Python Programming

Practice Python Exercises and Challenges with Solutions
Free Coding Exercises for Python Developers. Exercises cover Python Basics , Data structure , to Data analytics . As of now, this page contains 18 Exercises.
What included in these Python Exercises?
Each exercise contains specific Python topic questions you need to practice and solve. These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges.
- All exercises are tested on Python 3.
- Each exercise has 10-20 Questions.
- The solution is provided for every question.
- Practice each Exercise in Online Code Editor
These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Select the exercise you want to solve .
Basic Exercise for Beginners
Practice and Quickly learn Python’s necessary skills by solving simple questions and problems.
Topics : Variables, Operators, Loops, String, Numbers, List
Python Input and Output Exercise
Solve input and output operations in Python. Also, we practice file handling.
Topics : print() and input() , File I/O
Python Loop Exercise
This Python loop exercise aims to help developers to practice branching and Looping techniques in Python.
Topics : If-else statements, loop, and while loop.
Python Functions Exercise
Practice how to create a function, nested functions, and use the function arguments effectively in Python by solving different questions.
Topics : Functions arguments, built-in functions.
Python String Exercise
Solve Python String exercise to learn and practice String operations and manipulations.
Python Data Structure Exercise
Practice widely used Python types such as List, Set, Dictionary, and Tuple operations in Python
Python List Exercise
This Python list exercise aims to help Python developers to learn and practice list operations.
Python Dictionary Exercise
This Python dictionary exercise aims to help Python developers to learn and practice dictionary operations.
Python Set Exercise
This exercise aims to help Python developers to learn and practice set operations.
Python Tuple Exercise
This exercise aims to help Python developers to learn and practice tuple operations.
Python Date and Time Exercise
This exercise aims to help Python developers to learn and practice DateTime and timestamp questions and problems.
Topics : Date, time, DateTime, Calendar.
Python OOP Exercise
This Python Object-oriented programming (OOP) exercise aims to help Python developers to learn and practice OOP concepts.
Topics : Object, Classes, Inheritance
Python JSON Exercise
Practice and Learn JSON creation, manipulation, Encoding, Decoding, and parsing using Python
Python NumPy Exercise
Practice NumPy questions such as Array manipulations, numeric ranges, Slicing, indexing, Searching, Sorting, and splitting, and more.
Python Pandas Exercise
Practice Data Analysis using Python Pandas. Practice Data-frame, Data selection, group-by, Series, sorting, searching, and statistics.
Python Matplotlib Exercise
Practice Data visualization using Python Matplotlib. Line plot, Style properties, multi-line plot, scatter plot, bar chart, histogram, Pie chart, Subplot, stack plot.
Random Data Generation Exercise
Practice and Learn the various techniques to generate random data in Python.
Topics : random module, secrets module, UUID module
Python Database Exercise
Practice Python database programming skills by solving the questions step by step.
Use any of the MySQL, PostgreSQL, SQLite to solve the exercise
Exercises for Intermediate developers
The following practice questions are for intermediate Python developers.
If you have not solved the above exercises, please complete them to understand and practice each topic in detail. After that, you can solve the below questions quickly.
Exercise 1: Reverse each word of a string
Expected Output
- Use the split() method to split a string into a list of words.
- Reverse each word from a list
- finally, use the join() function to convert a list into a string
Steps to solve this question :
- Split the given string into a list of words using the split() method
- Use a list comprehension to create a new list by reversing each word from a list.
- Use the join() function to convert the new list into a string
- Display the resultant string
Exercise 2: Read text file into a variable and replace all newlines with space
Given : Assume you have a following text file (sample.txt).
Expected Output :
- First, read a text file.
- Next, use string replace() function to replace all newlines ( \n ) with space ( ' ' ).
Steps to solve this question : -
- First, open the file in a read mode
- Next, read all content from a file using the read() function and assign it to a variable.
- Display final string
Exercise 3: Remove items from a list while iterating
Description :
In this question, You need to remove items from a list while iterating but without creating a different copy of a list.
Remove numbers greater than 50
Expected Output : -
- Get the list's size
- Iterate list using while loop
- Check if the number is greater than 50
- If yes, delete the item using a del keyword
- Reduce the list size
Solution 1: Using while loop
Solution 2: Using for loop and range()
Exercise 4: Reverse Dictionary mapping
Exercise 5: display all duplicate items from a list.
- Use the counter() method of the collection module.
- Create a dictionary that will maintain the count of each item of a list. Next, Fetch all keys whose value is greater than 2
Solution 1 : - Using collections.Counter()
Solution 2 : -
Exercise 6: Filter dictionary to contain keys present in the given list
Exercise 7: print the following number pattern.
Refer to Print patterns in Python to solve this question.
- Use two for loops
- The outer loop is reverse for loop from 5 to 0
- Increment value of x by 1 in each iteration of an outer loop
- The inner loop will iterate from 0 to the value of i of the outer loop
- Print value of x in each iteration of an inner loop
- Print newline at the end of each outer loop
Exercise 8: Create an inner function
Question description : -
- Create an outer function that will accept two strings, x and y . ( x= 'Emma' and y = 'Kelly' .
- Create an inner function inside an outer function that will concatenate x and y.
- At last, an outer function will join the word 'developer' to it.
Exercise 9: Modify the element of a nested list inside the following list
Change the element 35 to 3500
Exercise 10: Access the nested key increment from the following dictionary
Under Exercises: -
Python Object-Oriented Programming (OOP) Exercise: Classes and Objects Exercises
Updated on: December 8, 2021 | 52 Comments
Python Date and Time Exercise with Solutions
Updated on: December 8, 2021 | 10 Comments
Python Dictionary Exercise with Solutions
Updated on: May 6, 2023 | 56 Comments
Python Tuple Exercise with Solutions
Updated on: December 8, 2021 | 96 Comments
Python Set Exercise with Solutions
Updated on: October 20, 2022 | 28 Comments
Python if else, for loop, and range() Exercises with Solutions
Updated on: September 3, 2024 | 301 Comments
Updated on: August 2, 2022 | 158 Comments
Updated on: September 6, 2021 | 109 Comments
Python List Exercise with Solutions
Updated on: December 8, 2021 | 203 Comments
Updated on: December 8, 2021 | 7 Comments
Python Data Structure Exercise for Beginners
Updated on: December 8, 2021 | 116 Comments
Python String Exercise with Solutions
Updated on: October 6, 2021 | 221 Comments
Updated on: March 9, 2021 | 24 Comments
Updated on: March 9, 2021 | 52 Comments
Updated on: July 20, 2021 | 29 Comments
Python Basic Exercise for Beginners
Updated on: August 29, 2024 | 503 Comments
Useful Python Tips and Tricks Every Programmer Should Know
Updated on: May 17, 2021 | 23 Comments
Python random Data generation Exercise
Updated on: December 8, 2021 | 13 Comments
Python Database Programming Exercise
Updated on: March 9, 2021 | 17 Comments
- Online Python Code Editor
Updated on: June 1, 2022 |
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .

Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python exercises.
Test your Python skills with exercises from all categories:
Get Started
Variable names, multiple variable values, output variable, global variable, slicing strings, modify strings, concatenate strings, format strings, access lists, change lists, add list items, remove list items, list comprehension, access tuples, update tuples, unpack tuples, loop tuples, join tuples, access sets, add set items, remove set items, dictionaries, access dictionaries, change dictionaries, add dictionary items, remove dictionary items, loop dictionaries, copy dictionaries, nested dictionaries, while loops, inheritance, polymorphism, string formatting, write to file, remove file, log in to track your progress.
If you haven't already, sign up to become a W3Schooler, and get points for every exercise you complete.
As a logged on W3Schools user you will have access to many features like having your own web page , track your learning progress , receive personal guided paths , and more .
The Exercise
The exercises are a mix of "multiple choice" and "fill in the blanks" questions. There are between 3 and 9 questions in each category. The answer can be found in the corresponding tutorial chapter. If you're stuck, or answer wrong, you can try again or hit the "Show Answer" button to see the correct answer.
Kickstart your career
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
35 Python Programming Exercises and Solutions
To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.
1. Python program to check whether the given number is even or not.
2. python program to convert the temperature in degree centigrade to fahrenheit, 3. python program to find the area of a triangle whose sides are given, 4. python program to find out the average of a set of integers, 5. python program to find the product of a set of real numbers, 6. python program to find the circumference and area of a circle with a given radius, 7. python program to check whether the given integer is a multiple of 5, 8. python program to check whether the given integer is a multiple of both 5 and 7, 9. python program to find the average of 10 numbers using while loop, 10. python program to display the given integer in a reverse manner, 11. python program to find the geometric mean of n numbers, 12. python program to find the sum of the digits of an integer using a while loop, 13. python program to display all the multiples of 3 within the range 10 to 50, 14. python program to display all integers within the range 100-200 whose sum of digits is an even number, 15. python program to check whether the given integer is a prime number or not, 16. python program to generate the prime numbers from 1 to n, 17. python program to find the roots of a quadratic equation, 18. python program to print the numbers from a given number n till 0 using recursion, 19. python program to find the factorial of a number using recursion, 20. python program to display the sum of n numbers using a list, 21. python program to implement linear search, 22. python program to implement binary search, 23. python program to find the odd numbers in an array, 24. python program to find the largest number in a list without using built-in functions, 25. python program to insert a number to any position in a list, 26. python program to delete an element from a list by index, 27. python program to check whether a string is palindrome or not, 28. python program to implement matrix addition, 29. python program to implement matrix multiplication, 30. python program to check leap year, 31. python program to find the nth term in a fibonacci series using recursion, 32. python program to print fibonacci series using iteration, 33. python program to print all the items in a dictionary, 34. python program to implement a calculator to do basic operations, 35. python program to draw a circle of squares using turtle.
For practicing more such exercises, I suggest you go to hackerrank.com and sign up. You’ll be able to practice Python there very effectively.
I hope these exercises were helpful to you. If you have any doubts, feel free to let me know in the comments.
12 thoughts on “ 35 Python Programming Exercises and Solutions ”
I don’t mean to nitpick and I don’t want this published but you might want to check code for #16. 4 is not a prime number.
You can only check if integer is a multiple of 35. It always works the same – just multiply all the numbers you need to check for multiplicity.
v_str = str ( input(‘ Enter a valid string or number :- ‘) ) v_rev_str=” for v_d in v_str: v_rev_str = v_d + v_rev_str
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Recent Posts
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Different Forms of Assignment Statements in Python
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Similar Reads
- Python Programs
- python-basics
Please Login to comment...
- Best Free Workout Apps in 2024: Top-Rated Fitness and Exercise Apps for Home and Gym
- Top 10 Xfinity Alternatives & Competitors in 2024
- Top VMware Alternatives 2024
- Top Airbnb Alternatives in 2024
- GeeksforGeeks Practice - Leading Online Coding Platform
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Python Home
- ▼Python Exercises
- Exercises Home
- ▼Python Basic
- Basic - Part-I
- Basic - Part-II
- Python Programming Puzzles
- Mastering Python
- ▼Python Advanced
- Python Advanced Exercises
- ▼Python Control Flow
- Condition Statements and Loops
- ▼Python Data Types
- List Advanced
- Collections
- ▼Python Class
- ▼Python Concepts
- Python Unit test
- Python Exception Handling
- Python Object-Oriented Programming
- ▼Functional Programming
- Filter Function
- ▼Date and Time
- Pendulum Module
- ▼File Handling
- CSV Read, Write
- ▼Regular Expressions
- Regular Expression
- ▼Data Structures and Algorithms
- Search and Sorting
- Linked List
- Binary Search Tree
- Heap queue algorithm
- ▼Advanced Python Data Types
- Boolean Data Type
- None Data Type
- Bytes and Byte Arrays
- Memory Views exercises
- Frozenset Views exercises
- NamedTuple exercises
- OrderedDict exercises
- Counter exercises
- Ellipsis exercises
- ▼Concurrency and Threading
- Asynchronous
- ▼Python Modules
- Operating System Services
- SQLite Database
- ▼Miscellaneous
- Cyber Security
- Generators Yield
- ▼Python GUI Tkinter, PyQt
- Tkinter Home
- Tkinter Basic
- Tkinter Layout Management
- Tkinter Widgets
- Tkinter Dialogs and File Handling
- Tkinter Canvas and Graphics
- Tkinter Events and Event Handling
- Tkinter Custom Widgets and Themes
- Tkinter File Operations and Integration
- PyQt Widgets
- PyQt Connecting Signals to Slots
- PyQt Event Handling
- ▼Python NumPy
- Python NumPy Home
- ▼Python urllib3
- Python urllib3 Home
- ▼Python Metaprogramming
- Python Metaprogramming Home
- ▼Python GeoPy
- Python GeoPy Home
- ▼BeautifulSoup
- BeautifulSoup Home
- ▼Arrow Module
- ▼Python Pandas
- Python Pandas Home
- ▼Pandas and NumPy Exercises
- Pandas and NumPy Home
- ▼Python Machine Learning
- Machine Learning Home
- TensorFlow Basic
- ▼Python Web Scraping
- Web Scraping
- ▼Python Challenges
- Challenges-1
- ▼Python Mini Project
- Python Projects
- ▼Python Natural Language Toolkit
- Python NLTK
- ▼Python Project
- Novel Coronavirus (COVID-19)
- ..More to come..
- Python Exercises, Practice, Solution
What is Python language?
Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
Python supports multiple programming paradigms, including object-oriented, imperative and functional programming or procedural styles. It features a dynamic type system and automatic memory management and has a large and comprehensive standard library
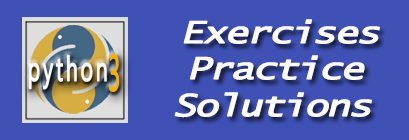
The best way we learn anything is by practice and exercise questions. We have started this section for those (beginner to intermediate) who are familiar with Python.
Hope, these exercises help you to improve your Python coding skills. Currently, following sections are available, we are working hard to add more exercises .... Happy Coding!
You may read our Python tutorial before solving the following exercises.
Latest Articles : Python Interview Questions and Answers Python PyQt
List of Python Exercises :
- Python Basic (Part -I) [ 150 Exercises with Solution ]
- Python Basic (Part -II) [ 150 Exercises with Solution ]
- Python Programming Puzzles [ 100 Exercises with Solution ]
- Mastering Python [ 150 Exercises with Solution ]
- Python Advanced [ 15 Exercises with Solution ]
- Python Conditional statements and loops [ 44 Exercises with Solution]
- Recursion [ 11 Exercises with Solution ]
- Python Data Types - String [ 113 Exercises with Solution ]
- Python JSON [ 9 Exercises with Solution ]
- Python Data Types - List [ 281 Exercises with Solution ]
- Python Data Types - List Advanced [ 15 Exercises with Solution ]
- Python Data Types - Dictionary [ 80 Exercises with Solution ]
- Python Data Types - Tuple [ 33 Exercises with Solution ]
- Python Data Types - Sets [ 30 Exercises with Solution ]
- Python Data Types - Collections [ 36 Exercises with Solution ]
- Python Array [ 24 Exercises with Solution ]
- Python Enum [ 5 Exercises with Solution ]
- Python Class [ 28 Exercises with Solution ]
- Python Unit test [ 10 Exercises with Solution ]
- Python Exception Handling [ 10 exercises with solution ]
- Python Object-Oriented Programming [ 11 Exercises with Solution ]
- Python Decorator [ 12 Exercises with Solution ]
- Python functions [ 21 Exercises with Solution ]
- Python Lambda [ 52 Exercises with Solution ]
- Python Map [ 17 Exercises with Solution ]
- Python Itertools [ 44 exercises with solution ]
- Python - Filter Function [ 11 Exercises with Solution ]
- Python Date Time [ 63 Exercises with Solution ]
- Python Pendulum (DATETIMES made easy) Module [34 Exercises with Solution]
- Python File Input Output [ 21 Exercises with Solution ]
- Python CSV File Reading and Writing [ 11 exercises with solution ]
- Python Regular Expression [ 58 Exercises with Solution ]
- Search and Sorting [ 39 Exercises with Solution ]
- Linked List [ 14 Exercises with Solution ]
- Binary Search Tree [ 6 Exercises with Solution ]
- Python heap queue algorithm [ 29 exercises with solution ]
- Python Bisect [ 9 Exercises with Solution ]
- Python Boolean Data Type [ 10 Exercises with Solution ]
- Python None Data Type [ 10 Exercises with Solution ]
- Python Bytes and Byte Arrays Data Type [ 10 Exercises with Solution ]
- Python Memory Views Data Type [ 10 Exercises with Solution ]
- Python frozenset Views [ 10 Exercises with Solution ]
- Python NamedTuple [ 9 Exercises with Solution ]
- Python OrderedDict [ 10 Exercises with Solution ]
- Python Counter [ 10 Exercises with Solution ]
- Python Ellipsis (...) [ 9 Exercises with Solution ]
- Python Multi-threading and Concurrency [ 7 exercises with solution ]
- Python Asynchronous [ 8 Exercises with Solution ]
- Python built-in Modules [ 31 Exercises with Solution ]
- Python Operating System Services [ 18 Exercises with Solution ]
- Python Math [ 94 Exercises with Solution ]
- Python Requests [ 9 exercises with solution ]
- Python SQLite Database [ 13 Exercises with Solution ]
- Python SQLAlchemy [ 14 exercises with solution ]
- Python - PPrint [ 6 Exercises with Solution ]
- Python - Cyber Security [ 10 Exercises with Solution ]
- Python Generators Yield [ 17 exercises with solution ]
- More to come
Python GUI Tkinter, PyQt
- Python Tkinter Home
- Python Tkinter Basic [ 16 Exercises with Solution ]
- Python Tkinter layout management [ 11 Exercises with Solution ]
- Python Tkinter widgets [ 22 Exercises with Solution ]
- Python Tkinter Dialogs and File Handling [ 13 Exercises with Solution ]
- Python Tkinter Canvas and Graphics [ 14 Exercises with Solution ]
- Python Tkinter Events and Event Handling [ 13 Exercises with Solution ]
- Python Tkinter Customs Widgets and Themes [ 12 Exercises with Solution ]
- Python Tkinter - File Operations and Integration [12 exercises with solution]
- Python PyQt Basic [10 exercises with solution]
- Python PyQt Widgets[12 exercises with solution]
- Python PyQt Connecting Signals to Slots [15 exercises with solution]
- Python PyQt Event Handling [10 exercises with solution]
Python Challenges :
- Python Challenges: Part -1 [ 1- 64 ]
Python Projects :
- Python Numbers : [ 11 Mini Projects with solution ]
- Python Web Programming: [ 12 Mini Projects with solution ]
- 100 Python Projects for Beginners with solution.
- Python Projects: Novel Coronavirus (COVID-19) [ 14 Exercises with Solution ]
Learn Python packages using Exercises, Practice, Solution and explanation
Python urllib3 :
- Python urllib3 [ 26 exercises with solution ]
Python Metaprogramming :
- Python Metaprogramming [ 12 exercises with solution ]
Python GeoPy Package :
- Python GeoPy Package [ 7 exercises with solution ]
Python BeautifulSoup :
- Python BeautifulSoup [ 36 exercises with solution ]
Python Arrow Module :
- Python Arrow Module [ 27 exercises with solution ]
Python Web Scraping :
- Python Web Scraping [ 27 Exercises with solution ]
Python Natural Language Toolkit :
- Python NLTK [ 22 Exercises with solution ]
Python NumPy :
Basic Operations and Arrays
- Python NumPy Basic [ 59 Exercises with Solution ]
- Python NumPy arrays [ 205 Exercises with Solution ]
Mathematics, Linear Algebra, and Statistics
- Python NumPy Mathematics [ 41 Exercises with Solution ]
- Python NumPy Linear Algebra [ 19 Exercises with Solution ]
- Python NumPy Statistics [ 14 Exercises with Solution ]
Random Numbers
- Python NumPy Random [ 17 Exercises with Solution ]
Sorting, Searching, and Indexing
- Python NumPy Sorting and Searching [ 9 Exercises with Solution ]
- NumPy Advanced Indexing [ 20 exercises with solution ]
Datetime and String Operations
- Python NumPy DateTime [ 7 Exercises with Solution ]
- Python NumPy String [ 22 Exercises with Solution ]
Broadcasting and Memory Layout
- NumPy Broadcasting [ 20 exercises with solution ]
- NumPy Memory Layout [ 19 exercises with solution ]
Performance Optimization and Interoperability
- NumPy Performance Optimization [ 20 exercises with solution ]
- NumPy Interoperability [ 20 exercises with solution ]
Input/Output (I/O) Operations
- NumPy I/O Operations [ 20 exercises with solution ]
Functions and Masked Arrays
- NumPy Universal Functions [ 20 exercises with solution ]
- NumPy Masked Arrays [ 20 exercises with solution ]
Structured Arrays and SciPy Integration
- NumPy Structured Arrays [ 20 exercises with solution ]
- NumPy Integration with SciPy [ 19 exercises with solution ]
Advanced Topics and Mastery
- Advanced NumPy [ 33 exercises with solution ]
- Mastering NumPy [ 100 Exercises with Solutions ]
Python Pandas :
- Pandas Data Series [ 40 exercises with solution ]
- Pandas DataFrame [ 81 exercises with solution ]
- Pandas Index [ 26 exercises with solution ]
- Pandas String and Regular Expression [ 41 exercises with solution ]
- Pandas Joining and merging DataFrame [ 15 exercises with solution ]
- Pandas Grouping and Aggregating [ 32 exercises with solution ]
- Pandas Time Series [ 32 exercises with solution ]
- Pandas Filter [ 27 exercises with solution ]
- Pandas GroupBy [ 32 exercises with solution ]
- Pandas Handling Missing Values [ 20 exercises with solution ]
- Pandas Style [ 15 exercises with solution ]
- Pandas Excel Data Analysis [ 25 exercises with solution ]
- Pandas Pivot Table [ 32 exercises with solution ]
- Pandas Datetime [ 25 exercises with solution ]
- Pandas Plotting [ 19 exercises with solution ]
- Pandas Performance Optimization [ 20 exercises with solution ]
- Pandas Advanced Indexing and Slicing [ 15 exercises with solution ]
- Pandas SQL database Queries [ 24 exercises with solution ]
- Pandas Resampling and Frequency Conversion [ 15 exercises with solution ]
- Pandas Advanced Grouping and Aggregation [ 15 exercises with solution ]
- Pandas IMDb Movies Queries [ 17 exercises with solution ]
- Mastering NumPy: 100 Exercises with solutions for Python numerical computing
- Pandas Practice Set-1 [ 65 exercises with solution ]
Pandas and NumPy Exercises :
- Pandas and NumPy for Data Analysis [ 37 Exercises ]
Python Machine Learning :
- Python Machine learning Iris flower data set [38 exercises with solution]
Note : Download Python from https://www.python.org/ftp/python/3.2/ and install in your system to execute the Python programs. You can read our Python Installation on Fedora Linux and Windows 7, if you are unfamiliar to Python installation. You may accomplish the same task (solution of the exercises) in various ways, therefore the ways described here are not the only ways to do stuff. Rather, it would be great, if this helps you anyway to choose your own methods.
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
More to Come !
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
[ Want to contribute to Python exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Test your Python skills with w3resource's quiz
Follow us on Facebook and Twitter for latest update.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/
- Weekly Trends and Language Statistics
Interested in a verified certificate or a professional certificate ?
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and “debug” it. Designed for students with or without prior programming experience who’d like to learn Python specifically. Learn about functions, arguments, and return values (oh my!); variables and types; conditionals and Boolean expressions; and loops. Learn how to handle exceptions, find and fix bugs, and write unit tests; use third-party libraries; validate and extract data with regular expressions; model real-world entities with classes, objects, methods, and properties; and read and write files. Hands-on opportunities for lots of practice. Exercises inspired by real-world programming problems. No software required except for a web browser, or you can write code on your own PC or Mac.
Whereas CS50x itself focuses on computer science more generally as well as programming with C, Python, SQL, and JavaScript, this course, aka CS50P, is entirely focused on programming with Python. You can take CS50P before CS50x, during CS50x, or after CS50x. But for an introduction to computer science itself, you should still take CS50x!
How to Take this Course
Even if you are not a student at Harvard, you are welcome to “take” this course for free via this OpenCourseWare by working your way through the course’s ten weeks of material. For each week, follow this workflow:
And then submit the course’s final project .
To submit the course’s problem sets and final project for feedback, be sure to create an edX account , if you haven’t already. Ask questions along the way via any of the course’s communities !
- If interested in a verified certificate from edX , enroll at cs50.edx.org/python instead.
- If interested in a professional certificate from edX , enroll at cs50.edx.org/programs/python (for Python) or cs50.edx.org/programs/data (for Data Science) instead.
How to Teach this Course
If you are a teacher, you are welcome to adopt or adapt these materials for your own course, per the license . Additionally, we encourage teachers to participate in the CS50 Educator Workshop to learn more about CS50’s curriculum, technology, and pedagogy.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively.
Python Examples
The best way to learn Python is by practicing examples. This page contains examples on basic concepts of Python. We encourage you to try these examples on your own before looking at the solution.
All the programs on this page are tested and should work on all platforms.
Want to learn Python by writing code yourself? Enroll in our Interactive Python Course for FREE.
- Python Program to Check Prime Number
- Python Program to Add Two Numbers
- Python Program to Find the Factorial of a Number
- Python Program to Make a Simple Calculator
- Python Program to Print Hello world!
- Python Program to Find the Square Root
- Python Program to Calculate the Area of a Triangle
- Python Program to Solve Quadratic Equation
- Python Program to Swap Two Variables
- Python Program to Generate a Random Number
- Python Program to Convert Kilometers to Miles
- Python Program to Convert Celsius To Fahrenheit
- Python Program to Check if a Number is Positive, Negative or 0
- Python Program to Check if a Number is Odd or Even
- Python Program to Check Leap Year
- Python Program to Find the Largest Among Three Numbers
- Python Program to Print all Prime Numbers in an Interval
- Python Program to Display the multiplication Table
- Python Program to Print the Fibonacci sequence
- Python Program to Check Armstrong Number
- Python Program to Find Armstrong Number in an Interval
- Python Program to Find the Sum of Natural Numbers
- Python Program to Display Powers of 2 Using Anonymous Function
- Python Program to Find Numbers Divisible by Another Number
- Python Program to Convert Decimal to Binary, Octal and Hexadecimal
- Python Program to Find ASCII Value of Character
- Python Program to Find HCF or GCD
- Python Program to Find LCM
- Python Program to Find the Factors of a Number
- Python Program to Shuffle Deck of Cards
- Python Program to Display Calendar
- Python Program to Display Fibonacci Sequence Using Recursion
- Python Program to Find Sum of Natural Numbers Using Recursion
- Python Program to Find Factorial of Number Using Recursion
- Python Program to Convert Decimal to Binary Using Recursion
- Python Program to Add Two Matrices
- Python Program to Transpose a Matrix
- Python Program to Multiply Two Matrices
- Python Program to Check Whether a String is Palindrome or Not
- Python Program to Remove Punctuations From a String
- Python Program to Sort Words in Alphabetic Order
- Python Program to Illustrate Different Set Operations
- Python Program to Count the Number of Each Vowel
- Python Program to Merge Mails
- Python Program to Find the Size (Resolution) of an Image
- Python Program to Find Hash of File
- Python Program to Create Pyramid Patterns
- Python Program to Merge Two Dictionaries
- Python Program to Safely Create a Nested Directory
- Python Program to Access Index of a List Using for Loop
- Python Program to Flatten a Nested List
- Python Program to Slice Lists
- Python Program to Iterate Over Dictionaries Using for Loop
- Python Program to Sort a Dictionary by Value
- Python Program to Check If a List is Empty
- Python Program to Catch Multiple Exceptions in One Line
- Python Program to Copy a File
- Python Program to Concatenate Two Lists
- Python Program to Check if a Key is Already Present in a Dictionary
- Python Program to Split a List Into Evenly Sized Chunks
- Python Program to Parse a String to a Float or Int
- Python Program to Print Colored Text to the Terminal
- Python Program to Convert String to Datetime
- Python Program to Get the Last Element of the List
- Python Program to Get a Substring of a String
- Python Program to Print Output Without a Newline
- Python Program Read a File Line by Line Into a List
- Python Program to Randomly Select an Element From the List
- Python Program to Check If a String Is a Number (Float)
- Python Program to Count the Occurrence of an Item in a List
- Python Program to Append to a File
- Python Program to Delete an Element From a Dictionary
- Python Program to Create a Long Multiline String
- Python Program to Extract Extension From the File Name
- Python Program to Measure the Elapsed Time in Python
- Python Program to Get the Class Name of an Instance
- Python Program to Convert Two Lists Into a Dictionary
- Python Program to Differentiate Between type() and isinstance()
- Python Program to Trim Whitespace From a String
- Python Program to Get the File Name From the File Path
- Python Program to Represent enum
- Python Program to Return Multiple Values From a Function
- Python Program to Get Line Count of a File
- Python Program to Find All File with .txt Extension Present Inside a Directory
- Python Program to Get File Creation and Modification Date
- Python Program to Get the Full Path of the Current Working Directory
- Python Program to Iterate Through Two Lists in Parallel
- Python Program to Check the File Size
- Python Program to Reverse a Number
- Python Program to Compute the Power of a Number
- Python Program to Count the Number of Digits Present In a Number
- Python Program to Check If Two Strings are Anagram
- Python Program to Capitalize the First Character of a String
- Python Program to Compute all the Permutation of the String
- Python Program to Create a Countdown Timer
- Python Program to Count the Number of Occurrence of a Character in String
- Python Program to Remove Duplicate Element From a List
- Python Program to Convert Bytes to a String
Python Practice for Beginners: 15 Hands-On Problems

- online practice
Want to put your Python skills to the test? Challenge yourself with these 15 Python practice exercises taken directly from our Python courses!
There’s no denying that solving Python exercises is one of the best ways to practice and improve your Python skills . Hands-on engagement with the language is essential for effective learning. This is exactly what this article will help you with: we've curated a diverse set of Python practice exercises tailored specifically for beginners seeking to test their programming skills.
These Python practice exercises cover a spectrum of fundamental concepts, all of which are covered in our Python Data Structures in Practice and Built-in Algorithms in Python courses. Together, both courses add up to 39 hours of content. They contain over 180 exercises for you to hone your Python skills. In fact, the exercises in this article were taken directly from these courses!
In these Python practice exercises, we will use a variety of data structures, including lists, dictionaries, and sets. We’ll also practice basic programming features like functions, loops, and conditionals. Every exercise is followed by a solution and explanation. The proposed solution is not necessarily the only possible answer, so try to find your own alternative solutions. Let’s get right into it!
Python Practice Problem 1: Average Expenses for Each Semester
John has a list of his monthly expenses from last year:
He wants to know his average expenses for each semester. Using a for loop, calculate John’s average expenses for the first semester (January to June) and the second semester (July to December).
Explanation
We initialize two variables, first_semester_total and second_semester_total , to store the total expenses for each semester. Then, we iterate through the monthly_spending list using enumerate() , which provides both the index and the corresponding value in each iteration. If you have never heard of enumerate() before – or if you are unsure about how for loops in Python work – take a look at our article How to Write a for Loop in Python .
Within the loop, we check if the index is less than 6 (January to June); if so, we add the expense to first_semester_total . If the index is greater than 6, we add the expense to second_semester_total .
After iterating through all the months, we calculate the average expenses for each semester by dividing the total expenses by 6 (the number of months in each semester). Finally, we print out the average expenses for each semester.
Python Practice Problem 2: Who Spent More?
John has a friend, Sam, who also kept a list of his expenses from last year:
They want to find out how many months John spent more money than Sam. Use a for loop to compare their expenses for each month. Keep track of the number of months where John spent more money.
We initialize the variable months_john_spent_more with the value zero. Then we use a for loop with range(len()) to iterate over the indices of the john_monthly_spending list.
Within the loop, we compare John's expenses with Sam's expenses for the corresponding month using the index i . If John's expenses are greater than Sam's for a particular month, we increment the months_john_spent_more variable. Finally, we print out the total number of months where John spent more money than Sam.
Python Practice Problem 3: All of Our Friends
Paul and Tina each have a list of their respective friends:
Combine both lists into a single list that contains all of their friends. Don’t include duplicate entries in the resulting list.
There are a few different ways to solve this problem. One option is to use the + operator to concatenate Paul and Tina's friend lists ( paul_friends and tina_friends ). Afterwards, we convert the combined list to a set using set() , and then convert it back to a list using list() . Since sets cannot have duplicate entries, this process guarantees that the resulting list does not hold any duplicates. Finally, we print the resulting combined list of friends.
If you need a refresher on Python sets, check out our in-depth guide to working with sets in Python or find out the difference between Python sets, lists, and tuples .
Python Practice Problem 4: Find the Common Friends
Now, let’s try a different operation. We will start from the same lists of Paul’s and Tina’s friends:
In this exercise, we’ll use a for loop to get a list of their common friends.
For this problem, we use a for loop to iterate through each friend in Paul's list ( paul_friends ). Inside the loop, we check if the current friend is also present in Tina's list ( tina_friends ). If it is, it is added to the common_friends list. This approach guarantees that we test each one of Paul’s friends against each one of Tina’s friends. Finally, we print the resulting list of friends that are common to both Paul and Tina.
Python Practice Problem 5: Find the Basketball Players
You work at a sports club. The following sets contain the names of players registered to play different sports:
How can you obtain a set that includes the players that are only registered to play basketball (i.e. not registered for football or volleyball)?
This type of scenario is exactly where set operations shine. Don’t worry if you never heard about them: we have an article on Python set operations with examples to help get you up to speed.
First, we use the | (union) operator to combine the sets of football and volleyball players into a single set. In the same line, we use the - (difference) operator to subtract this combined set from the set of basketball players. The result is a set containing only the players registered for basketball and not for football or volleyball.
If you prefer, you can also reach the same answer using set methods instead of the operators:
It’s essentially the same operation, so use whichever you think is more readable.
Python Practice Problem 6: Count the Votes
Let’s try counting the number of occurrences in a list. The list below represent the results of a poll where students were asked for their favorite programming language:
Use a dictionary to tally up the votes in the poll.
In this exercise, we utilize a dictionary ( vote_tally ) to count the occurrences of each programming language in the poll results. We iterate through the poll_results list using a for loop; for each language, we check if it already is in the dictionary. If it is, we increment the count; otherwise, we add the language to the dictionary with a starting count of 1. This approach effectively tallies up the votes for each programming language.
If you want to learn more about other ways to work with dictionaries in Python, check out our article on 13 dictionary examples for beginners .
Python Practice Problem 7: Sum the Scores
Three friends are playing a game, where each player has three rounds to score. At the end, the player whose total score (i.e. the sum of each round) is the highest wins. Consider the scores below (formatted as a list of tuples):
Create a dictionary where each player is represented by the dictionary key and the corresponding total score is the dictionary value.
This solution is similar to the previous one. We use a dictionary ( total_scores ) to store the total scores for each player in the game. We iterate through the list of scores using a for loop, extracting the player's name and score from each tuple. For each player, we check if they already exist as a key in the dictionary. If they do, we add the current score to the existing total; otherwise, we create a new key in the dictionary with the initial score. At the end of the for loop, the total score of each player will be stored in the total_scores dictionary, which we at last print.
Python Practice Problem 8: Calculate the Statistics
Given any list of numbers in Python, such as …
… write a function that returns a tuple containing the list’s maximum value, sum of values, and mean value.
We create a function called calculate_statistics to calculate the required statistics from a list of numbers. This function utilizes a combination of max() , sum() , and len() to obtain these statistics. The results are then returned as a tuple containing the maximum value, the sum of values, and the mean value.
The function is called with the provided list and the results are printed individually.
Python Practice Problem 9: Longest and Shortest Words
Given the list of words below ..
… find the longest and the shortest word in the list.
To find the longest and shortest word in the list, we initialize the variables longest_word and shortest_word as the first word in the list. Then we use a for loop to iterate through the word list. Within the loop, we compare the length of each word with the length of the current longest and shortest words. If a word is longer than the current longest word, it becomes the new longest word; on the other hand, if it's shorter than the current shortest word, it becomes the new shortest word. After iterating through the entire list, the variables longest_word and shortest_word will hold the corresponding words.
There’s a catch, though: what happens if two or more words are the shortest? In that case, since the logic used is to overwrite the shortest_word only if the current word is shorter – but not of equal length – then shortest_word is set to whichever shortest word appears first. The same logic applies to longest_word , too. If you want to set these variables to the shortest/longest word that appears last in the list, you only need to change the comparisons to <= (less or equal than) and >= (greater or equal than), respectively.
If you want to learn more about Python strings and what you can do with them, be sure to check out this overview on Python string methods .
Python Practice Problem 10: Filter a List by Frequency
Given a list of numbers …
… create a new list containing only the numbers that occur at least three times in the list.
Here, we use a for loop to iterate through the number_list . In the loop, we use the count() method to check if the current number occurs at least three times in the number_list . If the condition is met, the number is appended to the filtered_list .
After the loop, the filtered_list contains only numbers that appear three or more times in the original list.
Python Practice Problem 11: The Second-Best Score
You’re given a list of students’ scores in no particular order:
Find the second-highest score in the list.
This one is a breeze if we know about the sort() method for Python lists – we use it here to sort the list of exam results in ascending order. This way, the highest scores come last. Then we only need to access the second to last element in the list (using the index -2 ) to get the second-highest score.
Python Practice Problem 12: Check If a List Is Symmetrical
Given the lists of numbers below …
… create a function that returns whether a list is symmetrical. In this case, a symmetrical list is a list that remains the same after it is reversed – i.e. it’s the same backwards and forwards.
Reversing a list can be achieved by using the reverse() method. In this solution, this is done inside the is_symmetrical function.
To avoid modifying the original list, a copy is created using the copy() method before using reverse() . The reversed list is then compared with the original list to determine if it’s symmetrical.
The remaining code is responsible for passing each list to the is_symmetrical function and printing out the result.
Python Practice Problem 13: Sort By Number of Vowels
Given this list of strings …
… sort the list by the number of vowels in each word. Words with fewer vowels should come first.
Whenever we need to sort values in a custom order, the easiest approach is to create a helper function. In this approach, we pass the helper function to Python’s sorted() function using the key parameter. The sorting logic is defined in the helper function.
In the solution above, the custom function count_vowels uses a for loop to iterate through each character in the word, checking if it is a vowel in a case-insensitive manner. The loop increments the count variable for each vowel found and then returns it. We then simply pass the list of fruits to sorted() , along with the key=count_vowels argument.
Python Practice Problem 14: Sorting a Mixed List
Imagine you have a list with mixed data types: strings, integers, and floats:
Typically, you wouldn’t be able to sort this list, since Python cannot compare strings to numbers. However, writing a custom sorting function can help you sort this list.
Create a function that sorts the mixed list above using the following logic:
- If the element is a string, the length of the string is used for sorting.
- If the element is a number, the number itself is used.
As proposed in the exercise, a custom sorting function named custom_sort is defined to handle the sorting logic. The function checks whether each element is a string or a number using the isinstance() function. If the element is a string, it returns the length of the string for sorting; if it's a number (integer or float), it returns the number itself.
The sorted() function is then used to sort the mixed_list using the logic defined in the custom sorting function.
If you’re having a hard time wrapping your head around custom sort functions, check out this article that details how to write a custom sort function in Python .
Python Practice Problem 15: Filter and Reorder
Given another list of strings, such as the one below ..
.. create a function that does two things: filters out any words with three or fewer characters and sorts the resulting list alphabetically.
Here, we define filter_and_sort , a function that does both proposed tasks.
First, it uses a for loop to filter out words with three or fewer characters, creating a filtered_list . Then, it sorts the filtered list alphabetically using the sorted() function, producing the final sorted_list .
The function returns this sorted list, which we print out.
Want Even More Python Practice Problems?
We hope these exercises have given you a bit of a coding workout. If you’re after more Python practice content, head straight for our courses on Python Data Structures in Practice and Built-in Algorithms in Python , where you can work on exciting practice exercises similar to the ones in this article.
Additionally, you can check out our articles on Python loop practice exercises , Python list exercises , and Python dictionary exercises . Much like this article, they are all targeted towards beginners, so you should feel right at home!
You may also like
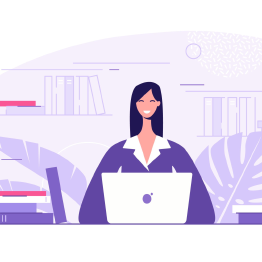
How Do You Write a SELECT Statement in SQL?
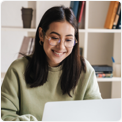
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query

IMAGES
VIDEO
COMMENTS
Coding Exercises with solutions for Python developers. Practice 220+ Python Topic-specific exercises. Solve Python challenges, assignments, programs.
The exercises are a mix of "multiple choice" and "fill in the blanks" questions. There are between 3 and 9 questions in each category. The answer can be found in the corresponding tutorial chapter. If you're stuck, or answer wrong, you can try again or hit the "Show Answer" button to see the correct answer.
Welcome to 101 Exercises for Python Fundamentals. Solving these exercises will help make you a better programmer. Solve them in order, because each solution builds scaffolding, working code,...
35 Python Programming Exercises and Solutions. To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.
List of Python Programming Exercises. In the below section, we have gathered chapter-wise Python exercises with solutions. So, scroll down to the relevant topics and try to solve the Python program practice set. Table of Content. Conditional Statement Exercises. List Exercises. String Exercises. Tuple Exercises. Dictionary Exercises. Set Exercises.
Different Forms of Assignment Statements in Python. We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and “debug” it. Designed for students with or without prior programming experience who’d like to learn Python specifically.
The best way to learn Python is by practicing examples. This page contains examples on basic concepts of Python. We encourage you to try these examples on your own before looking at the solution. All the programs on this page are tested and should work on all platforms.
Improve your Python game with hands-on practice! Solve 15 beginner-friendly exercises and solidify your Python skills.