuits.
To demonstrate this concept, let’s consider a circuit featuring an AND gate and an OR gate. The circuit diagram below shows this circuit.
The code below shows the implementation of this circuit using VHDL.
This code should be easy to understand as it makes use of the logical operators we have already talked about. However, it is important to use brackets when modelling circuits with multiple logic gates, as shown in the above example. Not only does this ensure that the design works as intended, it also makes the intention of the code easier to understand.
- Reduction Functions
We can also use the logical operators on vector types in order to reduce them to a single bit. This is a useful feature as we can determine when all the bits in a vector are either 1 or 0.
We commonly do this for counters where we may want to know when the count reaches its maximum or minimum value.
The logical reduction functions were only introduced in VHDL-2008. Therefore, we can not use the logical operators to reduce vector types to a single bit when working with earlier standards.
The code snippet below shows the most common use cases for the VHDL reduction functions.
Mulitplexors in VHDL
In addition to logic gates, we often use multiplexors (mux for short) in combinational digital circuits. In VHDL, there are two different concurrent statements which we can use to model a mux.
The VHDL with select statement, also commonly referred to as selected signal assignment, is one of these constructs.
The other method we can use to concurrently model a mux is the VHDL when else statement.
In addition to this, we can also use a case statement to model a mux in VHDL . However, we talk about this in more detail in a later post as this method also requires us to have an understanding of the VHDL process block .
Let's look at the VHDL concurrent statements we can use to model a mux in more detail.
VHDL With Select Statement
When we use the with select statement in a VHDL design, we can assign different values to a signal based on the value of some other signal in our design.
The with select statement is probably the most intuitive way of modelling a mux in VHDL.
The code snippet below shows the basic syntax for the with select statement in VHDL.
When we use the VHDL with select statement, the <mux_out> field is assigned data based on the value of the <address> field.
When the <address> field is equal to <address1> then the <mux_out> signal is assigned to <a>, for example.
We use the the others clause at the end of the statement to capture instance when the address is a value other than those explicitly listed.
We can exclude the others clause if we explicitly list all of the possible input combinations.
- With Select Mux Example
Let’s consider a simple four to one multiplexer to give a practical example of the with select statement. The output Q is set to one of the four inputs (A,B, C or D) depending on the value of the addr input signal.
The circuit diagram below shows this circuit.
This circuit is simple to implement using the VHDL with select statement, as shown in the code snippet below.
VHDL When Else Statements
We use the when statement in VHDL to assign different values to a signal based on boolean expressions .
In this case, we actually write a different expression for each of the values which could be assigned to a signal. When one of these conditions evaluates as true, the signal is assigned the value associated with this condition.
The code snippet below shows the basic syntax for the VHDL when else statement.
When we use the when else statement in VHDL, the boolean expression is written after the when keyword. If this condition evaluates as true, then the <mux_out> field is assigned to the value stated before the relevant when keyword.
For example, if the <address> field in the above example is equal to <address1> then the value of <a> is assigned to <mux_out>.
When this condition evaluates as false, the next condition in the sequence is evaluated.
We use the else keyword to separate the different conditions and assignments in our code.
The final else statement captures the instances when the address is a value other than those explicitly listed. We only use this if we haven't explicitly listed all possible combinations of the <address> field.
- When Else Mux Example
Let’s consider the simple four to one multiplexer again in order to give a practical example of the when else statement in VHDL. The output Q is set to one of the four inputs (A,B, C or D) based on the value of the addr signal. This is exactly the same as the previous example we used for the with select statement.
The VHDL code shown below implements this circuit using the when else statement.
- Comparison of Mux Modelling Techniques in VHDL
When we write VHDL code, the with select and when else statements perform the same function. In addition, we will get the same synthesis results from both statements in almost all cases.
In a purely technical sense, there is no major advantage to using one over the other. The choice of which one to use is often a purely stylistic choice.
When we use the with select statement, we can only use a single signal to determine which data will get assigned.
This is in contrast to the when else statements which can also include logical descriptors.
This means we can often write more succinct VHDL code by using the when else statement. This is especially true when we need to use a logic circuit to drive the address bits.
Let's consider the circuit shown below as an example.
To model this using a using a with select statement in VHDL, we would need to write code which specifically models the AND gate.
We must then include the output of this code in the with select statement which models the multiplexer.
The code snippet below shows this implementation.
Although this code would function as needed, using a when else statement would give us more succinct code. Whilst this will have no impact on the way the device works, it is good practice to write clear code. This help to make the design more maintainable for anyone who has to modify it in the future.
The VHDL code snippet below shows the same circuit implemented with a when else statement.
Instantiating Components in VHDL
Up until this point, we have shown how we can use the VHDL language to describe the behavior of circuits.
However, we can also connect a number of previously defined VHDL entity architecture pairs in order to build a more complex circuit.
This is similar to connecting electronic components in a physical circuit.
There are two methods we can use for this in VHDL – component instantiation and direct entity instantiation .
- VHDL Component Instantiation
When using component instantiation in VHDL, we must define a component before it is used.
We can either do this before the main code, in the same way we would declare a signal, or in a separate package.
VHDL packages are similar to headers or libraries in other programming languages and we discuss these in a later post.
When writing VHDL, we declare a component using the syntax shown below. The component name and the ports must match the names in the original entity.
After declaring our component, we can instantiate it within an architecture using the syntax shown below. The <instance_name> must be unique for every instantiation within an architecture.
In VHDL, we use a port map to connect the ports of our component to signals in our architecture.
The signals which we use in our VHDL port map, such as <signal_name1> in the example above, must be declared before they can be used.
As VHDL is a strongly typed language, the signals we use in the port map must also match the type of the port they connect to.
When we write VHDL code, we may also wish to leave some ports unconnected.
For example, we may have a component which models the behaviour of a JK flip flop . However, we only need to use the inverted output in our design meaning. Therefore, we do not want to connect the non-inverted output to a signal in our architecture.
We can use the open keyword to indicate that we don't make a connection to one of the ports.
However, we can only use the open VHDL keyword for outputs.
If we attempt to leave inputs to our components open, our VHDL compiler will raise an error.
- VHDL Direct Entity Instantiation
The second instantiation technique is known as direct entity instantiation.
Using this method we can directly connect the entity in a new design without declaring a component first.
The code snippet below shows how we use direct entity instantiation in VHDL.
As with the component instantiation technique, <instance_name> must be unique for each instantiation in an architecture.
There are two extra requirements for this type of instantiation. We must explicitly state the name of both the library and the architecture which we want to use. This is shown in the example above by the <library_name> and <architecture_name> labels.
Once the component is instantiated within a VHDL architecture, we use a port map to connect signals to the ports. We use the VHDL port map in the same way for both direct entity and component instantiation.
Which types can not be used with the VHDL logical operators?
Scalar types such as integer and real.
Write the code for a 4 input NAND gate
We can use two different types of statement to model multiplexors in VHDL, what are they?
The with select statement and the when else statement
Write the code for an 8 input multiplexor using both types of statement
Write the code to instantiate a two input AND component using both direct entity and component instantiation. Assume that the AND gate is compiled in the work library and the architecture is named rtl.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Process statements and sequential execution in VHDL
For process statements in VHDL, it is said that the order of execution is sequential. My question is, are the signals a , b , and c assigned to their new values concurrently, or sequentially?
If this is sequential, I must say that after the end of the process, a is equal to b , b is equal to c , and c is equal to b , because we assigned b to a before we assigned a to c .
And finally a must be b , because c is assigned to b before last signal assignment. However, this does seem impossible to implement in hardware.

- \$\begingroup\$ stackoverflow.com/questions/13954193/… \$\endgroup\$ – user16324 Commented May 3, 2018 at 17:42
3 Answers 3
Within a process, statements are indeed carried out sequentially. However, values assigned to signals are not carried out immediately but scheduled to occur at the end of the process.
For example, when your third assignment c <= a; is carried out, the value of a is still the value a had at the start of the process.
This is because the first assignment a <= b; has not yet been carried out and a has not changed.
In fact, the first assignment will never be carried out because of the fourth assignment a <= c; , which will be scheduled to occur at the process end instead.
This behaviour reflects the behaviour of real logic circuitry, which is what VHDL was designed to do.
I recommend you read up on how VHDL processes work. Make sure you avoid a classic HDL trap: seeing a Hardware Design Language that models a logic circuit as a computer program that is executed by a CPU.

- \$\begingroup\$ I believe the compiler should warn or throw an error about multiple drivers for a . \$\endgroup\$ – Eugene Sh. Commented May 3, 2018 at 16:04
- 3 \$\begingroup\$ @EugeneSh., no, there's only one driver for 'a', that process. It contains multiple assignments to 'a', which is perfectly legal and commonplace in a process e.g. a<='1'; if b then a<='0'; end if; \$\endgroup\$ – TonyM Commented May 3, 2018 at 16:06
It often helps to look at the RTL schematic. Your code:
yields this:

Reading your statements in reverse order:
- a <= c -- the a register gets whatever was in the c register
- c <= a -- the c register gets whatever was in the a register
- b <= c -- the b register gets whatever was in the c register
- a <= b -- this statement is ignored 1 .
1 There can't be two drivers for a single FF input so, in VHDL, when multiple assignments to a signal occur within the same process statement, the last assigned value is the value which is propagated.
Notice also that b_reg and a_reg are exactly the same (i.e. they each clock the same signal in and out). If this simple example were to be synthesized, one of them would almost certainly be removed, and bout would be tied to aout . In fact, it took some keep attributes just to tell Vivado to not eliminate the b_reg for the RTL.
In VHDL, statements in process execute sequentially. As you mentioned a, b, c and d are signals (if they were variables, they had different manner). assume these statements in process:
a <= b; c <= a;
At the end of the process old value of b assigned to a. and old value of a assigned to c.
we can think in simpler way: statements in process executed sequentially, but the signals don't get the new values before end of the process. see this example that simulated in vivado:

you can see the initial value of the signal before rising edge of clock.
a=1; b=1; c=0;
In the rising edge of clock, the statements in the process executed. so at the end of process the new value of signals (according to assignment operations given in the question) are:

Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged vhdl or ask your own question .
- The Overflow Blog
- One of the best ways to get value for AI coding tools: generating tests
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
Hot Network Questions
- Is a thing just a class with only one member?
- Will "universal" SMPS work at any voltage in the range, even DC?
- Doesn't nonlocality follow from nonrealism in the EPR thought experiment and Bell tests?
- Stuck as a solo dev
- What place does the Abarbanel call טראסיי"א?
- What was the main implementation programming language of old 16-bit Windows versions (Windows 1 - Windows 3.11)?
- Whom did Jesus' followers accompany -- a soldier or a civilian?
- How to increase distance by a unit after every instance in Geometric Nodes array?
- Why was Esther included in the canon?
- Function with memories of its past life
- When I use \llap to overlap words, the space between the overlapped words and the rest of the text is too much: how do I fix it?
- What was the newest chess piece
- What common proverb does this string refer to?
- usetagform works inside equation but not work inside align
- What is the difference between a "statutory instruments" and "ministerial directions"?
- What would a planet need for rain drops to trigger explosions upon making contact with the ground?
- Place with signs in Chinese & Arabic
- Getting a planet to be as volcanic as Io
- What is the action-cost of grabbing spell components?
- Who pays the cost of Star Alliance lounge usage for cross-airline access?
- How in the world do I fix this anciently old slop sink? I am okay even with a temporary fix
- Ubuntu 22.04.5 - Final Point Release
- The consequence of a good letter of recommendation when things do not work out
- How frequently is random number generated when plotting function containing RandomReal?
- Network Sites:
- Technical Articles
- Market Insights

- Or sign in with
- iHeartRadio

The Variable: A Valuable Object in Sequential VHDL
Join our engineering community sign-in with:.
This article will discuss the important features of variables in VHDL.
The previous article in this series discussed that sequential statements allow us to describe a digital system in a more intuitive way. Variables are useful objects that can further facilitate the behavioral description of a circuit. This article will discuss the important features of variables. Several examples will be discussed to clarify the differences between variables and signals. Let’s first review VHDL signals.
Multiple Assignments to a Signal
VHDL uses signals to represent the circuit interconnects or wires. For example, consider the circuit in Figure 1.

The architecture of the VHDL code for this circuit is
As you can see, a signal has a clear mapping into hardware: it becomes a (group of) wire(s). Does it make sense to have multiple assignments to a signal? For example, consider the following code section:
If these two assignments are in the concurrent part of the code, then they are executed simultaneously. We can consider the equivalent hardware of the above code as shown in Figure 2.

Figure 2 suggests that multiple assignments to a signal in the concurrent part of the code is not a good idea because there can be a conflict between these assignments. For example, if A=C=0 and B=D=1, the first line would assign sig1 = (0 and 1) =0, while the second would attempt to assign sig1 = (0 or 1) = 1. That’s why, in the concurrent part of the code, VHDL doesn’t allow multiple assignments to a signal. What if these two assignments were in the sequential part of the code? A compiler may accept multiple assignments inside a process but, even in this case, only the last assignment will survive and the previous ones will be ignored. To explain this, note that a process can be thought of as a black box whose inner operation may be given by some abstract behaviour description. This description uses sequential statements. The connection between the process black box and the outside world is achieved through the signals. The process may read the value of these signals or assign a value to them. So VHDL uses signals to connect the sequential part of the code to the concurrent domain. Since a signal is connected to the concurrent domain of the code, it doesn’t make sense to assign multiple values to the same signal. That’s why, when facing multiple assignments to a signal, VHDL considers only the last assignment as the valid assignment.
Updating the Value of a Signal
The black box interpretation of a process reveals another important property of a signal assignment inside a process: When we assign a value to a signal inside a process, the new value of the signal won’t be available immediately. The value of the signal will be updated only after the conclusion of the current process run. The following example further clarifies this point. This example uses the VHDL “if” statements. Please note that we’ll see more examples of this statement in future articles; however, since it is similar to the conditional structures of other programming languages, the following code should be readily understood. You can find a brief description of this statement in a previous article.
Example: Write the VHDL code for a counter which counts from 0 to 5.
One possible VHDL description is given below:
In this example, sig1 is defined as a signal of type integer in the declarative part of the architecture. With each rising edge of clk, the value of the signal sig1 will increase by one. When sig1 reaches 6, the condition of the “if” statement in line 14 will be evaluated as true and sig1 will take the value zero. So it seems that sig1 , whose value is eventually passed to the output port out1 , will always take the values in the range 0 to 5. In other words, it seems that the “if” statement of line 14 will never let sig1 take the value 6. Let’s examine the operation of the code more closely.
Assume that a previous run of the process sets sig1 to 5. With the next rising edge of clk , the statements inside the “if” statement of line 12 will be executed. Line 13 will add one to the current value of sig1, which is 5, and assign the result to sig1 . Hence, the new value of sig1 will be 6; however, we should note that the value of the signal sig1 will be updated only after the conclusion of the current process run. As a result, in this run of the process, the condition of the “if” statement in line 14 will be evaluated as false and the corresponding “then” branch will be bypassed. Reaching the end of the process body, the value of sig1 will be updated to 6. While we intended sig1 to be in the range 0 to 5, it can take the value 6!
Similarly, at the next rising edge of clk, line 13 will assign 7 to sig1 . However, the signal value update will be postponed until we reach the end of the process body. In this run of the process, the condition of the “if” statement in line 14 returns true and, hence, line 15 will set sig1 to zero. As you see, in this run of the process, there are two assignments to the same signal. Based on the discussion of the previous section, only the last assignment will take effect, i.e. the new value of sig1 will be zero. Reaching the end of this process run, sig1 will take this new value. As you see, sig1 will take the values in the range from 0 to 6 rather than from 0 to 5! You can verify this in the following ISE simulation of the code.

Hence, when using signals inside a process, we should note that the new value of a signal will be available at the end of the current run of the process. Not paying attention to this property is a common source of mistake particularly for those who are new to VHDL.
To summarize our discussion so far, a signal models the circuit interconnections. If we assign multiple values to a signal inside a process, only the last assignment will be considered. Moreover, the assigned value will be available at the end of the process run and the updates are not immediate.
Variable: Another Useful VHDL Object
As discussed in a previous article, sequential statements allow us to have an algorithmic description of a circuit. The code of such descriptions is somehow similar to the code written by a computer programming language. In computer programming, “variables” are used to store information to be referenced and used by programs. With variables, we can more easily describe an algorithm when writing a computer program. That’s why, in addition to signals, VHDL allows us to use variables inside a process. While both signals and variables can be used to represent a value, they have several differences. A variable is not necessarily mapped into a single interconnection. Besides, we can assign several values to a variable and the new value update is immediate. In the rest of the article, we will explain these properties in more detail.
Before proceeding, note that variables can be declared only in a sequential unit such as a process (the only exception is a “shared” variable which is not discussed in this article). To get more comfortable with VHDL variables, consider the following code segment which defines variable var1 .
Similar to a signal, a variable can be of any data type (see the previous articles in this series to learn more about different data types). However, variables are local to a process. They are used to store the intermediate values and cannot be accessed outside of the process. Moreover, as shown by line 4 of the above code, the assignment to a variable uses the “:=” notation, whereas, the signal assignment uses “<=”.
Multiple Assignments to a Variable
Consider the following code. In this case, a variable, var1 , of type std_logic is defined. Then in lines 12, 13, and 14, three values are assigned to this variable.
Figure 4 shows the RTL schematic of the above code which is generated by Xilinx ISE.

It’s easy to verify that the produced schematic matches the behavior described in the process; however, this example shows that mapping variables into the hardware is somehow more complicated than that of signals. This is due to the fact that the sequential statements describe the behavior of a circuit. As you can see, in this example, each variable assignment operation of lines 13 and 14 have created a different wire though both of these two assignments use the same variable name, i.e. var1 .
Updating the Value of a Variable
Variables are updated immediately. To examine this, we’ll modify the code of the above counter and use a variable instead of a signal. The code is given below:
Since the new value of a variable is immediately available, the output will be in the range 0 to 5. This is shown in the following ISE simulation result.

- A signal models the circuit interconnections. If we assign multiple values to a signal inside a process, only the last assignment will be considered. Moreover, the assigned value will be available at the end of the current process run and the updates are not immediate.
- A single variable can produce several circuit interconnections.
- We can assign multiple values to the same variable and the assigned new values will take effect immediately.
- Similar to a signal, a variable can be of any data type.
- Variables are local to a process. They are used to store the intermediate values and cannot be accessed outside of the process.
- The assignment to a variable uses the “:=” notation, whereas, the signal assignment uses “<=”.
To see a complete list of my articles, please visit this page .
Related Content
- Safety in Sensing: Sensor Technology in Smart Cities
- Test & Measurement in Quantum Computing
- Reducing Distortion in Tape Recordings with Hysteresis in SPICE
- Explainer Video: Moving Object Simulation
- Sequential VHDL: If and Case Statements
- Managing Electromagnetic Interference in PCB Design
Learn More About:
- programmable logic
- sequential vhdl
- vhdl variable

You May Also Like

A Riveting Tale of the LED and its Journey to PCB Stardom
In Partnership with Autodesk

Overcoming the Challenges of Silicon Carbide to Ensure Application Success


Bluetooth Can Now Measure Distance, With Hardware Support En Route
by Duane Benson

Benefits of Designing with Wolfspeed Silicon Carbide (SiC) in Low Voltage Motor Drives
by Wolfspeed

Introduction to the Full-Bridge Rectifier
by Robert Keim

Welcome Back
Don't have an AAC account? Create one now .
Forgot your password? Click here .

Success! Subscription added.
Success! Subscription removed.
Sorry, you must verify to complete this action. Please click the verification link in your email. You may re-send via your profile .
- Intel Community
- Product Support Forums
- Intel® Quartus® Prime Software
VHDL sequential vs concurrent statement
- Subscribe to RSS Feed
- Mark Topic as New
- Mark Topic as Read
- Float this Topic for Current User
- Printer Friendly Page
- Mark as New
- Report Inappropriate Content
- All forum topics
- Previous topic
Link Copied

Community support is provided Monday to Friday. Other contact methods are available here .
Intel does not verify all solutions, including but not limited to any file transfers that may appear in this community. Accordingly, Intel disclaims all express and implied warranties, including without limitation, the implied warranties of merchantability, fitness for a particular purpose, and non-infringement, as well as any warranty arising from course of performance, course of dealing, or usage in trade.
For more complete information about compiler optimizations, see our Optimization Notice .
- ©Intel Corporation
- Terms of Use
- *Trademarks
- Supply Chain Transparency
Thank you for visiting nature.com. You are using a browser version with limited support for CSS. To obtain the best experience, we recommend you use a more up to date browser (or turn off compatibility mode in Internet Explorer). In the meantime, to ensure continued support, we are displaying the site without styles and JavaScript.
- View all journals
- Explore content
- About the journal
- Publish with us
- Sign up for alerts
- Published: 14 September 2024
Datopotamab–deruxtecan in early-stage breast cancer: the sequential multiple assignment randomized I-SPY2.2 phase 2 trial
- Katia Khoury ORCID: orcid.org/0000-0003-3899-8649 1 na1 ,
- Jane L. Meisel 2 na1 ,
- Christina Yau 3 ,
- Hope S. Rugo ORCID: orcid.org/0000-0001-6710-4814 3 ,
- Rita Nanda ORCID: orcid.org/0000-0001-5248-0876 4 ,
- Marie Davidian 5 ,
- Butch Tsiatis 5 ,
- A. Jo Chien 3 ,
- Anne M. Wallace 6 ,
- Mili Arora 7 ,
- Mariya Rozenblit 8 ,
- Dawn L. Hershman 9 ,
- Alexandra Zimmer ORCID: orcid.org/0000-0001-6789-0982 10 ,
- Amy S. Clark 11 ,
- Heather Beckwith 12 ,
- Anthony D. Elias 13 ,
- Erica Stringer-Reasor 1 ,
- Judy C. Boughey ORCID: orcid.org/0000-0003-3820-3228 14 ,
- Chaitali Nangia 15 ,
- Christos Vaklavas ORCID: orcid.org/0000-0002-9919-2748 16 ,
- Coral Omene 17 , 18 ,
- Kathy S. Albain 19 ,
- Kevin M. Kalinsky ORCID: orcid.org/0000-0002-3113-7902 2 ,
- Claudine Isaacs ORCID: orcid.org/0000-0002-9646-1260 20 ,
- Jennifer Tseng 21 ,
- Evanthia T. Roussos Torres ORCID: orcid.org/0000-0002-0740-5102 22 ,
- Brittani Thomas 23 ,
- Alexandra Thomas ORCID: orcid.org/0000-0001-9022-2229 24 ,
- Amy Sanford 25 ,
- Ronald Balassanian 3 ,
- Cheryl Ewing 3 ,
- Kay Yeung 6 ,
- Candice Sauder ORCID: orcid.org/0000-0001-6869-8634 7 ,
- Tara Sanft 8 ,
- Lajos Pusztai ORCID: orcid.org/0000-0001-9632-6686 8 ,
- Meghna S. Trivedi 9 ,
- Ashton Outhaythip 10 ,
- Natsuko Onishi ORCID: orcid.org/0000-0003-4495-9187 3 ,
- Adam L. Asare 3 , 26 ,
- Philip Beineke 26 ,
- Peter Norwood 26 ,
- Lamorna Brown-Swigart ORCID: orcid.org/0000-0003-2076-5177 3 ,
- Gillian L. Hirst ORCID: orcid.org/0000-0002-4502-0035 3 ,
- Jeffrey B. Matthews 3 ,
- Brian Moore 24 ,
- W. Fraser Symmans 27 ,
- Elissa Price 3 ,
- Carolyn Beedle 3 ,
- Jane Perlmutter 28 ,
- Paula Pohlmann ORCID: orcid.org/0000-0001-7914-5162 27 ,
- Rebecca A. Shatsky 6 ,
- Angela DeMichele ORCID: orcid.org/0000-0003-1297-4251 11 ,
- Douglas Yee ORCID: orcid.org/0000-0002-3387-4009 12 ,
- Laura J. van ‘t Veer ORCID: orcid.org/0000-0002-9838-8298 3 ,
- Nola M. Hylton 3 &
- Laura J. Esserman ORCID: orcid.org/0000-0001-9202-4568 3
Nature Medicine ( 2024 ) Cite this article
Metrics details
- Breast cancer
- Clinical trial design
Among the goals of patient-centric care are the advancement of effective personalized treatment, while minimizing toxicity. The phase 2 I-SPY2.2 trial uses a neoadjuvant sequential therapy approach in breast cancer to further these goals, testing promising new agents while optimizing individual outcomes. Here we tested datopotamab–deruxtecan (Dato-DXd) in the I-SPY2.2 trial for patients with high-risk stage 2/3 breast cancer. I-SPY2.2 uses a sequential multiple assignment randomization trial design that includes three sequential blocks of biologically targeted neoadjuvant treatment: the experimental agent(s) (block A), a taxane-based regimen tailored to the tumor subtype (block B) and doxorubicin–cyclophosphamide (block C). Patients are randomized into arms consisting of different investigational block A treatments. Algorithms based on magnetic resonance imaging and core biopsy guide treatment redirection after each block, including the option of early surgical resection in patients predicted to have a high likelihood of pathological complete response, the primary endpoint. There are two primary efficacy analyses: after block A and across all blocks for the six prespecified breast cancer subtypes (defined by clinical hormone receptor/human epidermal growth factor receptor 2 (HER2) status and/or the response-predictive subtypes). We report results of 103 patients treated with Dato-DXd. While Dato-DXd did not meet the prespecified threshold for success (graduation) after block A in any subtype, the treatment strategy across all blocks graduated in the hormone receptor-negative HER2 − Immune − DNA repair deficiency − subtype with an estimated pathological complete response rate of 41%. No new toxicities were observed, with stomatitis and ocular events occurring at low grades. Dato-DXd was particularly active in the hormone receptor-negative/HER2 − Immune − DNA repair deficiency − signature, warranting further investigation, and was safe in other subtypes in patients who followed the treatment strategy. ClinicalTrials.gov registration: NCT01042379 .
This is a preview of subscription content, access via your institution
Access options
Access Nature and 54 other Nature Portfolio journals
Get Nature+, our best-value online-access subscription
24,99 € / 30 days
cancel any time
Subscribe to this journal
Receive 12 print issues and online access
195,33 € per year
only 16,28 € per issue
Buy this article
- Purchase on SpringerLink
- Instant access to full article PDF
Prices may be subject to local taxes which are calculated during checkout
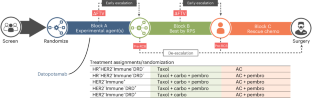
Data availability
De-identified subject level data and/or clinical specimens are made available to members of the research community upon approval of the I-SPY Data Access and Publications Committee. Details of the application and review process are available at https://www.quantumleaphealth.org/for-investigators/clinicians-proposal-submissions/ . I-SPY aims to make complete patient-level clinical datasets available for public access within 6 months of publication, as the data is complex and requires extensive annotation to ensure its usability.
Code availability
The statistical code used in this clinical trial is available to other investigators for approved research purposes. Investigators interested in accessing the code complete an application at https://www.quantumleaphealth.org/for-investigators/clinicians-proposal-submissions/ and include a brief description of the intended use. Access to the code will be granted upon approval of the request, subject to compliance with ethical guidelines and applicable institutional and regulatory requirements.
Nanda, R. et al. Effect of pembrolizumab plus neoadjuvant chemotherapy on pathologic complete response in women with early-stage breast cancer. JAMA Oncol. 6 , 676–684 (2020).
Article PubMed Google Scholar
Schmid, P. et al. Pembrolizumab for early triple-negative breast cancer. N. Engl. J. Med. 382 , 810–821 (2020).
Article CAS PubMed Google Scholar
I-SPY2 Trial Consortium. Association of event-free and distant recurrence–free survival with individual-level pathologic complete response in neoadjuvant treatment of stages 2 and 3 breast cancer. JAMA Oncol. 6 , 1355–1362 (2020).
Article Google Scholar
Li, W. et al. Abstract P4-02-10: MRI models by response predictive subtype for predicting pathologic complete response. Cancer Res. 83 , P4-02-10 (2023).
Google Scholar
Wolf, D. M. et al. Redefining breast cancer subtypes to guide treatment prioritization and maximize response: Predictive biomarkers across 10 cancer therapies. Cancer Cell 40 , 609–623.e6 (2022).
Article CAS PubMed PubMed Central Google Scholar
Onishi, N. et al. Abstract P3-03-01: functional tumor volume at 3 and 6 week MRI as an indicator of patients with inferior outcome after neoadjuvant chemotherapy. Cancer Res. 82 , P3-03-01 (2022).
Onishi, N. et al. Prospective performance of an MRI algorithm for early re-direction of breast cancer neoadjuvant treatment. In Proc. 32nd Annual Scientific Meeting and Exhibition of the International Society for Magnetic Resonance in Medicine (International Society for Magnetic Resonance in Medicine, 2024).
Okajima, D. et al. Datopotamab deruxtecan (Dato-DXd), a novel TROP2-directed antibody–drug conjugate, demonstrates potent antitumor activity by efficient drug delivery to tumor cells. Mol. Cancer Ther. 20 , 2329–2340 (2021).
Article PubMed PubMed Central Google Scholar
Sakach, E., Sacks, R. & Kalinsky, K. Trop-2 as a therapeutic target in breast cancer. Cancers 14 , 5936 (2022).
Bardia, A. et al. Datopotamab deruxtecan in advanced or metastatic HR+/HER2− and triple-negative breast cancer: results from the phase I TROPION-PanTumor01 study. J. Clin. Oncol. 42 , 2281–2294 (2024).
Gadaleta-Caldarola, G. et al. Safety evaluation of datopotamab deruxtecan for triple-negative breast cancer: a meta-analysis. Cancer Treat. Res. Commun. 37 , 100775 (2023).
Dent, R. A. et al. TROPION-Breast02: datopotamab deruxtecan for locally recurrent inoperable or metastatic triple-negative breast cancer. Future Oncol. 19 , 2349–2359 (2023).
Bardia, A. et al. TROPION-Breast03: a randomized phase III global trial of datopotamab deruxtecan ± durvalumab in patients with triple-negative breast cancer and residual invasive disease at surgical resection after neoadjuvant therapy. Ther. Adv. Med. Oncol. 16 , 17588359241248336 (2024).
Bardia, A. et al. TROPION-Breast01: datopotamab deruxtecan vs chemotherapy in pre-treated inoperable or metastatic HR+/HER2− breast cancer. Futur. Oncol. 20 , 423–436 (2024).
Article CAS Google Scholar
Rugo, H. S. et al. Adaptive randomization of veliparib–carboplatin treatment in breast cancer. N. Engl. J. Med. 375 , 23–34 (2016).
Shatsky, R. A. et al. Datopotamab–deruxtecan plus durvalumab in early-stage breast cancer: the sequential multiple assignment randomized I-SPY2.2 phase 2 trial. Nat. Med. https://doi.org/10.1038/s41591-024-03267-1 (2024).
Lavori, P. W. & Dawson, R. Introduction to dynamic treatment strategies and sequential multiple assignment randomization. Clin. Trials 11 , 393–399 (2014).
Li, W. et al. Predicting breast cancer response to neoadjuvant treatment using multi-feature MRI: results from the I-SPY 2 TRIAL. NPJ Breast Cancer 6 , 63 (2020).
Symmans, W. F. et al. Measurement of residual breast cancer burden to predict survival after neoadjuvant chemotherapy. J. Clin. Oncol. 25 , 4414–4422 (2007).
Yau, C. et al. Residual cancer burden after neoadjuvant chemotherapy and long-term survival outcomes in breast cancer: a multicentre pooled analysis of 5161 patients. Lancet Oncol. 23 , 149–160 (2022).
Common terminology criteria for adverse events (CTCAE) protocol development. CTEP National Cancer Institute https://ctep.cancer.gov/protocolDevelopment/electronic_applications/ctc.htm (2021).
Basch, E. et al. Development of the National Cancer Institute’s patient-reported outcomes version of the common terminology criteria for adverse events (PRO-CTCAE). J. Natl Cancer Inst. 106 , dju244 (2014).
Jacob, S. et al. Use of PROMIS to capture patient reported outcomes over time for patients on I-SPY2. J. Clin. Oncol. 41 , 611 (2023).
Pearman, T. P., Beaumont, J. L., Mroczek, D., O’Connor, M. & Cella, D. Validity and usefulness of a single-item measure of patient-reported bother from side effects of cancer therapy. Cancer 124 , 991–997 (2018).
Oken, M. M. et al. Toxicity and response criteria of the eastern-cooperative-oncology-group. Am. J. Clin. Oncol. 5 , 649–655 (1982).
Cardoso, F. et al. 70-Gene signature as an aid to treatment decisions in early-stage breast cancer. N. Engl. J. Med. 375 , 717–729 (2016).
Beltran, P. J. et al. Ganitumab (AMG 479) inhibits IGF-II–dependent ovarian cancer growth and potentiates platinum-based chemotherapy. Clin. Cancer Res. 20 , 2947–2958 (2014).
Pusztai, L. et al. Durvalumab with olaparib and paclitaxel for high-risk HER2-negative stage II/III breast cancer: results from the adaptively randomized I-SPY2 trial. Cancer Cell 39 , 989–998.e5 (2021).
Piccart, M. et al. 70-Gene signature as an aid for treatment decisions in early breast cancer: updated results of the phase 3 randomised MINDACT trial with an exploratory analysis by age. Lancet Oncol. 22 , 476–488 (2021).
Brahmer, J. R. et al. Society for Immunotherapy of Cancer (SITC) clinical practice guideline on immune checkpoint inhibitor-related adverse events. J. Immunother. Cancer 9 , e002435 (2021).
Haanen, J. et al. Management of toxicities from immunotherapy: ESMO clinical practice guideline for diagnosis, treatment and follow-up. Ann. Oncol. 33 , 1217–1238 (2022).
Download references
Acknowledgements
Research reported in this paper was supported by the NCI of the National Institutes of Health (NIH) under award numbers P01CA210961 and U01CA225427 (L.J.E., N.H.). We acknowledge the generous support of the study sponsors and operations management, Quantum Leap Healthcare Collaborative (QLHC, 2013 to present) and the Foundation for the NIH (2010 to 2012; to L.J.E.). We sincerely appreciate the ongoing support for the I-SPY2.2 Trial from the Safeway Foundation, the William K. Bowes, Jr. Foundation, Give Breast Cancer the Boot and QLHC (all to L.J.E.) and the Breast Cancer Research Foundation (to L.J.E. and L.J.v.V.). We thank all the patients who volunteered to participate in I-SPY2. AstraZeneca provided funds and study drugs (datopotamab–deruxtecan and durvalumab). With the exception of QLHC, the funders had no role in study design, data collection and analysis, decision to publish or preparation of the manuscript. We thank D. Wolf and J. Gibbs and the I-SPY patient advocates for all their important contributions to this work.
Author information
These authors contributed equally: Katia Khoury, Jane L. Meisel.
Authors and Affiliations
University of Alabama at Birmingham, Birmingham, AL, USA
Katia Khoury & Erica Stringer-Reasor
Emory University, Atlanta, GA, USA
Jane L. Meisel & Kevin M. Kalinsky
University of California San Francisco, San Francisco, CA, USA
Christina Yau, Hope S. Rugo, A. Jo Chien, Ronald Balassanian, Cheryl Ewing, Wen Li, Natsuko Onishi, Adam L. Asare, Lamorna Brown-Swigart, Gillian L. Hirst, Jeffrey B. Matthews, Elissa Price, Carolyn Beedle, Laura J. van ‘t Veer, Nola M. Hylton & Laura J. Esserman
University of Chicago, Chicago, IL, USA
North Carolina State University, Raleigh, NC, USA
Marie Davidian & Butch Tsiatis
University of California San Diego, San Diego, CA, USA
Anne M. Wallace, Kay Yeung & Rebecca A. Shatsky
University of California Davis, Davis, CA, USA
Mili Arora & Candice Sauder
Yale University, New Haven, CT, USA
Mariya Rozenblit, Tara Sanft & Lajos Pusztai
Columbia University, New York, NY, USA
Dawn L. Hershman & Meghna S. Trivedi
Oregon Health Sciences University, Portland, OR, USA
Alexandra Zimmer & Ashton Outhaythip
University of Pennsylvania, Philadelphia, PA, USA
Amy S. Clark & Angela DeMichele
University of Minnesota, Minneapolis, MN, USA
Heather Beckwith & Douglas Yee
University of Colorado Denver, Denver, CO, USA
Anthony D. Elias
The Mayo Clinic, Rochester, MN, USA
Judy C. Boughey
HOAG Family Cancer Institute, Newport Beach, CA, USA
Chaitali Nangia
Huntsman Cancer Institute, University of Utah, Salt Lake City, UT, USA
Christos Vaklavas
Cooperman Barnabas Medical Center, New Brunswick, NJ, USA
Coral Omene
Rutgers Cancer Institute of New Jersey, New Brunswick, NJ, USA
Stritch School of Medicine, Loyola University Chicago, Chicago, IL, USA
Kathy S. Albain
Lombardi Comprehensive Cancer Center, Georgetown University, Washington DC, USA
Claudine Isaacs
City of Hope Orange County Lennar Foundation Cancer Center, Orange County, CA, USA
Jennifer Tseng
University of Southern California, Los Angeles, CA, USA
Evanthia T. Roussos Torres
Sparrow Health System, Lansing, MI, USA
Brittani Thomas
Wake Forest University, Winston–Salem, NC, USA
Alexandra Thomas & Brian Moore
Sanford Health, Sioux Falls, SD, USA
Amy Sanford
Quantum Leap Healthcare Collaborative, San Francisco, CA, USA
Adam L. Asare, Philip Beineke & Peter Norwood
University of Texas MD Anderson Cancer Center, Houston, TX, USA
W. Fraser Symmans & Paula Pohlmann
The Gemini Group, Ann Arbor, MI, USA
Jane Perlmutter
You can also search for this author in PubMed Google Scholar
Contributions
Arm chaperones: J.L.M. and K.K. Leadership: C.Y., H.S.R., R.N., B.M., J.P., P.P., R.A.S., A.D., D.Y., L.J.v.V., N.M.H. and L.J.E. Study design: C.Y., M.D., B. Tsiatis, A.D., D.Y., L.J.v.V., N.M.H. and L.J.E. Formal analysis: C.Y. and P.N. Enrolled patients: J.L.M., K.K., H.S.R., R.N., A.J.C., A.M.W., M.A., M.R., D.L.H., A.Z., A.S.C., H.B., A.D.E., E.S.-R., J.C.B., C.N., C.V., C.O., K.S.A., K.M.K., C.I., J.T., E.T.R.T., B.T., A.T., A.S., R.B., C.E., K.Y., C.S., T.S., L.P., M.S.T., A.O., R.A.S., A.D., D.Y. and L.J.E. Laboratory studies: L.B.-S., G.L.H. and L.J.v.V. Imaging/pathology: W.L., N.O., W.F.S. and N.M.H. Data management: A.L.A. and P.B. Administration: G.L.H. and J.B.M. First draft: J.L.M., K.K., C.Y., P.N., J.B.M. and L.J.E. Edit and review: J.L.M., K.K., C.Y., H.S.R., R.N., M.D., B. Tsiatis, A.J.C., A.M.W., M.A., M.R., D.L.H., A.Z., A.S.C., H.B., A.D.E., E.S.-R., J.C.B., C.N., C.V., C.O., K.S.A., K.M.K., C.I., J.T., E.T.R.T., B. Thomas, A.T., A.S., R.B., C.E., K.Y., C.S., T.S., L.P., M.S.T., A.O., W.L., N.O., A.L.A., P.B., P.N., L.B.-S., G.L.H., J.B.M., B.M., W.F.S., E.P., C.B., J.P., P.P., R.A.S., A.D., D.Y., L.J.v.V., N.M.H. and L.J.E.
Corresponding author
Correspondence to Laura J. Esserman .
Ethics declarations
Competing interests.
J.L.M. reports institutional research funding from AstraZeneca, Seagen, Sermonix and Olema and advisory and consulting roles with Pfizer, Seagen, Sermonix, Novartis, Stemline, AstraZeneca, Olema, GlaxoSmithKline and GE Healthcare. C.Y. reports institutional research grant from NCI/NIH; salary support and travel reimbursement from QLHC; a United States patent titled ‘Breast cancer response prediction subtypes’ (no. 18/174,491); and University of California Inventor Share. H.S.R. reports institutional research support from AstraZeneca, Daiichi Sankyo, Inc., F. Hoffmann–La Roche AG/Genentech, Inc., Gilead Sciences, Inc., Lilly; Merck and Co., Novartis Pharmaceuticals Corporation, Pfizer, Stemline Therapeutics, OBI Pharma, Ambrx, Greenwich Pharma; and advisory and consulting roles with Chugai, Puma, Sanofi, Napo and Mylan. R.N. reports research funding from Arvinas, AstraZeneca, BMS, Corcept Therapeutics, Genentech/Roche, Gilead, GSK, Merck, Novartis, OBI Pharma, OncoSec, Pfizer, Relay, Seattle Genetics, Sun Pharma and Taiho and advisory roles with AstraZeneca, BeyondSpring, Daiichi Sankyo, Exact Sciences, Fujifilm, GE, Gilead, Guardant Health, Infinity, iTeos, Merck, Moderna, Novartis, OBI, OncoSec, Pfizer, Sanofi, Seagen and Stemline. M.D. reports research grants from NIH/NCI and NIH/NIA, and contracts from PCORI. A.J.C. reports institutional research funding from Merck, Amgen, Puma, Seagen, Pfizer and Olema and advisory roles with AstraZeneca and Genentech. A.Z. reports institutional research funding from Merck, honoraria for Medscape and participation on Pfizer Advisory Board. A.S.C. reports institutional research funding from Novartis and Lilly. A.D.E. reports support from Scorpion, Infinity and Deciphera. E.S.-R. reports grants from V Foundation, NIH, Susan G. Komen; institutional research funding from GSK, Seagen, Pfizer, Lilly; consulting and honoraria from Novartis, Merck, Seagen, AstraZeneca, Lilly; Cancer Awareness Network Board member and support from ASCO and NCCN. J.C.B. reports institutional research funding from Eli Lilly and SymBioSis, participation on the Data Safety Monitoring Committee of Cairn Surgical and honoraria from PER, PeerView, OncLive, EndoMag and UpToDate. C.V. reports institutional research funding from Pfizer, Seagen, H3 Biomedicine/Eisai, AstraZeneca, CytomX; research funding to previous institution from Genentech, Roche, Pfizer, Incyte, Pharmacyclics, Novartis, TRACON Pharmaceuticals, Innocrin Pharmaceuticals, Zymeworks and H3 Biomedicine; advisory and consulting roles with Guidepoint, Novartis, Seagen, Daiichi Sankyo, AstraZeneca and Cardinal Health; unpaid consulting with Genentech; and participation in non-CME activity with Gilead, AstraZeneca. C.O. reports consulting fees from AstraZeneca, Guardant Health and Jazz Pharmaceuticals. K.S.A. reports institutional research funding from AstraZeneca, Daiichi Sankyo, Seattle Genetics and QLHC; Independent Data and Safety Monitoring committee at Seattle Genetics. K.M.K. reports advisory and consultant roles for Eli Lilly, Pfizer, Novartis, AstraZeneca, Daiichi Sankyo, Puma, 4D Pharma, OncoSec, Immunomedics, Merck, Seagen, Mersana, Menarini Silicon Biosystems, Myovant, Takeda, Biotheranostics, Regor, Gilead, Prelude Therapeutics, RayzeBio, eFFECTOR Therapeutics and Cullinan Oncology; and reports institutional research funding from Genentech/Roche, Novartis, Eli Lilly, AstraZeneca, Daiichi Sankyo and Ascentage. C.I. reports institutional research funding from Tesaro/GSK, Seattle Genetics, Pfizer, AstraZeneca, BMS, Genentech, Novartis and Regeneron; consultancy roles with AstraZeneca, Genentech, Gilead, ION, Merck, Medscape, MJH Holdings, Novartis, Pfizer, Puma and Seagen; and royalties from Wolters Kluwer (UptoDate) and McGraw Hill (Goodman and Gillman). J.T. serves as institutional principal investigator for clinical trial with Intuitive Surgical; editor lead for ABS, CGSO, SCORE, Breast Education Committee Track Leader, ASCO SESAP 19 and Breast Co-Chair, ACS. A.T. owns stock at Johnson and Johnsons, Gilead, Bristol Myers Squibb, reports participation on Pfizer Advisory Board: AstraZeneca and reports institutional research funding from Merck and Sanofi and royalties from UptoDate. R.B. reports a consultancy role at Genentech and stock ownership at Cerus Corp. K.Y. received research support unrelated to this work and paid to the institution from Pfizer, Gilead, Seagen, Dantari Pharmaceuticals, Treadwell Therapeutics, and Relay Therapeutics; support from American Cancer Society IRG grant no. IRG-19-230-48-IRG, UC San Diego Moores Cancer Center, Specialized Cancer Center support grant NIH/NCI P30CA023100, Curebound Discovery Award (2023, 2024). T.S. reports honoraria from Hologic. L.P. reports institutional research funding from Susan Komen Foundation, Breast Cancer Research Foundation, NCI, Pfizer, AstraZeneca, Menarini/Stemline, Bristol Myers Squibb, Merck and Co.; consulting fees from AstraZeneca, Merck, Novartis, Genentech, Natera, Personalis, Exact Sciences and Stemline/Menarini; patent titled ‘Method of measuring residual cancer and predicting patient survival’ (no. 7711494); and Data and Safety Monitoring Board member of the DYNASTY Breast02, OPTIMA and PARTNER trials. M.S.T. reports institutional research funding from Lilly, Gilead Sciences, Phoenix Molecular Designs, AstraZeneca, Regeneron, Merck and Novartis. A.L.A., P.B. and P.N. are employees of QLHC. G.L.H. reports institutional research grant from NIH (1R01CA255442). W.F.S. reports shares of IONIS Pharmaceuticals and Eiger Biopharmaceuticals, received consulting fees from AstraZeneca, is a cofounder with equity in Delphi Diagnostics and issued patents for (1) a method to calculate residual cancer burden and (2) genomic signature to measure sensitivity to endocrine therapy. J.P. reports honoraria from Methods in Clinical Research—faculty SCION workshop; support from ASCO and advocate scholarship; AACR—SSP program; VIVLI, U Wisc SPORE—EAB, QuantumLEAD—COVID DSMB, PCORI—reviewer and I-SPY advocate lead. P.P. reports institutional research funding from Genentech/Roche, Fabre-Kramer, Advanced Cancer Therapeutics, Caris Centers of Excellence, Pfizer, Pieris Pharmaceuticals, Cascadian Therapeutics, Bolt, Byondis, Seagen, Orum Therapeutics and Carisma Therapeutics; consulting fees from Personalized Cancer Therapy, OncoPlex Diagnostics, Immunonet BioSciences, Pfizer, HERON, Puma Biotechnology, Sirtex, CARIS Life sciences, Juniper, Bolt Biotherapeutics and AbbVie; honoraria from DAVA Oncology, OncLive/MJH Life Sciences, Frontiers—publisher, SABCS and ASCO; Speakers’ Bureau: Genentech/Roche (past); United States patent no. 8486413, United States patent no. 8501417, United States patent no. 9023362, United States patent no. 9745377; uncompensated roles with Pfizer, Seagen and Jazz. R.A.S. reports institutional research funding from OBI Pharma, QLHC, AstraZeneca and Gilead, serves on AstraZeneca and Stemline Advisory Boards and Gilead Speaker’s Bureau and reports consultancy role with QLHC. A.D. reports institutional research funding from Novartis, Pfizer, Genentech and NeoGenomics; Program Chair, Scientific Advisory Committee, ASCO. D.Y. reports research funding from NIH/NCI P30 CA 077598, P01 CA234228-01 and R01CA251600, consulting fees from Martell Diagnostics, and honoraria and travel for speaking at the ‘International Breast Cancer Conference.’ L.J.v.V. is a founding advisor and shareholder of Exai Bio and is a part-time employee and owns stock in Agendia. N.M.H. reports institutional research funding from NIH. L.J.E. reports funding from Merck and Co., participation on an advisory board for Blue Cross Blue Shield and personal fees from UpToDate and is an unpaid board member of QLHC. The other authors declare no competing interests.
Peer review
Peer review information.
Nature Medicine thanks Barbara Pistilli and Sze-Huey Tan for their contribution to the peer review of this work. Primary Handling Editor: Ulrike Harjes, in collaboration with the Nature Medicine team.
Additional information
Publisher’s note Springer Nature remains neutral with regard to jurisdictional claims in published maps and institutional affiliations.
Supplementary information
Supplementary information.
Supplementary figures and tables.
Reporting Summary
Rights and permissions.
Springer Nature or its licensor (e.g. a society or other partner) holds exclusive rights to this article under a publishing agreement with the author(s) or other rightsholder(s); author self-archiving of the accepted manuscript version of this article is solely governed by the terms of such publishing agreement and applicable law.
Reprints and permissions
About this article
Cite this article.
Khoury, K., Meisel, J.L., Yau, C. et al. Datopotamab–deruxtecan in early-stage breast cancer: the sequential multiple assignment randomized I-SPY2.2 phase 2 trial. Nat Med (2024). https://doi.org/10.1038/s41591-024-03266-2
Download citation
Received : 07 August 2024
Accepted : 23 August 2024
Published : 14 September 2024
DOI : https://doi.org/10.1038/s41591-024-03266-2
Share this article
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
Quick links
- Explore articles by subject
- Guide to authors
- Editorial policies
Sign up for the Nature Briefing: Cancer newsletter — what matters in cancer research, free to your inbox weekly.

- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
When do signals get assigned in VHDL?
Considering this code:
Is the second line going to respect that a changed in the other process or are all signals only at the end of architecture assigned?

- related stackoverflow.com/questions/5060635/… – Ciro Santilli OurBigBook.com Commented Jun 20, 2016 at 0:53
2 Answers 2
Careful with your terminology. When you say a changed in the other "process", that has a specific meaning in VHDL (process is a keyword in VHDL), and your code does not have any processes.
Synthesizers will treat your code as:
Simulators will typically assign a in a first pass, then assign b on a second pass one 'delta' later. A delta is an infinitesimal time delay that takes place at the same simulation time as the initial assignment.
Note this is a gross generalization of what really happens...if you want full details, read up on the documentation provided with your tool chain.
- 1 We learned that those lines above are called implicit processes , is there another name for them? – Georg Schölly Commented Oct 11, 2011 at 7:40
- 2 @GeorgSchölly You are correct. Concurrent statements get elaborated as processes. Hence, your signal assignments could be designated as processes. – Philippe Commented Oct 11, 2011 at 11:28
- 2 @Charles behavior is not tool dependent, but it is well defined by the VHDL standard. Here is a blog post that explains about delta cycles: sigasi.com/content/vhdls-crown-jewel – Philippe Commented Oct 11, 2011 at 11:30
It sounds like you are thinking of signal behaviour within a single process when you say this. In that context, the signals are not updated until the end of the process, so the b update will use the "old" value of a
However, signal assignments not inside a process statement are executed continuously, there is nothing to "trigger" an architecture to "run". Or alternatively, they are all individual separate implied processes (as you have commented), with a sensitivity list implied by everything on the "right-hand-side".
In your particular case, the b assignment will use the new value of a , and the assignment will happen one delta-cycle after the a assignment.
For a readable description of how simulation time works in VHDL, see Jan Decaluwe's page here:
http://www.sigasi.com/content/vhdls-crown-jewel
And also this thread might be instructive:
https://groups.google.com/group/comp.lang.vhdl/browse_thread/thread/e47295730b0c3de4/d5bd4532349aadf0?hl=en&ie=UTF-8&q=vhdl+concurrent+assignment#d5bd4532349aadf0
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged vhdl or ask your own question .
- The Overflow Blog
- One of the best ways to get value for AI coding tools: generating tests
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- Should I be careful about setting levels too high at one point in a track?
- Who pays the cost of Star Alliance lounge usage for cross-airline access?
- How did people know that the war against the mimics was over?
- What factors cause differences between dried herb/spice brands?
- Why was Panama Railroad in poor condition when US decided to build Panama Canal in 1904?
- What common proverb does this string refer to?
- Will "universal" SMPS work at any voltage in the range, even DC?
- History of the migration of ERA from AMS to AIMS in 2007
- What is the action-cost of grabbing spell components?
- Does SpaceX Starship have significant methane emissions?
- What's the difference between "Erase All Content and Settings" and using the Disk Utility to erase a Mac with Apple silicon
- The meaning of an implication with the existential quantifier
- Place with signs in Chinese & Arabic
- Offset+Length vs 2 Offsets
- Why did early ASCII have ← and ↑ but not ↓ or →?
- Is it possible to draw this picture without lifting the pen? (I actually want to hang string lights this way in a gazebo without doubling up)
- Is it true that before European modernity, there were no "nations"?
- Swapping front Shimano 105 R7000 34x50t 11sp Chainset with Shimano Deore FC-M5100 chainset; 11-speed 26x36t
- How to increase distance by a unit after every instance in Geometric Nodes array?
- Why does a capacitor act as an open circuit under a DC circuit?
- Understanding symmetry in a double integral
- What’s the name of this horror movie where a girl dies and comes back to life evil?
- Could Prop be the top universe?
- What place does the Abarbanel call טראסיי"א?

IMAGES
VIDEO
COMMENTS
signals on the right-hand side of sequential signal assignments are in the sensitivity list. Thus these synthesis tools will interpret the two processes in Example 6.2 to be identical. Example 6.2 proc1: process (a, b, c) begin x <= a and b and c; end process proc1; proc2: process (a, b) begin x <= a and b and c; end process proc2;
A sequential signal assignment takes effect only when the process suspends. If there is more than one assignment to the same signal before suspension, the last one executed takes effect: ... In VHDL-93, any signal assignment statement may have an optional label: label: signal_name <= expression; A delayed signal assignment with inertial delay ...
Signal assignments and procedure calls that are done in the architecture are concurrent. Sequential statements (other than wait) run when the code around it also runs. Interesting note, while a process is concurrent (because it runs independently of other processes and concurrent assignments), it contains sequential statements.
Conditional Signal Assignment or the "When/Else" Statement. The "when/else" statement is another way to describe the concurrent signal assignments similar to those in Examples 1 and 2. Since the syntax of this type of signal assignment is quite descriptive, let's first see the VHDL code of a one-bit 4-to-1 multiplexer using the ...
A process is a wrapper for sequential statements. Sequential statements model combinational or synchronous logic (or both) Statements within a process are 'executed' sequentially (but use care in interpreting this statement) Signal assignments can be both sequential and concurrent. 'Variables' may be declared within a process (more later ...
VHDL-93: Section 8.3; Syntax . signal_name <= expression; signal_name <= expression after delay; Rules and Examples . A sequential signal assignment takes effect only when the process suspends. If there is more than one assignment to the same signal before suspension, the last one executed takes effect:
The signal assignment statement is typically considered a concurrent statement rather than a sequential statement. It can be used as a sequential statement but has the side effect of obeying the general rules for when the target actually gets updated. In particular, a signal can not be declared within a process or subprogram but must be ...
The "if" statements of VHDL are similar to the conditional structures utilized in computer programming languages. Listing 1 below shows a VHDL "if" statement. Listing 1. 1if Expression_1 then. 2Output_signal <= Value1; 3elsif Expression_2 then. 4Output_signal <= Value2; 5elsif Expression_3 then.
Sequential signal assignment statement. Syntax. signal_name <= value_expression; Syntax is identical to the simple concurrent signal assignment. Caution: Inside a process, a signal can be assigned multiple times, but only the last assignment takes effect. RTL Hardware Design by P. Chu. Chapter 5.
A conditional assignment statement is also a concurrent signal assignment statement. target <= waveform when choice; -- choice is a boolean expression target <= waveform when choice else waveform; sig <= a_sig when count>7; sig2 <= not a_sig after 1 ns when ctl='1' else b_sig; "waveform" for this statement seems to include [ delay_mechanism ...
Hardware Design with VHDL Sequential Stmts ECE 443 ECE UNM 6 (9/14/09) Sequential Signal Assignment Statement This process immediately executes and computes the output for y It then waits for a change on a, b or c-- on a change it continues and resets the output y to a new value based on the input signal change, and suspends again Note this describes the 3-input AND gate as well, however, the ...
The VHDL code shown below uses one of the logical operators to implement this basic circuit. and_out <= a and b; Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals.
VHDL CONSTRUCTS C. E. Stroud, ECE Dept., Auburn Univ. 3 8/04 Signal assignments in a concurrent statement: Like a process with implied sensitivity list (right-hand side of <=) ∴ multiple concurrent statements work like multiple 1-line processes updates assigned signal whenever right-hand has event Example: D <= Q xor CIN; COUT <= Q xor CIN;
The form of this is a 'conditional signal assignment', which happens to have been added to sequential signal assignments by IEEE Std 1076-2008 (10.5.3 Conditional signal assignments, § 10 is entitled Sequential statements). ... VHDL signals with initialized values. Hot Network Questions
Add a comment. In VHDL, statements in process execute sequentially. As you mentioned a, b, c and d are signals (if they were variables, they had different manner). assume these statements in process: a <= b; c <= a; At the end of the process old value of b assigned to a. and old value of a assigned to c.
So VHDL uses signals to connect the sequential part of the code to the concurrent domain. Since a signal is connected to the concurrent domain of the code, it doesn't make sense to assign multiple values to the same signal. That's why, when facing multiple assignments to a signal, VHDL considers only the last assignment as the valid assignment.
So can I conclude there is no such thing as sequential signal assignment in VHDL? In most books and online tutorials, e.g. in RTL Hardware Design by Prof Chu, it is written "The syntax of a sequential signal assignment is identical to that of the simple concurrent signal assignment of Chapter 4 except that the former is inside a process"- Page 100.
The simulator is only be able to perform one process at the time due to a sequential simulation model (not to confuse with the concurrent model of vhdl). So if process A is simulated first and A changes the signal, B would have the wrong signal value. Therefore the signal can only be changed after all the triggered processes are done.
I-SPY2.2, targeting early breast cancer, is a hybrid design consisting of a sequential multiple assignment randomized trial 17 that integrates a phase 2 adaptive platform approach for early breast ...
Any variable which does not assign its value to a signal, but only to other variables, is a perfectly acceptable "wire". My understanding of the whole subject is this: A signal assignment inside a process will disregard other signal assignments made in the same process "instantiation".
1. Careful with your terminology. When you say a changed in the other "process", that has a specific meaning in VHDL (process is a keyword in VHDL), and your code does not have any processes. Synthesizers will treat your code as: a <= c and d; b <= (c and d) and c; Simulators will typically assign a in a first pass, then assign b on a second ...