Signal Assignment | LRM §8.4, §9.5. |
A signal assignment statement modifies the target signal

Description:
A Signal assignment statement can appear inside a process (sequential statement) or directly in an architecture (concurrent statement). The target signal can be either a name (simple, selected, indexed, or slice) or an aggregate .
A signal assignment with no delay (or zero delay) will cause an event after delta delay, which means that the event happens only when all of the currently active processes have finished executing (i.e. after one simulation cycle).
The default delay mode ( inertial ) means that pulses shorter than the delay (or the reject period if specified) are ignored. Transport means that the assignment acts as a pure delay line.
VHDL'93 defines the keyword unaffected which indicates a choice where the signal is not given a new assignment. This is roughly equivalent to the use of the null statement within case (see Examples).
- Delays are usually ignored by synthesis tool.
Aggregate , Concurrent statement , Sequential statement , Signal , Variable assignment
VHDL Logical Operators and Signal Assignments for Combinational Logic
In this post, we discuss the VHDL logical operators, when-else statements , with-select statements and instantiation . These basic techniques allow us to model simple digital circuits.
In a previous post in this series, we looked at the way we use the VHDL entity, architecture and library keywords. These are important concepts which provide structure to our code and allow us to define the inputs and outputs of a component.
However, we can't do anything more than define inputs and outputs using this technique. In order to model digital circuits in VHDL, we need to take a closer look at the syntax of the language.
There are two main classes of digital circuit we can model in VHDL – combinational and sequential .
Combinational logic is the simplest of the two, consisting primarily of basic logic gates , such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
Sequential circuits use a clock and require storage elements such as flip flops . As a result, changes in the output are synchronised to the circuit clock and are not immediate. We talk more specifically about modelling combinational logic in this post, whilst sequential logic is discussed in the next post.
Combinational Logic
The simplest elements to model in VHDL are the basic logic gates – AND, OR, NOR, NAND, NOT and XOR.
Each of these type of gates has a corresponding operator which implements their functionality. Collectively, these are known as logical operators in VHDL.
To demonstrate this concept, let us consider a simple two input AND gate such as that shown below.
The VHDL code shown below uses one of the logical operators to implement this basic circuit.
Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals. This is roughly equivalent to the = operator in most other programming languages.
In addition to signals, we can also define variables which we use inside of processes. In this case, we would have to use a different assignment operator (:=).
It is not important to understand variables in any detail to model combinational logic but we talk about them in the post on the VHDL process block .
The type of signal used is another important consideration. We talked about the most basic and common VHDL data types in a previous post.
As they represent some quantity or number, types such as real, time or integer are known as scalar types. We can't use the VHDL logical operators with these types and we most commonly use them with std_logic or std_logic_vectors.
Despite these considerations, this code example demonstrates how simple it is to model basic logic gates.
We can change the functionality of this circuit by replacing the AND operator with one of the other VHDL logical operators.
As an example, the VHDL code below models a three input XOR gate.
The NOT operator is slightly different to the other VHDL logical operators as it only has one input. The code snippet below shows the basic syntax for a NOT gate.
- Mixing VHDL Logical Operators
Combinational logic circuits almost always feature more than one type of gate. As a result of this, VHDL allows us to mix logical operators in order to create models of more complex circuits.
To demonstrate this concept, let’s consider a circuit featuring an AND gate and an OR gate. The circuit diagram below shows this circuit.
The code below shows the implementation of this circuit using VHDL.
This code should be easy to understand as it makes use of the logical operators we have already talked about. However, it is important to use brackets when modelling circuits with multiple logic gates, as shown in the above example. Not only does this ensure that the design works as intended, it also makes the intention of the code easier to understand.
- Reduction Functions
We can also use the logical operators on vector types in order to reduce them to a single bit. This is a useful feature as we can determine when all the bits in a vector are either 1 or 0.
We commonly do this for counters where we may want to know when the count reaches its maximum or minimum value.
The logical reduction functions were only introduced in VHDL-2008. Therefore, we can not use the logical operators to reduce vector types to a single bit when working with earlier standards.
The code snippet below shows the most common use cases for the VHDL reduction functions.
Mulitplexors in VHDL
In addition to logic gates, we often use multiplexors (mux for short) in combinational digital circuits. In VHDL, there are two different concurrent statements which we can use to model a mux.
The VHDL with select statement, also commonly referred to as selected signal assignment, is one of these constructs.
The other method we can use to concurrently model a mux is the VHDL when else statement.
In addition to this, we can also use a case statement to model a mux in VHDL . However, we talk about this in more detail in a later post as this method also requires us to have an understanding of the VHDL process block .
Let's look at the VHDL concurrent statements we can use to model a mux in more detail.
VHDL With Select Statement
When we use the with select statement in a VHDL design, we can assign different values to a signal based on the value of some other signal in our design.
The with select statement is probably the most intuitive way of modelling a mux in VHDL.
The code snippet below shows the basic syntax for the with select statement in VHDL.
When we use the VHDL with select statement, the <mux_out> field is assigned data based on the value of the <address> field.
When the <address> field is equal to <address1> then the <mux_out> signal is assigned to <a>, for example.
We use the the others clause at the end of the statement to capture instance when the address is a value other than those explicitly listed.
We can exclude the others clause if we explicitly list all of the possible input combinations.
- With Select Mux Example
Let’s consider a simple four to one multiplexer to give a practical example of the with select statement. The output Q is set to one of the four inputs (A,B, C or D) depending on the value of the addr input signal.
The circuit diagram below shows this circuit.
This circuit is simple to implement using the VHDL with select statement, as shown in the code snippet below.
VHDL When Else Statements
We use the when statement in VHDL to assign different values to a signal based on boolean expressions .
In this case, we actually write a different expression for each of the values which could be assigned to a signal. When one of these conditions evaluates as true, the signal is assigned the value associated with this condition.
The code snippet below shows the basic syntax for the VHDL when else statement.
When we use the when else statement in VHDL, the boolean expression is written after the when keyword. If this condition evaluates as true, then the <mux_out> field is assigned to the value stated before the relevant when keyword.
For example, if the <address> field in the above example is equal to <address1> then the value of <a> is assigned to <mux_out>.
When this condition evaluates as false, the next condition in the sequence is evaluated.
We use the else keyword to separate the different conditions and assignments in our code.
The final else statement captures the instances when the address is a value other than those explicitly listed. We only use this if we haven't explicitly listed all possible combinations of the <address> field.
- When Else Mux Example
Let’s consider the simple four to one multiplexer again in order to give a practical example of the when else statement in VHDL. The output Q is set to one of the four inputs (A,B, C or D) based on the value of the addr signal. This is exactly the same as the previous example we used for the with select statement.
The VHDL code shown below implements this circuit using the when else statement.
- Comparison of Mux Modelling Techniques in VHDL
When we write VHDL code, the with select and when else statements perform the same function. In addition, we will get the same synthesis results from both statements in almost all cases.
In a purely technical sense, there is no major advantage to using one over the other. The choice of which one to use is often a purely stylistic choice.
When we use the with select statement, we can only use a single signal to determine which data will get assigned.
This is in contrast to the when else statements which can also include logical descriptors.
This means we can often write more succinct VHDL code by using the when else statement. This is especially true when we need to use a logic circuit to drive the address bits.
Let's consider the circuit shown below as an example.
To model this using a using a with select statement in VHDL, we would need to write code which specifically models the AND gate.
We must then include the output of this code in the with select statement which models the multiplexer.
The code snippet below shows this implementation.
Although this code would function as needed, using a when else statement would give us more succinct code. Whilst this will have no impact on the way the device works, it is good practice to write clear code. This help to make the design more maintainable for anyone who has to modify it in the future.
The VHDL code snippet below shows the same circuit implemented with a when else statement.
Instantiating Components in VHDL
Up until this point, we have shown how we can use the VHDL language to describe the behavior of circuits.
However, we can also connect a number of previously defined VHDL entity architecture pairs in order to build a more complex circuit.
This is similar to connecting electronic components in a physical circuit.
There are two methods we can use for this in VHDL – component instantiation and direct entity instantiation .
- VHDL Component Instantiation
When using component instantiation in VHDL, we must define a component before it is used.
We can either do this before the main code, in the same way we would declare a signal, or in a separate package.
VHDL packages are similar to headers or libraries in other programming languages and we discuss these in a later post.
When writing VHDL, we declare a component using the syntax shown below. The component name and the ports must match the names in the original entity.
After declaring our component, we can instantiate it within an architecture using the syntax shown below. The <instance_name> must be unique for every instantiation within an architecture.
In VHDL, we use a port map to connect the ports of our component to signals in our architecture.
The signals which we use in our VHDL port map, such as <signal_name1> in the example above, must be declared before they can be used.
As VHDL is a strongly typed language, the signals we use in the port map must also match the type of the port they connect to.
When we write VHDL code, we may also wish to leave some ports unconnected.
For example, we may have a component which models the behaviour of a JK flip flop . However, we only need to use the inverted output in our design meaning. Therefore, we do not want to connect the non-inverted output to a signal in our architecture.
We can use the open keyword to indicate that we don't make a connection to one of the ports.
However, we can only use the open VHDL keyword for outputs.
If we attempt to leave inputs to our components open, our VHDL compiler will raise an error.
- VHDL Direct Entity Instantiation
The second instantiation technique is known as direct entity instantiation.
Using this method we can directly connect the entity in a new design without declaring a component first.
The code snippet below shows how we use direct entity instantiation in VHDL.
As with the component instantiation technique, <instance_name> must be unique for each instantiation in an architecture.
There are two extra requirements for this type of instantiation. We must explicitly state the name of both the library and the architecture which we want to use. This is shown in the example above by the <library_name> and <architecture_name> labels.
Once the component is instantiated within a VHDL architecture, we use a port map to connect signals to the ports. We use the VHDL port map in the same way for both direct entity and component instantiation.
Which types can not be used with the VHDL logical operators?
Scalar types such as integer and real.
Write the code for a 4 input NAND gate
We can use two different types of statement to model multiplexors in VHDL, what are they?
The with select statement and the when else statement
Write the code for an 8 input multiplexor using both types of statement
Write the code to instantiate a two input AND component using both direct entity and component instantiation. Assume that the AND gate is compiled in the work library and the architecture is named rtl.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
Declaration | ---- used in ----> | Entity Package Process Architecture Procedure Function |
Syntax |
alias_name : alias_type object_name; |
Rules and Examples |
An alias is an alternative name for an existing object (signal, variable or constant). It does not define a new object. |
aliases provide a useful "shorthand" for referencing complex slices etc.: |
An alias of an array object can be indexed in the opposite direction |
Synthesis Issues |
A work-around is to declare new "alias" signals, variables or constants, and assign them with the slice expression. With signals and variables this increases simulation overhead, but preserves readability.
Such "alias" signals should be assigned concurrently, and "alias" variables should be reassigned each time their process is activated.
Whats New in '93 |
Aliases may be applied much more extensively in VHDL -93.
All objects may be aliased, i.e. signals, files, variables and constants.
All "non-objects" can also be aliased, except labels, loop parameters and generate parameters. For instance:
- Product Manual
- Knowledge Base
- Release Notes
- Tech Articles
- Screencasts
Signal Assignments in VHDL: with/select, when/else and case
Sometimes, there is more than one way to do something in VHDL. OK, most of the time , you can do things in many ways in VHDL. Let’s look at the situation where you want to assign different values to a signal, based on the value of another signal.
With / Select
The most specific way to do this is with as selected signal assignment. Based on several possible values of a , you assign a value to b . No redundancy in the code here. The official name for this VHDL with/select assignment is the selected signal assignment .
When / Else Assignment
The construct of a conditional signal assignment is a little more general. For each option, you have to give a condition. This means that you could write any boolean expression as a condition, which give you more freedom than equality checking. While this construct would give you more freedom, there is a bit more redundancy too. We had to write the equality check ( a = ) on every line. If you use a signal with a long name, this will make your code bulkier. Also, the separator that’s used in the selected signal assignment was a comma. In the conditional signal assignment, you need the else keyword. More code for the same functionality. Official name for this VHDL when/else assignment is the conditional signal assignment
Combinational Process with Case Statement
The most generally usable construct is a process. Inside this process, you can write a case statement, or a cascade of if statements. There is even more redundancy here. You the skeleton code for a process (begin, end) and the sensitivity list. That’s not a big effort, but while I was drafting this, I had put b in the sensitivity list instead of a . Easy to make a small misstake. You also need to specify what happens in the other cases. Of course, you could do the same thing with a bunch of IF-statements, either consecutive or nested, but a case statement looks so much nicer.
While this last code snippet is the largest and perhaps most error-prone, it is probably also the most common. It uses two familiar and often-used constructs: the process and the case statements.
Hard to remember
The problem with the selected and conditional signal assignments is that there is no logic in their syntax. The meaning is almost identical, but the syntax is just different enough to throw you off. I know many engineers who permanenty have a copy of the Doulos Golden Reference Guide to VHDL lying on their desks. Which is good for Doulos, because their name gets mentioned all the time. But most people just memorize one way of getting the job done and stick with it.
- VHDL Pragmas (blog post)
- Records in VHDL: Initialization and Constraining unconstrained fields (blog post)
- Finite State Machine (FSM) encoding in VHDL: binary, one-hot, and others (blog post)
- "Use" and "Library" in VHDL (blog post)
- The scope of VHDL use clauses and VHDL library clauses (blog post)
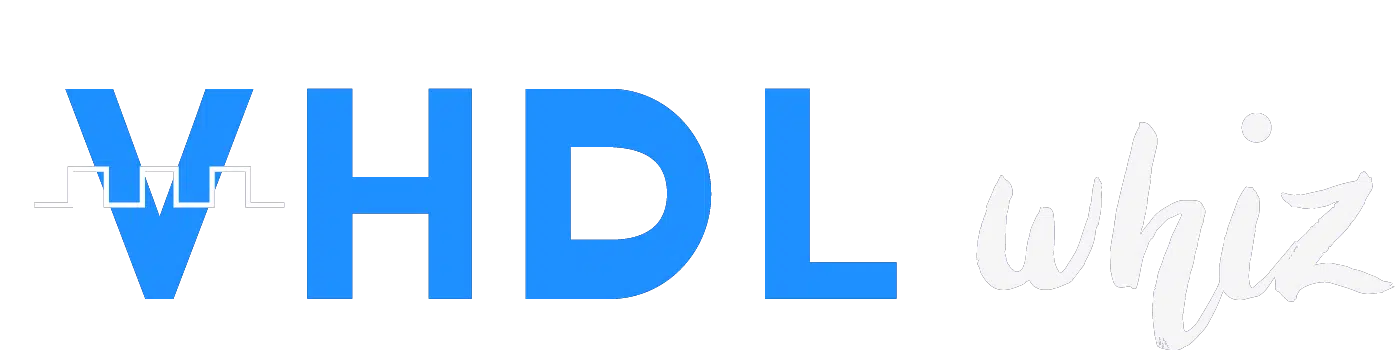
How to create a signal vector in VHDL: std_logic_vector
The std_logic_vector type can be used for creating signal buses in VHDL. The std_logic is the most commonly used type in VHDL, and the std_logic_vector is the array version of it.
While the std_logic is great for modeling the value that can be carried by a single wire, it’s not very practical for implementing collections of wires going to or from components. The std_logic_vector is a composite type, which means that it’s a collection of subelements. Signals or variables of the std_logic_vector type can contain an arbitrary number of std_logic elements.
This blog post is part of the Basic VHDL Tutorials series.
The syntax for declaring std_logic_vector signals is:
where <name> is an arbitrary name for the signal and <initial_value> is an optional initial value. The <lsb> is the index of the least significant bit, and <msb> is the index of the most significant bit.
The to or downto specifies the direction of the range of the bus, basically its endianness. Although both work equally well, it’s most common for VHDL designers to declare vectors using downto . Therefore, I recommend that you always use downto when you declare bit vectors to avoid confusion.
The VHDL code for declaring a vector signal that can hold a byte:
The VHDL code for declaring a vector signal that can hold one bit:
The VHDL code for declaring a vector signal that can hold zero bits (an empty range ):
In this video tutorial, we will learn how to declare std_logic_vector signals and give them initial values. We also learn how to iterate over the bits in a vector using a For-Loop to create a shift register :
The final code we created in this tutorial:
The waveform window in ModelSim after we pressed run, and zoomed in on the timeline:
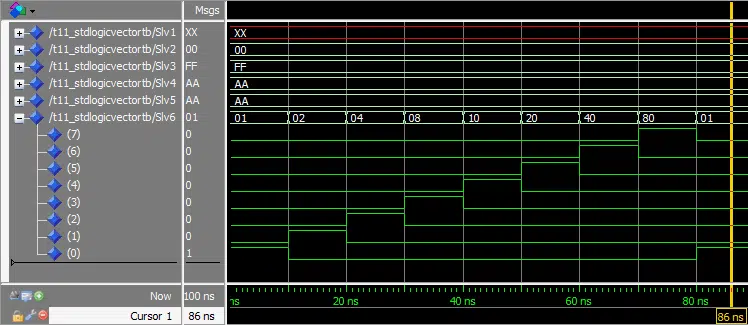
Need the Questa/ModelSim project files?
Let me send you a Zip with everything you need to get started in 30 seconds
By submitting, you consent to receive marketing emails from VHDLwhiz (unsubscribe anytime).
In this exercise we declared six std_logic_vector buses, each eight bits long (one byte).
Signal Slv1 was declared without a initial value. The bus is seen having the value XX in the waveform screenshot. This is because the value that is displayed on the bus is in hexadecimals, and XX indicates a non-hex value. But when we expanded the bus in the waveform, we could see that the individual bits were indeed U’s.
Signal Slv2 was declared using an initial value of all 0’s. Instead of specifying the exact value for each bit, we used (other => '0') in place of the initial value. This is known as an aggregate assignment. The important part is that it will set all bits in the vector to whatever you specify, no matter how long it is.
Signal Slv3 was declared using an aggregate assignment to give all bits the initial value of 1. We can see FF displayed on this signal in the waveform, which is hex for eight 1’s.
Signal Slv4 was declared with an initial value specified in hex, AA. Each hex digit is 4 bits long, therefore we must supply two digits (AA) for our vector which is 8 bits long.
Signal Slv5 declares exactly the same initial value as Slv4 , but now we specified it as the binary value 10101010. We can see from the waveform that both signals have the hex value AA.
Signal Slv6 was declared with an initial value of all zeros, except for the rightmost bit which was '1' . We used a process to create a shift register from this signal. The shift register, as the name implies, shifts the contents of the vector one place to the left every 10 nanoseconds.
Our process wakes up every 10 ns, and the For-Loop shifts all bits in the vector one place to the left. The final bit is shifted back into the first index by the Slv6(Slv6'right) <= Slv6(Slv6'left); statement. In the waveform we can see the '1' ripple through the vector.
By using the 'left' and 'right attributes, we made our code more generic. If we change the width of Sig6 , the process will still work. It’s good design practice to use attributes where you can instead of hardcoding values.
You may be wondering if there are more attributes that you can use, and there are . I won’t be talking more about them in this tutorial series, because I consider them to be advanced VHDL features.
Get free access to the Basic VHDL Course
Download the course material and get started.
You will receive a Zip with exercises for the 23 video lessons as VHDL files where you fill in the blanks, code answers, and a link to the course.
- N-bit vectors should be declared using std_logic_vector(N-1 downto 0)
- A vector can be assigned as a whole or bits within it can be accessed individually
- All bits in a vector can be zeroed by using the aggregate assignment (others => '0')
- Code can be made more generic by using attributes like 'left and 'right
Take the Basic VHDL Quiz – part 2 » or Go to the next tutorial »
I’m from Norway, but I live in Bangkok, Thailand. Before I started VHDLwhiz, I worked as an FPGA engineer in the defense industry. I earned my master’s degree in informatics at the University of Oslo.
Similar Posts
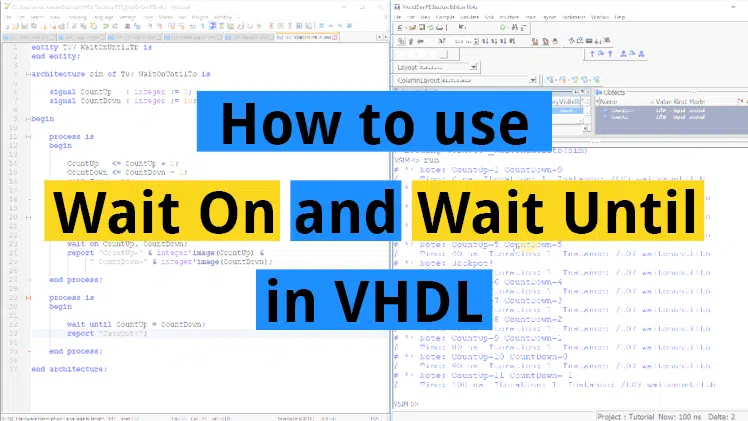
How to use Wait On and Wait Until in VHDL
In the previous tutorial we learned the main differences between signals and variables. We learned that signals have a broader scope than variables, which are only accessible within one process. So how can we use signals for communication between several processes? We have already learned to use wait; to wait infinitely, and wait for to…
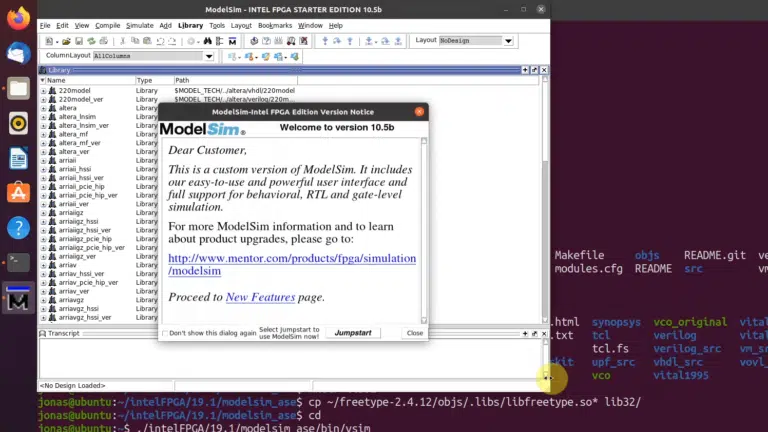
How to make ModelSim from Quartus Prime Lite work on Ubuntu 20.04
The ModelSim version that comes with Intel Quartus Prime Lite Edition is a good alternative if you want to try out VHDL simulation on your home computer. The software is available for both Windows and Linux, but Intel only supports Red Hat-based distros like CentOS Linux. Fortunately, you are just a few hacks away from…
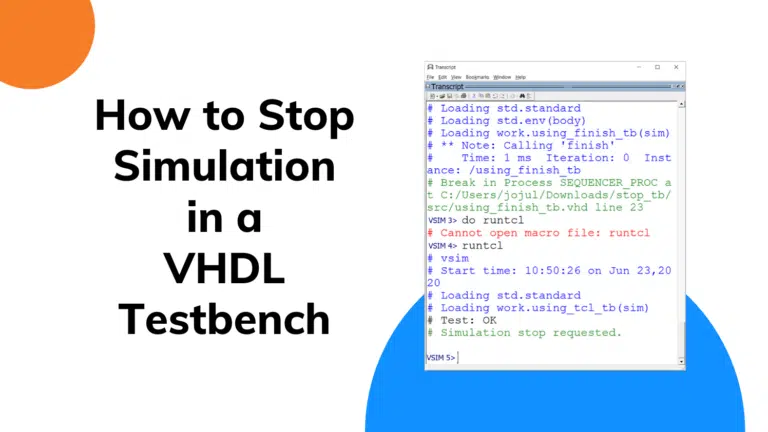

How to stop simulation in a VHDL testbench
How do you stop the VHDL simulator when the simulation is complete? There are several ways to do that. In this article, we will examine the most common ways to end a successful testbench run. The VHDL code presented here is universal, and it should work in any capable VHDL simulator. For the methods involving…
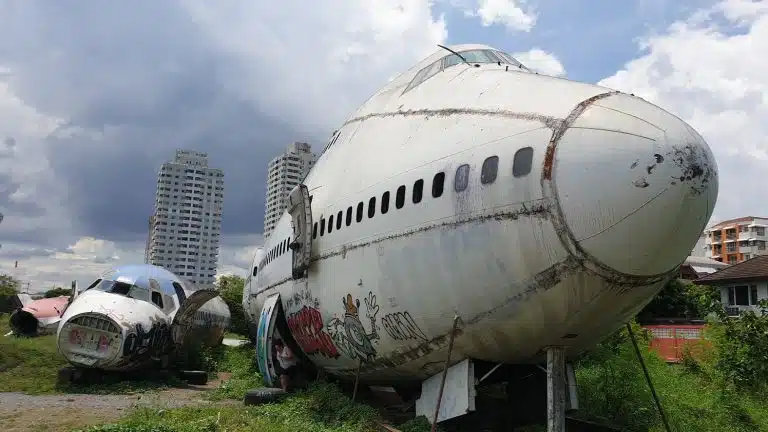
BMP file bitmap image read using TEXTIO
Converting the image file to a bitmap format makes for the easiest way to read a picture using VHDL. Support for the BMP raster graphics image file format is built into the Microsoft Windows operating system. That makes BMP a suitable image format for storing photos for use in VHDL testbenches. In this article, you…
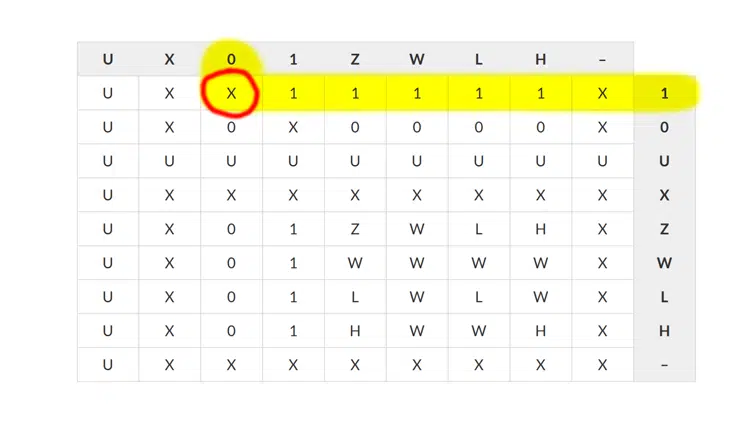
std_logic vs std_ulogic
VHDL includes few built-in types but offers several additional types through extension packages. Two of the most widely used types are std_logic and std_ulogic. The difference between them is that the former is resolved while the latter isn’t. Before we go on to investigate what it means that a type is resolved, let’s first look…
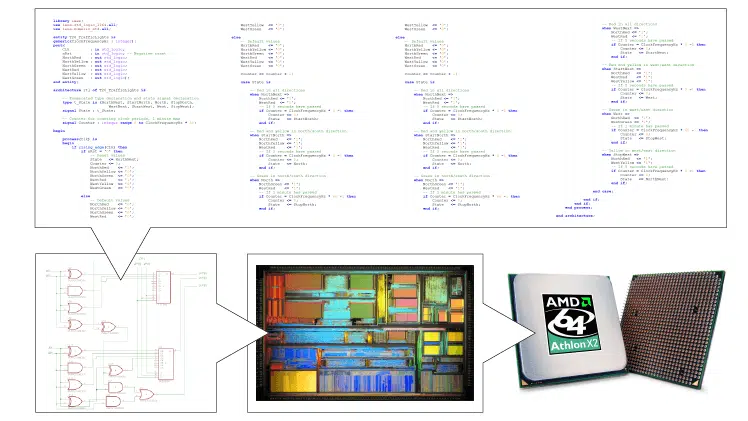
What is VHDL?
In short, VHDL is a computer language used for designing digital circuits. I use the term “computer language” to distinguish VHDL from other, more common programming languages like Java or C++. But is VHDL a programming language? Yes, it is. It’s a programming language that is of no use when it comes to creating computer…
18 Comments
Hi! Excellent article. I have a question, why would you ever use std_logic_vector(0 downto 0) instead of just std_logic?
I have seen std_logic_vector(0 downto 0) being used for mapping a std_logic to a module that has a vector with generic width as an input. Consider this port declaration:
If we want to use a std_logic as input here, we need to first convert it to a std_logic_vector .
In deep logic where generics are used, you may also encounter the zero-width bus. That is, when generics causes a bus to evaluate to this: std_logic_vector(-1 downto 0) . It is legal, but it is also a source of synthesis warnings. For example this warning message from Vivado:
"WARNING: [Synth 8-3919] null assignment ignored"
Hey, I would like to thank you for these tutorials, it not easy to find short and well-explained VHDL lessons nowadays.
I’m glad you found the tutorials helpful. Stay tuned for more advanced topics about VHDL on the blog in the upcoming weeks and months.
Hi sir, i want to take values to array of bit vector from my input bit stream how can i assign? i can not do it by slicing of my array and assigning input value. then how can i do the same
Hi Archana,
To deserialize data that is arriving over a one-bit interface, you can clock the data into a shift register like I’m doing in this blog post. Read out the data word (or byte) when the shift register is full.
The other method is to assign the received bits directly to the correct index in the receive buffer.
Either way, you will have to count the number of received bits to keep track of the position within the received word. You should implement a simple state machine with a counter to take care of this.
Thank you sir..
I have a signal of type “” type ram is array( 0 to 15) of bit_vector(3 down to 0);”” and i have to read elements from text file to this . how can i do this? i am using Textio library. now i am doing it by creating individual 16 signals of bit_vector(3 down to 0) and read the file text to this from the variable and then assigning four such signal to input of type ram is array( 0 to 15) of bit_vector(3 down to 0); like,
how can i do the same by avoiding these steps?
Oh, I see. You want to initialize a RAM from a text file. In the example below, I am initializing your RAM in the declarative region of the VHDL file by using an impure function.
I’ve used your types and signal names. First, we declare the type. Then, we declare an impure function which reads the bits from the data.txt file. Finally, we use the return value from the function as an initial value for the indata1 RAM signal.
Thank You Sir.
I would also like to say that your website has been very helpful! Thanks for all of your efforts on the website and also for any input on my question below, if you have time.
Is it possible to create a port with mixed inputs and outputs other than using inout? That generates a lot of warnings which doesn’t hurt anything however I usually try to avoid warning.
Hello Steve,
A common way to handle this is to declare a local copy of the output signal. Look at the example below to see what I mean.
Thanks for your reply!
I was hoping to have a single “Bus name” in the entity port list with some of the signals in the bus being inputs and others being outputs.
I might be misinterpreting your response or my question might not be clear. Either way, thanks for your time and if you have any additional suggestions, feel free to post them but if not, no worries. Warnings really don’t matter but I prefer to avoid them.
For example: – CPLD_B2:26 – CPLD_B2:01 are dedicated outputs – CPLD_B2:27 is a dedicated input.
I can assign them “inout” but warnings are generated as follows:
Following 27 pins have no output enable or a GND or VCC output enable Info (169065): Pin CPLD_B2[1] has a permanently enabled output enable File.
Oh, I see. That’s only possible in VHDL-2019. Unfortunately, not all FPGA tools support the newest VHDL revision yet. You can read about the change here: https://vhdlwhiz.com/vhdl-2019/#interfaces
Thanks and keep up the great website!
Could you shed some light on toggling multiple bits in a logic vector at the same time?
Hello Sankalp,
You can use a For loop to flip every bit in the vector:
Would this vhdl code be on an infinite loop? Continuously shifting the 1 left?
Hello Jareb,
I wouldn’t consider the shift register process to be an infinite loop because there is a wait for 10 ns; statement inside of it. But I guess from a software perspective, it is an infinite loop because it never stops.
After the simulator executes the last line of a process without a sensitivity list, it immediately continues from the first line again. That’s why we always need to have a Wait statement inside of the process, or we have to use a sensitivity list.
Try to remove the Wait statement from the process and simulate! You will see ModelSim complaining because it’s an infinite loop that continues without any time passing.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Notify me of replies to my comment via email
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
VHDL assignment slice not implemented?
Is it possible to specify a bit range to be assigned on the left side of the assignment?
eg. R(15 downto 8) <= R(15 downto 8) -D;
The above gives me the compiler error: Error 722: Assignment to a signal slice is not implemented. I've tried Googling the error to no avail.
However this works:
R <= R(15 downto 8) - D;
- Perhaps you'd be willing to tell us which (who's) analyzer is telling you this? A slice name is legal as a target of a signal assignment statement in VHDL. The format looks like that of the PICA VHDL tool, wherein the 722 would represent a line number. – user1155120 Commented Sep 24, 2013 at 18:58
- Apologies for leaving out my environment details. Using DesignWorks Professional 5 on Windows 7. The 722 is the error code. The line number was expressed separately. – cbrad Commented Sep 24, 2013 at 19:36
2 Answers 2
Assignments to slices are allowed. What tool are you using? What is the size of D? Pay attention to result sizes. If the size of D is larger than R, then the result size matches the size of D.
What happens in the following assignments:
If this assignment works, then you need to also slice D:
If this does not get it, what does the rest of the code look like?

- I'm using DesignWorks Professional 5. It's not a size issue. I should have mentioned that the assignment is on a signal variable, not an ordinary variable, which is apparently illegal. – cbrad Commented Sep 24, 2013 at 19:27
The answer pertains to my environment, specifically DesignWorks Professional 5, on Windows 7.
You cannot assign values to a slice of a signal variable bit vector. eg.
You can assign values to a slice of a an ordinary variable bit vector. eg.
Each case attempts to assign 16 bits to a 16 bit variable.
- 1 If that second one compiles, and the first does not, I strongly urge you to get a better synthesis tool. The first should compile, and the second should only compile if you use D(15 downto 0) := X"00"; . – zennehoy Commented Sep 25, 2013 at 9:17
- Maybe the first one does not compile because you are assigning an 8 bit vector X"00" to a 16 bit signal. Try: R(15 downto 0) <= X"0000"; That should absolutely compile. And zennehoy is correct, when assigning variables use the := assignment rather than <=. – Russell Commented Sep 25, 2013 at 12:11
- @Russell: You're right about the vector size. It was incorrect in my above answer, it's correct in my code. Edited now. – cbrad Commented Sep 25, 2013 at 15:26
- @zennehoy: I wish I could, unfortunately I'm stuck with this compiler (reasons withheld). Also you're correct about my incorrect ordinary variable assignment, it should have been ":=" instead of "<=". It's edited now, but still doesn't compile. – cbrad Commented Sep 25, 2013 at 15:27
- @cbrad This is almost certainly a tool issue - it apparently doesn't fully implement the VHDL standard. If you're stuck using that tool, I'm afraid you'll have to work around the problem in some other way. For example, you could create two signals for the upper and lower parts of R, then assign to R at the architecture level: R <= R_top & R_bot; – zennehoy Commented Sep 25, 2013 at 15:37
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged vhdl slice or ask your own question .
- The Overflow Blog
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- Odorless color less , transparent fluid is leaking underneath my car
- How can we speed up the process of returning our lost luggage?
- How many engineers/scientists believed that human flight was imminent as of the late 19th/early 20th century?
- How to change my document's font
- Could a Gamma Ray Burst knock a Space Mirror out of orbit?
- How to normalise note lengths when analysing music
- security concerns of executing mariadb-dump with password over ssh
- A word like "science/scientific" that can be used for ALL academic fields?
- How do I annotate a molecule on Chemfig?
- How to deal with coauthors who just do a lot of unnecessary work and exploration to be seen as hard-working and grab authorship?
- Fast leap year check
- Finding out if a series converges without solving linear recurrence relation
- Stuck as a solo dev
- Arduino Uno Serial.write() how many bits are actually transmitted at once by UART and effect of baudrate on other interrupts
- Where are the DC-3 parked at KOPF?
- Does the science work for why my trolls explode?
- Positivity for the mild solution of a heat equation on the torus
- Will a recent B2 travel to the same location as my F1 college application affect the decision
- Which cartoon episode has Green Lantern hitting Superman with a tennis racket and sending him flying?
- If someone threatens force to prevent another person from leaving, are they holding them hostage?
- 120V on fridge door handle when ground broken
- Convert base-10 to base-0.1
- What's the strongest material known to humanity that we could use to make Powered Armor Plates?
- How would you address the premises of Schellenberg's non-resistant divine hiddenness argument?
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Default assignment in VHDL
I'm a little confuse about this default assignment concept. Normally, in order to avoid a latch, we should explicitly give a value to a signal in every case. For example:
But why in the following process, an else statement is not necessary.
Can anyone help me out? Thanks!

Within your target device, be it FPGA, CPLD, ASIC or PLA, the configurable digital logic is implemented (a) by combinatorial gates or (b) by registers (latches or flip-flops).
Combinatorial logic implements AND, OR, XOR and NOT functions and their derivatives, like mux, NAND, NOR and so on. A combinatorial logic circuit can transform its input pattern to a new output pattern. A combinatorial logic circuit always produces one repeatable output for each combination of its inputs.
A register is a 1-bit memory. It can simply copy its data input to its data output in response to an edge (flip-flop) or level (latch) on its clock input. Registers can also have asynchronous set and reset inputs that change the output without clock changing.
In VHDL, you describe (model) the behaviour you would like and the synthesis software tool tries to make a circuit to do it using the configurable digital logic in your target device. Your VHDL implies the circuit behaviour it wants. The synthesis tool attempts to infer these functions from your VHDL and produce the desired logic circuit.
In your VHDL, combinatorial functions must be implied by concurrent statements or by a process that always produces an output for every combination of its inputs. Registers must be implied by a process that only changes in response to (a) a level or edge event on a single input representing the register clock and (b) one input representing set/reset that loads 1's or 0's (but not other signals) into the output signals.
If your VHDL contains a process that is intended to be combinatorial but does not produce an output for every pattern on its inputs, it will infer that a latch is required because at least one output is being shown to stay the same.
So in your examples, the process you question is implying a D-type Flip-Flop (DFF). Under the conditions that reset is inactive and the clock has not risen, the digital logic register output must stay the same. Accordingly, this process must not contain an 'else' term describing what to do when reset is inactive and clock has not risen.

Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged fpga vhdl or ask your own question .
- The Overflow Blog
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
Hot Network Questions
- How can we speed up the process of returning our lost luggage?
- Offline autocue prog for Windows?
- The walk radical in Traditional Chinese
- Is Entropy time-symmetric?
- Positivity for the mild solution of a heat equation on the torus
- I have been trying to solve this Gaussian integral, which comes up during the perturbation theory
- Can All Truths Be Scientifically Verified?
- How was Adam given the 7 Noahide laws without animals or women in existence?
- How to normalise note lengths when analysing music
- How did people know that the war against the mimics was over?
- how does the US justice system combat rights violations that happen when bad practices are given a new name to avoid old rulings?
- Is Sagittarius A* smaller than we might expect given the mass of the Milky Way?
- How to reply to a revise and resubmit review, saying is all good?
- C++ std::function-like queue
- Do black holes convert 100% of their mass into energy via Hawking radiation?
- What makes amplifiers so expensive?
- What was the newest chess piece
- Can't find AVI Raw output on Blender (not on video editing)
- 120V on fridge door handle when ground broken
- Explicit Examples for Unrestricted Hartree Fock Calculations
- If someone threatens force to prevent another person from leaving, are they holding them hostage?
- I am an imaginary variance
- Is it true that before European modernity, there were no "nations"?
- Why is steaming food faster than boiling it?

IMAGES
VIDEO
COMMENTS
26. I'm developing a little thing in VHDL and am quite new to it. I'm having trouble figuring out how to slice a bigger std_logic_vector into a smaller one. For instance I have 3 signals: signal allparts: std_logic_vector(15 downto 0); signal firstpart: std_logic_vector(7 downto 0); signal secondpart: std_logic_vector(7 downto 0);
VHDL SLV dynamic width slice assignment. Ask Question Asked 5 years, 6 months ago. Modified 5 years, 6 months ago. Viewed 922 times 0 \$\begingroup\$ I have some code that takes in 2^X samples and outputs 2^Y samples, where X and Y are integers and Y is less than X. So for example X=6,Y=4, I ingest 64 samples and output 16 samples.
Essential VHDL. 7. This text is about starting to learn how to design large digital systems. A large part of that activity is knowing how to use and apply electronic design automation tools including hardware description languages (HDLs) for design specification, simulation, and synthesis. This chapter describes the essential VHDL needed for ...
A Signal assignment statement can appear inside a process (sequential statement) or directly in an architecture (concurrent statement). The target signal can be either a name (simple, selected, indexed, or slice) or an aggregate. A signal assignment with no delay (or zero delay) will cause an event after delta delay, which means that the event ...
So how should an alias of array slice be created in this case? Declare type array_1_t as an unbounded array type so you can constrain it in the aliases: library ieee; use ieee.std_logic_1164.all; package alias_slice_pkg is type array_1_t is array (natural range <>) of std_logic_vector(31 downto 0); signal my_array_1: array_1_t(0 to 73); alias ...
The VHDL code shown below uses one of the logical operators to implement this basic circuit. and_out <= a and b; Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals.
Synthesis Issues. Aliases are not supported by many logic synthesis tools. A work-around is to declare new "alias" signals, variables or constants, and assign them with the slice expression. With signals and variables this increases simulation overhead, but preserves readability. Such "alias" signals should be assigned concurrently, and "alias ...
\$\begingroup\$ If doing anything critical I usually prefer to make direct explicit assignments for all possibilities so there is no way the synthesizer could misinterpret what I intended ... which is possibly one of the strictest VHDL compilers according to standards ... Why does VHDL not allow to alias slice of an array in this way? 0.
With / Select. The most specific way to do this is with as selected signal assignment. Based on several possible values of a, you assign a value to b. No redundancy in the code here. The official name for this VHDL with/select assignment is the selected signal assignment. with a select b <= "1000" when "00", "0100" when "01", "0010" when "10 ...
The std_logic_vector type can be used for creating signal buses in VHDL. The std_logic is the most commonly used type in VHDL, and the std_logic_vector is the array version of it.. While the std_logic is great for modeling the value that can be carried by a single wire, it's not very practical for implementing collections of wires going to or from components.
Why does my VHDL array slice assignment inside of a loop not give the... If you assign an array slice using a loop and assign other elements in a different process then you may see that the elements assigned outside of the loop result in 'X' or 'U' in order to comply with.
CAUSE: In an assignment at the specified location in a VHDL Design File you assigned a slice of an array type object to the slice of another array type object. However, as specified in the message text, the number of elements in the two slices are not the same.
VHDL assignment slice not implemented? 2 VHDL- vector slicing. 2 VHDL Dynamic slicing using mathematical expression. 6 signed to std_logic_vector, slice results. 0 VHDL: slice a various part of an array. 2 how to assign a slice of signal to single std logic without looping? 1 ...
The term aggregate mean "collection". That is true for the right hand side of an assignment, but not for the left hand side (LHS). The LHS is the reverse operation - a split operation. It is called decomposition or unpacking. The correct way in the VHDL LRM would be to have a e.g. decomposition BNF rule, that is an alias for the aggregate rule.
LINUX : VIVADO 2020.2 : VHDL 2008. There is a record to define a 'typ_M_AXI_TO_SLAVE' transaction type that contains unconstrained arrays. The type is used on an entity and the unconstrained arrays are elaborated as normal, using generics on the interface. M_AXI_TO_SLAVE : OUT typ_AXI_MASTER_TO_SLAVE(.
The answer pertains to my environment, specifically DesignWorks Professional 5, on Windows 7. You cannot assign values to a slice of a signal variable bit vector. eg. signal R: std_logic_vector(15 downto 0); R(15 downto 0) <= X"0000"; -- will not compile. You can assign values to a slice of a an ordinary variable bit vector. eg.
\$\begingroup\$ VHDL -2008 has unary logical operators. if not (or my_vector) then here relying on an implicit condition operator. See IEEE Std 1076-2008 9. Expressions 9.1 General NOTE 2 (telling us the parentheses are required without resort to parsing the condition expression) and 9.2.9 Condition operator.
(1 downto 0 => '1', others => '0') and (0 to 1 => '1', others => '0') are called anonymous aggregates. They have no type by themselves. When the synthesizer sees downto, it creates a (7 downto 0) aggregate which it fills with 00000011.. When it sees to, it creates a (0 to 7) aggregate which it fills with 1100000.. Then these aggregates are assigned to the constants bit per bit: Left to left ...
In VHDL, you describe (model) the behaviour you would like and the synthesis software tool tries to make a circuit to do it using the configurable digital logic in your target device. Your VHDL implies the circuit behaviour it wants. The synthesis tool attempts to infer these functions from your VHDL and produce the desired logic circuit.